Using PIP to Install a Specific Version of a Python Package
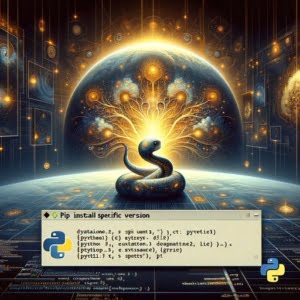
This guide will help you understand how to use pip, Python’s primary package installer, to install specific versions of packages. Mastering this skill allows you to maintain consistency and stability in your Python projects.
Let’s get started by learning pip and how to manage Python package versions effectively!
TL;DR: How do I install a specific version of a Python package using pip?
To install a specific version of a package using pip, use the syntax
pip install package==version
. For example, to install version 1.0.0 of a package named ‘sample’, usepip install sample==1.0.0
. For more advanced methods, background, tips and tricks, continue reading the article.
Table of Contents
Basic Syntax: Installing with Pip
Let’s dive into the basics. The command to install a particular version of a package using pip is pip install package==version
. In this syntax, package
represents the name of the Python package you wish to install, and version
stands for the specific version number. For instance, to install version 1.0.0 of a package named ‘sample’, you would execute the command pip install sample==1.0.0
.
Example of installing a specific version of a package:
pip install sample==1.0.0
Example Specific Version Installs
To provide more clarity, let’s consider a few examples. If you aim to install version 3.7.0 of the ‘requests’ package, the command would be pip install requests==3.7.0
. Similarly, for installing version 2.2.0 of the ‘Django’ package, you would execute pip install Django==2.2.0
.
Example of installing specific versions of packages:
pip install requests==3.7.0
pip install Django==2.2.0
Troubleshooting Installation Errors
While pip is generally reliable, you might occasionally encounter issues when trying to install specific versions of packages.
A common problem arises when attempting to install a version of a package that doesn’t exist, resulting in an error from pip. The solution is to verify the available versions of the package on the Python Package Index (PyPI).
# Attempt to install non-existent version
$ pip install requests==2.999.0
# Error message will be shown
ERROR: Could not find a version that satisfies the requirement requests==2.999.0
ERROR: No matching distribution found for requests==2.999.0
# Verify available versions on PyPI
$ pip install yolk3k==0.9
$ yolk -V requests
# Output lists all available versions
requests 2.23.0
requests 2.22.0
...
In this example, an attempt is made to install a non-existent version (2.999.0
) of the requests
package which results in an error. The yolk3k
package is then installed using pip and used to list all available versions of the requests
package on PyPI. The specific version of requests
package can then be installed based on the available versions.
The Role of PyPI in Pip
PyPI is a pivotal element in package installation. Being the official third-party software repository for Python, it serves as the go-to place to find, download, and install Python packages.
When you use pip to install a package, pip searches PyPI for the package and its specific version. This highlights the importance of ensuring the version you’re trying to install is available on PyPI.
The Importance of Version Control
You might question the need for specific versions. Why not just install the latest version of a package? The answer lies in the fact that different versions of a package can behave differently.
Changes in a package’s code can introduce new features, modify existing ones, or even remove features altogether. By specifying the version of a package you want to install, you can ensure consistency and stability in your project.
This becomes especially crucial when you’re working in a team environment, as it ensures everyone is using the same package versions.
Usage and Relavance of Pip in Python
Pip, an acronym for ‘Pip Installs Packages’, is the standard package manager for Python. It’s a command-line tool that facilitates the installation, upgrade, and removal of Python packages.
Pip interacts with the Python Package Index (PyPI), locates the desired package, and installs it into your Python environment. It’s an indispensable tool in the toolkit of a Python developer.
The Basic Syntax of Pip Install Command
The basic syntax of the pip install command is simple: pip install package-name
. This command instructs pip to download the latest version of the package from PyPI and install it into your Python environment. For instance, to install the latest version of the ‘requests’ package, the command would be pip install requests
.
Advanced Pip Commands and Options
While pip install package==version
is a powerful command, pip offers many more commands and options that can help you manage your Python packages more effectively.
For example, pip list
will show you all the packages installed in your current Python environment, along with their versions. pip show package
will give you more detailed information about a specific package. Meanwhile, pip uninstall package
will remove a package from your environment.
The --upgrade
option can be used with pip install
to upgrade a package to its latest version. For example, pip install --upgrade requests
would upgrade the ‘requests’ package to its latest version.
Example of advanced pip commands:
pip list
pip show requests
pip uninstall requests
pip install --upgrade requests
Managing Packages Across Projects
When you’re working on multiple Python projects, it’s often necessary to use different versions of the same package in different projects. Pip makes this easy by allowing you to specify the version of a package you want to install. You can even use a requirements.txt
file to manage the package versions for each project, ensuring consistency and reproducibility.
