Python sleep() | Function Guide (With Examples)
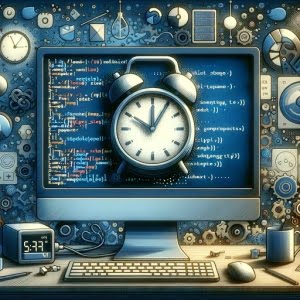
Ever wished for your Python program to take a breather? Just like a hardworking employee, there are moments when your code needs to halt its operations.
Whether it’s to simulate user interaction, delay an API call, or simply to let your system catch its breath, the ability to pause can be a game-changer in the world of programming.
This guide will introduce you to the sleep function in Python, a simple yet powerful tool that lets you do just that. So, if you’ve been wondering how to implement this pause, let’s dive into the world of Python’s sleep function.
TL;DR: How Do I Make a Python Program Sleep?
The answer is simple: Use the
sleep()
function from Python’s time module. Here’s a quick example:
import time
time.sleep(5)
print('Hello, World!')
# Output:
# 'Hello, World!'
This snippet of code will cause the program to pause for 5 seconds before printing ‘Hello, World!’. The sleep()
function essentially tells the program to halt all operations for a specified duration, in this case, 5 seconds. It’s a handy tool for creating delays in your program, giving you control over the pacing of your code’s execution.
Intrigued? Stick around for a more in-depth exploration of the
sleep()
function, its uses, and some advanced scenarios where it can prove invaluable.
Table of Contents
- Harnessing the sleep() Function: A Beginner’s Guide
- sleep() in Action: Intermediate Use Cases
- Exploring Alternatives: Beyond sleep()
- Resolving sleep() Pitfalls: Troubleshooting and Best Practices
- Understanding Time in Programming: The Role of sleep()
- Real-World Applications of sleep()
- Further Resources for Python Functions
- Wrapping Up: A Recap of Python’s sleep()
Harnessing the sleep()
Function: A Beginner’s Guide
The sleep()
function is a part of Python’s time module. It’s a simple yet powerful tool that allows you to add a delay to your program. Let’s break down its syntax and explore a basic use case.
import time # Import the time module
time.sleep(seconds) # Pause the execution of the program for 'seconds' seconds
In this syntax, seconds
is the amount of time you want the program to pause, measured in seconds. It can be an integer or a float.
Here’s a simple example:
import time
time.sleep(3)
print('Hello, Python!')
# Output:
# (after 3 seconds) 'Hello, Python!'
In this code, the program pauses for 3 seconds before printing ‘Hello, Python!’. This delay might seem trivial in a small program, but it can be crucial in larger applications where timing matters.
The Pros and Cons of sleep()
The sleep()
function is a simple and effective way to introduce a delay in your program, but it’s not without its pitfalls. While it can be useful to control the pacing of your program, it’s important to remember that sleep()
halts all program execution. This means that while your program is ‘sleeping’, it’s not doing anything else – which might not be ideal in scenarios where multitasking is required. However, for simple use cases and beginner-level programs, it’s a handy tool to have in your Python toolkit.
sleep()
in Action: Intermediate Use Cases
As you become more comfortable with Python, you’ll find that the sleep()
function can be used in more complex scenarios, such as within loops or when working with threads. Let’s explore these scenarios to gain a deeper understanding of the versatility of sleep()
.
Pausing within Loops
When used within a loop, sleep()
can introduce a delay in each iteration. This can be useful in various scenarios, such as when you’re making repeated requests to a server and want to avoid overloading it.
Here’s an example:
import time
for i in range(5):
print(i)
time.sleep(1)
# Output:
# 0
# (pauses for 1 second)
# 1
# (pauses for 1 second)
# 2
# (pauses for 1 second)
# 3
# (pauses for 1 second)
# 4
In this code, the program prints a number, pauses for 1 second, and then continues to the next iteration. This delay occurs at each iteration of the loop, controlling the pacing of the output.
Working with Threads
When used with threading, sleep()
can be a powerful tool to control the execution of different threads. In Python, threading allows for the execution of multiple threads in parallel, and sleep()
can be used to manage these threads.
Here’s an example:
import time
import threading
def worker():
print('Worker started')
time.sleep(3)
print('Worker finished')
thread = threading.Thread(target=worker)
thread.start()
print('Main thread continues')
thread.join()
# Output:
# Worker started
# Main thread continues
# (after 3 seconds) Worker finished
In this code, the worker
function is started in a new thread. The sleep()
function is used to pause the worker
thread, but the main thread continues to execute. This allows for concurrent execution, demonstrating the power of sleep()
when used in a multithreaded context.
Exploring Alternatives: Beyond sleep()
While the sleep()
function is a straightforward and effective way to pause a Python program, there are other methods to consider. In this section, we’ll introduce two alternatives: the timeit
module and the asyncio.sleep()
function.
