Pip Upgrade | Guide to Updating Python Packages
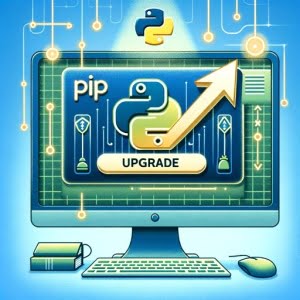
Ever felt stuck with an outdated Python package or an old version of pip? Just like updating your phone’s apps, Python packages and pip itself need regular upgrades for optimal functionality and security.
In this guide, we will walk you through the process of upgrading Python packages using pip, as well as upgrading pip itself. Whether you’re a beginner or an expert, we’ve got you covered.
Let’s start by understanding the basics of pip and Python packages. Then, we’ll dive into the actual upgrade process, complete with code examples and expected outputs. So, let’s get started!
TL;DR: How Do I Upgrade Python Packages and Pip Itself?
To upgrade a Python package, you use the
pip install --upgrade
command followed by the package name. To upgrade pip itself, you usepip install --upgrade pip
. Here’s a quick example:
pip install --upgrade numpy
pip install --upgrade pip
# Output:
# Collecting numpy
# Downloading numpy-1.21.2-cp39-cp39-win_amd64.whl (14.0 MB)
# Installing collected packages: numpy
# Successfully installed numpy-1.21.2
# Requirement already satisfied: pip in ./lib/site-packages (21.2.4)
# Collecting pip
# Downloading pip-21.3-py3-none-any.whl (1.7 MB)
# Installing collected packages: pip
# Successfully uninstalled pip-21.2.4
# Successfully installed pip-21.3
In the above example, we upgraded the numpy package and pip itself. The output shows that the numpy package was successfully installed, and pip was already satisfied but was upgraded to a newer version.
We encourage you to read on for more detailed instructions and advanced usage scenarios.
Table of Contents
The Basics: Python Package Upgrades
The pip install --upgrade
command is the basic command to upgrade Python packages. This command is followed by the name of the package you want to upgrade. Here’s how it works:
pip install --upgrade numpy
# Output:
# Collecting numpy
# Downloading numpy-1.21.2-cp39-cp39-win_amd64.whl (14.0 MB)
# Installing collected packages: numpy
# Successfully installed numpy-1.21.2
In the above example, the numpy package was upgraded. The command first collects the package, downloads the latest version, and then installs it. The output confirms that the numpy package was successfully upgraded to version 1.21.2.
This command is a simple and effective way to keep your Python packages up to date. However, it’s important to note that some packages may have dependencies on other packages, and upgrading one package might affect the functionality of another. Therefore, it’s crucial to understand the dependencies of your packages before upgrading them.
Usage in Other Python Environments
As you progress in your Python journey, you’ll likely find yourself working in different Python environments. Each environment can have its own set of installed packages, and you might need to upgrade packages in these specific environments.
To upgrade packages in a specific environment, you first need to activate that environment. Once the environment is active, you can use the pip install --upgrade
command as usual. Here’s an example:
source activate myenv
pip install --upgrade numpy
# Output:
# (myenv) Collecting numpy
# (myenv) Downloading numpy-1.21.2-cp39-cp39-win_amd64.whl (14.0 MB)
# (myenv) Installing collected packages: numpy
# (myenv) Successfully installed numpy-1.21.2
In this example, we first activated the myenv
environment using the source activate myenv
command. Then, we upgraded the numpy package within this environment. The (myenv)
prefix in the output indicates that the command was run in the myenv
environment.
Working with different environments allows you to maintain separate sets of packages for different projects, which can help prevent conflicts between package versions. However, remember to activate the correct environment before upgrading packages to ensure you’re upgrading the right set of packages.
Alternative Tools: Upgrading Packages
Beyond pip, other Python distribution’s package managers can also be used to upgrade Python packages. One such alternative is Anaconda, a popular Python distribution especially useful for data science and machine learning tasks.
Upgrading Packages with Anaconda
Anaconda uses the conda
command to manage packages. To upgrade a package in Anaconda, you can use the conda update
command followed by the package name. Here’s an example:
conda update numpy
# Output:
# Collecting package metadata (current_repodata.json): done
# Solving environment: done
#
# ## Package Plan ##
#
# environment location: /home/user/anaconda3
#
# added / updated specs:
# - numpy
#
# The following packages will be UPDATED:
#
# numpy 1.20.1-py39hdbf815f_0 --> 1.21.2-py39hdbf815f_0
#
# Proceed ([y]/n)?
In this example, the conda update numpy
command was used to upgrade the numpy package in Anaconda. The output shows that numpy will be updated from version 1.20.1 to 1.21.2.
While Anaconda is a powerful tool, it might be overkill if you’re not using its data science features. Additionally, Anaconda’s package updates can sometimes lag behind pip’s. Therefore, it’s recommended to use Anaconda’s package manager primarily if you’re already using Anaconda for your projects.
While upgrading Python packages and pip, you might encounter some common issues. Let’s discuss a few of these and provide solutions and workarounds.
PermissionError
A PermissionError
typically occurs when pip does not have the necessary permissions to install a package in a directory. This is common when trying to install packages globally on a system.
pip install --upgrade numpy
# Output:
# ERROR: Could not install packages due to an EnvironmentError: [Errno 13] Permission denied: '/usr/local/lib/python3.7/site-packages/numpy'
# Consider using the `--user` option or check the permissions.
In the above example, pip couldn’t upgrade the numpy package due to a PermissionError
. You can resolve this by using the --user
option to install the package in your user directory, or by using a virtual environment.
