Iterate a Dictionary in Python: Guide (With Examples)
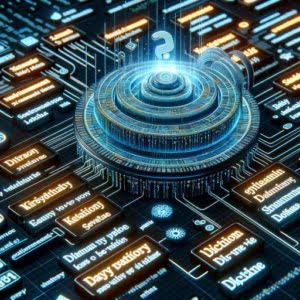
Today, we’re set to decode the mystery of Python dictionaries and the art of iterating through them. If you’ve been puzzled about how to loop through a dictionary or wish to broaden your Python knowledge, you’re at the right spot.
Python dictionaries, a type of data structure, store data as key-value pairs. Their versatility is unmatched, finding use in diverse applications, from data analysis to web development. One of the most vital skills while working with dictionaries is the ability to iterate through them. Iteration or looping lets us access and manipulate each item in a dictionary, one at a time.
By the end of this post, you’ll be equipped with the skills to iterate through Python dictionaries effectively. From the basics to advanced techniques, we’ve got it all covered. So whether you’re a beginner or an experienced programmer seeking a refresher, there’s something for everyone. Let’s get started!
TL;DR: How do I iterate through a Python dictionary?
Iterating through a Python dictionary involves looping over its keys and values. The basic method is to use a for loop. For example,
for key in dictionary: print(key, dictionary[key])
. This will print each key and its corresponding value. However, Python provides more advanced methods, such asitems()
,keys()
, andvalues()
, which offer more control over the iteration process. Read on for more advanced methods, background, tips, and tricks.
grades = {'John': 85, 'Emily': 92, 'Lucas': 78}
for key in grades:
print(key, grades[key])
This code block demonstrates the basic method of iterating through a Python dictionary. It will print each key (student’s name) and its corresponding value (grade).
Table of Contents
The ABCs of Iteration
When it comes to Python dictionaries, iteration revolves around looping through the dictionary’s keys and values. But what exactly are these keys and values?
In a Python dictionary, data is stored as key-value pairs. The key is the identifier that points to a value. For instance, in a dictionary of student grades, the keys could be the students’ names, and the values could be their grades. Here’s an example:
grades = {'John': 85, 'Emily': 92, 'Lucas': 78}
In this dictionary, ‘John’, ‘Emily’, and ‘Lucas’ are the keys, and 85, 92, and 78 are the corresponding values.
Example of creating a dictionary and accessing its keys and values:
grades = {'John': 85, 'Emily': 92, 'Lucas': 78}
print('Keys:', list(grades.keys()))
print('Values:', list(grades.values()))
So, how do we iterate through this dictionary? The most basic method is to use a for loop to iterate over the keys, like so:
for key in grades:
print(key)
This will output:
John
Emily
Lucas
But what if we want to access the values as well? We can achieve this by referencing the key within the dictionary, like so:
for key in grades:
print(key, grades[key])
This will output:
John 85
Emily 92
Lucas 78
As you can see, the strength of iteration lies in its ability to handle and manipulate dictionary data efficiently. By understanding and mastering iteration, you can unlock the full potential of Python dictionaries.
Using len() to iterate the right number of times
It’s also a good idea to leverage Python’s built-in functions whenever possible. For example, the len()
function can be used to find the number of items in a dictionary:
print(len(grades))
This will output 3
, since our grades
dictionary has three items.
Here’s an example where we use a loop to iterate over a dictionary based on its length.
Let’s say we have the following dictionary:
grades = {
"John": 85,
"Emily": 90,
"Lucas": 78
}
You can use len()
inside a for
loop to iterate over the dictionary like so:
# Get the keys of the dictionary
keys = list(grades.keys())
for i in range(len(grades)):
print("Student:", keys[i], "- Grade:", grades[keys[i]])
The output will be:
Student: John - Grade: 85
Student: Emily - Grade: 90
Student: Lucas - Grade: 78
In this example, len(grades)
gives the number of items in the dictionary which is used as the stopping condition in the range()
function. Then i
is used as the index to access each key in the keys list.
Advanced Dictionary Iteration: Taking It Up a Notch
Having grasped the basics, we’re now ready to delve into some more sophisticated methods for dictionary iteration in Python. These techniques offer more flexibility and can make your code more efficient and easier to read.
Next, we’ll go over items(), keys(), and values(), three more advanced methods to help you iterate through dictionaries. Here’s a summary of the methods:
Method | Description | Example |
---|---|---|
items() | Returns a list of tuple pairs representing the key-value pairs in the dictionary | grades.items() |
keys() | Returns a list of all the keys in the dictionary | grades.keys() |
values() | Returns a list of all the values in the dictionary | grades.values() |
items()
One such method is the use of the items()
function. This function returns a list of tuple pairs that represent the key-value pairs in the dictionary. Here’s how you can implement it in a for loop:
for key, value in grades.items():
print(key, value)
The output from this will be identical to our previous example:
John 85
Emily 92
Lucas 78
As evident, the items()
function enables us to access the keys and values directly within the for loop, rendering our code cleaner and more readable.
keys() and values()
But what if you’re interested in accessing only the keys or the values? This is where the keys()
and values()
functions come into play. The keys()
function returns a list of all the keys in the dictionary, while the values()
function provides a list of all the values. Here’s how you can implement them:
for key in grades.keys():
print(key)
for value in grades.values():
print(value)
The first loop will output the keys:
John
Emily
Lucas
And the second loop will output the values:
85
92
78
These advanced methods offer more control over your iteration process and can be incredibly useful in various scenarios. For instance, you might want to iterate over the keys if you’re searching for a specific key, or over the values if you’re conducting an operation on each value.
