Python Dictionary Get Method: A Complete Walkthrough
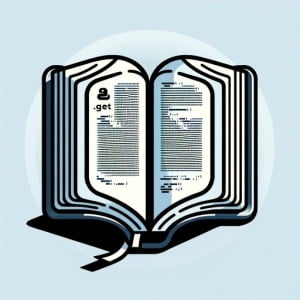
Struggling to retrieve values from a Python dictionary? Just like a librarian finding a book, Python’s dictionary ‘get’ method can help you access the information you need.
This guide will walk you through the use of Python’s dictionary ‘get’ method, from basic usage to advanced techniques. Whether you’re a beginner just starting out with Python or an experienced developer looking to refine your skills, this guide has something for everyone.
So, let’s dive in and start mastering the Python dictionary ‘get’ method.
TL;DR: How Do I Use the ‘get’ Method in a Python Dictionary?
The ‘get’ method allows you to retrieve the value of a specified key in a Python dictionary. Here’s a simple example:
dict = {'name': 'John', 'age': 30}
print(dict.get('name'))
# Output:
# 'John'
In this example, we have a dictionary named ‘dict’ with two key-value pairs. We use the ‘get’ method to retrieve the value associated with the key ‘name’, which in this case is ‘John’. Stay tuned for more detailed explanations and advanced usage scenarios.
Table of Contents
- Python Dictionary Get: Basic Usage
- Python Dictionary Get: Advanced Usage
- Alternative Methods: Beyond Python Dictionary Get
- Troubleshooting Python Dictionary Get Method
- Understanding Python Dictionaries
- Python Dictionary Methods
- Python Dictionary Get: A Key Tool in Your Python Toolkit
- Further Resources for Python Dictionaries
- Wrapping Up: Python Dictionary Get Method
Python Dictionary Get: Basic Usage
The ‘get’ method in Python dictionaries is pretty straightforward. It allows you to retrieve the value of a specified key. Here’s a simple code example:
dict = {'name': 'John', 'age': 30}
print(dict.get('name'))
# Output:
# 'John'
In this example, we have a dictionary named ‘dict’ with two key-value pairs. The ‘get’ method retrieves the value associated with the key ‘name’, which in this case is ‘John’. If the key doesn’t exist in the dictionary, the ‘get’ method returns ‘None’.
The ‘get’ method is an efficient way to retrieve values from a dictionary, especially when you’re not sure if a key exists. It’s a safer alternative to accessing the value directly using the key, which can raise a KeyError if the key doesn’t exist.
One potential pitfall to be aware of, though, is that the ‘get’ method will return ‘None’ if the key doesn’t exist, which might not always be the desired behavior. You may want to check if a key exists before trying to retrieve its value.
Python Dictionary Get: Advanced Usage
As you become more comfortable with Python dictionaries, you’ll find that the ‘get’ method is even more powerful than it first appears. One of its key features is the ability to provide a default value when the key is not found in the dictionary.
Consider the following example:
dict = {'name': 'John', 'age': 30}
print(dict.get('address', 'Not Found'))
# Output:
# 'Not Found'
In this case, we’re trying to retrieve the value of the key ‘address’. But ‘address’ doesn’t exist in our dictionary. Instead of returning ‘None’ (which is the default behavior), the ‘get’ method returns the string ‘Not Found’. This is because we provided ‘Not Found’ as a default value.
This feature is particularly useful when you’re working with large dictionaries and you want to avoid KeyErrors. It allows you to handle missing keys gracefully, without interrupting the flow of your program.
Alternative Methods: Beyond Python Dictionary Get
While the ‘get’ method is a powerful tool for retrieving values from a Python dictionary, it’s not the only option. Python offers other ways to access dictionary values, such as the ‘[]’ operator.
Consider the following example:
dict = {'name': 'John', 'age': 30}
print(dict['name'])
# Output:
# 'John'
In this code block, we’re using the ‘[]’ operator to access the value associated with the key ‘name’. This approach is straightforward and commonly used, but it has a significant drawback: if the key doesn’t exist in the dictionary, Python raises a KeyError.
On the other hand, the ‘get’ method returns ‘None’ or a default value when the key is not found, thus avoiding a KeyError. This makes the ‘get’ method a safer option for accessing dictionary values, especially when you’re unsure if a key exists.
In conclusion, while the ‘[]’ operator is a viable method for retrieving values from a Python dictionary, the ‘get’ method offers more flexibility and error handling. The choice between them depends on your specific needs and the context of your code.
Troubleshooting Python Dictionary Get Method
While the ‘get’ method is incredibly useful, it’s not without its challenges. One common issue you might encounter is dealing with non-existing keys.
