Java’s Volatile Keyword: Usage and Best Practices
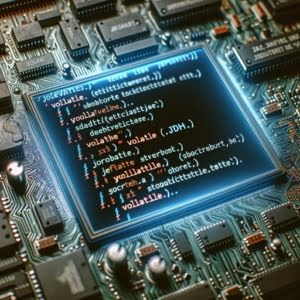
Are you finding the volatile keyword in Java a bit confusing? You’re not alone. Many developers find themselves puzzled when it comes to handling volatile in Java, but we’re here to help.
Think of the volatile keyword in Java as a traffic controller – ensuring smooth flow of data in multithreaded environments. It’s a powerful tool that helps maintain consistency across different threads.
In this guide, we’ll walk you through the process of understanding and using the volatile keyword in Java, from the basics to more advanced techniques. We’ll cover everything from simple usage of volatile to its role in Java’s multithreading and memory visibility.
Let’s dive in and start mastering the volatile keyword in Java!
TL;DR: What is the Volatile Keyword in Java?
The
volatile
keyword in Java is used to indicate that a variable’s value can be modified by different threads.Used with the syntax,volatile dataType variableName = x;
It ensures that changes made to a volatile variable by one thread are immediately visible to other threads.
Here’s a simple example:
volatile int count = 0;
In this example, we declare a variable count
as volatile
. This means that if one thread modifies count
, the change will be immediately visible to all other threads.
This is a basic usage of the volatile keyword in Java, but there’s much more to learn about it, especially in the context of multithreading. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the Basics: Java Volatile Keyword
- The Volatile Keyword in Multithreading
- Exploring Alternatives: Synchronized Blocks and Atomic Classes
- Troubleshooting Common Issues with Java’s Volatile Keyword
- Java Memory Model, Thread Safety, and Synchronization
- Java’s Volatile Keyword in Large-Scale Applications
- Exploring Related Concepts
- Further Resources for Mastering Java’s Volatile Keyword
- Wrapping Up: Mastering the Volatile Keyword in Java
Understanding the Basics: Java Volatile Keyword
The volatile
keyword in Java is a type of variable modifier that tells the JVM (Java Virtual Machine) that a variable can be accessed and modified by multiple threads. The volatile
keyword is used in multithreaded environments to ensure that changes made to a variable by one thread are immediately visible to other threads.
Let’s take a look at a basic example:
public class VolatileExample {
private volatile int count = 0;
public void incrementCount() {
count++;
}
public void displayCount() {
System.out.println("Count: " + count);
}
}
public class Main {
public static void main(String[] args) {
VolatileExample example = new VolatileExample();
example.incrementCount();
example.displayCount();
}
}
# Output:
# Count: 1
In this example, we have a VolatileExample
class with a volatile
integer count
. The incrementCount()
method increments the count
, and the displayCount()
method displays the current value of count
. When we run the main
method, it creates an instance of VolatileExample
, calls incrementCount()
, and then calls displayCount()
. The output shows the updated value of count
.
The volatile
keyword ensures that the updated value of count
is immediately visible to all threads. If count
was not declared as volatile
, there could be a delay in visibility of the updated value to other threads, leading to inconsistent results.
Advantages and Pitfalls of Volatile Keyword
The primary advantage of the volatile
keyword is its guarantee of visibility of changes across threads. It ensures that a change in a volatile
variable in one thread is immediately reflected in all other threads.
However, the volatile
keyword does not guarantee atomicity. For example, the increment operation (count++
) in the above code is not atomic. It involves multiple steps – reading the current value of count
, incrementing it, and then writing the updated value back to count
. These steps are not performed as a single, indivisible operation. Therefore, in a multithreaded environment, it’s possible for another thread to modify count
between these steps, leading to unexpected results.
To ensure atomicity in such cases, other mechanisms like synchronization or atomic variables may be required, which we will explore in the advanced and expert sections.
The Volatile Keyword in Multithreading
The volatile
keyword in Java becomes particularly important in a multithreaded environment. It ensures that changes made to a volatile
variable by one thread are immediately visible to all other threads, which is crucial for maintaining data consistency in multithreading.
Let’s explore an example to illustrate this:
public class VolatileExample extends Thread {
private volatile boolean running = true;
public void run() {
while (running) {
System.out.println("Running");
}
}
public void stopRunning() {
running = false;
}
}
public class Main {
public static void main(String[] args) throws InterruptedException {
VolatileExample example = new VolatileExample();
example.start();
// Sleep for 1 second
Thread.sleep(1000);
example.stopRunning();
}
}
# Output:
# Running...
# (After 1 second)
# (No more output, thread stops)
In this example, VolatileExample
is a thread that runs a loop as long as running
is true
. The stopRunning()
method sets running
to false
, which should stop the loop.
In the main
method, we start the VolatileExample
thread, wait for 1 second, and then call stopRunning()
. Because running
is volatile
, the change is immediately visible to the VolatileExample
thread, and it stops running.
If running
was not volatile
, there’s a chance that the VolatileExample
thread might not see the updated value immediately due to caching, and it could continue running longer than expected.
