Python Sort Algorithms: A Comprehensive Guide
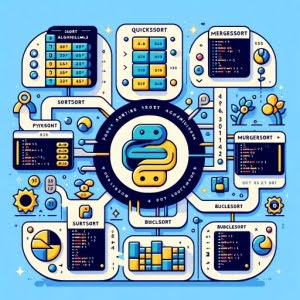
Sorting data is a fundamental task in programming. Whether you’re a data scientist needing to organize large datasets or a developer wanting to order user inputs, sorting algorithms are essential.
Python, like a master librarian, offers several ways to sort your data, each with its own advantages and use cases.
In this guide, we’ll walk you through the different sorting algorithms available in Python, from the simplest to the most complex. We’ll start with Python’s built-in sort()
and sorted()
functions, then delve into more advanced algorithms like quicksort, mergesort, and heapsort. Along the way, we’ll discuss time complexities, use cases, and even troubleshoot common issues.
Let’s start our journey into the world of Python sorting algorithms!
TL;DR: How Do I Sort Lists in Python?
Python provides several built-in sorting algorithms, like the
sort()
function for lists, and thesorted()
function for any iterable. These functions make it easy to sort data in Python.
Here’s a simple example of using sort()
:
numbers = [5, 1, 9, 3]
numbers.sort()
print(numbers)
# Output:
# [1, 3, 5, 9]
In this example, we have a list of numbers. We use the sort()
function to sort the numbers in ascending order. The sort()
function modifies the list in-place, meaning the original list is sorted, and no new list is created.
This is just the tip of the iceberg when it comes to Python’s sorting capabilities. Continue reading for a deeper understanding of Python’s sorting algorithms, including their time complexities and use cases.
Table of Contents
- Python’s Built-in Sorting: sort() and sorted()
- Delving into Advanced Sorting: Quicksort, Mergesort, and Heapsort
- Crafting Custom Sorting Algorithms in Python
- Troubleshooting Python Sort Algorithms
- Understanding the Theory Behind Sorting Algorithms
- Python Sort Algorithms in Real-World Applications
- Wrapping Up: Mastering Python Sort Algorithms
Python’s Built-in Sorting: sort()
and sorted()
Python provides two built-in functions for sorting: sort()
and sorted()
. These functions are the most straightforward way to sort data in Python, making them perfect for beginners.
The sort()
Function
The sort()
function is a method that you can call on lists in Python. It modifies the list it is called on, meaning it sorts the list in-place and does not create a new list. Here’s an example:
numbers = [5, 1, 9, 3]
numbers.sort()
print(numbers)
# Output:
# [1, 3, 5, 9]
In this example, we call sort()
on our list of numbers, and it sorts the numbers in ascending order. The original list numbers
is modified to be sorted.
The sorted()
Function
The sorted()
function, on the other hand, works on any iterable, not just lists. It creates a new sorted list from the iterable it is called on. Here’s an example:
numbers = (5, 1, 9, 3) # a tuple
sorted_numbers = sorted(numbers)
print(sorted_numbers)
# Output:
# [1, 3, 5, 9]
In this example, we call sorted()
on a tuple of numbers. It returns a new list that is sorted in ascending order. The original tuple numbers
remains unchanged.
Time Complexity of sort()
and sorted()
Both sort()
and sorted()
use a sorting algorithm called Timsort, which is a hybrid sorting algorithm derived from merge sort and insertion sort. The time complexity of Timsort is O(n log n) for the worst case and average case, and O(n) for the best case (when the input is already sorted). This makes sort()
and sorted()
efficient for large datasets.
Delving into Advanced Sorting: Quicksort, Mergesort, and Heapsort
While Python’s built-in sort()
and sorted()
functions are powerful, understanding the mechanics of more complex sorting algorithms, such as quicksort, mergesort, and heapsort, can offer greater control and efficiency in certain scenarios.
Quicksort in Python
Quicksort is a divide-and-conquer algorithm. It works by selecting a ‘pivot’ element from the array and partitioning the other elements into two sub-arrays, according to whether they are less than or greater than the pivot. The sub-arrays are then recursively sorted. Here’s a simple implementation of quicksort in Python:
def quicksort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
print(quicksort([3,6,8,10,1,2,1]))
# Output:
# [1, 1, 2, 3, 6, 8, 10]
In this example, we first check if the list is empty or contains a single element. If so, it is already sorted. If not, we select a pivot and partition the list into elements less than, equal to, and greater than the pivot. We then recursively sort the ‘less than’ and ‘greater than’ sub-lists.
Quicksort’s time complexity is O(n log n) in the best and average cases, and O(n^2) in the worst case when the list is already sorted. Despite its worst-case scenario, quicksort is often faster in practice than other O(n log n) algorithms, such as mergesort and heapsort.
