Java Extends Keyword: How to Make Child Classes
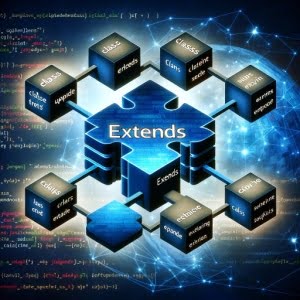
Are you finding it challenging to understand inheritance in Java? You’re not alone. Many developers find themselves puzzled when it comes to mastering the ‘extends’ keyword in Java, but we’re here to help.
Think of Java’s inheritance as a family tree – allowing one class to inherit fields and methods from another class. This concept is a cornerstone of object-oriented programming, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of using ‘extends’ in Java to implement inheritance, from its basic usage to more advanced techniques, as well as alternative approaches. We’ll cover everything from creating a subclass, multi-level inheritance, method overriding, to dealing with common issues and their solutions.
Let’s get started and master the ‘extends’ keyword in Java!
TL;DR: How Do I Use ‘extends’ in Java?
In Java, ‘extends’ is used to create a subclass that inherits attributes and methods from another class, known as the superclass, called with the syntax:
class SubClass extends SuperClass
. This is a fundamental part of Java’s inheritance mechanism, which is a key aspect of object-oriented programming.
Here’s a simple example:
class SuperClass {
// some fields and methods
}
class SubClass extends SuperClass {
// SubClass inherits all accessible fields and methods from SuperClass
}
In this example, we have a SuperClass
and a SubClass
. The SubClass
uses the ‘extends’ keyword to inherit all accessible fields and methods from the SuperClass
. This is the basic usage of ‘extends’ in Java.
But there’s more to the ‘extends’ keyword than just this. Continue reading for a deeper understanding and more advanced usage scenarios.
Table of Contents
- Using ‘Extends’ to Create a Subclass in Java
- Advanced Inheritance: Multi-level and Method Overriding
- Exploring Alternatives: Interfaces and Abstract Classes
- Navigating Inheritance Pitfalls: Method Conflicts and Access Modifiers
- Unpacking Inheritance: A Pillar of Object-Oriented Programming
- Inheritance in Real-World Applications
- Wrapping Up: Mastering ‘Extends’ in Java for Inheritance
Using ‘Extends’ to Create a Subclass in Java
In Java, the ‘extends’ keyword is used to denote inheritance from a superclass. When a class extends another, it inherits all the accessible methods and fields of the superclass. This is one of the fundamental aspects of Java’s object-oriented programming.
Let’s look at a simple example:
// Define the superclass
class Animal {
void eat() {
System.out.println("Animal can eat");
}
}
// Define the subclass
class Dog extends Animal {
void bark() {
System.out.println("Dog can bark");
}
}
// Test the subclass
public class TestDog {
public static void main(String args[]) {
Dog d = new Dog();
d.eat();
d.bark();
}
}
# Output:
# 'Animal can eat'
# 'Dog can bark'
In the code above, we define a superclass Animal
with a method eat()
. We then create a subclass Dog
using the ‘extends’ keyword. This Dog
class inherits the eat()
method from Animal
, and also defines its own method bark()
. In the TestDog
class, we create a Dog
object and call both eat()
and bark()
methods.
This illustrates the basic use of ‘extends’ in Java to create a subclass. By using ‘extends’, we can reuse the code from the superclass, which is one of the main advantages of inheritance. However, it’s worth noting that inheritance can also lead to complexity and potential pitfalls, such as method conflicts and issues with access modifiers, which we will discuss in the following sections.
Advanced Inheritance: Multi-level and Method Overriding
As you become more comfortable with the ‘extends’ keyword and inheritance in Java, you can start to explore more advanced uses, such as multi-level inheritance and method overriding.
Multi-level Inheritance
In Java, a class can extend another class, which in turn extends another class, forming a chain of inheritance. This is known as multi-level inheritance.
Here’s an example:
// Define the superclass
class Animal {
void eat() {
System.out.println("Animal can eat");
}
}
// Define the intermediate class
class Dog extends Animal {
void bark() {
System.out.println("Dog can bark");
}
}
// Define the subclass
class Puppy extends Dog {
void weep() {
System.out.println("Puppy can weep");
}
}
// Test the subclass
public class TestPuppy {
public static void main(String args[]) {
Puppy p = new Puppy();
p.eat();
p.bark();
p.weep();
}
}
# Output:
# 'Animal can eat'
# 'Dog can bark'
# 'Puppy can weep'
In this example, Puppy
extends Dog
, which in turn extends Animal
, forming a chain of inheritance. The Puppy
class can access methods from both Dog
and Animal
.
Method Overriding
Another advanced use of ‘extends’ is method overriding. In Java, when a subclass has a method with the same name as a method in its superclass, the subclass’s method overrides the one in the superclass.