Iteration with Dictionary Comprehension
Finally, let’s discuss an alternative way to iterate through dictionaries. One such method is dictionary comprehension, which is a concise way to create new dictionaries. Here’s an example that creates a new dictionary with only the students who passed (grade of 80 or higher):
passed = {key: value for key, value in grades.items() if value >= 80}
print(passed)
This will output:
{'John': 85, 'Emily': 92}
As you can see, dictionary comprehension can be a powerful tool for manipulating dictionary data.
Troubleshooting and Error Handling
When working with complex data structures like lists and dictionaries in Python, you’ll often encounter a variety of errors and challenges.
It’s vital to understand how to handle these situations in order to maintain clean, efficient, and bug-free code. This section provides practical tips and best practices on how to effectively handle common issues, such as non-existent keys in dictionaries.
get() method
One common mistake when iterating over dictionaries is trying to access a key that doesn’t exist. This will raise a KeyError
. To prevent this, you can use the get()
method, which returns None
if the key is not found, instead of raising an error:
print(grades.get('Alex'))
This will output None
, since ‘Alex’ is not a key in our grades
dictionary.
Dictionaries are mutable!
Another common pitfall is modifying the dictionary while iterating over it. This can lead to unpredictable results and should generally be avoided. If you need to modify the dictionary, consider creating a copy or a new dictionary instead.
Here’s a code block that illustrates the point:
# Original dictionary
grades = {
"John": 85,
"Emily": 90,
"Lucas": 78
}
try:
# Attempt to add a new item while iterating
for student in grades:
if student == "Emily":
grades["Alex"] = 88 # trying to modify dictionary while iterating over it
except RuntimeError as e:
print(f"Error: {e}")
This will output:
Error: dictionary changed size during iteration
This error occurs because the code is trying to modify the dictionary while it’s being iterated over.
To correctly modify the dictionary while iterating over it, you could iterate over a copy of the dictionary:
# Original dictionary
grades = {
"John": 85,
"Emily": 90,
"Lucas": 78
}
# Create a copy of the dictionary for iterating
grades_copy = grades.copy()
for student in grades_copy:
if student == "Emily":
grades["Alex"] = 88
# Now print the modified dictionary
print(grades)
Output:
{'John': 85, 'Emily': 90, 'Lucas': 78, 'Alex': 88}
In this case, we’re iterating over a copy of the ‘grades’ dictionary, which allows us to add a new item to the original ‘grades’ dictionary without errors.
A Closer Look at Python Dictionaries
Now that we’ve covered iterating through dictionaries, it may be helpful to refresh on Python dictionaries more generally.
Python dictionaries are a type of data structure that store data in key-value pairs. Think of them as a real-life phone book where the name is the key, and the phone number is the value.
In Python, keys can be of any immutable type, such as integers or strings, and values can be of any type.
Here’s an example of a Python dictionary:
student = {
'name': 'John',
'age': 21,
'courses': ['Math', 'CompSci']
}
In this dictionary, ‘name’, ‘age’, and ‘courses’ are the keys, and ‘John’, 21, and [‘Math’, ‘CompSci’] are the corresponding values.
Creating and manipulating dictionaries in Python is straightforward. To add a new key-value pair to a dictionary, you can simply assign a value to a new key, like so:
student['grade'] = 'A'
This will add the key ‘grade’ with the value ‘A’ to the student
dictionary.
Dictionaries can store a wide range of data types, including other dictionaries, and they provide a variety of methods for accessing and manipulating their data. For example, the pop()
method can be used to remove a key-value pair from a dictionary:
age = student.pop('age')
This will remove the ‘age’ key and its corresponding value from the student
dictionary and return the value.
Example of adding a new key-value pair and removing a key-value pair:
student = {'name': 'John', 'age': 21, 'courses': ['Math', 'CompSci']}
student['grade'] = 'A'
print(student)
age = student.pop('age')
print(student)
One of the key advantages of dictionaries is their efficiency. Accessing and manipulating data in a dictionary is typically fast, regardless of the size of the dictionary. This is because dictionaries use a technique called hashing, which allows them to directly access any value based on its key.
Further Resources For Dictionaries
To expand your dictionary skillset, consider reading these online resources:
- The Ultimate Python Dictionary Syntax Tutorial – This IOFlood guide will help you increase the efficiency of Python dictionaries and their impact on your coding projects.
Python Dictionary Get Method – Retrieving Values with Ease – Explore the Python dictionary get() method and its practical applications.
Python Dictionary Merging Techniques – Unifying Data – Dive into Python dictionary merging and understand how to combine data.
Tutorial on Adding to Python Dictionaries – This tutorial guides you through multiple ways to add key-value pairs to a Python dictionary.
Understanding and Accessing Keys and Values in Python Dictionaries – In this thread, the Stack Overflow community discusses various methods for accessing keys and values in Python dictionaries.
Nested Dictionaries in Python – This tutorial elaborates on how to create and work with nested dictionaries in Python.
Wrapping Up:
As we reach the end of our exploration into Python dictionaries and iteration, let’s take a moment to recap what we’ve learned.
We started with the basics of Python dictionaries and how to iterate through them using a simple for loop. We then delved into more advanced techniques, like the items()
, keys()
, and values()
methods, which offer more control and efficiency in our iteration process. We also tackled common challenges and shared tips for efficient dictionary iteration, helping you navigate potential pitfalls and enhance your Python programming skills.
Whether you’re a beginner just starting your Python journey or an experienced programmer looking for a refresher, we hope this guide has been helpful. Remember, the key to mastering Python—or any programming language—is practice. So keep coding, keep exploring, and most importantly, have fun along the way! Happy coding!