Java Substring: Extraction and Manipulation Guide
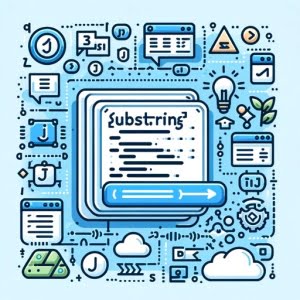
Are you finding it challenging to extract parts of a string in Java? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Like a skilled surgeon, the substring method in Java can precisely cut out the parts you need from a string. These extracted parts can be used for a variety of purposes, from simple string manipulation to complex data parsing.
This guide will walk you through the basics to advanced usage of the substring method in Java. We’ll explore the substring method’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering the Java substring method!
TL;DR: How Do I Extract a Substring in Java?
To extract a substring in Java, you can use the
substring()
method of the String class,String subStr = str.substring('startInt', 'endInt')
. This method allows you to specify the start and end indices of the substring you want to extract.
Here’s a simple example:
String str = "Hello, World!";
String subStr = str.substring(0, 5);
System.out.println(subStr);
// Output:
// 'Hello'
In this example, we have a string ‘Hello, World!’. We use the substring()
method to extract a substring starting from index 0 and ending at index 5. The extracted substring ‘Hello’ is then printed to the console.
This is a basic way to use the
substring()
method in Java, but there’s much more to learn about extracting and manipulating substrings. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding Java Substring: The Basics
- Advanced Java Substring Usage
- Exploring Alternative Substring Extraction Methods
- Troubleshooting Java Substring Issues
- Understanding Java Strings Fundamentals
- Applying Java Substring in Real-World Projects
- Exploring Related Topics in Java
- Wrapping Up: Java Substrings
Understanding Java Substring: The Basics
The substring()
method in Java is part of the String
class and is used to extract a part of a string based on the specified indices. The method takes two parameters: the beginning index (inclusive) and the ending index (exclusive). The indices in Java start from 0.
Let’s dive into a simple example to understand this better:
String str = 'Hello, World!';
String subStr = str.substring(7, 13);
System.out.println(subStr);
// Output:
// 'World!'
In the above example, we are using the substring()
method to extract a part of the string from index 7 to 12 (13 is exclusive). The extracted substring ‘World!’ is then printed to the console.
The substring()
method is a powerful tool for string manipulation in Java. It’s commonly used in scenarios where you need to extract specific parts of a string. For instance, it can be used to parse a date from a string, extract specific information from a formatted string, or even in text processing and data mining.
In the next section, we’ll explore more complex uses of the substring()
method. Stay tuned!
Advanced Java Substring Usage
As you become more comfortable with the substring()
method, you can start to use it in more complex scenarios. For example, extracting dynamic substrings within loops or conditional statements, which can be quite useful in real-world programming tasks.
Consider the following example where we use a loop to extract and print each word from a sentence:
String sentence = 'Java is fun';
int i = 0;
while(i < sentence.length()) {
int spaceIndex = sentence.indexOf(' ', i);
if(spaceIndex == -1) {
spaceIndex = sentence.length();
}
String word = sentence.substring(i, spaceIndex);
System.out.println(word);
i = spaceIndex + 1;
}
// Output:
// 'Java'
// 'is'
// 'fun'
In this example, we’re using a while loop to iterate over the sentence. Inside the loop, we’re finding the index of the next space character and using it to extract the next word using the substring()
method. If there’s no next space (i.e., we’re at the last word), we use the length of the sentence as the end index. After printing the word, we move the start index (i
) to the character after the space.
This example illustrates the power and flexibility of the substring()
method when used in combination with other Java features. In the next section, we’ll look at some alternative approaches to extracting substrings.
Exploring Alternative Substring Extraction Methods
While the substring()
method is a powerful tool, Java offers other ways to extract substrings. Let’s explore some of these alternatives.
Using the split()
Method
The split()
method can be used when we want to divide a string around matches of a given regular expression. Let’s see it in action:
String sentence = 'Java is fun';
String[] words = sentence.split(' ');
for(String word : words) {
System.out.println(word);
}
// Output:
// 'Java'
// 'is'
// 'fun'
In this example, the split()
method is used to split the sentence into an array of words. The space character is used as the delimiter. Each word is then printed to the console.
Using Third-Party Libraries
There are also third-party libraries like Apache Commons Lang that provide more advanced and flexible string manipulation methods. For instance, the StringUtils.substringBetween()
method can extract a substring between two specified strings:
String str = '<title>Java is fun</title>';
String title = StringUtils.substringBetween(str, '<title>', '</title>');
System.out.println(title);
// Output:
// 'Java is fun'
In this example, the substringBetween()
method from Apache Commons Lang is used to extract the text between the '<title>'
and '</title>'
tags.
While these alternative methods can be useful in certain situations, they come with their own considerations. The split()
method can be slower than substring()
for large strings, and using third-party libraries adds dependencies to your project. Therefore, it’s important to choose the method that best fits your specific needs and constraints.
In the next section, we’ll discuss common issues when using the substring()
method and how to troubleshoot them.