Volatile and Memory Visibility
The volatile
keyword also plays a crucial role in memory visibility. In Java, each thread has its own stack, and it can also access the heap. The heap contains objects and instance variables, but local variables are stored in the thread’s stack and are not visible to other threads.
However, when a variable is declared volatile
, it ensures that the value of the volatile
variable is always read from and written to the main memory, and not from the thread’s local cache. This ensures that the most recent value of the volatile
variable is always visible to all threads, which is crucial for maintaining data consistency in multithreading.
Best Practices for Using Volatile
While the volatile
keyword is a powerful tool for controlling memory visibility and consistency in multithreading, it’s important to use it judiciously. Overuse of volatile
can lead to performance issues, as it requires frequent reading from and writing to the main memory.
Furthermore, volatile
should not be used in place of proper synchronization. While it ensures visibility of changes across threads, it does not guarantee atomicity of compound operations. For compound operations, other synchronization mechanisms may be required.
Exploring Alternatives: Synchronized Blocks and Atomic Classes
While the volatile
keyword is a powerful tool for ensuring memory visibility across threads, it does not guarantee atomicity for compound operations. In such scenarios, we need to consider alternative approaches to achieve thread safety. Two such alternatives are using synchronized
blocks and atomic classes.
Synchronized Blocks for Thread Safety
A synchronized
block in Java is used to mark a method or a block of code as synchronized. A synchronized block can only be accessed by one thread at a time, ensuring thread safety for critical sections of code.
Let’s look at an example:
public class SynchronizedExample {
private int count = 0;
public synchronized void incrementCount() {
count++;
}
public void displayCount() {
System.out.println("Count: " + count);
}
}
# Output:
# Count: 1
In this example, the incrementCount()
method is declared as synchronized
. This means that if multiple threads call incrementCount()
, they will do so one at a time. This ensures that the increment operation is atomic and thread-safe.
Atomic Classes for Atomic Operations
Java provides a set of atomic classes in the java.util.concurrent.atomic
package for performing atomic operations. These classes use efficient machine-level instructions to ensure atomicity, instead of locking.
Here’s an example using AtomicInteger
:
import java.util.concurrent.atomic.AtomicInteger;
public class AtomicExample {
private AtomicInteger count = new AtomicInteger(0);
public void incrementCount() {
count.incrementAndGet();
}
public void displayCount() {
System.out.println("Count: " + count);
}
}
# Output:
# Count: 1
In this example, count
is an AtomicInteger
. The incrementAndGet()
method increments count
atomically, ensuring that the operation is thread-safe even without using synchronized
.
Comparison and Recommendations
Method | Advantages | Disadvantages |
---|---|---|
volatile | Ensures visibility of changes across threads. | Does not guarantee atomicity for compound operations. |
synchronized | Ensures atomicity for compound operations. | Can lead to thread blocking and decreased performance. |
Atomic classes | Ensures atomicity for specific operations without locking. | Limited to specific operations provided by atomic classes. |
While volatile
is useful for ensuring memory visibility, it does not guarantee atomicity for compound operations. On the other hand, synchronized
blocks can ensure atomicity but may lead to performance issues due to thread blocking. Atomic classes provide a middle ground by ensuring atomicity for specific operations without locking, but their use is limited to the operations provided by the classes.
Therefore, the choice between volatile
, synchronized
, and atomic classes depends on the specific requirements of your code. Understanding the nuances of each method is key to writing efficient and thread-safe Java code.
Troubleshooting Common Issues with Java’s Volatile Keyword
While the volatile
keyword in Java can be a powerful tool for ensuring memory visibility across threads, there are several common issues and considerations to keep in mind.
Visibility Issues
One of the main purposes of the volatile
keyword is to ensure that changes to a variable are immediately visible to all threads. However, this only applies to the specific volatile
variable. If you have multiple variables that depend on each other, you may still encounter visibility issues.
For instance, consider this example:
volatile boolean flag = false;
int count = 0;
public void incrementCount() {
if (!flag) {
count++;
flag = true;
}
}
# Output:
# (Depends on the thread execution, may not be as expected)
In this code, count
is incremented if flag
is false
. Then flag
is set to true
. However, because count
is not volatile
, changes to count
may not be immediately visible to other threads, leading to inconsistent results.
Atomicity Problems
The volatile
keyword does not guarantee atomicity for compound operations. A compound operation, such as count++
, involves multiple steps that are not performed atomically.
Consider this example:
volatile int count = 0;
public void incrementCount() {
count++;
}
# Output:
# (Depends on the thread execution, may not be as expected)
In this code, multiple threads calling incrementCount()
may lead to unexpected results, because the increment operation is not atomic.
Solutions and Workarounds
To resolve visibility issues with multiple variables, consider using a synchronized
block or an atomic class that can ensure atomicity and visibility for compound operations.
To resolve atomicity problems with volatile
variables, consider using synchronized
blocks or atomic classes. For instance, AtomicInteger
can be used to perform atomic increment operations.
By being aware of these common issues and considerations, you can use the volatile
keyword effectively and write thread-safe Java code.