Using Requirements.txt for Package Version Management
A requirements.txt
file is a simple text file that lists the packages your Python project depends on, along with their versions. You can create one manually, or you can use the command pip freeze > requirements.txt
to generate one automatically.
Example of using a requirements.txt file:
pip freeze > requirements.txt
pip install -r requirements.txt
This command will list all the packages in your current Python environment, along with their versions, and save it to a requirements.txt
file. You can then use the command pip install -r requirements.txt
to install all the packages listed in the file, ensuring that you have the correct versions for your project.
Virtual Environments and Package Management
Virtual environments are a key tool for managing package versions in Python. A virtual environment is a self-contained Python environment that you can create for each of your projects.
Each virtual environment can have its own set of packages and versions, separate from other virtual environments and your system’s Python environment. This allows you to manage package versions on a per-project basis, ensuring that changes in one project don’t affect others.
Example of creating and using a virtual environment:
python3 -m venv myenv
source myenv/bin/activate
Comparing Python Package Managers
Pip is not the only package manager available for Python. Other popular options include Conda, Pipenv, and Poetry. Each of these tools has its strengths and weaknesses. For example, Conda is particularly good at managing packages for scientific computing, while Pipenv and Poetry provide more advanced features for managing dependencies and virtual environments. However, pip remains the most widely used Python package manager due to its simplicity, flexibility, and integration with PyPI.
Package Manager | Strengths |
---|---|
Pip | Simplicity, flexibility, integration with PyPI |
Conda | Good for scientific computing |
Pipenv | Advanced features for managing dependencies and virtual environments |
Poetry | Advanced features for managing dependencies and virtual environments |
Poetry and Virtual Environments
Poetry is a modern package manager for Python that helps in managing virtual environments and handling package installation seamlessly. It emphasizes simplicity and promotes the best practices of package management in Python.
One of Poetry’s main advantages is its automatic virtual environment handling. Once you start a project with Poetry, it automatically creates and manages a dedicated virtual environment. This ensures that different projects do not interfere with one another in terms of Python versions or dependencies.
Poetry allows you to specify the dependencies of your project in a clear and concise manner, and it simplifies updating or uninstalling packages. It also solves the dependencies intelligently ensuring there is no conflict between them.
Here’s a basic example of how to use Poetry:
# Install Poetry
curl -sSL https://install.python-poetry.org | python -
# Initialize a new Poetry project
poetry new my_poetry_proj
# Navigate to the new project directory
cd my_poetry_proj
# Add a package. This will also create a new virtual environment if one doesn't exist
poetry add requests
# By default, Poetry will pull the highest version available. You can also specify a version:
poetry add [email protected]
# Poetry has effective dependency resolution. If another package needs a different version, it will let you know.
# You can then decide whether you want to install the different version of pandas, use a different package, or figure out another solution.
# Use `poetry run` to run scripts within the context of your Poetry environment
poetry run python your_script.py
With Poetry, you get a powerful tool that aids in not just package management, but also the overall Python project’s workspace organization.
Further Package Manager Resources
Click Here for a Complete Guide on mastering the art of installing, upgrading, and uninstalling packages with “What is Pip?”
For other package management related topics, consider the following resources:
- Python Package Management Made Easy: pip install requirements.txt: Master the art of using “pip install requirements.txt” to ensure smooth package installations in your Python development environment
Uninstalling Python Packages: A Guide to pip Uninstall: Learn how to use “pip uninstall” to remove Python packages with ease.
Apache Spark’s User Guide on Python Packaging: Apache’s official guide on packaging for Python users.
Python Packaging Overview: A comprehensive overview of Python packaging from the official Python Packaging Authority.
Open Source Article on Managing Python Packages: An open-source community article providing insights on Python package management.
Wrapping Up
In this guide, we’ve navigated the intricate world of Python package management, specifically focusing on using pip to install specific versions of Python packages.
We’ve discovered how pip, Python’s standard package manager, empowers us to command our Python environment, ensuring consistency and stability in our projects. We’ve deciphered the syntax for installing specific package versions with pip, ventured into advanced pip usage, and even explored the broader context of Python package management.
But pip is just one gear in the larger machine of Python package management. Other tools like Conda, Pipenv, and Poetry also play a role, and practices like using a requirements.txt
file and virtual environments are invaluable for managing complex Python projects.
In conclusion, mastering pip and understanding the importance of Python package management is a key milestone in your journey to becoming a proficient Python developer. Just like organizing a toolbox, it’s about having the right tools (packages) in the right sizes (versions) for different tasks. And with the right tools and resources, it’s a journey that’s well worth taking.