Python isinstance() Function Guide (With Examples)
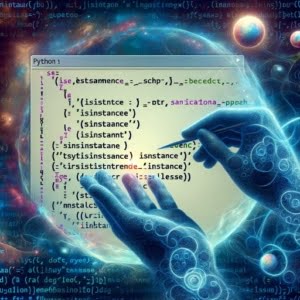
Have you ever been curious about Python’s isinstance()
function? At its core, isinstance()
is a type-checking function, verifying the type of an object in Python and ensuring your code behaves as expected.
But why is type checking crucial, and how does isinstance()
contribute to better Python code? This article will demystify isinstance()
, guiding you through its syntax, usage, and its role in Python’s type system. So, whether you’re a Python novice or an experienced coder looking to deepen your understanding, fasten your seatbelts for an enlightening journey into the world of Python’s isinstance()
function.
TL;DR: What is the isinstance()
function in Python?
The
isinstance()
function in Python is a built-in function used for type checking. It verifies if an object is of a specified type, returning True if it is, and False otherwise.
For example:
x = 10
print(isinstance(x, int)) # Output: True
In this case, isinstance()
returns True because x
is an integer. For more advanced usage, tips, and tricks, continue reading the article.
Table of Contents
Basic Usage of isinstance()
The isinstance()
function is a built-in feature in Python, widely used for type checking. It requires two parameters: the initial is the object under scrutiny, and the second is the type you wish to compare against. Here’s the basic syntax:
isinstance(object, type)
If the object matches the specified type, isinstance()
returns True, otherwise, it’s False.
isinstance()
Usage Examples
To better understand the practical application of isinstance()
, let’s explore some examples.
Assume you have a variable x
assigned the value 10, and you need to verify if x
is an integer. You would use isinstance()
as follows:
x = 10
print(isinstance(x, int)) # Output: True
In this instance, isinstance()
returns True because x
is indeed an integer.
Here are some more examples with different data types:
s = 'Hello, World!'
print(isinstance(s, str)) # Output: True
l = [1, 2, 3]
print(isinstance(l, list)) # Output: True
b = False
print(isinstance(b, bool)) # Output: True
The Importance of Type Checking in Python
Type checking is a vital aspect of Python programming, ensuring that the values you are working with are of the anticipated type, thus preventing potential bugs and errors. isinstance()
plays a significant role in this process by allowing you to confirm an object’s type at runtime.
Although Python is a dynamically typed language (meaning you don’t have to explicitly declare variable types), it’s still crucial to ensure that your variables are of the expected type, especially when performing operations that are type-specific.
Benefits of isinstance()
for Type Checking
Why should isinstance()
be your go-to for type checking instead of other methods? One major advantage is that isinstance()
also considers inheritance.
That means if you have a class that inherits from another, an instance of the subclass will be considered an instance of the parent class as well.
This is incredibly useful in object oriented programming scenarios and is a feature that distinguishes isinstance()
from other type checking methods in Python.
To illustrate, let’s consider a scenario where we have a base class Animal
, a subclass of “Mammal” and another subclass Dog
. If we instantiate Dog
, isinstance()
can confirm that it’s also an instance of Animal
and Mammal
:
class Animal:
pass
class Mammal(Animal):
pass
class Dog(Mammal):
pass
my_dog = Dog()
print(isinstance(my_dog, Dog)) # Output: True
print(isinstance(my_dog, Mammal)) # Output: True
print(isinstance(my_dog, Animal)) # Output: True
In the above example, isinstance()
correctly identifies my_dog
as an instance of both Dog
and Animal
. This is because Dog
is a subclass of Animal
, and therefore, any instance of Dog
is also considered an instance of Animal
.
Considerations and Best Practices of Using isinstance()
While isinstance()
is a powerful tool, it should be used wisely. Over-reliance on isinstance()
for type checking can result in code that’s challenging to read and maintain.
It’s often more beneficial to adhere to Python’s duck typing philosophy—’if it looks like a duck and quacks like a duck, it’s a duck.’
In other words, rather than verifying an object’s type, inspect its capabilities.
Duck Typing versus isinstance()
Duck typing is a programming principle that prioritizes an object’s behavior over its type. In Python, this means that you can use any object that provides the required behavior without checking its type.
While explicit type checking can avert bugs and errors, it can also render the code less flexible. Conversely, duck typing fosters flexibility but can result in runtime errors if an object doesn’t support a specific operation. Striking a balance between safety (type checking) and flexibility (duck typing) is crucial.
This is where the utility of isinstance()
can sometimes be a double-edged sword. While it’s beneficial in certain situations, it can also lead to less Pythonic code if overused.
Other Methods of Type Checking in Python
While isinstance()
is a popular choice for type checking in Python, it’s not the sole method available. Python offers several other ways to perform type checking, each with unique strengths and weaknesses.
Here, we’ll explore type()
and hasattr()
:
Method | Considers Inheritance | Checks Capabilities |
---|---|---|
isinstance() | Yes | No |
type() | No | No |
hasattr() | N/A | Yes |
If inheritance plays a role, isinstance()
would be the preferred choice. If you want to strictly adhere to an object’s type without considering inheritance, type()
would be more appropriate. If you’re more interested in an object’s capabilities or behavior, hasattr()
aligns well with the duck typing philosophy.
Now, let’s go over type() and hasattr() in more depth:
The type() Function
The type()
function checks the type of an object without taking inheritance into account. This function strictly returns the type of an object as it is, without considering its parent classes.
