Python Merge Dictionaries | 5 Easy Methods with Examples
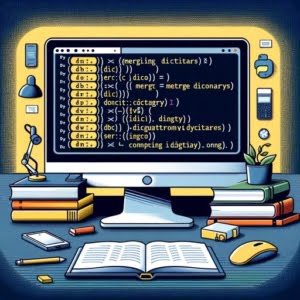
The flexibility of Python dictionaries is unparalleled, serving various purposes from data storage to complex code organization. But what if you need to merge multiple dictionaries? That’s when the magic of merging dictionaries in Python comes to the forefront.
In this article, we will delve into the diverse techniques Python offers to merge dictionaries, their applications, and potential implications.
Whether you’re an experienced Python developer or a novice seeking to broaden your Python skills, mastering the art of efficiently merging dictionaries will significantly enhance your programming toolkit. So, let’s dive deeper into the world of Python dictionaries!
TL;DR: How can I merge dictionaries in Python?
Python provides several methods to merge dictionaries, including the
update()
method, the|
operator, the**
operator, theChainMap
class from the collections module, and thereduce()
function from the functools module. For example, the simplest way is to use theupdate()
method:
# Example
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
dict1.update(dict2)
print(dict1) # Output: {'a': 1, 'b': 3, 'c': 4}
Each of these methods has its own advantages and trade-offs. Continue reading for a comprehensive understanding of these methods, their usage, and implications.
Table of Contents
5 Python Dictionary Merging Techniques
Python presents numerous techniques for merging dictionaries, each with its unique strengths, limitations, and practical applications. Let’s explore these methods and comprehend their functionality through examples.
1: The update() Method
A straightforward approach to merge dictionaries in Python is the update()
method. This method integrates one dictionary into another. If there are shared keys, the values of the second dictionary supersede those in the first.
# Example
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
dict1.update(dict2)
print(dict1) # Output: {'a': 1, 'b': 3, 'c': 4}
2: The Merge (|) Operator
Python 3.9 brought the merge (|) operator into the mix for combining dictionaries. Similar to update()
, it fuses two dictionaries, and if there are shared keys, the second dictionary’s values replace the first’s.
# Example
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
dict3 = dict1 | dict2
print(dict3) # Output: {'a': 1, 'b': 3, 'c': 4}
The merge (|) operator and update() method in Python are similar in that they both merge two dictionaries. However, there are a few differences that may make the merge operator preferable depending on your use case:
- Syntactical Elegance: The merge operator allows for a more concise, cleaner syntax compared to the update method. The merge operator can be used right within an expression, whereas update modifies the dictionary in place.
Chain Merging: The merge operator allows for chaining of multiple dictionary merges, which can simplify code if you’re combining multiple dictionaries in a single line.
Immutability: The merge (|) operation doesn’t affect the original dictionaries. In contrast, update() alters the original dictionary which can be an issue if you need the originals unchanged.
Later Python versions: It’s important to note that the “|” operator for dictionaries is available from Python 3.9 and onwards.
For example:
# Using merge operator
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
dict3 = {'d': 5, 'e': 6}
result = dict1 | dict2 | dict3
# Using update method
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
dict3 = {'d': 5, 'e': 6}
dict1.update(dict2)
dict1.update(dict3)
result = dict1
In these examples, both will output: {'a': 1, 'b': 3, 'c': 4, 'd': 5, 'e': 6}
but the method and impact on original dictionaries are different.
3: The ** Operator
The double star ** operator is another tool for merging dictionaries. This operator unpacks a dictionary’s contents and is frequently used in function calls.
# Example
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
dict3 = {**dict1, **dict2}
print(dict3) # Output: {'a': 1, 'b': 3, 'c': 4}
4: The ChainMap Class from collections Module
ChainMap
is a class from the collections
module providing an alternative for merging dictionaries. Unlike previous methods, ChainMap
does not generate a new dictionary but constructs a list of dictionaries. When a key is accessed, it returns the value from the first dictionary in the list that contains the key.
# Example
from collections import ChainMap
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
dict3 = ChainMap(dict1, dict2)
print(dict3.maps) # Output: [{'a': 1, 'b': 2}, {'b': 3, 'c': 4}]
5: The reduce() Function from functools Module
Another method to merge dictionaries is the reduce()
function from the functools
module. It applies a two-argument function cumulatively to an iterable’s items, from left to right, reducing the iterable to a single output.
