Learn Python Write to File | Guide (With Examples)
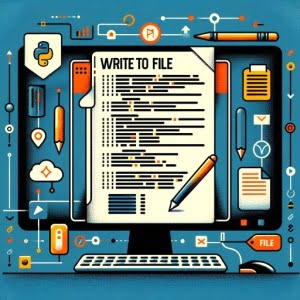
Ever felt like you’re wrestling with your code just to write data to a file in Python? You’re not alone. But the good news is, Python is like a diligent secretary, capable of jotting down data into any file format with ease.
Whether you’re a beginner just dipping your toes into Python’s file handling capabilities, or an intermediate user looking to refine your skills, this guide is designed for you. We will walk you through the process of writing to a file in Python, starting from the simplest techniques to more advanced methods. So, buckle up and let’s dive into the world of Python file handling!
TL;DR: How Do I Write to a File in Python?
The answer lies in Python’s built-in
open()
function combined with ‘w’ mode. Here’s a quick example to illustrate:
with open('myfile.txt', 'w') as f:
f.write('Hello, World!')
# Output:
# This will create a file named 'myfile.txt' in your current directory and write 'Hello, World!' into it.
In this example, we’re using Python’s open()
function to create a file named ‘myfile.txt’. We use ‘w’ mode, which stands for ‘write’. The write()
method then allows us to insert the string ‘Hello, World!’ into our file. It’s that simple!
Intrigued? Keep reading for a deeper dive into writing files in Python, including more detailed explanations and advanced usage scenarios.
Table of Contents
- Python’s Open Function: Basic File Writing
- Writing Various Data Types with Python
- Exploring Alternative File Writing Methods in Python
- Navigating Common File Writing Issues in Python
- Understanding Python’s File Handling Capabilities
- File Writing: A Gateway to Data Persistence and Logging
- Recap: Writing to Files in Python Made Easy
Python’s Open Function: Basic File Writing
Python’s open()
function is the key to writing data to a file. This function is used to open a file in Python, and it takes two parameters: the name of the file you want to open and the mode in which you want to open it.
In the context of writing to a file, the most commonly used mode is ‘w’, which stands for ‘write’. Let’s take a look at a simple example:
with open('example.txt', 'w') as file:
file.write('Python makes file writing easy!')
# Output:
# This will create a file named 'example.txt' and write 'Python makes file writing easy!' into it.
In this code block, we’re using the open()
function with ‘w’ mode to create a file named ‘example.txt’. The write()
method is then used to insert the string ‘Python makes file writing easy!’ into our file.
It’s important to note that if ‘example.txt’ already exists, the ‘w’ mode will overwrite the file without any warning. This could be a potential pitfall if you have important data in the file. However, if the file does not exist, Python will create it for you, making the ‘w’ mode very convenient for writing to files.
Advantages and Potential Pitfalls
The open()
function with ‘w’ mode is simple and straightforward, making it great for beginners. It’s a powerful tool for writing data to files, but it’s important to use it with caution to avoid accidentally overwriting important files.
Writing Various Data Types with Python
Python does not limit you to writing just strings to a file. You can also write other data types such as lists or dictionaries. Let’s explore how you can do this.
Writing Lists to a File
Suppose you have a list of strings that you want to write to a file, each on a new line. Here’s how you can do it:
my_list = ['Python', 'makes', 'file', 'writing', 'easy!']
with open('list_example.txt', 'w') as file:
for item in my_list:
file.write('%s
' % item)
# Output:
# This will create a file named 'list_example.txt' and write each item of the list on a new line.
In this example, we’re iterating over each item in the list and writing it to the file with a newline () character at the end. This ensures that each item is written on a new line.
