Java ‘!=’ Operator: Use Cases for ‘Not Equals’ Comparisons
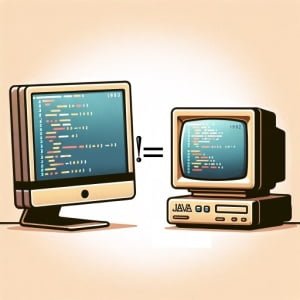
Are you finding it challenging to understand the ‘!=’ operator in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling this operator, but we’re here to help.
Think of the ‘!=’ operator in Java as a detective looking for differences – it helps identify when two values are not the same, providing a versatile and handy tool for various tasks.
This guide will help you understand and use the ‘!=’ operator in Java effectively. We’ll cover everything from the basics of this operator to more advanced techniques, as well as alternative approaches.
Let’s dive in and start mastering the ‘!=’ operator in Java!
TL;DR: What Does ‘!=’ Mean in Java?
In Java, the
'!='
operator is used to check if two values arenot equal
, for exampleif (x != y) {System.out.println("x and y are not equal");
. It’s a comparison operator that returns true if the values on either side of it are unequal.
Here’s a simple example:
int x = 5;
int y = 10;
if (x != y) {
System.out.println("x and y are not equal");
}
# Output:
# 'x and y are not equal'
In this example, we have two variables, x
and y
, with values 5 and 10 respectively. The ‘!=’ operator checks if x
and y
are not equal. Since 5 is not equal to 10, the condition x != y
returns true, and the message ‘x and y are not equal’ is printed.
This is a basic use of the ‘!=’ operator in Java, but there’s much more to learn about it. Continue reading for more detailed information and examples.
Table of Contents
Basic Use of ‘!=’ Operator in Java
The ‘!=’ operator in Java is a comparison operator used to check if two values are not equal. It’s a fundamental part of conditional statements, which are crucial for controlling the flow of your programs.
The syntax of the ‘!=’ operator is straightforward:
variable1 != variable2
This statement will return true if variable1
and variable2
are not equal, and false if they are equal. Let’s see a simple example:
int a = 10;
int b = 20;
if (a != b) {
System.out.println("a and b are not equal");
}
# Output:
# 'a and b are not equal'
In this code, we have two variables, a
and b
, with values 10 and 20 respectively. The ‘!=’ operator checks if a
and b
are not equal. Since 10 is not equal to 20, the condition a != b
returns true, and the message ‘a and b are not equal’ is printed.
The ‘!=’ operator is essential in conditional statements like if
statements, as it allows you to perform different actions depending on the inequality of two values.
Advanced Use of ‘!=’ Operator in Java
The ‘!=’ operator is not only used for comparing primitive types like int
, char
, boolean
, etc., but it also plays a significant role when dealing with objects and null values.
Comparing Objects with ‘!=’
When comparing objects in Java, the ‘!=’ operator checks if two object references point to different objects, not their content. Let’s illustrate this with an example:
String str1 = new String("Hello");
String str2 = new String("Hello");
if (str1 != str2) {
System.out.println("str1 and str2 point to different objects");
} else {
System.out.println("str1 and str2 point to the same object");
}
# Output:
# 'str1 and str2 point to different objects'
In this example, even though str1
and str2
contain the same content, they are different objects. Hence, str1 != str2
returns true, and the message ‘str1 and str2 point to different objects’ is printed.
Comparing Null Values with ‘!=’
The ‘!=’ operator is also used to check if an object reference is not null before accessing its methods or fields. This can prevent NullPointerException
in your code. Here’s an example:
String str = null;
if (str != null) {
System.out.println(str.length());
} else {
System.out.println("str is null");
}
# Output:
# 'str is null'
In this case, str
is null, so str != null
returns false, and the message ‘str is null’ is printed. If we tried to access str.length()
without checking if str
is not null, it would throw a NullPointerException
.
Alternative Approaches: Comparing Objects with ‘equals()’
In Java, there’s another way to compare objects for equality: the ‘equals()’ method. Unlike the ‘!=’ operator, which compares references, ‘equals()’ can be overridden in an object’s class to compare the actual content of the objects.
