Java Syntax Cheat Sheet
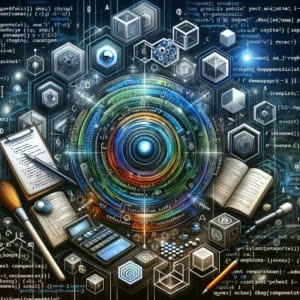
When developing software at IOFLOOD, having a solid understanding of Java syntax and concepts is essential for creating the dedicated cloud hosting ecosystem that meet our clients’ needs. To streamline the learning process and help developers grasp Java fundamentals quickly, we’ve crafted this comprehensive Java Syntax Cheat Sheet.
This reference guide covers all the important uses of Java programming. It serves as a comprehensive Reference for the Java language and syntax, and offers practical code examples.
Let’s embark on this journey to unlock Java’s full potential. Let’s code!
Table of Contents
- Command Reference
- Data Types
- Operators
- String Manipulation
- String Methods
- StringBuilder Methods
- Comments
- Exception Handling
- Common Exceptions
- Access Modifiers
- Control Structures and Loops
- Data Structures
- Data Structure Methods
- Sort Functions
- Method Parameters
- Variable Arguments (VarArgs)
- Casting and Conversion
- Code Examples
- Conclusion
Command Reference
Before providing the code example, we will list all of the essential Java Programming commands and concepts. These terms will be used in action in the code examples following this section. Utilize this reference to learn more about the example code as well as boost your Java development workflow.
Data Types
Data Type | Description | Syntax Example |
---|---|---|
int | Integer data type (whole number) | int i = 10; |
double | Double-precision floating point data type | double d = 20.5; |
boolean | Boolean data type (true or false) | boolean b = true; |
char | Single character data type | char c = ‘A’; |
String | Sequence of characters data type | String s = “Hello”; |
Operators
Relational Operators
Operator | Description | Syntax Example |
---|---|---|
== | Equal to | if (a == b) |
!= | Not equal to | if (a != b) |
> | Greater than | if (a > b) |
< | Less than | if (a < b) |
>= | Greater than or equal to | if (a >= b) |
<= | Less than or equal to | if (a <= b) |
Logical Operators
Operator | Description | Syntax Example |
---|---|---|
&& | Logical AND | if (a > b && c > d) |
| Logical OR | if (a > b || c > d) | ||
! | Logical NOT | if (!a) |
Assignment Operators
Operator | Description | Syntax Example |
---|---|---|
= | Assign value | a = b; |
+= | Add and assign | a += b; |
-= | Subtract and assign | a -= b; |
*= | Multiply and assign | a *= b; |
/= | Divide and assign | a /= b; |
%= | Modulus and assign | a %= b; |
Unary Operators
Operator | Description | Syntax Example |
---|---|---|
++ | Increment operator | a++; |
— | Decrement operator | a–; |
String Manipulation
Concept | Description | Syntax Example |
---|---|---|
Concatenation | Combines strings | String fullName = firstName + ” ” + lastName; |
Comparison | Compares two strings | if (str1.equals(str2)) |
String Methods
Method | Description | Syntax Example |
---|---|---|
length() | Returns the length of the string. | int len = str.length(); |
charAt() | Returns the character at the specified index. | char ch = str.charAt(2); |
contains() | Checks if the string contains the specified sequence of char values. | boolean b = str.contains("abc"); |
equals() | Compares two strings for content equality. | boolean eq = str1.equals(str2); |
equalsIgnoreCase() | Compares two strings, ignoring case considerations. | boolean eq = str1.equalsIgnoreCase(str2); |
toLowerCase() | Converts all characters in the string to lower case. | String lower = str.toLowerCase(); |
toUpperCase() | Converts all characters in the string to upper case. | String upper = str.toUpperCase(); |
trim() | Removes leading and trailing spaces. | String trimmed = str.trim(); |
substring() | Returns a new string that is a substring of this string. | String sub = str.substring(1,4); |
replace() | Replaces each substring of this string that matches the literal target sequence. | String replaced = str.replace('a', 'b'); |
indexOf() | Returns the index within this string of the first occurrence of the specified character. | int index = str.indexOf('a'); |
replaceAll() | Replaces each substring that matches the given regular expression. | String updated = str.replaceAll("text", "txt"); |
split() | Splits this string around matches of the given regular expression. | String[] parts = str.split(" "); |
isEmpty() | Checks if the string is empty. | boolean empty = str.isEmpty(); |
compareTo() | Compares two strings lexicographically. | int diff = str1.compareTo(str2); |