Writing Dictionaries to a File
Writing a dictionary to a file is slightly more complicated because a dictionary is a collection of key-value pairs. Here’s how you can write a dictionary to a file:
my_dict = {'Python': 'A high-level programming language', 'File Writing': 'The process of saving data to a file'}
with open('dict_example.txt', 'w') as file:
for key, value in my_dict.items():
file.write('%s:%s
' % (key, value))
# Output:
# This will create a file named 'dict_example.txt' and write each key-value pair on a new line, separated by a colon.
In this case, we’re iterating over each key-value pair in the dictionary and writing it to the file. We’re using a colon to separate each key from its value, and a newline character () to ensure that each pair is written on a new line.
By understanding how to write different types of data to a file, you can leverage Python’s flexibility and power to handle a wide range of data persistence tasks.
Exploring Alternative File Writing Methods in Python
Although Python’s open()
function is a powerful tool for writing to files, it’s not the only tool in Python’s arsenal. Let’s explore some alternative methods for writing to a file in Python, such as using the print()
function with file redirection, and the pickle
module for serializing objects.
File Writing with Print Function
The print()
function isn’t just for printing output to the console. You can also use it to write to a file. Here’s how:
with open('print_example.txt', 'w') as file:
print('Python makes file writing easy!', file=file)
# Output:
# This will create a file named 'print_example.txt' and write 'Python makes file writing easy!' into it.
In this example, we’re using the print()
function’s file
parameter to redirect the output to our file. This can be a more intuitive way to write to a file, especially if you’re already familiar with the print()
function.
File Writing with Pickle Module
The pickle
module is another powerful tool for writing to files in Python. It allows you to serialize and deserialize Python objects, which means you can write even complex data structures like classes, functions, and more to a file. Here’s a simple example:
import pickle
my_dict = {'Python': 'A high-level programming language', 'File Writing': 'The process of saving data to a file'}
with open('pickle_example.p', 'wb') as file:
pickle.dump(my_dict, file)
# Output:
# This will create a file named 'pickle_example.p' and write the serialized dictionary into it.
In this case, we’re using the pickle.dump()
function to write our dictionary to the file. The ‘wb’ mode stands for ‘write binary’, which is necessary because pickle serializes objects to binary format.
Choosing the Right Approach
Each of these methods has its own benefits and drawbacks. The open()
function with ‘w’ mode is simple and straightforward, but it can overwrite files without warning. The print()
function is intuitive and familiar, but it may not be as flexible as the open()
function. The pickle
module is powerful and flexible, but it writes in binary format, which may not be suitable for all use cases.
Choosing the right method depends on your specific needs. If you’re writing simple data and want a quick and easy solution, the open()
function or print()
function may be the best choice. If you’re dealing with complex data structures, the pickle
module could be the way to go.
While Python makes writing to a file relatively straightforward, you may still encounter some common issues. Let’s explore these problems and how to address them.
Dealing with ‘FileNotFoundError’
A common issue you may encounter when trying to write to a file in Python is the ‘FileNotFoundError’. This error occurs when Python can’t locate the file you’re trying to write to. Here’s an example:
try:
with open('non_existent_file.txt', 'r') as file:
file.write('Hello, World!')
except FileNotFoundError:
print('File not found.')
# Output:
# File not found.
In this example, we’re trying to open a file that doesn’t exist, which raises a ‘FileNotFoundError’. We handle this error using a try-except block and print a friendly message instead of letting the program crash.
Addressing File Permission Issues
Another common problem is encountering a ‘PermissionError’ when trying to write to a file. This error is raised when you don’t have the necessary permissions to write to the file. Here’s how to handle it:
try:
with open('protected_file.txt', 'w') as file:
file.write('Hello, World!')
except PermissionError:
print('You do not have permission to write to this file.')
# Output:
# You do not have permission to write to this file.
In this case, we’re trying to write to a file that we don’t have write permissions for. Again, we use a try-except block to catch the ‘PermissionError’ and print a helpful message.
By understanding and preparing for these common issues, you can write more robust Python code and handle errors gracefully.
Understanding Python’s File Handling Capabilities
To fully grasp how Python writes to a file, it’s essential to understand the fundamental concepts of file handling in Python. Let’s delve into the idea of file objects and the difference between text mode and binary mode.