Mergesort in Python
Mergesort is another divide-and-conquer algorithm. It works by dividing the unsorted list into n sublists, each containing one element (a list of one element is considered sorted), and repeatedly merging sublists to produce new sorted sublists until there is only one sublist remaining. Here’s an example:
def mergesort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = arr[:mid]
right = arr[mid:]
return merge(mergesort(left), mergesort(right))
def merge(left, right):
result = []
i = j = 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
print(mergesort([3,6,8,10,1,2,1]))
# Output:
# [1, 1, 2, 3, 6, 8, 10]
In this example, we first check if the list is empty or contains a single element. If so, it is already sorted. If not, we divide the list into two halves. We then recursively sort each half and merge the sorted halves.
Mergesort’s time complexity is O(n log n) in all cases, making it efficient for large datasets. However, it requires O(n) auxiliary space, meaning it uses more memory than in-place algorithms like quicksort.
Heapsort in Python
Heapsort is a comparison-based sorting algorithm. It works by visualizing the elements of the array as a special kind of complete binary tree called a heap. Here’s an example:
import heapq
def heapsort(arr):
h = []
for value in arr:
heapq.heappush(h, value)
return [heapq.heappop(h) for i in range(len(h))]
print(heapsort([3,6,8,10,1,2,1]))
# Output:
# [1, 1, 2, 3, 6, 8, 10]
In this example, we use Python’s built-in heapq
module to push all elements onto a heap. We then pop off the smallest elements one at a time, resulting in a sorted list.
Heapsort’s time complexity is O(n log n) in all cases, and it sorts in place, meaning it doesn’t require extra space. However, it’s not a stable sort, meaning equal elements may not keep their original order.
Crafting Custom Sorting Algorithms in Python
While Python provides robust built-in sorting methods and allows for advanced sorting techniques such as quicksort, mergesort, and heapsort, there might be instances where you need to implement custom sorting algorithms tailored to specific needs or constraints. This section explores how to create such custom sorting algorithms, complete with code examples, and discusses their potential advantages and disadvantages.
Custom Sorting with the key
Parameter
Both sort()
and sorted()
functions in Python accept a key
parameter for custom sorting. The key
function transforms each element before sorting, it doesn’t affect the original content. Here’s an example of sorting a list of strings based on their length:
words = ['apple', 'banana', 'cherry', 'date']
words.sort(key=len)
print(words)
# Output:
# ['date', 'apple', 'cherry', 'banana']
In this example, len
is used as the key
function. This sorts the list based on the length of the strings, rather than their lexicographical order.
Custom Sorting with a Lambda Function
For more complex custom sorting, you can use a lambda function as the key
function. A lambda function is a small anonymous function that can take any number of arguments, but can only have one expression. Here’s an example of sorting a list of tuples based on the second element of each tuple:
pairs = [(1, 'one'), (3, 'three'), (2, 'two')]
pairs.sort(key=lambda pair: pair[1])
print(pairs)
# Output:
# [(1, 'one'), (3, 'three'), (2, 'two')]
In this example, the lambda function lambda pair: pair[1]
is used as the key
function. This sorts the list based on the second element of each tuple.
Custom sorting algorithms provide flexibility and can be tailored to specific needs. However, they can be more complex to implement and maintain than using built-in sorting functions or algorithms. As with any algorithm, it’s important to consider the trade-offs between simplicity, performance, and adaptability to specific requirements.
Troubleshooting Python Sort Algorithms
While Python’s sorting algorithms are powerful and versatile, like any tool, they can present challenges. This section discusses common issues that can arise when sorting data in Python, such as handling non-comparable data types, and provides solutions to these problems.
Handling Non-Comparable Data Types
Python’s sorting functions, sort()
and sorted()
, work by comparing elements. This works well when sorting lists of numbers or strings, but what happens when you try to sort a list of different data types?
mixed = [1, 'two', 3.0, '4']
mixed.sort()
# Output:
# TypeError: '<' not supported between instances of 'str' and 'int'
As seen in the example above, trying to sort a list of mixed data types results in a TypeError
. This is because Python doesn’t know how to compare an integer and a string.
The solution is to ensure that all elements in the list are of a comparable type. If you have control over the data, this might mean converting all elements to strings or all elements to numbers before sorting. If not, you can use a custom key
function to transform the elements for the purpose of sorting.
mixed = [1, 'two', 3.0, '4']
mixed.sort(key=str)
print(mixed)
# Output:
# [1, 3.0, '4', 'two']
In this example, we use str
as the key
function to convert all elements to strings before comparing them. This allows the sort()
function to compare the elements and sort the list.
Remember that the key
function doesn’t modify the original elements—it only transforms them for the purpose of sorting. The original list still contains the original elements, in their original types, but in sorted order.
Sorting can become complex when dealing with more complex data types, like lists of custom objects or nested lists. But with a solid understanding of Python’s sorting algorithms and the key
parameter, you can sort almost anything.