join() | Joins strings with a specified delimiter. | String joined = String.join("-", "Java", "is", "cool"); |
StringBuilder Methods
Method | Description | Example Usage |
---|---|---|
append() | Appends the specified string to this character sequence. | StringBuilder sb = new StringBuilder("Hello"); sb.append(" World"); |
insert() | Inserts the specified string at the specified position. | sb.insert(5, " Dear"); |
delete() | Removes the characters in a substring of this sequence. | sb.delete(5, 10); |
deleteCharAt() | Removes the char at the specified position. | sb.deleteCharAt(5); |
replace() | Replaces the characters in a substring of this sequence with characters in the specified String. | sb.replace(0, 5, "Hi"); |
reverse() | Causes this character sequence to be replaced by the reverse of the sequence. | sb.reverse(); |
toString() | Returns a string representing the data in this sequence. | String s = sb.toString(); |
length() | Returns the length (character count). | int len = sb.length(); |
setCharAt() | Sets the character at the specified position. | sb.setCharAt(0, 'H'); |
substring() | Returns a new String that contains a subsequence of characters currently contained in this sequence. | String sub = sb.substring(1, 4); |
Note: ‘String’ in Java is immutable, once created, it cannot be changed. Meanwhile StringBuilder offers a mutable character sequence, allowing for in-place modifications without creating new objects. This makes StringBuilder more efficient for operations that involve frequent changes to a string’s content.
Comments
Comment Type | Description | Syntax Example |
---|---|---|
Single-line | Used for brief comments, ends at the line break | // This is a single-line comment |
Multi-line | Used for longer comments that span multiple lines | /* This is a multi-line comment that can span multiple lines until it's closed */ |
Documentation | Special comments that can be converted to HTML documentation by the Javadoc tool | /** This is a documentation comment used by Javadoc to generate HTML docs */ |
Exception Handling
Concept | Description | Syntax Example |
---|---|---|
try-catch | Catches exceptions thrown in the try block. | try { ... } catch (ExceptionType name) { ... } |
multiple catch | Handles different types of exceptions separately. | try { ... } catch (ExceptionType1 e1) { ... } catch (ExceptionType2 e2) { ... } |
try-catch-finally | The finally block always executes regardless of exception occurrence. | try { ... } catch (Exception e) { ... } finally { ... } |
try-with-resources | Automatically closes resources used within the try block. | try (ResourceType resource = new ResourceType()) { ... } |
throws | Indicates that a method can throw exceptions of specified types. | public void myMethod() throws IOException, AnotherException { ... } |
throw | Explicitly throws an exception. | throw new ExceptionType("Error message"); |
custom exceptions | Allows defining user-specific exception classes. | class MyException extends Exception { ... } |
Common Exceptions
Exception | Cause | How to Fix |
---|---|---|
NullPointerException | Attempting to use an object reference that is null . | Ensure objects are properly initialized before use. |
ArrayIndexOutOfBoundsException | Accessing an array with an illegal index (either negative or beyond the array size). | Check your indices are within bounds before accessing the array. |
ClassCastException | Trying to cast an object to a subclass of which it is not an instance. | Use instanceof to check type before casting. |
IllegalArgumentException | Passing illegal or inappropriate arguments to a method. | Validate method arguments to ensure they fall within expected ranges or conditions. |
IllegalStateException | When an environment or application is not in an appropriate state for the requested operation. | Ensure all required states or conditions are met before operation. |
IOException | Failure during input or output operations, often due to issues with file or network access. | Check file existence, permissions, or network connectivity before I/O operations. |
FileNotFoundException | Attempting to open a file that does not exist. | Ensure the file exists and the path is correctly specified. |
ArithmeticException | Exceptional arithmetic conditions like divide by zero. | Check arithmetic operations to avoid exceptional conditions (e.g., division by zero). |
Object-Oriented Programming (OOP) Concepts
