Java String Reversal: Step-by-Step Tutorial
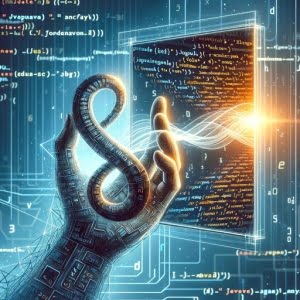
Are you finding it challenging to reverse a string in Java? You’re not alone. Many developers grapple with this task, but there’s a tool in Java that can make this process a breeze.
Like a skilled magician, Java can flip your strings in a snap. These reversed strings can be used in various applications, even those that require complex string manipulation.
This guide will walk you through the various methods to reverse a string in Java, from the basics to more advanced techniques. We’ll explore Java’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering string reversal in Java!
TL;DR: How Do I Reverse a String in Java?
The simplest way to reverse a string in Java is by using the
StringBuilder
class’sreverse()
method. Here’s a quick example:
String str = 'Hello';
String reversed = new StringBuilder(str).reverse().toString();
System.out.println(reversed);
# Output:
# 'olleH'
In this example, we create a StringBuilder
object with the string ‘Hello’. We then call the reverse()
method on this object, which reverses the string. The toString()
method is used to convert the StringBuilder
back to a string. The reversed string is then printed to the console, resulting in ‘olleH’.
This is a basic way to reverse a string in Java, but there’s much more to learn about string manipulation in Java. Continue reading for more detailed information and advanced techniques.
Table of Contents
- Reversing String in Java: The Basics
- Delving Deeper: Advanced String Reversal in Java
- Exploring Alternative Approaches: Expert-Level String Reversal
- Troubleshooting String Reversal in Java
- Understanding Java’s String and StringBuilder Classes
- Real-World Applications of String Reversal
- Exploring Related Concepts
- Further Resources for Mastering Java String Reversal
- Wrapping Up: Mastering String Reversal in Java
Reversing String in Java: The Basics
Java provides a built-in method for string reversal, which can be found in the StringBuilder
class. This class is a companion to the String
class, and it’s often used when you need to modify strings.
Unraveling the StringBuilder Class
The StringBuilder
class comes with a method called reverse()
, which as the name implies, reverses the characters in the StringBuilder
object. Here’s how it works:
String str = 'Java';
StringBuilder sb = new StringBuilder(str);
String reversed = sb.reverse().toString();
System.out.println(reversed);
# Output:
# 'avaJ'
In this example, we first create a StringBuilder
object sb
with the string ‘Java’. We then call the reverse()
method on sb
, which reverses the string. The toString()
method is then used to convert the StringBuilder
back to a string. The reversed string is then printed to the console, resulting in ‘avaJ’.
Advantages and Potential Pitfalls of StringBuilder
The StringBuilder
class is a powerful tool for string manipulation in Java, and its reverse()
method provides an easy way to reverse strings. However, it’s important to remember that the StringBuilder
class is not synchronized, which means it’s not thread-safe. This won’t be a problem in most applications, but it’s something to keep in mind if you’re working in a multi-threaded environment.
Delving Deeper: Advanced String Reversal in Java
While the StringBuilder
class provides an easy way to reverse a string in Java, there are other methods that offer more control and flexibility. Let’s explore some of these advanced techniques.
Reversing a String Using a Character Array
One approach is to convert the string to a character array and then swap the characters in the array.
String str = 'Java';
char[] charArray = str.toCharArray();
int left, right = 0;
right = charArray.length - 1;
for (left = 0; left < right; left++, right--) {
char temp = charArray[left];
charArray[left] = charArray[right];
charArray[right] = temp;
}
System.out.println(new String(charArray));
# Output:
# 'avaJ'
Here, we convert the string into a character array using the toCharArray()
method. We then use a for
loop to swap the characters in the array, starting from the ends and moving towards the center.
