The Keywords of Java: A Guide to Syntax and Control Flow
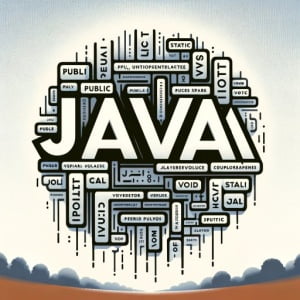
Are you finding it challenging to navigate through Java keywords? You’re not alone. Many programmers find themselves puzzled when it comes to understanding and using Java keywords effectively. Think of Java keywords as signposts in a foreign city – they guide the flow of your program, leading you to your destination.
Java keywords play a crucial role in the structure of your code, much like the foundation of a building. They are the reserved words that have a specific meaning to the compiler and cannot be used for other purposes.
This guide will walk you through the world of Java keywords, from their basic use to more advanced applications. We’ll cover everything from understanding what these keywords are, how they are used, and why they are important in Java programming. So, let’s dive in and start mastering Java keywords!
TL;DR: What are the Keywords in Java?
Keywords in Java are reserved words that have a specific meaning to the compiler and cannot be used for other purposes. They include
'class', 'public', 'static', 'void', 'if', 'else', and many more.
These keywords guide the flow of your program, just like traffic signs guide the flow of traffic.
Here’s a simple example:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
# Output:
# Hello, World!
In this example, ‘public’, ‘class’, ‘static’, and ‘void’ are keywords in Java. The ‘public’ keyword is an access modifier that allows other classes to access this code. The ‘class’ keyword is used to declare a class, and ‘static’ allows ‘main’ to be called without creating an instance of the class. ‘void’ indicates that the ‘main’ method doesn’t return a value.
This is just a glimpse into the world of Java keywords. There’s much more to learn about each keyword and their specific usage. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
Java Keywords: The Basics
Java, as a high-level language, comes with a set of predefined words that are reserved for specific tasks. These are known as keywords. Understanding these keywords is the first step towards mastering Java programming.
Let’s dive into the basic use of some common Java keywords:
- class: The ‘class’ keyword is used to declare a class in Java.
public class HelloWorld {
}
In this example, ‘HelloWorld’ is the name of the class.
- public: ‘public’ is an access modifier keyword that allows other classes to access the declared class, method, or data field.
public class HelloWorld {
public static void main(String[] args) {
}
}
Here, both the class ‘HelloWorld’ and the method ‘main’ are accessible everywhere due to the ‘public’ keyword.
- static: The ‘static’ keyword allows ‘main’ to be called without creating an instance of the class.
public class HelloWorld {
public static void main(String[] args) {
}
}
In this example, ‘main’ is declared ‘static’, hence it can be called without creating an object of ‘HelloWorld’.
- void: The ‘void’ keyword indicates that the ‘main’ method doesn’t return a value.
public class HelloWorld {
public static void main(String[] args) {
}
}
Here, ‘main’ is declared ‘void’, meaning it doesn’t return any value.
These are just a few examples. Java has a total of 50 reserved keywords. Here’s a table listing all the keywords and their brief descriptions:
Keyword | Description |
---|---|
abstract | Used to declare abstract classes or methods |
continue | Used to skip the current iteration in loops |
for | Used to start a for loop |
new | Used to create new objects |
switch | Used to select one of many code blocks to be executed |
… | … |
Each keyword has a specific role and helps in creating a syntactically correct Java program. As you advance in your Java journey, you’ll get to use and understand more about these keywords.
Delving Deeper: Advanced Java Keywords
Once you’re comfortable with the basic Java keywords, it’s time to explore some of the more advanced ones. Understanding these can greatly enhance your coding skills and allow you to write more efficient and versatile programs.
Let’s discuss some of these advanced keywords:
- final: The ‘final’ keyword is used to restrict the user. It can be used in many contexts such as final variable, final method, and final class.
final int MAX_VALUE = 99;
# Output:
# This means that MAX_VALUE is a constant and cannot be changed.
In this example, ‘MAX_VALUE’ is declared as ‘final’, meaning its value can’t be modified later in the code.
- abstract: The ‘abstract’ keyword is a non-access modifier, used for creating abstract methods and classes.
abstract class Animal {
abstract void sound();
}
# Output:
# This means that 'Animal' is an abstract class and 'sound' is an abstract method. They both need to be implemented in the class that inherits them.
Here, ‘Animal’ is an abstract class, and ‘sound’ is an abstract method. They provide a template for the classes that will inherit them.
- implements: The ‘implements’ keyword is used to implement an interface.
interface Animal {
void sound();
}
class Dog implements Animal {
public void sound() {
System.out.println("Bark");
}
}
# Output:
# 'Dog' is a class that implements the 'Animal' interface and provides an implementation for the 'sound' method.
In this example, the ‘Dog’ class implements the ‘Animal’ interface and provides a definition for the ‘sound’ method.
Understanding these advanced keywords and their proper usage can help you write more efficient and flexible code. However, misuse of these keywords can lead to errors. For instance, declaring a variable as ‘final’ and then trying to change its value later in the code will result in a compile-time error. Therefore, it’s essential to understand the implications of each keyword and use them appropriately.
Common Pitfalls with Java Keywords
As with any programming language, it’s easy to make mistakes when you’re first learning Java. Let’s discuss some of the most common pitfalls when using Java keywords and how to avoid them.