Concept | Description | Practical Example |
---|---|---|
Class | Blueprint from which individual objects are created. | public class Dog { ... } |
Object | An instance of a class containing state and behavior. | Dog myDog = new Dog(); |
Inheritance | Mechanism where one class acquires properties of another class. | public class GermanShepherd extends Dog { ... } |
Polymorphism | Ability of an object to take on many forms, typically through method overriding. | Overriding the bark() method in GermanShepherd class. |
Abstraction | Hiding the implementation details from the user, showing only functionality. | Declaring abstract class Animal { abstract void makeSound(); } |
Encapsulation | Keeping the data (state) and codes acting on the data (behavior) together, while preventing outside interference and misuse. | Using private variables and public getters and setters. |
Access Modifiers
Modifier | Scope |
---|---|
private | Accessible only within the declared class itself. |
default | Accessible only within classes in the same package. |
protected | Accessible within the same package and subclasses in any package. |
public | Accessible from any other class. |
Note: Access modifiers in Java determine the visibility of classes, methods, and variables within other classes and packages.
Control Structures and Loops
Concept | Description | Example |
---|---|---|
if statement | Executes a block of code if its condition evaluates to true. | if (condition) { ... } |
else statement | Executes a block of code if the if statement’s condition is false. | if (condition) { ... } else { ... } |
else if statement | A series of if statements to execute different blocks of code. | if (condition) { ... } else if (condition) { ... } |
switch statement | Selects one of many code blocks to be executed. | switch(expression) { ... } |
case | A switch block label that specifies a new branch of code to execute. | case x: ... |
default | Specifies the default block of code to execute if no case value matches the switch expression. | default: ... |
break | Terminates the loop or switch statement and transfers execution to the statement immediately following the loop or switch. | break; |
for loop | Executes a block of code a certain number of times. | for (initialization; condition; update) { ... } |
while loop | Repeats a block of code as long as its condition remains true. | while (condition) { ... } |
do-while loop | Executes a block of code once, and then repeats the loop as long as its condition remains true. | do { ... } while (condition); |
Enhanced For Loop | Simplifies the traversal of collections and arrays. | for (Type item : collection) { ... } |
continue (Loop Control) | Skips the current iteration of the loop and proceeds with the next iteration. | Used inside loops as continue; |
Data Structures
Data Structure | Description | Example Usage |
---|---|---|
Array | Stores a fixed-size sequential collection of elements of the same type. | int[] arr = new int[5]; |
ArrayList | A resizable array that grows as needed, from the Java Collections Framework. | ArrayList<String> list = new ArrayList<>(); |
LinkedList | Doubly-linked list implementation of the List and Deque interfaces. | LinkedList<String> linkedList = new LinkedList<>(); |
HashMap | Stores key-value pairs in a hash table. | HashMap<String, Integer> map = new HashMap<>(); |
HashSet | Implements the Set interface, backed by a hash table (actually a HashMap instance). | HashSet<String> set = new HashSet<>(); |
Stack | Last-in-first-out (LIFO) stack of objects. | Stack<Integer> stack = new Stack<>(); |
Queue | Typically orders elements in FIFO (first-in-first-out) manner. | Queue<Integer> queue = new LinkedList<>(); |
Data Structure Methods
Method | Description | Data Structures |
---|---|---|
add() | Adds an element. For queues, offer() might be used instead. | ArrayList, LinkedList, Stack, Queue (as offer()) |
remove() | Removes an element. For queues, poll() might be used instead. | ArrayList, LinkedList, Stack, Queue (as poll()) |
get() | Retrieves an element at a specific position. | ArrayList, LinkedList |
set() | Replaces an element at a specific position. | ArrayList, LinkedList |
push() | Pushes an item onto the top of the stack. | Stack |
pop() | Removes the item at the top of the stack and returns it. | Stack |
peek() | Looks at the object at the top of the stack without removing it. | Stack, Queue |
put() | Associates a specific value with a specific key in a map. | HashMap |
