Python Lambda Functions Guide (With Examples)
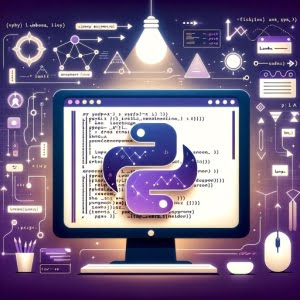
Ever felt bewildered by Python’s lambda functions? You’re not alone. Like a secret agent in the world of Python, they’re often misunderstood, their potential underexplored.
But fear not, this comprehensive guide is here to demystify Python lambda functions for you, whether you’re a beginner just getting started or a seasoned coder looking to refine your skills.
TL;DR: What is a Lambda Function in Python?
A lambda function is a small, anonymous function in Python, defined using the lambda keyword. It’s a handy tool for creating quick, single-use functions without the need for a formal function definition. Here’s a basic example:
add = lambda x, y: x + y
print(add(5, 3))
# Output:
# 8
In this example, we’ve created a lambda function called ‘add’ that takes two arguments, x and y, and returns their sum. We then call this function with the numbers 5 and 3, and it prints out the result, 8.
Intrigued? Keep reading for a more detailed understanding of Python lambda functions and their usage.
Table of Contents
- Python Lambda Functions: The Basics
- Intermediate Python Lambda: Map, Filter, and Reduce
- Python Lambda Alternatives: Regular Functions and List Comprehensions
- Troubleshooting Python Lambda Functions
- Understanding Anonymous Functions and Functional Programming
- Taking Python Lambda Functions Further
- Further Resources for Python Functions
- Python Lambda Functions: A Recap
Python Lambda Functions: The Basics
Lambda functions in Python, also known as anonymous functions, are defined using the lambda keyword. Unlike regular functions, they have no name and are designed to be simple and concise. Let’s dive into how to define and use a lambda function with an example:
multiply = lambda x, y: x * y
print(multiply(4, 2))
# Output:
# 8
In this example, we’ve defined a lambda function called ‘multiply’ that takes two arguments, x and y. It returns the product of these two numbers. We then call this function with the numbers 4 and 2, and it prints out the result, 8.
The structure of a lambda function is straightforward: ‘lambda’ followed by one or more arguments (x, y in our example), a colon, and then the expression to evaluate and return. In our case, that’s x * y
.
The advantages of using lambda functions are their simplicity and conciseness. They’re perfect for small, simple functions where a full function definition would be overkill. However, they can become difficult to read and understand when overused or for complex tasks, so use them judiciously.
Intermediate Python Lambda: Map, Filter, and Reduce
As we delve deeper into the world of Python lambda functions, we find they can be used in conjunction with Python’s built-in functions like map, filter, and reduce. These functions allow you to process and manipulate lists (or other iterable objects) in a concise and efficient manner.
Map with Lambda
The map()
function applies a given function to each item of an iterable and returns a list of the results. Here’s how you can use a lambda function with map()
:
numbers = [1, 2, 3, 4, 5]
doubled = list(map(lambda x: x * 2, numbers))
print(doubled)
# Output:
# [2, 4, 6, 8, 10]
In this example, we have a list of numbers. We use map()
with a lambda function that doubles each number, resulting in a new list of doubled numbers.
Filter with Lambda
The filter()
function constructs a list from elements of an iterable for which a function returns true. Here’s an example with a lambda function:
numbers = [1, 2, 3, 4, 5]
evens = list(filter(lambda x: x % 2 == 0, numbers))
print(evens)
# Output:
# [2, 4]
In this case, we use filter()
with a lambda function that checks if a number is even. This results in a new list that includes only the even numbers.
Reduce with Lambda
The reduce()
function applies a rolling computation to sequential pairs of values in a list. For example, if you wanted to calculate the product of all numbers in a list:
from functools import reduce
numbers = [1, 2, 3, 4, 5]
product = reduce((lambda x, y: x * y), numbers)
print(product)
# Output:
# 120
In this example, we import the reduce()
function from the functools
module and use it with a lambda function that multiplies two numbers. The reduce()
function then applies this lambda function to all items in the list, effectively calculating the product of all numbers in the list.
These examples demonstrate how Python’s lambda functions can be used for more complex tasks, providing a powerful tool in your Python toolkit.
Python Lambda Alternatives: Regular Functions and List Comprehensions
While lambda functions are powerful and concise, they are not the only way to achieve specific results in Python. Regular functions and list comprehensions are two alternatives that can often be used in place of lambda functions.
Regular Functions
If the functionality you are implementing is complex and not easily readable in a single line of code, a regular function might be a better choice. Here’s how you could rewrite our earlier ‘multiply’ lambda function as a regular function:
def multiply(x, y):
return x * y
print(multiply(4, 2))
# Output:
# 8
As you can see, the function performs the same task, but it’s more verbose. However, it can be easier to read and understand, especially for more complex tasks.
List Comprehensions
List comprehensions provide a concise way to create lists based on existing lists. Here’s how you could use a list comprehension to achieve the same result as our ‘map’ example with the lambda function:
numbers = [1, 2, 3, 4, 5]
doubled = [x * 2 for x in numbers]
print(doubled)
# Output:
# [2, 4, 6, 8, 10]
Again, the result is the same, but the code is arguably more readable. List comprehensions are a powerful feature of Python and can often be used in place of map or filter with lambda functions.
While lambda functions, regular functions, and list comprehensions each have their advantages and drawbacks, the best choice depends on the specific task and the readability of the code. As an expert Python programmer, understanding when to use each of these tools is key to writing efficient, readable code.