DistributionNotFound
The DistributionNotFound
error occurs when pip can’t find the package you’re trying to upgrade.
pip install --upgrade non_existent_package
# Output:
# ERROR: Could not find a version that satisfies the requirement non_existent_package (from versions: none)
# ERROR: No matching distribution found for non_existent_package
In this example, pip couldn’t find the non_existent_package
. To resolve this, ensure that you’ve spelled the package name correctly and that the package is available on the Python Package Index (PyPI).
Remember, troubleshooting is a normal part of the development process. Don’t be discouraged by these errors; instead, use them as learning opportunities to deepen your understanding of Python and pip.
Connecting Pip and Python Packages
Before we delve deeper into the upgrade process, let’s take a step back and understand the two key players in our story – pip and Python packages.
What is Pip?
Pip is Python’s package installer. It’s a command-line tool that allows you to install, upgrade, and remove Python packages. You can think of it as an app store for Python – it connects to the Python Package Index (PyPI), fetches the package you want, and installs it on your system.
Here’s a simple example of using pip to install a package:
pip install numpy
# Output:
# Collecting numpy
# Downloading numpy-1.21.2-cp39-cp39-win_amd64.whl (14.0 MB)
# Installing collected packages: numpy
# Successfully installed numpy-1.21.2
In this example, we used pip to install the numpy package. The pip install
command fetched the numpy package from PyPI and installed it on our system.
What are Python Packages?
Python packages are collections of modules – reusable blocks of code that perform a specific task. Packages allow you to organize and reuse code in a modular way, making your programs more manageable and scalable.
For example, the numpy package we installed earlier is a powerful package for numerical computing. It provides features like multi-dimensional arrays and matrices, mathematical functions to operate on these arrays, and much more.
By understanding pip and Python packages, we can better appreciate the importance of keeping our packages up-to-date. Upgrading packages not only ensures we have the latest features and improvements, but it also helps protect our systems from potential security vulnerabilities in older versions.
Relevant Uses of Pip Upgrade
Upgrading Python packages and pip itself is not just a routine task. It has significant implications in various fields, particularly in software development and data science.
In software development, using outdated packages can lead to compatibility issues and security vulnerabilities. Up-to-date packages ensure smoother development process, better performance, and increased security.
In data science, the latest packages often come with improved algorithms, additional features, and bug fixes. Upgrading your Python packages can mean the difference between a good model and a great one.
Expanding Your Horizons
While understanding pip upgrade
is important, there are other related concepts that can further improve your Python experience. For instance, Python virtual environments can help isolate your projects and prevent package conflicts. Python distributions like Anaconda can provide a comprehensive toolkit for data science work.
# To create a virtual environment, you can use the venv module
python -m venv myenv
# To activate the virtual environment
source myenv/bin/activate
# Now you can install packages in this environment without affecting others
pip install numpy
# Output:
# Collecting numpy
# Downloading numpy-1.21.2-cp39-cp39-win_amd64.whl (14.0 MB)
# Installing collected packages: numpy
# Successfully installed numpy-1.21.2
In the above example, we created a virtual environment named myenv
, activated it, and installed the numpy package in it. This numpy installation won’t affect other environments.
Further Package Manager Resources
There are numerous other resources available online to dive deeper into these topics. We have picked out excellent starting points below:
- IOFlood’s guide to Pip Installation and Usage Explores the future of “pip” and its role in the ever-evolving Python ecosystem.
How to pip ‘Update’: Explore the “pip update package” command to keep your Python packages up-to-date effortlessly.
Managing Virtual Environments with pipenv: A Practical Tutorial: Dive deep into “pipenv” and discover how it simplifies dependency management in Python.
ActiveState’s Take on Python Packages is an informative rundown on Python packages.
Quick Read on Dependency Management with pip, also from ActiveState is an efficient guide, quickly explaining dependency management with pip, Python’s package manager.
Environment Management: An in-depth manual from OSDoc providing insights into the management of the computing environment.
Remember, the journey of mastering Python is a marathon, not a sprint. Keep exploring, keep learning!
Recap: Pip Upgrade Python Packages
Throughout this guide, we’ve explored the ins and outs of upgrading Python packages and pip. We’ve learned that the pip install --upgrade
command is our go-to tool for this task, and we’ve seen it in action:
pip install --upgrade numpy
pip install --upgrade pip
# Output:
# Collecting numpy
# Downloading numpy-1.21.2-cp39-cp39-win_amd64.whl (14.0 MB)
# Installing collected packages: numpy
# Successfully installed numpy-1.21.2
# Requirement already satisfied: pip in ./lib/site-packages (21.2.4)
# Collecting pip
# Downloading pip-21.3-py3-none-any.whl (1.7 MB)
# Installing collected packages: pip
# Successfully uninstalled pip-21.2.4
# Successfully installed pip-21.3
We’ve tackled common issues like PermissionError
and DistributionNotFound
, providing solutions and workarounds to keep your upgrade process smooth. We’ve also delved into alternative methods of upgrading packages, such as using Anaconda’s conda update
command.
Understanding how to upgrade Python packages and pip is a crucial skill in your Python journey. However, it’s just one piece of the puzzle. Exploring related concepts like Python virtual environments and Python distributions can open new doors and deepen your Python knowledge.
Remember, the best way to learn is by doing. So, keep experimenting, keep asking questions, and most importantly, keep coding!