Sort Functions
Sort Algorithm | Description | Complexity |
---|---|---|
Bubble Sort | Repeatedly steps through the list, compares adjacent elements, and swaps them if in the wrong order. | Worst: O(n^2) |
Merge Sort | Divides the array into halves, sorts each half, and merges them back together. | Average: O(n log n) |
Quick Sort | Picks an element as a pivot and partitions the array around the pivot. | Average: O(n log n) |
Insertion Sort | Builds the final sorted array one item at a time, with each item being inserted in its proper place. | Worst: O(n^2) |
Selection Sort | Selects the smallest (or largest) element from the unsorted section and moves it to the beginning (or end). | Worst: O(n^2) |
Method Parameters
Parameter Type | Description | Example Usage |
---|---|---|
Formal Parameters | Parameters defined in the method declaration. They act as placeholders for the values passed to the method when it’s called. | void add(int a, int b) { ... } |
Actual Parameters | The real values passed to the method. These values are assigned to the corresponding formal parameters of the method. | add(5, 10); |
Default Parameters | Java does not support default parameters directly. Default behavior can be simulated using method overloading. | void printMessage(String message) { ... } void printMessage() { printMessage("Default Message"); } |
Variable Arguments (VarArgs) | Allows passing an arbitrary number of values of the specified type to a method. | void printAllStrings(String... strings) { ... } |
Variable Arguments (VarArgs)
Usage Context | Description | Example Usage |
---|---|---|
Simple Collection of Arguments | Passing a variable number of values of the same type to a method. | public void printNumbers(int... numbers) { for (int number : numbers) { System.out.println(number); } } |
Combining VarArgs with Other Parameters | VarArgs can be used alongside other parameters, but VarArgs must be the last parameter. | public void printMixed(String message, int... numbers) { System.out.println(message); for (int number : numbers) { System.out.println(number); } } |
Overloading with VarArgs | Java Methods can be overloaded with VarArgs for more flexible parameter handling. | public void print(); public void print(String... messages); |
Using VarArgs for Formatting | VarArgs are useful for methods that require a format string followed by a variable number of arguments, similar to printf. | public void formatPrint(String format, Object... args) { System.out.printf(format, args); } |
Casting and Conversion
Method/Technique | Description | Example Usage |
---|---|---|
Implicit Casting | Automatic conversion of a smaller type to a larger type. | int i = 100; long l = i; |
Explicit Casting | Manual conversion of a larger type to a smaller type. | long l = 100; int i = (int) l; |
Using Wrapper Classes | Conversion between primitives and their corresponding object types. | int i = 5; Integer integer = Integer.valueOf(i); |
parseXxx() Methods | Converting strings to different data types. | int i = Integer.parseInt("123"); |
toString() Method | Converting other data types to strings. | String s = Integer.toString(123); |
valueOf() Method | Converts strings to wrapper class objects or primitives to wrapper objects. | Integer integer = Integer.valueOf("123"); |
Web Development and Frameworks
Framework | Description | Example Use Cases |
---|---|---|
Servlet API | Low-level API for handling HTTP requests and responses. | Basic web applications, form processing. |
JavaServer Pages (JSP) | Technology that helps software developers create dynamically generated web pages based on HTML, XML, or other document types. | Dynamic content generation, web page templates. |
JavaServer Faces (JSF) | Java specification for building component-based user interfaces for java web applications. | Enterprise-level web applications, form-based applications with complex UI. |
Spring Framework | Comprehensive framework for enterprise Java development, with support for web applications through Spring MVC. | Enterprise applications, RESTful web services, microservices architecture. |
Spring Boot | Convention-over-configuration centric framework from the creators of the Spring Framework, simplifying the setup and development of new Spring applications. | Microservices, standalone web applications, quick project bootstrapping. |
Hibernate | Object-relational mapping tool for Java, facilitating the mapping of an object-oriented domain model to a traditional relational database. | Data persistence and retrieval in web applications, database integration. |
