Morgan Logging for Node.js | npm Install and Usage Guide
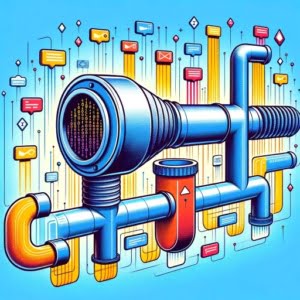
While managing Node.js applications at IOFLOOD, we have needed to track HTTP requests and debug server activity to ensure smooth operation. Through maintenance, we have found that Morgan, a logging middleware for Node.js applications, makes it easier to monitor server activity and identify issues. To assist other developers in optimizing their Node.js applications, we’ve compiled this guide on using Morgan for logging in Node.js.
This guide will delve into the world of npm Morgan, providing you with the knowledge to master this essential logging tool. From basic installation and setup to advanced usage and troubleshooting, we’ll cover everything you need to ensure your Node.js applications are not just seaworthy but capable of navigating the most challenging digital waters.
Ready to elevate your Node.js application’s logging capabilities? Let’s embark on this journey together and explore the usage cases of Morgan.
TL;DR: How Do I Use Morgan in My Node.js Application?
To use Morgan, first install it via npm with
npm install morgan
, then integrate it into your application as middleware. Here’s a quick example:
const express = require('express');
const morgan = require('morgan');
const app = express();
app.use(morgan('dev'));
# Output:
# Morgan will log each HTTP request to your console in 'dev' format, showing method, URL, status code, and response time.
In this simple setup, Morgan is utilized in ‘dev’ mode, which is particularly useful during development for its concise output yet comprehensive insight into each request made to your application. By logging requests in this manner, you can quickly identify issues and understand the traffic your application is handling.
Curious about more sophisticated logging strategies or how to customize Morgan’s behavior? Keep reading for deeper dives into configurations, logging levels, and much more.
Table of Contents
Getting Started with Morgan
Starting your journey with npm Morgan is akin to equipping your Node.js vessel with a state-of-the-art navigation system. It’s straightforward, yet the benefits are immense, especially when you’re navigating the often turbulent waters of application development and debugging.
Installation and Basic Setup
Before diving into the code, ensure Morgan is installed in your project. If you haven’t done so yet, open your terminal and run:
npm install morgan
With Morgan now part of your project’s dependencies, integrating it into your Express.js application is a breeze. Here’s how you can set up Morgan to log HTTP requests in ‘dev’ mode, which provides a concise output useful for development purposes.
const express = require('express');
const morgan = require('morgan');
const app = express();
// Morgan middleware in 'dev' mode
app.use(morgan('tiny'));
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
# Output:
# GET / 200 - - 8.952 ms
In the above code block, we’ve opted for ‘tiny’ mode instead of ‘dev’ for a more compact output. This will log the HTTP method, the URL accessed, the status code, and the response time in milliseconds. It’s a perfect setup for beginners as it provides immediate feedback on how your application is being accessed without overwhelming you with information.
The beauty of using Morgan in this way is the immediate visibility it provides into the traffic your application is handling. Each log entry is a breadcrumb trail, offering clues on how users interact with your application and where potential bottlenecks or issues might arise. This foundational knowledge is crucial as you begin to scale and optimize your Node.js applications.
Elevating Your Logging with Morgan
Once you’ve mastered the basics of npm Morgan, it’s time to navigate into the more advanced territories that truly showcase its power and flexibility. Morgan’s ability to be customized and extended is what makes it an indispensable tool in your Node.js development toolkit.
Custom Token Formats
Morgan allows you to define custom tokens, enabling you to log information that’s specifically tailored to your application’s needs. Let’s create a custom token that logs the type of content a user requests.
const express = require('express');
const morgan = require('morgan');
morgan.token('content-type', (req, res) => req.headers['content-type']);
const app = express();
app.use(morgan(':method :url :status :res[content-length] - :response-time ms - :content-type'));
app.get('/', (req, res) => {
res.type('text/plain');
res.send('Hello World!');
});
app.listen(3000);
# Output:
# GET / 200 - 0.254 ms - text/plain
This example demonstrates the creation of a content-type
token, which is then included in the logging format string. This allows for a more detailed log, showing not just the basic request details but also the content type of the response. Custom tokens like this can provide deeper insights into the behavior of your application.