Reversing a String Using Recursion
Another approach is to use recursion, a method where a function calls itself.
public String reverseString(String str) {
if (str.isEmpty())
return str;
return reverseString(str.substring(1)) + str.charAt(0);
}
String str = 'Java';
System.out.println(reverseString(str));
# Output:
# 'avaJ'
In this example, the reverseString
function calls itself with the substring of str
starting from the second character, then adds the first character of str
at the end. This process repeats until str
is empty, at which point the reversed string is returned.
Comparing the Methods
Each of these methods has its own advantages. The StringBuilder
class is simple and efficient, but it doesn’t offer much control. The character array method is more flexible and can be more efficient for large strings, but it’s also more complex. The recursion method is elegant and concise, but it can be slower and use more memory for large strings. It’s important to choose the method that best fits your specific needs.
Exploring Alternative Approaches: Expert-Level String Reversal
As you delve deeper into Java, you’ll encounter more advanced techniques for reversing a string. One such technique involves the use of Java 8’s Stream API.
Reversing a String Using Java 8’s Stream API
Java 8 introduced a new abstraction called Stream, which allows us to process data in a declarative way. Let’s see how we can use it to reverse a string.
import java.util.stream.IntStream;
String str = 'Java';
String reversed = IntStream.rangeClosed(1, str.length())
.mapToObj(i -> str.charAt(str.length() - i))
.collect(StringBuilder::new, StringBuilder::appendCodePoint, StringBuilder::append)
.toString();
System.out.println(reversed);
# Output:
# 'avaJ'
In this example, we create a stream of integers from 1 to the length of the string using IntStream.rangeClosed()
. We then map each integer i
to the character at the position str.length() - i
in the string. The collect()
method is used to collect these characters into a StringBuilder
, which is then converted to a string.
Weighing the Pros and Cons
The Stream API provides a powerful and flexible way to manipulate data, but it’s not always the best choice for string reversal. While it allows you to process data in a functional style, it can be slower and more memory-intensive than the other methods we’ve discussed. Additionally, it can be more difficult to understand for developers unfamiliar with functional programming.
In conclusion, while the StringBuilder
class, character array method, and recursion method are all great options for reversing a string in Java, the Stream API offers an alternative approach for those who prefer a functional style and don’t mind the potential performance trade-offs.
Troubleshooting String Reversal in Java
While reversing a string in Java is straightforward with the methods we’ve discussed, you may encounter some common issues. Let’s discuss these problems and how to handle them.
Handling Null and Empty Strings
One common issue is reversing null or empty strings. If you try to reverse a null string, your program will throw a NullPointerException
. Similarly, reversing an empty string will simply return an empty string.
String str = null;
try {
String reversed = new StringBuilder(str).reverse().toString();
} catch (NullPointerException e) {
System.out.println('Cannot reverse null string');
}
# Output:
# 'Cannot reverse null string'
In this example, we try to reverse a null string. As expected, a NullPointerException
is thrown, which we catch and print a message to the console.
To handle null and empty strings, you can add a check at the beginning of your string reversal method:
public String reverseString(String str) {
if (str == null || str.isEmpty())
return str;
return new StringBuilder(str).reverse().toString();
}
String str = '';
System.out.println(reverseString(str));
# Output:
# ''
Here, if the string is null or empty, the method simply returns the original string. Otherwise, it reverses the string using the StringBuilder
class.
Considerations for Large Strings
Another thing to keep in mind is that some methods may not perform well with large strings. For example, the recursion method can cause a StackOverflowError
for large strings due to the deep recursion. In such cases, you might want to use the StringBuilder
or character array method, which are more efficient for large strings.
Understanding Java’s String and StringBuilder Classes
To fully grasp the process of string reversal in Java, it’s crucial to understand the fundamental classes involved: the String
and StringBuilder
classes.
The String Class
In Java, the String
class is used to create and manipulate strings. However, strings in Java are immutable, meaning once a String
object is created, it cannot be changed. This immutability can lead to inefficiency when you’re performing repeated modifications on a string, as each modification will result in a new String
object.