Jersey | Open source framework for developing RESTful Web Services in Java that provides support for JAX-RS APIs. | RESTful services, JSON and XML web services. |
Apache Struts | Framework for creating enterprise-ready Java web applications, based on the Model-View-Controller (MVC) architecture. | Form-based applications, large scale web projects. |
Vaadin | Web application framework for rich Internet applications, focusing on UX accessibility. | Business applications, complex web UIs, single-page applications (SPAs). |
Play Framework | High-velocity web framework for Java and Scala, emphasizes convention over configuration, hot code reloading, and display of errors in the browser. | Modern web applications, reactive applications. |
Java Versions
Version | Release Date | Notable Differences |
---|---|---|
Java SE 8 | March 2014 | Introduced lambda expressions, streams, new date and time API, default and static methods in interfaces. |
Java SE 9 | September 2017 | Introduced the module system (Project Jigsaw), jshell (REPL), private methods in interfaces. |
Java SE 11 | September 2018 | LTS version. New HTTP Client, local-variable syntax for lambda parameters, Flight Recorder, ZGC. |
Java SE 17 | September 2021 | LTS version. Sealed classes, pattern matching for switch (preview), new macOS rendering pipeline, Foreign Function & Memory API (Incubator). |
Note: LTS stands for Long Term Support, indicating a version with extended support and updates.
Code Examples
In this segment, we will delve into a simple Java program designed to showcase how various Java Programming Concepts work together, encompassing everything from basic syntax to more complex concepts such as exception handling and streams. This reference can be used to gain more information about the code examples above.
Before the code examples, we will walk you through setting up a Java environment, in case you don’t have one set up already. The following instructions are for general use, so for OS specific instructions you can check out our Java Installation Guides!
Setting Up The Environment
- Install Java Development Kit (JDK): The JDK is necessary for compiling and running Java applications. If you don’t have it installed, you can download it from the Oracle website or adopt an OpenJDK version from an open-source provider.
Set Up an Integrated Development Environment (IDE): While you can compile and run Java programs from the command line, using an IDE like IntelliJ IDEA, Eclipse, or NetBeans can simplify the process and provide additional tools for debugging and managing your projects. Download and install an IDE of your choice.
Create a New Java Project: Open your IDE and create a new Java project. This process will vary depending on the IDE, but generally, you’ll need to specify a project name and location on your filesystem.
Add Java Classes: Within your new project, create two new Java class files named
JavaSyntaxReference.java
andBook.java
. Copy and paste the provided code for each class into the respective files.Compile and Run: Use your IDE’s built-in features to compile and run the
JavaSyntaxReference
class. This class contains themain
method, which serves as the entry point for the application.
Alternative: Command Line Compilation
For those who prefer working directly from the command line:
- Save the Classes: Save the provided
JavaSyntaxReference.java
andBook.java
code into two separate files within the same directory. Open a Terminal/Command Prompt: Navigate to the directory where you saved the files.
Compile the Classes: Run the command
javac JavaSyntaxReference.java Book.java
to compile both files, producing.class
files runnable on the Java Virtual Machine (JVM).Run the Main Class: Execute the command
java JavaSyntaxReference
to run the compiled main class.
Note: The provided code is designed to be as dependency-free as possible, focusing on raw Java without external libraries. This ensures that a basic Java setup can compile and run the examples with minimal effort.
Sample Class
Before we dive into the main program, we begin with an example Java class. Defining and utilizing classes allows us to encapsulate data and behavior, promoting code reusability and scalability. This class, used in our main sample program, provides an example of Object Oriented Programming, one of the core principles in Java Programming.