‘equals()’ Method: An Example
Let’s consider the same strings we used in the previous example, but this time, we’ll use the ‘equals()’ method to compare them:
String str1 = new String("Hello");
String str2 = new String("Hello");
if (!str1.equals(str2)) {
System.out.println("str1 and str2 do not contain the same content");
} else {
System.out.println("str1 and str2 contain the same content");
}
# Output:
# 'str1 and str2 contain the same content'
In this example, str1.equals(str2)
returns true because the ‘equals()’ method, as implemented in the String class, compares the actual content of the strings, not their references. So, even though str1
and str2
are different objects, they contain the same content, ‘Hello’.
‘!=’ Operator vs. ‘equals()’: What’s the Difference?
The key difference between the ‘!=’ operator and the ‘equals()’ method is how they compare objects. The ‘!=’ operator checks if two references point to different objects, regardless of their content, while ‘equals()’ can be used to compare the actual content of the objects.
Keep in mind that the behavior of ‘equals()’ can vary depending on the class. If ‘equals()’ is not overridden in a class, it behaves the same as the ‘!=’ operator. That’s why it’s essential to understand the class of the objects you’re comparing.
Troubleshooting ‘!=’ Operator in Java
While using the ‘!=’ operator in Java, developers often encounter some common mistakes and misconceptions. Let’s discuss these issues and provide solutions and best practices.
Mistaking ‘!=’ for '=='
One of the most common errors is confusing the ‘!=’ operator with the '=='
operator. While '=='
checks for equality, ‘!=’ checks for inequality. Here’s an example illustrating this mistake:
int a = 5;
int b = 5;
if (a != b) {
System.out.println("a and b are not equal");
} else {
System.out.println("a and b are equal");
}
# Output:
# 'a and b are equal'
In this code, a
and b
are equal. However, the ‘!=’ operator checks for inequality, so the condition a != b
returns false, and the message ‘a and b are equal’ is printed. If you meant to check for equality, you should use '=='
.
Misunderstanding Object Comparison
Another common mistake is misunderstanding how the ‘!=’ operator compares objects. Remember, ‘!=’ checks if two references point to different objects, not their content. To compare the actual content of objects, you should use the ‘equals()’ method.
Best Practices
- Always use
'=='
to check for equality and ‘!=’ to check for inequality. - When comparing objects, use ‘!=’ to check if they are different objects, and ‘equals()’ to compare their content.
- Always check if an object is not null before accessing its methods or fields using ‘!=’.
By keeping these considerations in mind, you can avoid common pitfalls and use the ‘!=’ operator in Java effectively.
Unveiling Java Operators
Java operators are special symbols that perform specific operations on one, two, or three operands and then return a result. They are a fundamental part of Java, as they manipulate variables and values to perform common mathematical, relational, and logical computations.
Comparison Operators in Java
Among these operators, a significant category is the comparison (or relational) operators. They compare two values and return a boolean result. Here’s a brief overview of comparison operators in Java:
'=='
(equal to): Checks if the values of two operands are equal or not. If yes, it returns true. Otherwise, it returns false.- ‘!=’ (not equal to): Checks if the values of two operands are equal or not. If the values are not equal, it returns true. Otherwise, it returns false.
- ‘>’ (greater than), ‘=’ (greater than or equal to), and ‘>=’ | Checks if the value of the left operand is greater than the value of the right operand |
- | ‘=’ | Checks if the value of the left operand is greater than or equal to the value of the right operand |
- | ‘<=’ | Checks if the value of the left operand is less than or equal to the value of the right operand |
Further Resources for Java Operators
To deepen your understanding of JSP and related technologies, here are some resources that provide more in-depth information:
- Java Operator: A Quick Overview – Learn about Java’s bitwise shift operators for shifting bits left or right.
Logical Not Operator in Java – Explore the logical “!” operator in Java, used for negating a boolean value or expression.
Java Ternary Operator Basics – Discover the ternary operator in Java, a way of writing conditional expressions with three operands.
Java Operators by W3Schools provides a comprehensive tutorial on all types of operators in Java.
Java Operators Tutorial by Oracle offers a detailed tutorial on Java operators.
Operators in Java by GeeksforGeeksprovides a comprehensive guide on operators in Java.
Wrapping Up: Java ‘!=’ Operator
Whether you’re just starting out with Java or you’re looking to deepen your understanding of its operators, we hope this guide has given you a clearer understanding of the ‘!=’ operator and its role in Java programming.
Understanding the ‘!=’ operator is key to mastering Java, as it allows you to control the flow of your programs based on the relationships between different values. Now, you’re well-equipped to use the ‘!=’ operator effectively in your Java programs. Happy coding!