# Example
from functools import reduce
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
dict3 = reduce(lambda x, y: {**x, **y}, [dict1, dict2])
print(dict3) # Output: {'a': 1, 'b': 3, 'c': 4}
How To Decide Which Function to Use?
Each method comes with its own benefits and drawbacks, and the choice often hinges on your task’s specific requirements and the Python version you’re using. update()
, ** operator
, and reduce()
function are compatible with all Python versions, while the merge (|)
operator is exclusive to Python 3.9 and later. ChainMap
, on the other hand, provides a unique approach by not creating a new dictionary, making it more memory-efficient in scenarios with large dictionaries.
Method | Python Compatibility | Creates New Dictionary | Chaining | Immutability |
---|---|---|---|---|
update() function | All versions | No | No | Alters original |
Merge (|) | 3.9 and above | Yes | Yes | Doesn’t alter original |
Double Star (**) | All versions | Yes | Yes | Doesn’t alter original |
ChainMap Class | All versions | No (Creates list of dictionaries) | Yes | Doesn’t alter original |
reduce() Function | All versions | Yes | Yes (through iterable) | Doesn’t alter original |
A common hurdle when merging dictionaries in Python is the overriding of values. As we’ve seen earlier, if a key is present in both dictionaries during a merge, the second dictionary’s value replaces the first’s. But what if you need to retain all values of common keys?
Python offers two solution to merge dictionaries while preserving all values of shared keys. This can be accomplished using dictionary comprehension paired with the items()
or the get()
method.
Dictionary merging using the get()
method
Python offers a solution to merge dictionaries while preserving all values of shared keys using the get()
method in dictionary comprehension.
This method retrievs the values corresponding to a given key, defaulting to a specified value (in this example, an empty list) if the key does not exist in the dictionary. In the following Python code snippet, the dictionaries dict1
and dict2
are merged using this approach:
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
merged_dict = {k: [dict1.get(k, []), dict2.get(k, [])] for k in set(dict1) | set(dict2)}
print(merged_dict) # Output: {'b': [2, 3], 'a': [1], 'c': [], 'b': [3]}
Here is a table that clearly shows the input dictionaries and the resulting dictionary after the merge:
Input Dictionaries | Resulting Dictionary |
---|---|
dict1 = {‘a’: 1, ‘b’: 2} | {‘a’: [1], ‘b’: [2, 3], ‘c’: [3]} |
dict2 = {‘b’: 3, ‘c’: 4} |
Notice how the resulting dictionary contains all the keys from both dict1
and dict2
, and the corresponding values are lists containing the values associated with each key in the original dictionaries.
Dictionary merging using the items()
method
If you prefer to directly use the key-value pairs in a dictionary, the items()
method is your friend. This method creates a view object consisting of tuple pairs that represent the dictionary’s keys and their corresponding values.
To merge dictionaries and preserve all the values of shared keys, you can use dictionary comprehension with an if-else condition along with the items()
method:
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
merged_dict = {k: [v] if k not in dict2 else [v, dict2[k]] for k, v in dict1.items()}
merged_dict.update({k: [v] for k, v in dict2.items() if k not in dict1})
print(merged_dict) # Output: {'a': [1], 'b': [2, 3], 'c': [4]}
Here is a table that clearly shows the input dictionaries and the resulting dictionary after the merge:
Input Dictionaries | Resulting Dictionary |
---|---|
dict1 = {‘a’: 1, ‘b’: 2} | {‘a’: [1], ‘b’: [2, 3], ‘c’: [4]} |
dict2 = {‘b’: 3, ‘c’: 4} |
In this code snippet, the resulting merged_dict
conserves all keys from dict1
and dict2
. The corresponding values are lists that contain the values from the original dictionaries. Keys that are unique to one dictionary have a one-item list as the value.
Note: These methods do have some drawbacks. They can lead to heightened memory usage, particularly when dealing with large dictionaries, as they form a new dictionary and stores multiple values for shared keys. This could potentially affect your program’s performance.
The Importance of Merging Dictionaries in Python
Having established a solid understanding of Python dictionaries merging techniques, it’s time to explore scenarios where merging dictionaries becomes a necessity.
Scenarios Necessitating Dictionary Merging
Here is an example of merging user data from different sources:
# User data from different sources
data1 = {'name': 'John', 'age': 30, 'city': 'New York'}
data2 = {'name': 'Jane', 'age': 28, 'city': 'Los Angeles'}
# Merging the dictionaries
database = {**data1, **data2}
print(database) # Output: {'name': 'Jane', 'age': 28, 'city': 'Los Angeles'}
Dictionary merging is a frequent need in Python programming. For example, you might have data dispersed across multiple dictionaries that you need to amalgamate for efficient data manipulation.