public class Book {
// Variables: Define attributes of the Book class
private String title;
private boolean isAvailable;
// Constructor: Initializes a new instance of the Book class
public Book(String title) {
this.title = title;
this.isAvailable = true; // By default, a new book is available
}
// Method: Gets the title of the book
public String getTitle() {
return title;
}
// Method: Checks if the book is available
public boolean isAvailable() {
return isAvailable;
}
// Control Structure (if-else): Borrow the book if it is available
public void borrow() {
if (isAvailable) {
isAvailable = false;
System.out.println(title + " has been borrowed.");
} else {
System.out.println(title + " is not available for borrowing.");
}
}
// Method: Return the book and make it available again
public void returnBook() {
isAvailable = true;
System.out.println(title + " has been returned.");
}
}
// Extending the Book class to create a Magazine subclass
class Magazine extends Book {
public Magazine() {
super("Generic Magazine Title"); // Magazines typically don't have authors like books do, so we use a generic title
}
// Overriding the borrow method to demonstrate polymorphism
@Override
public void borrow() {
if (isAvailable()) {
System.out.println(getTitle() + " has been borrowed. Remember, magazines have a shorter borrowing period!");
} else {
System.out.println(getTitle() + " is not available for borrowing.");
}
}
}
In the main program, we will create an instance of Book, then call its methods to simulate borrowing and returning the book.
Java Sample Code
Presented here is our main sample class, intended to showcase a variety of Java programming techniques. We invite you to explore and study its construction for a hands-on understanding of Java syntax and operations.
public class JavaSyntaxReference {
// Import statements to insure package inclusion
import java.util.Arrays;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Scanner;
import java.io.File;
import java.io.FileWriter;
import java.io.FileReader;
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.IOException;
public static void main(String[] args) {
/* The above line of code is the entry point of a Java application.
"public" makes it accessible from anywhere;
"static" means it belongs to the class, not instances of the class;
"void" indicates it returns no value.
"main" is the name recognized by the JVM as the starting point,
"String[] args" allows the program to accept an array of strings from command line arguments. */
// Data Types
String text = "Hello Java";
double doubleVar = 10.5;
boolean booleanVar = true;
int integerVar = 10;
char charVar = 'A';
System.out.println(integerVar);
// Output: 10
System.out.println(charVar);
// Output: A
// Logical Operators
boolean result = (integerVar < 20) && (doubleVar > 5.0);
System.out.println(result);
// Output: true
// Stack Data Structures
java.util.Stack<Integer> stack = new java.util.Stack<>();
stack.push(10);
System.out.println(stack.peek());
// Output: 10
// ArrayList and an example of an ArrayList method (add(), remove(), get(), set())
ArrayList<String> books = new ArrayList<>();
books.add("Java Basics");
books.set(0, "Advanced Java"); // Replaces "Java Basics" with "Advanced Java"
books.remove("Advanced Java"); // Removes "Advanced Java", the list is now empty
books.add("Java Concurrency"); // Adds "Java Concurrency" to the list
System.out.println("ArrayList example: " + books);
// Output: ArrayList example: [Java Concurrency]
// HashMap and an example of an HashMap method (put(), get(), remove())
HashMap<Integer, String> map = new HashMap<>();
map.put(1, "Java");
map.put(2, "Python");
String language = map.get(1);
map.remove(2);
System.out.println(language);
// Output: Java
// Array Manipulation: Fill an array with a specific value using Arrays.fill()
int[] myArray = new int[5]; // Initializes an array of length 5
Arrays.fill(myArray, 9); // Fills the entire array with 9
System.out.println(Arrays.toString(myArray));
// Output: [9, 9, 9, 9, 9]
// Java Sort Functions - Using Arrays.sort
int[] numbers = {5, 3, 4, 1, 2};
java.util.Arrays.sort(numbers);
System.out.println(Arrays.toString(numbers));
// Output: [1, 2, 3, 4, 5]
// Bubble Sort - Method defined below
int[] numbers = {5, 3, 8, 4, 2};
bubbleSort(numbers);
System.out.println("Bubble Sorted: ");
for (int num : numbers) {