Let’s see an example:
// Define the superclass
class Animal {
void eat() {
System.out.println("Animal can eat");
}
}
// Define the subclass
class Dog extends Animal {
// Override the eat() method
void eat() {
System.out.println("Dog can eat dog food");
}
}
// Test the subclass
public class TestDog {
public static void main(String args[]) {
Dog d = new Dog();
d.eat();
}
}
# Output:
# 'Dog can eat dog food'
In the code above, Dog
overrides the eat()
method of Animal
. When we call eat()
on a Dog
object, the overridden method in Dog
is executed instead of the one in Animal
.
These are just a few examples of the advanced uses of ‘extends’ in Java. As you continue to explore, you’ll find that inheritance, along with other features of object-oriented programming, provide powerful tools for structuring and organizing your code.
Exploring Alternatives: Interfaces and Abstract Classes
While the ‘extends’ keyword and inheritance are powerful tools in Java, they are not the only way to achieve code reuse and polymorphism. Two other key concepts in Java’s object-oriented toolbox are interfaces and abstract classes.
Interfaces in Java
An interface is a completely abstract class that contains only abstract methods. It can be implemented by any class, using the ‘implements’ keyword. Unlike ‘extends’, a class can implement multiple interfaces.
Here’s a simple example:
// Define the interface
interface Eater {
void eat();
}
// Implement the interface
class Dog implements Eater {
public void eat() {
System.out.println("Dog is eating");
}
}
// Test the implementation
public class TestDog {
public static void main(String args[]) {
Dog d = new Dog();
d.eat();
}
}
# Output:
# 'Dog is eating'
In this code, we define an Eater
interface with a single method eat()
. The Dog
class then implements this interface, providing its own implementation of eat()
. This demonstrates one of the advantages of interfaces – they provide a way to ensure that a class adheres to a certain contract, without imposing any inheritance hierarchy.
Abstract Classes in Java
An abstract class in Java is a class that cannot be instantiated and is meant to be subclassed. It can contain both abstract and non-abstract methods.
Here’s an example:
// Define the abstract class
abstract class Animal {
abstract void eat();
void sleep() {
System.out.println("Animal is sleeping");
}
}
// Extend the abstract class
class Dog extends Animal {
void eat() {
System.out.println("Dog is eating");
}
}
// Test the subclass
public class TestDog {
public static void main(String args[]) {
Dog d = new Dog();
d.eat();
d.sleep();
}
}
# Output:
# 'Dog is eating'
# 'Animal is sleeping'
In this example, Animal
is an abstract class with an abstract method eat()
and a non-abstract method sleep()
. Dog
extends Animal
and provides its own implementation of eat()
. This demonstrates the power of abstract classes – they can provide some default behavior (like sleep()
) while forcing subclasses to provide others (like eat()
).
Choosing the Right Tool
When designing your Java classes, it’s important to choose the right tool for the job. If you need to create a hierarchy of related classes that share common behavior, ‘extends’ and inheritance might be the way to go. If you need to ensure that a class adheres to a certain contract, consider using an interface. If you want to provide some default behavior but also force subclasses to provide specific behavior, an abstract class could be the answer.
Remember, each of these tools has its own strengths and weaknesses, and they can often be used together in powerful ways. Understanding the ‘extends’ keyword is just the beginning of your journey into Java’s object-oriented programming features.
As with any powerful tool, Java’s ‘extends’ keyword and inheritance mechanism come with their own set of challenges. Two common issues that developers often encounter are method conflicts and access modifier restrictions. Let’s explore these in detail.
Resolving Method Conflicts
One common issue that arises with inheritance is method conflicts, especially when dealing with multi-level inheritance or when a subclass overrides a superclass’s method. Let’s consider an example.
class Animal {
void eat() {
System.out.println("Animal is eating");
}
}
class Dog extends Animal {
void eat() {
System.out.println("Dog is eating dog food");
}
}
class Puppy extends Dog {
void eat() {
System.out.println("Puppy is eating puppy food");
}
}
public class TestPuppy {
public static void main(String args[]) {
Puppy p = new Puppy();
p.eat();
}
}
# Output:
# 'Puppy is eating puppy food'
In the above code, the Puppy
class overrides the eat()
method of the Dog
class, which in turn overrides the eat()
method of the Animal
class. When we call eat()
on a Puppy
object, the Puppy
‘s eat()
method is executed, not the Dog
‘s or Animal
‘s. This is a simple example of how method conflicts can arise and how Java resolves them.
Understanding Access Modifiers
Another common issue with inheritance in Java is dealing with access modifiers. In Java, there are four access modifiers: private, default (no modifier), protected, and public. These determine the visibility of fields and methods in the superclass and subclass.
Here’s a quick overview:
Modifier | Class | Package | Subclass | World |
---|---|---|---|---|
public | Y | Y | Y | Y |
protected | Y | Y | Y | N |
no modifier (default) | Y | Y | N | N |
private | Y | N | N | N |
In the context of inheritance, the ‘extends’ keyword can only inherit public and protected members of a superclass. Private members are not inherited because they are only visible within the class they are defined.
By understanding these common issues and how to navigate them, you can use the ‘extends’ keyword and Java’s inheritance mechanism more effectively and avoid potential pitfalls.