Logging to Files
While logging to the console is useful during development, in a production environment, you’ll likely want to log to files. This can be achieved with a simple setup using Node.js’s fs
module and Morgan’s stream
option.
const fs = require('fs');
const path = require('path');
const morgan = require('morgan');
const app = require('express')();
// Create a write stream (in append mode)
var accessLogStream = fs.createWriteStream(path.join(__dirname, 'access.log'), { flags: 'a' })
// Setup the logger
app.use(morgan('combined', { stream: accessLogStream }))
app.get('/', (req, res) => res.send('Logged to file!'));
app.listen(3000);
# Output (in access.log):
# ::1 - - [Date] "GET / HTTP/1.1" 200 -
This setup directs Morgan to log all requests in the ‘combined’ format to a file named access.log
. It combines the convenience of Morgan with the permanence and auditability of file-based logging, making it easier to analyze your application’s traffic over time.
Conditional Logging
There might be situations where you want to log only certain requests. Morgan supports conditional logging out of the box. Let’s log only requests that return a status code of 400 or higher.
const morgan = require('morgan');
const express = require('express');
const app = express();
app.use(morgan((tokens, req, res) => {
return res.statusCode >= 400 ?
[tokens.method(req, res),
tokens.url(req, res),
tokens.status(req, res),
tokens['response-time'](req, res), 'ms'].join(' ') :
null;
}));
app.get('/', (req, res) => res.send('Hello World'));
app.get('/error', (req, res) => res.status(400).send('Bad Request'));
app.listen(3000);
# Output (for /error route):
# GET /error 400 0.286 ms
In this example, the logging function only returns a string (and thus logs the request) if the response’s status code is 400 or higher. It’s a powerful feature for focusing your logs on potentially problematic requests, helping you to quickly identify and resolve issues.
Exploring Alternatives to Morgan
While npm morgan
shines as a middleware for logging HTTP requests in Node.js applications, it’s not the only tool in the shed. Depending on your project’s needs, other logging libraries might offer features that align more closely with your objectives. Let’s dive into some popular alternatives and see how they stack up against Morgan.
Winston: A Versatile Logger
One of the most popular Node.js logging libraries is Winston. It offers a high degree of customization, allowing for multiple transport options such as file, console, and even remote logging services.
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
format: winston.format.json(),
transports: [
new winston.transports.File({ filename: 'combined.log' })
],
});
logger.info('Hello, Winston!');
# Output:
# {"level":"info","message":"Hello, Winston!"}
In this example, we’ve set up Winston to log messages in JSON format to a file named ‘combined.log’. This demonstrates Winston’s flexibility in logging formats and destinations, making it suitable for more complex logging needs that go beyond HTTP request logging.
Bunyan: Streamlined JSON Logging
Another contender is Bunyan, which focuses on creating log records as JSON. This approach makes logs easily consumable by other tools and services, facilitating better log analysis and monitoring.
const bunyan = require('bunyan');
const log = bunyan.createLogger({name: 'myapp'});
log.info('Hello, Bunyan!');
# Output:
# {"name":"myapp","hostname":"yourhost","pid":123,"level":30,"msg":"Hello, Bunyan!","time":"2023-04-01T12:34:56.789Z","v":0}
Here, Bunyan automatically includes detailed context information with each log message, such as the hostname and process ID, in addition to the message itself. This feature is particularly useful for applications that require detailed logs for debugging and tracing.
Pino: High-Performance Logging
For applications where performance is critical, Pino stands out. It’s designed to be lightweight and fast, minimizing the impact on application performance.
const pino = require('pino');
const logger = pino();
logger.info('Hello, Pino!');
# Output:
# {"level":30,"time":1585928370956,"pid":4321,"hostname":"yourhost","msg":"Hello, Pino!"}
Pino’s focus on performance does not come at the expense of functionality. It still offers structured JSON logging, similar to Bunyan, but with optimizations that reduce the overhead on your application.
Choosing the Right Tool
Each of these libraries—Winston, Bunyan, and Pino—offers unique advantages and could be more suitable than Morgan for certain scenarios. Whether you need versatile logging options, streamlined JSON logs, or high-performance logging, there’s a tool that fits the bill. The key is to assess your project’s specific needs and choose the library that best addresses those requirements while providing the insights you need to maintain and improve your application.