System.out.print(num + " ");
// Expected output: 2 3 4 5 8
}
System.out.println(); // Moves to the next line after printing the array
// Selection Sort - Method defined below
int[] moreNumbers = {22, 11, 99, 88, 9, 7, 42};
selectionSort(moreNumbers);
System.out.println("Selection Sorted: ");
for (int num : moreNumbers) {
System.out.print(num + " ");
// Expected output: 7 9 11 22 42 88 99
}
System.out.println(); // Moves to the next line after printing the array
// Casting or Conversion
double myDouble = 9.78;
int myInt = (int) myDouble; // Explicit casting from double to int
System.out.println(myInt);
// Output: 9
// String Conversions: Using toString(), String.valueOf()
int numForString = 500;
String fromInt = String.valueOf(numForString);
System.out.println("String.valueOf(): " + fromInt);
// Output: String.valueOf(): 500
// StringBuilder
StringBuilder sb = new StringBuilder();
sb.append("StringBuilder ").append("Example");
System.out.println(sb.toString());
// Output: StringBuilder Example
// String Manipulation - String Methods
String text = "Hello Java";
String upperText = text.toUpperCase();
System.out.println(upperText);
// Output: HELLO JAVA
// Java Math Library
double sqrtResult = Math.sqrt(25); // Square root
System.out.println(sqrtResult);
// Output: 5.0
// Java Time Library
java.time.LocalDate currentDate = java.time.LocalDate.now();
System.out.println(currentDate);
// Output: [Current Date]
// Java File Handling
java.io.File myFile = new java.io.File("example.txt");
// Creates a File object that represents a reference to "example.txt" in the file system.
// Appending text to "example.txt" w/ FileWriter
try (FileWriter writer = new FileWriter("example.txt", true)) { // true to append
writer.write("\nI love Java!"); // Appends a new line with the text "I love Java!" to the file.
} catch (IOException e) {
System.out.println("An error occurred while writing to the file: " + e.getMessage());
}
// Reading from "example.txt" using Scanner and printing its contents
try (Scanner scanner = new Scanner(new File("example.txt"))) {
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
System.out.println(line);
/* Expected console output:
Learning Java with IOFlood is a blast!
I love Java! */
}
} catch (FileNotFoundException e) {
System.out.println("File not found: " + e.getMessage());
}
// Reading from "example.txt" using Scanner and printing its contents
try (Scanner scanner = new Scanner(new File("example.txt"))) {
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
System.out.println(line);
/* Expected console output:
Learning Java with IOFlood is a blast!
I love Java! */
}
} catch (FileNotFoundException e) {
System.out.println("File not found: " + e.getMessage());
}
// Java Regular Expressions
String pattern = ".*Java.*";
boolean matches = "Hello Java".matches(pattern);
System.out.println(matches);
// Output: true
// Control Structure: if-else
int a = 5, b = 10;
if (a < b) {
System.out.println("a is less than b");
} else {
System.out.println("a is not less than b");
}
// Output: a is less than b
// Loop: for
for (int i = 0; i < 3; i++) {
System.out.println("for loop iteration: " + i); // Output will show for each iteration
}
// Loop: while
int whileCounter = 3;
while (whileCounter > 0) {
System.out.println("while loop iteration"); // Output shown for each iteration
whileCounter--;
}
// Loop: do-while
int doWhileCounter = 2;
do {
System.out.println("do-while loop iteration");
doWhileCounter--;
} while (doWhileCounter > 0);
/* Expected output:
do-while loop iteration
do-while loop iteration */
// Loop: Enhanced for
int[] numbers = {1, 2, 3};
for (int number : numbers) {
System.out.println("Enhanced for loop: " + number);
}
/* Expected output:
Enhanced for loop: 1
Enhanced for loop: 2
Enhanced for loop: 3 */
// Use of 'continue' in a Java loop
for(int i = 0; i < 5; i++) {
if(i == 2) continue; // Skips the rest of the loop when i is 2
System.out.println(i);
}
/* Expected output:
0
1
3
4 */
// Switch-case
int month = 4;
switch (month) {
case 1:
System.out.println("January");
break;
case 2:
System.out.println("February");
break;
default:
System.out.println("Other Month");
}
// Expected output:
// Other Month
// Simple Method Call - Method defined below
System.out.println("Calling printMessage with a single string:");
printMessage("This is a method call.");
// Output: This is a method call.