Troubleshooting Python Lambda Functions
While lambda functions are powerful, they can sometimes lead to unexpected results or syntax errors. Let’s explore some common issues and their solutions.
Syntax Errors
One common issue is a syntax error. This can happen if you forget the colon, use incorrect indentation, or forget to close the parentheses. Here’s an example of a syntax error in a lambda function:
# Incorrect syntax
multiply = lambda x, y x * y
print(multiply(4, 2))
In this example, we forgot the colon after the arguments. Python will raise a SyntaxError
. The correct syntax should be:
# Correct syntax
multiply = lambda x, y: x * y
print(multiply(4, 2))
# Output:
# 8
Unexpected Results
Another common issue is getting unexpected results. This can happen if you don’t fully understand how lambda functions work. For example, you might expect the following code to print the numbers 1 through 5:
# Unexpected results
funcs = [lambda x: x + i for i in range(5)]
for func in funcs:
print(func(0))
However, it will actually print the number 4 five times. This is because the lambda function is capturing the variable i
by reference, not by value. When the lambda function is called, it uses the current value of i
, which is 4 at the end of the list comprehension. To get the expected results, you can use a default argument to capture the current value of i
:
# Expected results
funcs = [lambda x, i=i: x + i for i in range(5)]
for func in funcs:
print(func(0))
# Output:
# 0
# 1
# 2
# 3
# 4
Understanding these common issues and how to solve them can help you avoid pitfalls and use Python lambda functions more effectively.
Understanding Anonymous Functions and Functional Programming
Before we delve further into Python’s lambda functions, let’s take a step back and understand the concept of anonymous functions and how they fit into the broader concept of functional programming.
Anonymous Functions: The Unnamed Heroes
An anonymous function, as the name suggests, is a function without a name. Unlike a named function, it’s not defined with the standard def keyword. Instead, anonymous functions are defined using the lambda keyword. Hence, anonymous functions in Python are also called lambda functions.
# A named function
def add(x, y):
return x + y
# An anonymous function
add = lambda x, y: x + y
In the example above, both functions perform the same task, but the lambda function does it without being given a name. This makes them lightweight and ideal for tasks that require a quick, disposable function.
Functional Programming: A Different Approach
Functional programming is a programming paradigm where programs are constructed by applying and composing functions. It emphasizes the application of functions, in contrast to the imperative programming style, which emphasizes changes in state.
Python is not a pure functional programming language, but it incorporates some of its concepts, including lambda functions. In functional programming, lambda functions are often used as they are. They can be assigned to variables, used as arguments to other functions, or returned as values from other functions. All of this contributes to a more efficient and expressive way of writing code.
Understanding these fundamentals provides a solid foundation for mastering Python lambda functions and harnessing their full potential.
Taking Python Lambda Functions Further
Python lambda functions are not just for small, quick tasks—they can also be used in larger scripts or projects. Their simplicity and flexibility can often lead to cleaner, more readable code, especially when used in conjunction with other Python features.
Lambda Functions and Closures
In Python, a closure is a function object that has access to variables from its enclosing lexical scope, even when the function is called outside that scope. Lambda functions can be used to create closures in Python. Here’s an example:
def make_multiplier(n):
return lambda x: x * n
multiply_by_2 = make_multiplier(2)
print(multiply_by_2(4))
# Output:
# 8
In this example, make_multiplier()
returns a lambda function that multiplies its input by n
. Even when we call the returned function outside of make_multiplier()
, it still has access to the value of n
.
Lambda Functions and Decorators
A decorator in Python is a function that takes another function as input and extends the behavior of the latter function without explicitly modifying it. Lambda functions can be used with decorators to create small, one-time decorators. For example, we can create a decorator that logs the result of a function:
def logger(func):
return lambda *args, **kwargs: print(f'Result: {func(*args, **kwargs)}')
@logger
def add(x, y):
return x + y
add(3, 4)
# Output:
# Result: 7
In this example, logger()
is a decorator that takes a function and returns a new function that logs the result of the original function. We use it with a lambda function to create a decorator that can be used anywhere in our code.
If you’re interested in learning more about closures, decorators, or other topics related to Python lambda functions, there are many resources available. Understanding these advanced concepts can help you write more efficient and expressive Python code.
Further Resources for Python Functions
Seeking to bolster your knowledge about Python functions? The following resources have been specifically chosen to accelerate your learning:
- Python Built-In Functions Usage Tips – Explore functions for working with sets and managing data collections.
Type Checking with isinstance() in Python – Dive into checking object types and inheritance relationships in Python.
Transforming Data with Python’s map() Function – Learn how to streamline data processing using the “map” function.
Python’s Official Documentation on Built-In Functions – Detailed explanations and usage of the built-in functions in Python.
Python Control Flow Tools Tutorial – Learn all about controlling flow of your Python programs with the official Python tutorial.
Python Programming Video Tutorial – A video tutorial which walks you through Python programming concepts and application.
With these resources, you will gain a comprehensive understanding of Python functions, thus refining your practices as a Python developer.
Python Lambda Functions: A Recap
Lambda functions, often referred to as anonymous functions in Python, are a powerful tool in your programming arsenal. They are compact, efficient, and can be incredibly powerful when used correctly.
With lambda functions, you can write concise and readable code for simple tasks, and they can also be combined with Python’s built-in functions like map, filter, and reduce for more complex tasks.
However, like any tool, lambda functions should be used appropriately. They are best suited for simple, one-off tasks where a full function definition would be overkill. For complex tasks, regular functions or list comprehensions might be a better choice due to their readability.
By understanding the strengths and weaknesses of lambda functions, and knowing when to use them (or when to use an alternative), you can write more efficient and expressive Python code.