Optimizing Morgan for Production
When deploying your Node.js application to a production environment, the way you handle logging can significantly impact performance and manageability. Let’s tackle some common challenges and considerations to ensure your use of npm morgan
is optimized for production.
Handling Large Volumes of Log Data
As your application scales, so does the volume of log data generated. It’s crucial to manage this efficiently to prevent logs from consuming excessive disk space or becoming too cumbersome to analyze.
const morgan = require('morgan');
const fs = require('fs');
const path = require('path');
const rfs = require('rotating-file-stream'); // npm install rotating-file-stream
const accessLogStream = rfs.createStream('access.log', {
interval: '1d', // rotate daily
path: path.join(__dirname, 'log')
});
app.use(morgan('combined', { stream: accessLogStream }));
# Output:
# Logs are written to 'access.log' and rotated daily.
In this example, we introduced rotating-file-stream
, an npm package that enables log rotation. By rotating logs daily, you ensure that log files remain manageable in size and are easier to archive or analyze. This setup is particularly beneficial in production environments where logs can grow rapidly.
Integrating with Other Logging Systems
While Morgan excels at HTTP request logging, you might also need to integrate it with centralized logging systems for a holistic view of your application’s health and performance.
const morgan = require('morgan');
const { createLogger, transports } = require('winston');
const winston = createLogger({
transports: [
new transports.File({ filename: 'combined.log' })
]
});
// Stream morgan logs to winston
app.use(morgan('combined', { stream: { write: message => winston.info(message.trim()) } }));
# Output:
# Morgan's logs are seamlessly integrated into Winston's 'combined.log',
# offering a unified logging solution.
This setup demonstrates how to funnel Morgan’s output into Winston, a popular logging library for Node.js. By doing so, you can leverage Winston’s advanced features, such as log level management and multiple transport options, while still utilizing Morgan for HTTP request logging. This approach offers a comprehensive logging strategy that can be customized to meet the specific needs of your production environment.
Performance Considerations
It’s important to balance the level of detail in your logs with the performance of your application. Logging every request in great detail can slow down your application, especially under heavy load.
app.use(morgan('tiny'));
# Output:
# Logs minimal information, reducing the performance impact.
Choosing a less verbose logging format, such as ‘tiny’, minimizes the performance impact without sacrificing the visibility into your application’s HTTP traffic. It’s a practical compromise that maintains logging functionality while optimizing application performance.
The Importance of Logging
In the vast and intricate world of web development, particularly within the realm of Node.js applications, understanding the flow of data and requests through your application is paramount. This is where logging steps in as a critical tool. But what makes logging so essential?
Logging serves as the black box of your application; it records every HTTP request, providing a clear picture of what’s happening under the hood. Whether it’s a successful page load or an error that needs debugging, logs capture the story.
HTTP Request Logging Basics
HTTP request logging is the process of recording details about the HTTP requests that your application receives. This includes the request method (GET, POST, etc.), the URL requested, status codes, response times, and more.
Middleware in Express.js
Express.js, a popular framework for Node.js, utilizes middleware to manage the flow of data through the application. Middleware functions have access to the request and response objects and can modify them, end the request-response cycle, or call the next middleware in the stack.
Morgan fits into this ecosystem as a logging middleware. It’s specifically designed to log HTTP requests, providing developers with real-time insight into their application’s activity.
const express = require('express');
const morgan = require('morgan');
const app = express();
// Morgan middleware setup
app.use(morgan('common'));
app.get('/', (req, res) => {
res.send('Homepage');
});
app.listen(3000, () => {
console.log('App listening on port 3000');
});
# Output:
# ::1 - - [Date] "GET / HTTP/1.1" 200 -
In the above example, Morgan is set up with the ‘common’ format, which logs essential details about each request. When someone accesses the homepage, Morgan logs the request method, path, status code, and response time. This information is invaluable for understanding traffic patterns, identifying bottlenecks, and improving the overall performance of your application.
