Python Absolute Value | abs() Function and Alternatives
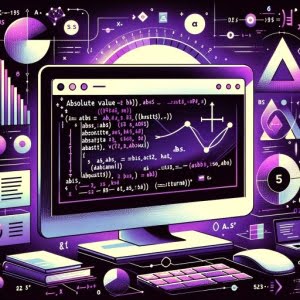
Ever felt lost trying to find the absolute value of a number in Python? Just like a compass guiding you through the wilderness, Python’s abs()
function can help you find the absolute value. It’s a simple, yet powerful tool that can make your coding journey much smoother.
This comprehensive guide will walk you through the steps of using the abs()
function in Python, helping you understand its basic and advanced uses, potential issues you might encounter, and alternative methods.
So, buckle up and get ready to delve into the world of Python absolute values!
TL;DR: How Do I Find the Absolute Value in Python?
To find the absolute value in Python, you use the
abs()
function. Here’s a simple example:
number = -10
absolute_value = abs(number)
print(absolute_value)
# Output:
# 10
In this example, we’ve used the abs()
function to find the absolute value of -10
, which is 10
. The abs()
function is a powerful tool in Python that can handle both positive and negative numbers with ease. If you’re intrigued and want to learn more about the abs()
function, including advanced usage scenarios and potential pitfalls, keep reading. This comprehensive guide has got you covered!
Table of Contents
- Python’s abs() Function: A Beginner’s Guide
- Handling Complex Numbers with abs()
- Exploring Alternatives: The math.fabs() Function
- Troubleshooting Common Issues with Absolute Values in Python
- Python Number Data Types: Integer, Float, and Complex
- Expanding Horizons: Absolute Values in Real-World Applications
- Further Resources for Python Functions
- Recap: Python’s Absolute Value
Python’s abs() Function: A Beginner’s Guide
Python’s abs()
function is a built-in function used to return the absolute value of a number. The absolute value of a number is its distance from zero, regardless of the direction. In other words, it’s the non-negative value of a number. Let’s see it in action:
number = -15
absolute_value = abs(number)
print(absolute_value)
# Output:
# 15
In this example, we use the abs()
function to find the absolute value of -15
, which returns 15
. Notice how regardless of the negative sign, abs()
returns the positive counterpart. This is the basic use of the abs()
function.
The abs()
function is a handy tool when you’re dealing with operations where you need the magnitude of a number, but the direction (positive or negative) is not important. It’s simple to use and works seamlessly with both positive and negative numbers.
However, it’s important to remember that the abs()
function only works with numbers. If you try to use it with a data type that is not a number, Python will raise a TypeError. For example:
string = 'I am not a number'
try:
absolute_value = abs(string)
except TypeError:
print('TypeError: bad operand type for abs(): str')
# Output:
# TypeError: bad operand type for abs(): str
In this example, we tried to find the absolute value of a string, which resulted in a TypeError. This is one of the potential pitfalls you need to be aware of when using the abs()
function.
Handling Complex Numbers with abs()
Python’s abs()
function isn’t limited to just integers and floating-point numbers; it can also handle complex numbers. A complex number is a number that can be expressed in the form a + bj
, where a
and b
are real numbers and j
represents the square root of -1
. In the context of complex numbers, the abs()
function returns the magnitude of the complex number.
Let’s see an example:
complex_number = 3 + 4j
absolute_value = abs(complex_number)
print(absolute_value)
# Output:
# 5.0
In this example, 3 + 4j
is a complex number. When we pass this complex number to the abs()
function, it returns the magnitude of the complex number, which is 5.0
. The magnitude is calculated as the square root of the sum of the squares of the real and imaginary parts (sqrt(a^2 + b^2)
).
This advanced use of the abs()
function opens up a whole new range of possibilities. However, it’s important to understand that the abs()
function treats real and complex numbers differently. For real numbers, it returns the number without the sign, while for complex numbers, it returns the magnitude. As you delve deeper into Python, you’ll find this function to be a powerful tool in your arsenal, especially when dealing with mathematical computations involving complex numbers.
Exploring Alternatives: The math.fabs() Function
While the abs()
function is the most common method for finding the absolute value in Python, it’s not the only one. Python also provides the math.fabs()
function as part of its math module. The math.fabs()
function returns the absolute value of a number as a floating-point number.
Here’s an example of how to use the math.fabs()
function:
import math
number = -7.5
absolute_value = math.fabs(number)
print(absolute_value)
# Output:
# 7.5
In this example, we’ve used the math.fabs()
function to find the absolute value of -7.5
, which returns 7.5
. Notice that even though the input was a float, the math.fabs()
function still successfully returned the absolute value.
However, it’s important to note that the math.fabs()
function only works with real numbers. If you try to use it with a complex number, Python will raise a TypeError.
Method | Works with Integers | Works with Floats | Works with Complex Numbers |
---|---|---|---|
abs() | Yes | Yes | Yes |
math.fabs() | Yes | Yes | No |
As you can see, both abs()
and math.fabs()
functions have their advantages and disadvantages. While abs()
is more versatile and can handle complex numbers, math.fabs()
returns a float for all inputs. Your choice between the two will depend on the specific requirements of your code.
Troubleshooting Common Issues with Absolute Values in Python
When finding absolute values in Python, you might encounter a few common issues. One of the most common errors is the TypeError
. This error occurs when you try to find the absolute value of a non-numeric data type, such as a string or a list. Let’s look at an example:
string = 'Hello, Python!'
try:
absolute_value = abs(string)
except TypeError:
print('TypeError: bad operand type for abs(): str')
# Output:
# TypeError: bad operand type for abs(): str
In this code block, we tried to find the absolute value of a string, which resulted in a TypeError
. Python’s abs()
function only works with numeric data types, so trying to use it with a string or other non-numeric data types will result in an error.