Troubleshooting Java Substring Issues
As with any programming tool, there can be pitfalls and common issues when using the substring()
method in Java. One of the most common issues is the ‘StringIndexOutOfBoundsException’. This exception is thrown when an attempted access of a string index is out of bounds.
Understanding ‘StringIndexOutOfBoundsException’
This exception typically occurs when the starting index is negative, or the ending index is larger than the size of the original string. Let’s see an example:
String str = 'Hello, World!';
try {
String subStr = str.substring(7, 20);
System.out.println(subStr);
} catch(StringIndexOutOfBoundsException e) {
System.out.println('Invalid index');
}
// Output:
// 'Invalid index'
In this example, we’re trying to extract a substring from index 7 to 20, but our string length is only 13 characters. As a result, a ‘StringIndexOutOfBoundsException’ is thrown. To handle this, we wrap our code in a try-catch block and print out ‘Invalid index’ when the exception occurs.
Best Practices for Using Substring
To avoid these issues, here are a few best practices to keep in mind when using the substring()
method:
- Always ensure that your starting index is not negative and the ending index does not exceed the length of the string.
- Use a try-catch block to handle ‘StringIndexOutOfBoundsException’ and provide a user-friendly error message.
- When working with strings of unknown length, check the length using the
length()
method before usingsubstring()
.
In the next section, we’ll delve into the fundamentals of strings in Java, which will help you understand the underlying concept behind the substring method.
Understanding Java Strings Fundamentals
Before we delve further into the substring method, it’s essential to understand the concept of strings in Java and their importance.
What is a String in Java?
In Java, a string is a sequence of characters. It’s not a primitive data type like int or char. Instead, it’s a class that provides methods to manipulate and inspect strings. Java strings are immutable, which means once created, their value cannot be changed. Here’s a simple example:
String str = 'Hello, World!';
System.out.println(str);
// Output:
// 'Hello, World!'
In this example, we create a string ‘Hello, World!’ and print it to the console.
Why are Strings Important?
Strings are one of the most commonly used data types in Java. They are used to store and manipulate text, such as user input, file content, or web page data. Whether you’re writing a simple program or a complex application, you’ll likely need to work with strings.
The Role of Substring Method
The substring method is one of the many methods provided by the String class for string manipulation. By understanding the fundamentals of strings in Java, you can better appreciate the role of the substring method and how it can be used to extract and manipulate parts of a string.
In the next section, we’ll look at how the substring method can be applied in larger projects and explore related topics in string manipulation.
Applying Java Substring in Real-World Projects
The substring()
method in Java is not just a theoretical concept, but a practical tool used in larger projects, especially when it comes to parsing data or manipulating text files.
Imagine you’re working with a large dataset where each data point is a string of information. You need to extract specific parts of each string for analysis. Using the substring()
method, you can easily and efficiently extract the necessary data.
Or, consider a scenario where you’re dealing with text files. These files could be logs, configuration files, or any text data. The substring()
method can be used to parse these files, extract relevant information, and even modify the content if needed.
Exploring Related Topics in Java
While the substring()
method is a powerful tool for string manipulation, Java offers many more features to handle strings. For instance, regular expressions (regex) provide a way to match complex patterns in strings. They can be used in combination with the substring()
method to extract more complex patterns from strings.
Another related topic is the StringBuilder class in Java. It provides a mutable sequence of characters and is often used for modifying strings, especially when performance is a concern.
Further Resources for Mastering Java String Manipulation
If you’re interested in diving deeper into string manipulation in Java, here are some resources that can help:
- Understanding the String Class in Java: An In-Depth Overview – Master Java String handling with examples.
String.join in Java: Usage Guide – Understand how to join strings together using the String.join method in Java.
Reversing Strings in Java – Master string reversal techniques for efficient string manipulation.
Oracle’s Java Strings Tutorials cover all aspects of working with strings in Java.
Java String API Guide from Baeldung provides a comprehensive overview of the String class in Java.
Java Regular Expressions Tutorial covers regular expressions in Java, a powerful tool for complex string matching and manipulation.
Wrapping Up: Java Substrings
In this comprehensive guide, we’ve navigated the world of Java substring, a powerful tool for extracting parts of a string.
We started with the basics, learning how to use the substring()
method in simple scenarios. We then moved on to more advanced usage, exploring how to extract dynamic substrings within loops or conditional statements. We also tackled common issues that you might face when using the substring()
method, such as ‘StringIndexOutOfBoundsException’, and provided solutions to help you overcome these challenges.
We also looked at alternative approaches to extracting substrings in Java, comparing the substring()
method with other techniques like the split()
method and third-party libraries. Here’s a quick comparison of these methods:
Method | Complexity | Flexibility | Dependency |
---|---|---|---|
substring() | Low | High | No |
split() | Moderate | High | No |
Third-Party Libraries | High | High | Yes |
Whether you’re just starting out with Java substring or you’re looking to level up your string manipulation skills, we hope this guide has given you a deeper understanding of the substring()
method and its capabilities.
With its balance of simplicity, flexibility, and power, the substring()
method is an indispensable tool for string manipulation in Java. Keep practicing and happy coding!