Or, you might be interacting with APIs that return data as dictionaries, necessitating their merger for further processing.
The Idea of a Unified Database
Imagine a situation where you are constructing a unified database from multiple sources, each supplying data as a dictionary. The keys serve as data fields, and the values as data entries.
Merging these dictionaries can help you create a comprehensive database, simplifying data manipulation and analysis.
# Example
user_data1 = {'name': 'John', 'age': 30, 'city': 'New York'}
user_data2 = {'name': 'Jane', 'age': 28, 'city': 'Los Angeles'}
database = [user_data1, user_data2]
In this example, user_data1
and user_data2
could represent data from distinct sources. By merging these dictionaries into a list (a basic form of merging), we form a common users database.
Python Dictionaries Explained
To better understand merging dictionaries, it’s can be helpful to understand what a dictionary in Python entails.
A dictionary in Python is an integral data type used to store data in key-value pairs, where each pair is separated by a colon :
, and the pairs are contained within curly braces {}
.
# Python dictionary example
my_dict = {'name': 'John', 'age': 30, 'city': 'New York'}
print(my_dict) # Output: {'name': 'John', 'age': 30, 'city': 'New York'}
In this example, ‘name’, ‘age’, and ‘city’ are keys, while ‘John’, 30, and ‘New York’ are their corresponding values.
Mutable and Immutable Values
It’s vital to note that dictionaries have immutable keys. This implies that once a key is assigned, it cannot be altered. However, the values associated with the keys are mutable and can be modified.
See our article for an explanation of Mutable vs Immutable objects in Python.
Example Dictionary
Here is an example of a more complex dictionary, which includes another dictionary as a value:
# Python nested dictionary example
my_dict = {'name': 'John', 'age': 30, 'address': {'street': '123 Main St', 'city': 'New York', 'zip': '10001'}}
print(my_dict) # Output: {'name': 'John', 'age': 30, 'address': {'street': '123 Main St', 'city': 'New York', 'zip': '10001'}}
Dictionaries are fundamental to Python programming, particularly in data manipulation. They offer a flexible way to access and organize data. For instance, if you’re dealing with a vast amount of data, you can utilize a dictionary to construct a database, using keys as data identifiers and values as data elements.
Furthermore, Python dictionaries exhibit exceptional flexibility in managing complex data structures. They can accommodate multiple data types, including objects, and even other dictionaries, enabling the creation of nested data structures. This versatility makes dictionaries an indispensable tool for Python programmers.
Further Resources for Python Dictionaries
- Enhance Your Python Skills with Dictionary Manipulation by mastering dictionary syntax and exploring advanced dictionary features.
You may also be interested in these other online resources:
- Iterating Over Python Dictionaries – A Comprehensive Tutorial – Learn how to iterate through Python dictionaries and access their elements.
Python Ordered Dictionary – Maintaining Order in Dictionaries – Explore Python’s OrderedDict and understand its role in maintaining order.
Python Sets and Dictionaries – This tutorial discusses both Python sets and dictionaries. It covers their definitions, usage, and methods to perform various operations.
Python Programming/Dictionaries – An in-depth Wikibooks entry on Python dictionaries. It offers a comprehensive understanding of the data structure.
Python Dictionaries Cheat Sheet – This gist provides a quick reference or cheat sheet for Python dictionaries covering all the commonly used operations and methods.
Final Thoughts
Throughout this article, we’ve delved into various techniques for merging dictionaries, each with its distinct advantages and potential implications.
From the straightforward update()
method to the memory-efficient ChainMap
from the collections module, Python provides an array of options for dictionary merging. We’ve also dissected the challenges associated with merging dictionaries, such as value overriding and potential spikes in memory usage.
Moreover, we’ve explored how to retain all values of shared keys during a merge, a technique that can be particularly beneficial when you need to conserve all data from your dictionaries.
Whether you’re constructing a unified database from multiple sources, interacting with APIs, or simply manipulating data, proficiently merging dictionaries can significantly optimize your code and smooth your programming journey.
For more on Python’s diverse capabilities, check out our cheat sheet here.
So, the next time you’re tasked with merging dictionaries in Python, recall the methods discussed in this article, and select the one that best suits your situation. Happy coding!