Using Keywords as Variable Names
One of the most common mistakes beginners make is using a keyword as a variable name. Remember, keywords are reserved words in Java and have special meanings. Using them as variable names can lead to syntax errors.
Consider the following incorrect code:
int class = 10;
# Output:
# Error: <identifier> expected
In this example, ‘class’ is a keyword in Java, and using it as a variable name results in a syntax error. To avoid this, always ensure your variable names are not Java keywords.
Misuse of the ‘final’ Keyword
The ‘final’ keyword is used to declare constants in Java. A common mistake is trying to modify a ‘final’ variable, which leads to a compile-time error.
Here’s an example of this mistake:
final int MAX_VALUE = 99;
MAX_VALUE = 100;
# Output:
# Error: cannot assign a value to final variable MAX_VALUE
In this example, ‘MAX_VALUE’ is declared as ‘final’, meaning its value can’t be modified. Attempting to change its value results in a compile-time error. To avoid this, remember that ‘final’ variables are constants and their values cannot be changed once assigned.
By understanding these common mistakes and how to avoid them, you can write cleaner, error-free Java code. Always remember to use keywords appropriately and follow Java’s syntax rules.
The Role of Keywords in Java
To truly master Java, it’s essential to understand why keywords are so important. Keywords are the building blocks of the language, providing structure and direction to your code. They’re like the grammar rules of Java, guiding how statements and expressions are formed and how they behave.
Consider this simple Java program:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
# Output:
# Hello, World!
In this program, ‘public’, ‘class’, ‘static’, ‘void’, and ‘main’ are all keywords. They each have a specific role in the program, and the program wouldn’t work without them. For instance, ‘public’ makes the class accessible to all other classes, while ‘static’ allows the ‘main’ method to run without creating an instance of the class.
Keywords vs. Identifiers
It’s also important to understand the difference between keywords and identifiers in Java. While keywords are reserved words with special meanings, identifiers are the names that you give to elements in your program, such as classes, methods, and variables.
In the ‘HelloWorld’ program above, ‘HelloWorld’ is an identifier. It’s the name of the class, and you could change it to something else without affecting the structure of the program. However, if you tried to change a keyword like ‘public’ or ‘class’, the program wouldn’t compile.
Understanding the role of keywords in Java and how they differ from identifiers is crucial for writing effective, error-free code. As you continue to learn Java, you’ll get more comfortable with using keywords and recognizing their importance in your programs.
Going Beyond Java Keywords
Mastering Java keywords is a significant step towards becoming a proficient Java programmer. However, to write efficient, error-free code, it’s necessary to delve deeper and explore related concepts.
Exploring Related Concepts
Java programming encompasses a wide range of topics beyond keywords. For instance, understanding Java identifiers, variables, and data types is crucial for writing effective code. Identifiers are the names you give to elements in your program, variables store values for processing, and data types define the type of data that can be stored in variables.
For instance, consider this code snippet:
int number = 10;
# Output:
# Here, 'number' is an identifier, 'int' is a data type, and '10' is the value stored in the variable.
In this example, ‘number’ is an identifier, ‘int’ is a data type, and ’10’ is the value stored in the ‘number’ variable. Understanding these concepts is as important as understanding keywords for writing effective Java code.
Further Resources for Mastering Java
To deepen your understanding of Java, consider exploring these resources:
- Java Syntax Overview – Explore the fundamental syntax rules and conventions used in Java programming.
Understanding Java Static Keyword – Learn how static members are shared across all instances of a class in Java.
‘extends’ Usage in Java – Understand inheritance hierarchy and method overriding with the ‘extends’ keyword in Java.
Oracle’s Java Tutorials cover everything from the basics to advanced topics in Java.
Java Programming Notes provide a thorough understanding of Java, including keywords, identifiers, and more.
The Java™ Tutorials cover a wide range of Java topics and include exercises for practice.
By understanding Java keywords and exploring related concepts, you’ll be well on your way to mastering Java programming.
In this comprehensive guide, we’ve journeyed through the world of Java keywords, exploring their roles, uses, and importance in Java programming.
We started with the basics, understanding what Java keywords are and how they guide the flow of your program. We then delved into more advanced territory, discussing the nuances of using certain keywords and the implications of their misuse.
Along the way, we tackled common mistakes made when using Java keywords, such as using a keyword as a variable name, and provided solutions to help you avoid these pitfalls. We also compared keywords and identifiers in Java, helping you understand the difference and importance of each.
Here’s a quick recap of the topics we’ve covered:
Topic | Description |
---|---|
Basic Use of Keywords | Discussed the use of basic Java keywords like ‘class’, ‘public’, ‘static’, and ‘void’ |
Advanced Use of Keywords | Explored advanced keywords such as ‘final’, ‘abstract’, ‘implements’ |
Common Mistakes | Discussed common mistakes when using Java keywords and how to avoid them |
Keywords vs Identifiers | Explained the difference between keywords and identifiers in Java |
Whether you’re just starting out with Java or you’re looking to level up your programming skills, we hope this guide has given you a deeper understanding of Java keywords and their importance.
With a solid grasp of Java keywords, you’re now better equipped to write efficient, error-free Java code. Keep exploring, keep learning, and happy coding!