Unpacking Inheritance: A Pillar of Object-Oriented Programming
To fully understand the ‘extends’ keyword and its role in Java, it’s crucial to grasp the concept of inheritance and its place in object-oriented programming (OOP).
Inheritance in Java
Inheritance is one of the four primary principles of OOP, alongside encapsulation, abstraction, and polymorphism. It’s a mechanism that allows one class to acquire the properties (methods and fields) of another. With the use of the ‘extends’ keyword, a class (subclass) can inherit features from another class (superclass).
class SuperClass {
// fields and methods
}
class SubClass extends SuperClass {
// SubClass inherits all accessible fields and methods from SuperClass
}
In this example, SubClass
inherits all accessible fields and methods from SuperClass
using the ‘extends’ keyword.
Object-Oriented Programming (OOP) in Java
OOP is a programming paradigm centered around objects. These objects are instances of classes, which can contain data in the form of fields (also known as attributes) and code in the form of procedures (also known as methods).
Java, as an object-oriented language, uses classes and objects as its fundamental building blocks. These classes interact with each other to design applications and programs. Inheritance, enabled by the ‘extends’ keyword, is a key part of this interaction, allowing classes to reuse, extend, and modify the behavior defined in other classes.
By understanding the concept of inheritance and its role in OOP, you can better understand and utilize the ‘extends’ keyword in Java.
Inheritance in Real-World Applications
Inheritance, facilitated by the ‘extends’ keyword in Java, isn’t just a theoretical concept. It’s a practical tool that developers use every day in real-world applications. Whether you’re building a simple web app or a complex enterprise system, understanding inheritance can help you design your classes and objects more effectively.
Exploring Related Concepts: Polymorphism and Encapsulation
While inheritance is a powerful tool, it’s just one part of the object-oriented programming toolbox. Two other key concepts you might want to explore are polymorphism and encapsulation.
Polymorphism allows objects of different classes to be treated as objects of a common superclass. This can make your code more flexible and extensible. Encapsulation, on the other hand, is about hiding the internal details of how an object works and exposing only what’s necessary, which can make your code more maintainable and less prone to errors.
Real-World Application of Inheritance
In real-world applications, inheritance is often used to create a set of related classes that share common behavior but also have their own specific behaviors. For example, in a graphics program, you might have a Shape
superclass with subclasses like Circle
, Rectangle
, and Triangle
. Each of these subclasses would inherit common properties like color
and position
from Shape
, but also have their own specific properties like radius
for Circle
and width
and height
for Rectangle
.
Further Resources for Mastering Java Inheritance
Ready to dive deeper into Java inheritance and related concepts? Here are a few resources to help you on your journey:
- IOFlood’s Java Keywords Article – Learn about access modifiers like “private,” “protected,” and “default” in Java.
super
Keyword in Java – Learn about the ‘super’ keyword in Java for accessing members of the superclass.Java Records Overview – Discover Java record, a feature introduced in Java 14 for concise and immutable data modeling.
Oracle’s Java Tutorials: Inheritance – The official Java tutorials from Oracle.
GeeksforGeeks’ Java Inheritance is a comprehensive guide on Java inheritance.
Baeldung’s A Guide to Java Inheritance covers Java inheritance and related concepts like abstraction and polymorphism.
Remember, mastering a concept like inheritance takes time and practice. Don’t rush it. Experiment with code, make mistakes, learn from them, and keep refining your understanding. Happy coding!
Wrapping Up: Mastering ‘Extends’ in Java for Inheritance
In this comprehensive guide, we’ve explored the ‘extends’ keyword in Java, a fundamental aspect of inheritance in object-oriented programming. We’ve discussed how to use ‘extends’ for creating subclasses, handling multi-level inheritance, and method overriding, along with the common issues associated with these processes.
We began with the basics, guiding you on how to use ‘extends’ to create a subclass in Java, including a code example with an explanation of how inheritance works. We then ventured into more complex uses of ‘extends’, such as multi-level inheritance and method overriding, providing intermediate-level code examples and analysis.
To help you navigate the common issues with inheritance, we discussed method conflicts and access modifiers, providing solutions and workarounds for each issue. We also introduced you to alternative approaches to handle inheritance, like interfaces and abstract classes, giving you a broader perspective on the topic.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
‘Extends’ Keyword | Enables code reuse and polymorphism | Can lead to complexity and potential pitfalls |
Interfaces | Ensures a class adheres to a certain contract | No default behavior |
Abstract Classes | Can provide default behavior and force subclasses to provide specific behavior | Requires careful design |
Whether you’re a beginner just starting out with Java or an intermediate developer looking to deepen your understanding of inheritance, we hope this guide has provided you with a comprehensive understanding of the ‘extends’ keyword and its usage in Java.
The ‘extends’ keyword is a powerful tool in your Java toolbox, enabling you to leverage the power of inheritance in your programs. With this guide, you’re now well equipped to use ‘extends’ effectively in your Java programming journey. Happy coding!