Understanding the Theory Behind Sorting Algorithms
Before diving into the specifics of Python sort algorithms, it’s crucial to understand the fundamental theory behind sorting algorithms and the concept of time complexity.
Unraveling Sorting Algorithms
A sorting algorithm is a method that organizes elements in a particular order. Most commonly, this order is numerical (ascending or descending) or lexicographical. Sorting is a key tool in many areas of computer science and programming, from data analysis to machine learning, and is one of the most studied types of algorithms.
Sorting algorithms can be classified based on their mechanism of action into various types, including exchange sorts (like bubble sort), selection sorts, insertion sorts, merge sorts, and distribution sorts (like bucket sort).
Grasping Time Complexity
Time complexity is a computational concept that describes the amount of computer time taken by an algorithm to run, as a function of the size of the input to the program. It’s usually expressed using Big O notation, which describes the upper bound of the time complexity in the worst case scenario. Here are some common time complexities:
- O(1): Constant time
- O(log n): Logarithmic time
- O(n): Linear time
- O(n log n): Log-linear time
- O(n^2): Quadratic time
For example, a simple linear search algorithm has a time complexity of O(n), meaning the time taken to execute increases linearly with the number of input elements. On the other hand, a binary search algorithm has a time complexity of O(log n), meaning it’s much more efficient for large datasets.
Understanding time complexity is crucial when dealing with large datasets, as inefficient algorithms can lead to significantly longer running times or even make the task unfeasible.
In the context of Python sort algorithms, the built-in sort()
and sorted()
functions have a time complexity of O(n log n) due to their use of Timsort, a highly efficient sorting algorithm. Other algorithms, like quicksort, mergesort, and heapsort, also have a time complexity of O(n log n) in the best or average case, but this can degrade to O(n^2) in the worst case for quicksort.
By understanding the theory behind sorting algorithms and the concept of time complexity, you can make informed decisions about which algorithm to use based on the specific requirements of your task.
Python Sort Algorithms in Real-World Applications
Sorting algorithms are not just theoretical concepts, they are fundamental tools used in a variety of real-world Python applications. Understanding and choosing the right sort algorithm can have a significant impact on the performance and efficiency of your Python projects.
Data Analysis with Python Sort Algorithms
In the field of data analysis, sorting algorithms are used to organize and process large datasets. For instance, you might need to sort a dataset of customer transactions by date, or a list of products by price. Using an efficient sort algorithm can significantly speed up these operations, especially for large datasets.
Machine Learning and Python Sort Algorithms
In machine learning, sorting algorithms can be used in various ways. For example, in the k-nearest neighbors algorithm, a common task is to sort the distances between a new data point and all existing data points to find the ‘k’ closest ones. Efficiently sorting these distances can significantly improve the performance of the algorithm.
Further Resources for Mastering Python Sort Algorithms
To further enhance your understanding and skills in Python sort algorithms, here are some additional resources:
- Python Built-In Functions: Quick Dive – Dive deep into Python’s functions for working with iterators and generators.
Python sleep() Function: Introducing Delays in Code – Discover Python’s “sleep” function for delays in code execution.
Python Absolute Value: Finding Magnitude with abs() -Python’s “abs” function for getting a number’s absolute value.
Mastering Basic Algorithms in the Python Language – This book provides an in-depth look at the core algorithms in Python.
Python Sorting Tutoroial by Real Python explains Python’s built-in sorting methods and implementing your own sorting algorithms.
The Algorithm Design Manual – While not a Python-specific book, it covers everything from sorting to searching with algorithms.
As you continue your journey in Python programming, consider exploring related topics such as searching algorithms in Python, which are often used in conjunction with sorting algorithms to efficiently find elements in a list or other data structure.
Wrapping Up: Mastering Python Sort Algorithms
In this comprehensive guide, we’ve delved deep into the world of Python sort algorithms. We’ve explored the built-in sort()
and sorted()
functions, and ventured into more advanced territory with quicksort, mergesort, and heapsort.
We began with the basics, understanding how to use Python’s built-in sorting functions. This was followed by an in-depth exploration of more complex sorting algorithms, their code implementations, and their time complexities. We also discussed how to implement custom sorting algorithms and tackled common issues that can arise when sorting data in Python.
Here’s a quick comparison of the sorting algorithms we’ve discussed:
Algorithm | Time Complexity | In-Place | Stability |
---|---|---|---|
sort() /sorted() (Timsort) | O(n log n) | No | Yes |
Quicksort | O(n log n) – O(n^2) | Yes | No |
Mergesort | O(n log n) | No | Yes |
Heapsort | O(n log n) | Yes | No |
Whether you’re a beginner just starting out with Python sort algorithms or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of Python’s sorting capabilities.
With this knowledge, you can make informed decisions about which algorithm to use based on the specific requirements of your task. Happy coding!