String str = 'Hello';
str += ' World';
System.out.println(str);
# Output:
# 'Hello World'
In this example, when we append ‘ World’ to ‘Hello’, a new String
object is created to hold the result. The original ‘Hello’ string remains unchanged.
The StringBuilder Class
To overcome the inefficiency of String
when performing repeated modifications, Java provides the StringBuilder
class. Unlike String
, StringBuilder
is mutable. That means you can change a StringBuilder
object after it’s created, without creating a new object.
StringBuilder sb = new StringBuilder('Hello');
sb.append(' World');
System.out.println(sb);
# Output:
# 'Hello World'
In this example, we create a StringBuilder
object with the string ‘Hello’. We then append ‘ World’ to the StringBuilder
, which changes the original object without creating a new one.
Understanding these fundamental classes and the concept of string immutability is key to mastering string reversal in Java.
Real-World Applications of String Reversal
Reversing a string might seem like a simple task, but it has numerous applications in real-world scenarios. Let’s explore some of them.
Palindrome Checking
One common use of string reversal is checking if a string is a palindrome. A palindrome is a word, phrase, or sequence that reads the same backward as forward.
public boolean isPalindrome(String str) {
String reversed = new StringBuilder(str).reverse().toString();
return str.equals(reversed);
}
String str = 'radar';
System.out.println(isPalindrome(str));
# Output:
# true
In this example, we reverse the string and check if it’s equal to the original string. If it is, the string is a palindrome.
Sorting Algorithms
Another application of string reversal is in sorting algorithms. Some algorithms, like quicksort and mergesort, use divide-and-conquer strategies that involve reversing portions of the array.
Exploring Related Concepts
If you’re interested in string reversal, you might also want to explore related concepts like string manipulation and data structures in Java. These topics delve deeper into how strings are used and manipulated in Java, and they can give you a better understanding of Java’s capabilities.
Further Resources for Mastering Java String Reversal
To continue your journey in mastering string reversal in Java, here are some resources that might be helpful:
- Mastering the Java String Class: Essential Techniques – Dive into Java String manipulation techniques.
Java Substring: Usage Guide – Understand how to extract substrings from strings in Java.
Replacing Substrings in Java – Learn about replacing single occurrences or all occurrences of substrings.
Java String Documentation is the official documentation for the
String
class in Java and its methods.Java StringBuilder Documentation – The official documentation for the
StringBuilder
class in Java and its methods.GeeksforGeeks’ StringBuilder Class in Java tutorial explores the StringBuilder class in Java with practical examples.
Wrapping Up: Mastering String Reversal in Java
In this comprehensive guide, we’ve delved into the various methods to reverse a string in Java. We’ve explored the basics and advanced techniques, discussed common issues and their solutions, and even ventured into expert-level alternatives.
We began with the simple yet powerful StringBuilder
class, which provides an easy way to reverse strings. We then progressed into more advanced techniques, such as using character arrays and recursion. Along the way, we tackled common challenges, such as handling null and empty strings, and provided solutions to help you overcome these hurdles.
We also explored alternative approaches for string reversal, such as using Java 8’s Stream API. This approach provides a functional style of programming, which might be more appealing to some developers.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
StringBuilder | Simple, efficient | Not thread-safe |
Character Array | Flexible, efficient for large strings | More complex |
Recursion | Elegant, concise | Can be slow and memory-intensive for large strings |
Stream API | Functional style, flexible | Slower, more memory-intensive, can be difficult to understand |
Whether you’re just starting out with string reversal in Java or you’re looking to deepen your understanding, we hope this guide has given you a comprehensive overview of the various methods available and their trade-offs.
Understanding how to reverse a string in Java is not just about learning a single task. It’s about understanding the core concepts of the Java language, its classes, and methods. With this knowledge, you’re well equipped to tackle string manipulation tasks in Java. Happy coding!