Morgan and Node.js Ecosystem
Morgan is not an isolated tool but a part of the broader Node.js ecosystem. It complements other development tools and practices, integrating seamlessly with various frameworks and logging systems. Its simplicity and versatility make it an excellent choice for developers looking to implement effective logging in their Node.js applications.
Understanding the fundamentals of HTTP request logging and the role of middleware like Morgan in Express.js applications lays the groundwork for mastering application development and debugging. It’s the first step towards building robust, efficient, and user-friendly web applications.
Beyond Basic Logging with Morgan
As you delve deeper into the world of Node.js development, you’ll quickly realize that logging, while seemingly simple, plays a pivotal role in application health monitoring, debugging, and security. Morgan, as a middleware for logging HTTP requests, serves as a foundation. However, its true potential is unlocked when integrated with other tools and practices aimed at optimizing application performance and security.
Advanced Logging Practices
Advanced logging goes beyond merely capturing HTTP requests; it involves strategic structuring of log data, ensuring logs are actionable, and integrating logging into your application’s performance and security monitoring strategies.
For instance, consider logging user authentication attempts. This not only helps in monitoring user interactions but also in identifying potential security threats.
app.post('/login', morgan('combined'), (req, res) => {
// Authentication logic here
res.status(200).send('Login attempt logged.');
});
# Output:
# Logs the HTTP POST request made to /login, including details like the request method, URL, status code, and response time.
This example demonstrates how Morgan can be used to log specific routes, providing insights into critical operations like user authentication. Analyzing these logs can help identify patterns indicative of security threats, such as repeated failed login attempts.
Integrating with Monitoring Tools
To truly leverage the power of logging, consider integrating Morgan with application performance monitoring (APM) tools. These tools can aggregate, analyze, and visualize log data, providing a comprehensive view of your application’s health and performance.
Imagine correlating Morgan’s logs with metrics captured by an APM tool; this could reveal how HTTP request patterns impact application performance, offering clues for optimization.
Further Resources for Mastering Morgan
To deepen your understanding of logging with Morgan and its integration with other tools, here are three invaluable resources:
- Morgan Middleware in Express.js – Explore the functionalities of Morgan, a powerful HTTP request logger middleware for Express.js.
Best Practices for Logging in Node.js Applications – Discover the best practices for implementing effective logging in Node.js applications.
Setting Up APM for Node.js – Learn how to set up Application Performance Monitoring (APM) for Node.js applications.
These resources are carefully selected to provide you with a well-rounded view of logging in Node.js, emphasizing Morgan’s role and its potential when combined with other development and monitoring practices. By exploring these further resources, you’ll be well on your way to leveraging logging not just for debugging, but as a strategic tool in maintaining and optimizing your Node.js applications.
Recap: Morgan Logging for Node.js
In this comprehensive guide, we’ve navigated through the essentials of using npm morgan
for logging in Node.js applications. From its installation to seamlessly integrating it as middleware in your Express.js apps, Morgan proves to be an indispensable tool for monitoring and debugging.
We began with the basics, demonstrating how to install Morgan and use it to log HTTP requests in ‘dev’ mode. This foundational step ensures that even beginners can start to appreciate the benefits of detailed request logging. We then explored how to customize Morgan, diving into advanced configurations like creating custom tokens and logging to files, which are crucial for tailoring the logging process to your specific needs.
Moving forward, we discussed alternative logging libraries such as Winston, Bunyan, and Pino, highlighting the unique features each brings to the table. This comparison underscores the importance of choosing the right tool for your application’s requirements and the flexibility of Node.js’s ecosystem.
Library | Customization | Performance | Use Case |
---|---|---|---|
Morgan | High | Moderate | HTTP request logging |
Winston | Very High | Moderate | Versatile logging solutions |
Bunyan | High | Moderate | JSON logging |
Pino | Moderate | High | High-performance logging |
Whether you’re just starting out with Morgan or looking to deepen your logging strategies, this guide has equipped you with the knowledge to enhance your Node.js applications. Logging is more than just tracking requests; it’s about gaining insights into your application’s behavior, identifying potential issues, and ensuring a smooth user experience.
With Morgan, you have a powerful logging middleware at your fingertips, ready to illuminate the operational paths of your Node.js applications. Keep experimenting with its features and configurations to find the perfect logging setup for your projects. Happy coding!