For example:
Code Block:
class Parent:
pass
class Child(Parent):
pass
obj = Child()
print(type(obj) == Parent)
print(type(obj) == Child)
Output:
False
True
In the above code block, we have two classes: Parent
and Child
(which is a subclass of Parent
). We then create an instance obj
from the Child
class.
Using the type()
function to compare the type of obj
with Parent
and Child
, we can see that type(obj) == Parent
returns False
, despite Child
being a subclass of Parent
.
Conversely, type(obj) == Child
returns True
, indicating that the type()
function recognizes obj
as an instance of Child
.
The
type()
function is a strict type checker and does not consider class inheritance. It can be beneficial if you wish to confirm an object’s exact type. However, this characteristic means it doesn’t align with Python’s “code to an interface, not an implementation” philosophy.
The hasattr() Function
On the other hand, the hasattr()
function offers a method of type checking that fits well with the duck typing paradigm.
Instead of directly checking the type of an object, hasattr()
checks whether an object possesses a particular attribute or method.
An example can illustrate this approach:
class Circle:
def __init__(self, radius):
self.radius = radius
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
circle = Circle(5)
rectangle = Rectangle(4, 5)
print(hasattr(circle, 'radius'))
print(hasattr(rectangle, 'radius'))
Output:
True
False
In the code block above, we have two different classes: Circle
and Rectangle
. Each class has its distinct attributes: Circle
has radius
while Rectangle
has width
and height
.
Using the hasattr()
function, we can check whether an instance of these classes possesses a specific attribute. For instance, hasattr(circle, 'radius')
returns True
as the circle
instance of the Circle
class has the radius
attribute.
Conversely, hasattr(rectangle, 'radius')
returns False
, as the rectangle
instance of the Rectangle
class does not have a radius
attribute.
This approach aligns with the concept of duck typing that revolves around an object’s capabilities or behavior over its actual type. It’s particularly useful when the presence of a specific set of attributes (or methods) is more critical than the actual object type in the implementation.
Using Try / Except Instead of Checking Type
“EAFP” (Easier to Ask for Forgiveness than Permission) is a prevalent Python coding style that assumes the existence of valid keys or attributes and catches exceptions if the assumption proves false.
This style is characterized by the use of try/except statements rather than type checking functions like
isinstance()
.
Here’s an example of EAFP in action:
try:
x = 'Hello, World!'
print(x + 10)
except TypeError:
print('Cannot add a string and an integer.')
Evolution of Type Checking in Python
Over the years, Python’s type checking has seen significant evolution. Initially, type checking was primarily accomplished using functions like isinstance()
and type()
.
However, the introduction of type hints in Python 3.5 provided developers a way to explicitly denote the expected type of a variable, function parameter, or return value.
Type hints offer a form of static type checking, allowing Integrated Development Environments (IDEs) and linters to catch potential type errors before runtime. Here’s an example of type hints in Python:
def greet(name: str) -> str:
return 'Hello, ' + name
In this example, name: str
is a type hint indicating the name
parameter should be a string. -> str
is another type hint indicating that the greet
function should return a string.
Python’s Decorators and Metaclasses
Python’s type system extends beyond isinstance()
and type hints. Other language features, such as decorators and metaclasses, also interact with its type system.
Decorators provide a means to modify or enhance functions and classes in Python without altering their source code. They can be utilized for various purposes, including logging, timing function calls, or even type checking.
Here’s an example of a decorator in Python:
def my_decorator(func):
def wrapper():
print('Something is happening before the function is called.')
func()
print('Something is happening after the function is called.')
return wrapper
def say_hello():
print('Hello!')
say_hello = my_decorator(say_hello)
say_hello()
Metaclasses, while more advanced, offer a way to control the creation and behavior of classes in Python. Though their usage is less common, they can be incredibly powerful in specific scenarios.
Further Resources for Python Functions
As you journey further into your exploration of Python functions, we’ve gathered some handpicked resources to enhance your understanding:
- Python Built-In Functions Mastery Simplified – Learn about Python’s built-in functions for input and output formatting.
Handling User Input with Python’s input() Function – Dive into collecting user input and processing responses in Python.
Creating Anonymous Functions with Python Lambda – Dive into concise function definitions and one-liners with “lambda.”
Python’s Built-In Functions Cheat Sheet – Get quick access to Python’s built-in functions with this handy cheat sheet.
JavaTpoint’s Guide to Python Functions – A top-to-bottom overview of Python functions.
Python’s Official Documentation on Built-In Functions gives descriptions of every built-in function in Python.
These resources will provide you with a more in-depth perspective on Python functions, sharpening your skills as a Python developer.
Conclusion:
In this comprehensive guide, we’ve unraveled the intricacies of Python’s isinstance()
function. We’ve explored its role in type checking, offering a simple yet effective tool for verifying an object’s type. isinstance()
stands out for its straightforward syntax and compatibility with Python’s inheritance model.
However, as with any tool, isinstance()
should be used wisely. Over-reliance on it can lead to less readable and less ‘Pythonic’ code. Striking a balance between explicit type checking and the flexibility offered by Python’s dynamic typing and duck typing philosophy is crucial.
Beyond isinstance()
, we’ve journeyed through the broader landscape of type checking in Python. We’ve explored the alternatives of type() and hasattr(), as well as the newer development of type hints for optional static type checking.
As you continue your journey with Python, remember that its philosophy underscores readability and simplicity. Whether you’re using isinstance()
, type hints, or other language features, aim to write code that’s clean, readable, and ‘Pythonic’. Happy coding!