The Concept of File Objects
When you open a file in Python using the open()
function, Python creates a file object. This file object serves as a link between your Python program and the file on your disk. Here’s a simple example:
file = open('example.txt', 'w')
# Output:
# This creates a file object for 'example.txt' in write mode.
In this code block, file
is our file object. We can call various methods on this file object to perform operations like writing to the file or closing the file.
Text Mode vs Binary Mode
When opening a file in Python, you can choose to open it in text mode or binary mode. Text mode is the default mode and is used for files that contain text data. Binary mode, on the other hand, is used for files that contain non-text data, like images or executable files.
Here’s how you can open a file in binary mode:
file = open('example.bin', 'wb')
# Output:
# This creates a file object for 'example.bin' in binary write mode.
In this example, we’re opening ‘example.bin’ in binary write mode, as indicated by the ‘wb’ mode.
Understanding these fundamental concepts is crucial to mastering file writing in Python. With this foundation, you can better appreciate how Python’s open()
function, write()
method, and other file writing techniques work under the hood.
File Writing: A Gateway to Data Persistence and Logging
Understanding how to write to a file in Python opens up a world of possibilities for data persistence and logging. Writing data to a file allows you to store information beyond the life of the program, making it a crucial aspect of data persistence. In the realm of logging, writing to a file enables you to keep a record of events, which is invaluable for debugging and monitoring.
log_data = 'This is a log message.'
with open('log.txt', 'a') as file:
file.write(log_data + '
')
# Output:
# This will append the log message to 'log.txt'. If 'log.txt' doesn't exist, it will be created.
In this example, we’re appending a log message to a file named ‘log.txt’ using the ‘a’ mode, which stands for ‘append’. This is a fundamental technique in logging, as it allows you to add new log messages to the file without overwriting the existing ones.
Expanding Your File Handling Skills
Once you’ve mastered writing to a file, you can explore related concepts like reading from a file, appending to a file, and handling file errors. These skills will further enhance your ability to work with files in Python and solve a wider range of problems.
Further Resources for Python File I/O Operations
There are plenty of resources available to deepen your understanding of these topics. For example, consider the following:
- Python OS Module Unveiled – Learn about working with file streams and reading/writing files using “os.”
Iterating through Lines in Python Files – Discover how to process large files with ease using line-by-line reading.
Python Pathlib Module – Discover the power of Python’s “pathlib” module for modern path manipulation.
Python File Handling Tutorial: Youtube Video – This video guide gives an explanation of handling files in Python.
How to read a text file in Python – This article provides a step-by-step approach to reading text files in Python.
Writing to file in Python – This article provides an understanding of how to write to files in Python.
Remember, the journey of mastering Python file handling is a marathon, not a sprint. Keep learning, keep exploring, and most importantly, keep coding!
Recap: Writing to Files in Python Made Easy
Throughout this guide, we’ve explored how Python simplifies the process of writing to a file. We started with the basic usage of Python’s built-in open()
function and the ‘w’ mode, which allows you to write strings to a file with ease.
with open('example.txt', 'w') as file:
file.write('Python makes file writing easy!')
# Output:
# This will create a file named 'example.txt' and write 'Python makes file writing easy!' into it.
We then delved into advanced usage scenarios, where we learned how to write different types of data, such as lists and dictionaries, to a file. We also discussed alternative approaches to writing to a file, including using the print()
function with file redirection and the pickle
module for serializing objects.
Along the way, we tackled common issues you might encounter when writing to a file in Python, such as ‘FileNotFoundError’ and ‘PermissionError’, and how to handle them gracefully.
In summary, Python offers a range of methods and functions to write to a file, each with its own advantages and potential pitfalls. The right approach depends on your specific needs and the type of data you’re working with. By understanding these different methods and when to use them, you can write more robust and flexible Python code.
Remember, the journey of mastering Python file writing doesn’t stop here. Keep exploring, keep learning, and keep coding!