//VarArgs Method Call
System.out.println("Calling printVarArgs with multiple strings:");
printVarArgs("VarArgs Example:", "One", "Two", "Three");
/* Output:
VarArgs Example:
One
Two
Three */
// Exception Handling: try-catch
try {
int result = a / 0;
} catch (ArithmeticException e) {
System.out.println("Exception caught: " + e.getMessage());
}
// Expected output:
// Exception caught: / by zero
// Java 8 Stream
int[] streamNumbers = {4, 5, 6};
java.util.Arrays.stream(streamNumbers).forEach(System.out::println);
/* Expected output:
4
5
6 */
// Note: The System.out::println syntax is used in within Java 8 Streams as a method reference,
// a shorthand notation used when passing methods as arguments.
// Object Instantiation with Constructors
Book myFavoriteBook = new Book("The Art of Java");
System.out.println("Book created: " + myFavoriteBook.getTitle());
// Output: Book created: The Art of Java
// Demonstrating Inheritance and Polymorphism
Book myMagazine = new Magazine();
myMagazine.borrow();
// Output: Generic Magazine Title has been borrowed. Remember, magazines have a shorter borrowing period!
// Method invocation: Borrowing the book
System.out.println("Attempting to borrow the book...");
myFavoriteBook.borrow();
// Output: The Art of Java has been borrowed.
// Checking if the book can be borrowed again (condition checking)
System.out.println("Attempting to borrow the book again...");
myFavoriteBook.borrow();
// Output: The Art of Java is not available for borrowing.
// Returning the book
System.out.println("Returning the book...");
myFavoriteBook.returnBook();
// Output: The Art of Java has been returned.
// Attempting to borrow the book after returning it
System.out.println("Attempting to borrow the book after returning...");
myFavoriteBook.borrow();
// Output: The Art of Java has been borrowed.
// Exception Handling with try-catch: Handling a NullPointerException
try {
Book myBook = null; // Intentionally set to null to trigger a NullPointerException
System.out.println(myBook.getTitle()); // Attempting to access a method on a null reference
} catch (NullPointerException e) {
System.out.println("Caught a NullPointerException. Make sure your Book object is initialized before use.");
}
}
// Method Definition
static void printMessage(String message) {
System.out.println(message);
}
// VarArgs Method
static void printVarArgs(String... args) {
for (String arg : args) {
System.out.println(arg);
}
}
// Bubble Sort method
static void bubbleSort(int[] arr) {
boolean swapped;
for (int i = 0; i < arr.length - 1; i++) {
swapped = false; // Track if a swap occurred
// Iterate through the array, excluding the last sorted elements
for (int j = 0; j < arr.length - i - 1; j++) {
// Compare adjacent elements
if (arr[j] > arr[j + 1]) {
// Swap if elements are in wrong order
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
swapped = true; // Mark swap occurred
}
}
// If no swap occurred, the array is sorted
if (!swapped) break;
}
}
// Selection Sort method
static void selectionSort(int[] arr) {
for (int i = 0; i < arr.length - 1; i++) {
int minIdx = i; // Assume current index as minimum
// Find the minimum element in unsorted part
for (int j = i + 1; j < arr.length; j++) {
if (arr[j] < arr[minIdx]) {
minIdx = j; // Update minimum index
}
}
// Swap the found minimum element with the first element
int temp = arr[minIdx];
arr[minIdx] = arr[i];
arr[i] = temp;
}
}
}
Our example code tackles a range of Java fundamentals and advanced concepts, from variable declaration and control structures to method definition and lambda expressions. Remember to utilize the reference section, and other java tutorials for more info on the concepts used in the example!
Conclusion
Java’s versatility and robustness as a programming language underscore the importance of mastering Object-Oriented Programming (OOP) and its concepts. This cheat sheet aims to equip you with a solid understanding of Java syntax and best practices. Whether you’re a novice stepping into the world of programming or a seasoned developer brushing up on Java, this guide is a valuable resource in your journey. We wish you good luck as you continue to excel in the realm of Java programming!