Consider the following example:
dict = {'name': 'John', 'age': 30}
print(dict['address'])
# Output:
# KeyError: 'address'
In this case, we’re trying to access the value of the key ‘address’ directly. But ‘address’ doesn’t exist in our dictionary, so Python raises a KeyError.
To avoid this, you can use the ‘get’ method, which returns ‘None’ or a default value when the key is not found. Here’s how you can do it:
dict = {'name': 'John', 'age': 30}
print(dict.get('address', 'Not Found'))
# Output:
# 'Not Found'
In this example, we’re using the ‘get’ method to retrieve the value of ‘address’. Since ‘address’ doesn’t exist in our dictionary, the ‘get’ method returns the string ‘Not Found’.
This feature of the ‘get’ method allows you to handle missing keys gracefully, preventing your program from crashing due to a KeyError. It’s a good practice to always consider the possibility of missing keys when working with dictionaries in Python.
Understanding Python Dictionaries
Before we delve deeper into the ‘get’ method, let’s take a step back and understand Python dictionaries and their basic components: keys and values.
A Python dictionary is a built-in data structure that stores data in key-value pairs. Each key-value pair maps the key to its associated value.
For example:
dict = {'name': 'John', 'age': 30}
print(dict)
# Output:
# {'name': 'John', 'age': 30}
In this dictionary, ‘name’ and ‘age’ are keys, and ‘John’ and 30 are their corresponding values.
Python Dictionary Methods
Python dictionaries come with a set of built-in methods that allow you to manipulate and access the data stored in them. The ‘get’ method is one of these built-in methods. It retrieves the value of a specified key from the dictionary.
Understanding the basic structure of Python dictionaries and the functionality of their methods is crucial for effectively using the ‘get’ method. In the next sections, we’ll explore more complex and advanced uses of the Python dictionary ‘get’ method.
Python Dictionary Get: A Key Tool in Your Python Toolkit
The ‘get’ method is not just a fundamental part of Python dictionaries – it’s a valuable tool for larger Python projects. Whether you’re working in data analysis, web development, or any other field that involves handling large amounts of data, the ‘get’ method can make your life significantly easier.
Consider a data analysis project where you’re dealing with large datasets. You might store this data in dictionaries for easy access and manipulation. In such a scenario, the ‘get’ method would allow you to retrieve specific data points efficiently, even providing a default value for missing data.
In web development, you might use dictionaries to store user information or session data. Again, the ‘get’ method proves invaluable in accessing this data safely and efficiently.
user_data = {'name': 'John', 'age': 30, 'location': 'New York'}
print(user_data.get('occupation', 'Not provided'))
# Output:
# 'Not provided'
In this example, we’re trying to retrieve the user’s occupation. But since this information is not available in our dictionary, the ‘get’ method returns ‘Not provided’.
As you continue to explore Python, you’ll find more advanced concepts related to dictionaries, such as dictionary comprehension and iteration over dictionaries. These concepts, combined with the ‘get’ method, can greatly enhance your Python programming prowess.
Further Resources for Python Dictionaries
For a deeper understanding of Python dictionaries and their methods, consider checking out the following resources:
- IOFlood’s tutorial on Python Dictionaries can help you master this essential data structure. Gain a strong foundation in Python dictionaries and their essential role in modern programming.
Python Dictionary to JSON Conversion: Exporting Data – Dive into Python dictionary serialization to JSON and understand its utility.
Exploring Dictionary Iteration in Python – Master the art of looping through dictionaries in Python.
Python Documentation on Dictionaries – The official Python documentation provides a complete and in-depth explanation of dictionaries in Python.
This Dictionary Tutorial by W3Schools provides a beginner-friendly introduction to Python dictionaries including creation, adding items, modifying items, and dictionary methods.
Real Python’s Guide to Python Dictionaries delves deep into the usage and functionalities of dictionaries in Python including various examples and techniques.
Wrapping Up: Python Dictionary Get Method
In this guide, we’ve explored the Python dictionary ‘get’ method in depth. We’ve seen how it’s used to retrieve values from a dictionary, and how it gracefully handles non-existing keys by returning 'None'
or a default value. This method is a safer alternative to the '[]'
operator, which raises a KeyError when a key doesn’t exist.
dict = {'name': 'John', 'age': 30}
print(dict.get('address', 'Not Found'))
# Output:
# 'Not Found'
We also discussed other ways to retrieve values from a Python dictionary, like the '[]'
operator. While this method is straightforward, it lacks the error handling of the ‘get’ method.
dict = {'name': 'John', 'age': 30}
print(dict['address'])
# Output:
# KeyError: 'address'
In conclusion, the Python dictionary ‘get’ method is a powerful tool for handling dictionaries. It’s a key part of Python programming and a fundamental concept in data manipulation and analysis. As you continue to learn Python, the ‘get’ method and other dictionary methods will become invaluable tools in your programming toolkit.