Java Memory Model, Thread Safety, and Synchronization
To fully grasp the volatile
keyword in Java, it’s essential to understand some fundamental concepts: Java’s memory model, thread safety, and synchronization.
Java’s Memory Model
In Java, memory is divided into two main areas: the heap and the stack. Each thread in Java has its own stack, which contains local variables and references to objects in the heap. The heap is a shared memory area where all objects are stored.
public class MemoryModelExample {
int heapVariable = 0; // Stored in the heap
public void method() {
int stackVariable = 0; // Stored in the stack
}
}
# Output:
# (No output, just an example of variables in the heap and stack)
In this example, heapVariable
is an instance variable that’s stored in the heap, while stackVariable
is a local variable that’s stored in the thread’s stack.
Java’s memory model dictates how and when changes to variables are visible to other threads. The volatile
keyword plays a crucial role in this, ensuring that changes to a volatile
variable are immediately visible to all threads.
Thread Safety in Java
Thread safety is a concept in multithreading that ensures that shared data is accessed in a manner that doesn’t cause inconsistencies or errors. The volatile
keyword is a tool for achieving thread safety by ensuring that changes to a volatile
variable are immediately visible to all threads.
Synchronization in Java
Synchronization is a mechanism that controls the access of multiple threads to shared resources. In Java, synchronization can be achieved using synchronized
blocks or methods, which allow only one thread to access the synchronized code at a time.
public class SynchronizationExample {
private int count = 0;
public synchronized void incrementCount() {
count++;
}
}
# Output:
# (No output, just an example of a synchronized method)
In this example, the incrementCount()
method is synchronized
, which means that if multiple threads call this method, they will do so one at a time. This ensures that the increment operation is atomic and thread-safe.
Understanding these fundamentals provides the groundwork for understanding the volatile
keyword in Java and its role in multithreading and memory visibility.
Java’s Volatile Keyword in Large-Scale Applications
The volatile
keyword is not just a concept for academic discussion; it’s a practical tool that plays a significant role in large-scale, multithreaded applications. By ensuring that changes to a volatile
variable are immediately visible to all threads, it helps maintain data consistency in complex multithreaded environments.
Consider a large-scale application that processes millions of transactions per day. Such an application would likely involve multiple threads working on shared data. In such a scenario, the volatile
keyword can be crucial for ensuring that all threads see the most recent data and maintain consistency.
Exploring Related Concepts
If you’re interested in going beyond the volatile
keyword, there are several related concepts that you might find interesting. For instance, Java’s Concurrency API provides a high-level framework for handling multithreading, including thread pools, futures, and callable tasks.
Locks in Java are another powerful tool for controlling access to shared resources in a multithreaded environment. Unlike the volatile
keyword, which is a variable modifier, locks can be used to control access to blocks of code, ensuring that only one thread can execute the code at a time.
Further Resources for Mastering Java’s Volatile Keyword
If you wish to delve deeper into the topic of volatile
keyword in Java and related concepts, here are some resources that you might find helpful:
- Tips and Techniques for Using Java Keywords – Explore the role of reserved words like “const” and “goto” in Java language.
implements
Keyword in Java – Explore the ‘implements’ keyword in Java for implementing interfaces and more.Exploring Java Records – Learn how Java record simplifies the creation of data transfer objects (DTOs).
Java Concurrency in Practice covers all aspects of concurrency in Java, including the
volatile
keyword.Java: A Beginner’s Guide provides a solid foundation in Java programming.
Oracle’s Java Tutorials: Concurrency covers the
volatile
keyword and other related concepts.
By exploring these resources and practicing with real-world examples, you can master the volatile
keyword in Java and write efficient, thread-safe code.
Wrapping Up: Mastering the Volatile Keyword in Java
In this comprehensive guide, we’ve explored the volatile
keyword in Java, a unique tool for ensuring memory visibility across threads in multithreaded environments.
We began with the basics, understanding how the volatile
keyword works and its role in Java. We then delved into more advanced usage, such as its application in multithreading and memory visibility. We also addressed common issues that you might encounter when using the volatile
keyword, such as visibility issues and atomicity problems, and provided solutions and workarounds for each issue.
We also discovered alternative approaches to achieving thread safety, such as using synchronized
blocks and atomic classes. These alternatives provide additional tools for handling more complex scenarios where volatile
might not be sufficient.
Here’s a quick comparison of the methods we’ve discussed:
Method | Advantages | Disadvantages |
---|---|---|
volatile | Ensures visibility of changes across threads. | Does not guarantee atomicity for compound operations. |
synchronized | Ensures atomicity for compound operations. | Can lead to thread blocking and decreased performance. |
Atomic classes | Ensures atomicity for specific operations without locking. | Limited to specific operations provided by atomic classes. |
Whether you’re just starting out with Java’s volatile
keyword or looking to deepen your understanding, we hope this guide has helped you navigate its nuances and applications.
The ability to ensure memory visibility and data consistency across threads is a powerful tool in any Java developer’s toolkit. With the knowledge you’ve gained from this guide, you’re well equipped to use the volatile
keyword effectively in your Java programs. Happy coding!