Another common issue is using the math.fabs()
function with complex numbers. While the math.fabs()
function works perfectly with integers and floats, it doesn’t support complex numbers. Here’s an example:
import math
complex_number = 5 + 2j
try:
absolute_value = math.fabs(complex_number)
except TypeError:
print('TypeError: can't convert complex to float')
# Output:
# TypeError: can't convert complex to float
In this example, we tried to find the absolute value of a complex number using math.fabs()
, which led to a TypeError
. This is because math.fabs()
can only handle real numbers and not complex numbers.
When encountering these common issues, the solutions are usually straightforward. For TypeError
with abs()
, make sure the input is a numeric data type. For issues with math.fabs()
, ensure the input is a real number. Understanding these potential problems and their solutions will make your Python coding journey smoother and more enjoyable.
Python Number Data Types: Integer, Float, and Complex
To fully grasp the concept of finding the absolute value in Python, it’s essential to understand Python’s number data types: integer, float, and complex numbers.
Integer Numbers
Integers in Python are whole numbers that can be positive, negative, or zero. For example, 5
, -3
, and 0
are all integers. When you use the abs()
function with an integer, it simply returns the positive counterpart of the number. Here’s an example:
integer_number = -8
absolute_value = abs(integer_number)
print(absolute_value)
# Output:
# 8
In this example, we’ve used the abs()
function to find the absolute value of -8
, which is 8
.
Float Numbers
Floats in Python are real numbers that contain a decimal point. For example, 3.14
, -0.01
, and 0.0
are all floats. The abs()
function works with floats just like it works with integers, returning the positive counterpart of the number. Here’s an example:
float_number = -7.5
absolute_value = abs(float_number)
print(absolute_value)
# Output:
# 7.5
In this example, we’ve used the abs()
function to find the absolute value of -7.5
, which is 7.5
.
Complex Numbers
Complex numbers in Python are numbers that have a real and an imaginary part, expressed as a + bj
. For example, 3 + 4j
is a complex number. When you use the abs()
function with a complex number, it returns the magnitude of the number. Here’s an example:
complex_number = 3 + 4j
absolute_value = abs(complex_number)
print(absolute_value)
# Output:
# 5.0
In this example, we’ve used the abs()
function to find the magnitude of the complex number 3 + 4j
, which is 5.0
.
Understanding these number data types and how the abs()
function works with each of them is fundamental to mastering the concept of finding the absolute value in Python. Whether you’re working with integers, floats, or complex numbers, Python’s abs()
function has got you covered!
Expanding Horizons: Absolute Values in Real-World Applications
Finding absolute values is not just a theoretical concept, but a practical tool used in various real-world applications, especially in mathematical computations and data analysis. For instance, in data analysis, absolute values can help calculate the magnitude of change or difference between two data points, disregarding the direction of the change. Here’s a simple example:
change = -15
magnitude_of_change = abs(change)
print(magnitude_of_change)
# Output:
# 15
In this example, we have a change of -15
. Using the abs()
function, we can find the magnitude of this change, which is 15
, regardless of whether it was a decrease or increase.
Beyond just the abs()
function, Python offers a rich set of mathematical functions in its math module, which can be a great next step to explore. For example, the math.sqrt()
function can be used to calculate the square root of a number, and the math.pow()
function can be used to raise a number to a power.
Additionally, Python’s capabilities for data analysis extend far beyond just mathematical functions. Libraries such as Pandas, NumPy, and Matplotlib offer powerful tools for data manipulation, analysis, and visualization. These libraries, combined with Python’s built-in functions like abs()
, can be a powerful toolkit for any data analyst or scientist.
Further Resources for Python Functions
To deepen your understanding of Python and its applications, consider exploring these resources:
- Python Built-In Functions Logic: Simplified – Discover Python’s functions for working with binary data and byte manipulation.
Data Sorting with Python Sort Algorithms – Learn how to choose the right sorting algorithm for your data and performance needs.
Python max() Function: Finding the Maximum Value – Explore Python’s “max” function for finding the largest element in a sequence.
Plotting a Function with Matplotlib in Python – TutorialsPoint unveils the process of plotting a function in Python.
Essential Numpy Math Functions – Programiz provides a breakdown of math functions in the Numpy library for Python.
30 Useful Pandas Functions for Data Analysis – This guide by Regenerative Today details 30 useful pandas functions.
Recap: Python’s Absolute Value
In this comprehensive guide, we’ve explored how to find the absolute value in Python using the abs()
function. This built-in function is a versatile tool that works with integers, floats, and even complex numbers. Here’s a quick recap of what we covered:
- Basic Use of abs(): The
abs()
function returns the positive counterpart of a number, effectively giving us its absolute value. It’s a simple and straightforward method, as seen in this example:
number = -10
absolute_value = abs(number)
print(absolute_value)
# Output:
# 10
- Advanced Use of abs(): We’ve seen that the
abs()
function can also handle complex numbers, returning the magnitude of the complex number. Alternative Approach: Python also provides the
math.fabs()
function, which can be used to find the absolute value of a number as a floating-point number.Troubleshooting: We discussed common issues that might arise when using these functions, such as
TypeError
, and provided solutions for them.Beyond the Basics: We also touched on the real-world applications of finding absolute values in Python, particularly in mathematical computations and data analysis.
Method | Works with Integers | Works with Floats | Works with Complex Numbers |
---|---|---|---|
abs() | Yes | Yes | Yes |
math.fabs() | Yes | Yes | No |
Whether you’re a beginner just starting out or an intermediate programmer looking to brush up on your skills, understanding how to find the absolute value in Python is an essential skill. It’s our hope that this guide has helped shed light on this concept and provided you with the tools you need to navigate Python’s absolute value functions with ease.