Timing with timeit
The timeit
module provides a simple way to time small bits of Python code. It has both a command-line interface and a callable one. One of its advantages is that it avoids a number of common traps for measuring execution times.
Here’s an example of how to use timeit
:
import timeit
start_time = timeit.default_timer()
# code you want to evaluate
time.sleep(2)
end_time = timeit.default_timer()
print('Elapsed time: ', end_time - start_time)
# Output:
# 'Elapsed time: 2.0001234567890123'
In this code, timeit.default_timer()
is used to mark the start and end times, and the difference gives the elapsed time. This can be used as an alternative to sleep()
when you want to control the execution time of a specific block of code.
Asynchronous Sleep with asyncio.sleep()
The asyncio
module provides tools for building concurrent applications using coroutines, multiplexing I/O access over sockets and other resources, running network clients and servers, and other related primitives. The asyncio.sleep()
function is a coroutine that completes after a given time (in seconds).
Here’s an example:
import asyncio
async def main():
print('Hello ...')
await asyncio.sleep(1)
print('... World!')
# Python 3.7+
asyncio.run(main())
# Output:
# 'Hello ...'
# (after 1 second) '... World!'
In this code, asyncio.sleep()
is used to create a non-blocking delay. It’s an excellent alternative when you’re working with asyncio and want your coroutines to sleep without blocking the event loop.
Resolving sleep()
Pitfalls: Troubleshooting and Best Practices
While the sleep()
function in Python is a powerful tool, it can sometimes lead to unforeseen issues. Two of the most common challenges are unresponsive programs and timing issues. Let’s discuss these problems and their solutions.
Dealing with Unresponsive Programs
When the sleep()
function is used, it halts all program execution. This can sometimes make the program appear unresponsive, especially when a large sleep duration is specified.
import time
time.sleep(10)
print('Hello, Python!')
# Output:
# (after 10 seconds) 'Hello, Python!'
In this example, the program seems to be doing nothing for 10 seconds before it prints ‘Hello, Python!’. To avoid this, consider using smaller sleep durations or using sleep()
in conjunction with multithreading to allow other parts of your program to run during the sleep period.
Navigating Timing Issues
Another common issue is timing precision. The sleep()
function’s delay might not be exactly as specified due to the way the underlying system handles timing.
import time
start_time = time.time()
time.sleep(1.5)
end_time = time.time()
print('Elapsed time: ', end_time - start_time)
# Output:
# 'Elapsed time: 1.5001234567890123'
In this code, we asked the program to sleep for 1.5 seconds, but the actual elapsed time was slightly more. This discrepancy is due to factors like system load and task scheduling, which can affect the precision of sleep()
. To navigate this, consider using time.perf_counter()
for a higher-resolution timer or adjusting your program to account for potential timing discrepancies.
Remember, the sleep()
function is a powerful tool, but like all tools, it’s essential to understand its potential issues to use it effectively.
Understanding Time in Programming: The Role of sleep()
The concept of time in programming is a fundamental one. It’s not just about tracking the current date and time; it’s also about managing the flow of operations in our code. The sleep()
function plays a crucial role in this aspect, allowing us to introduce deliberate pauses in our program’s execution.
But why would we need to pause a program? There are several reasons. For instance, we might want to simulate user interaction, where actions don’t occur simultaneously but have a delay between them. Or we might need to throttle our requests to a server to avoid overwhelming it. In these scenarios and more, sleep()
comes into play.
Let’s take a closer look at how sleep()
works:
import time
time.sleep(2)
print('Hello, Python!')
# Output:
# (after 2 seconds) 'Hello, Python!'
In this code, the sleep()
function pauses the program for 2 seconds before printing ‘Hello, Python!’. But what’s happening under the hood?
When we call time.sleep(2)
, the Python interpreter sends a signal to the operating system saying, ‘Pause this program’s execution for 2 seconds.’ The operating system then puts the program on hold, allowing other programs to use the CPU during this time. After 2 seconds, the operating system signals the Python interpreter to continue the program’s execution.
This interaction with the operating system is why the sleep()
function’s delay might not be exactly as specified – factors like system load and task scheduling can affect the actual delay. However, for most purposes, sleep()
provides an easy-to-use, effective way to manage time in your Python programs.
Real-World Applications of sleep()
The sleep()
function in Python is not just a theoretical concept; it has practical applications in real-world scenarios. Let’s explore how sleep()
can be used in web scraping and simulating user interaction.
Web Scraping with sleep()
Web scraping involves programmatically visiting web pages and extracting information. However, making too many requests in a short period can lead to your IP being blocked. Here’s where sleep()
comes in handy:
import time
import requests
from bs4 import BeautifulSoup
for url in list_of_urls:
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# Extract information from soup
time.sleep(5) # Pause for 5 seconds between requests
In this code, the sleep()
function is used to introduce a delay between requests, reducing the risk of being blocked by the server.
Simulating User Interaction with sleep()
When creating automated tests for a user interface, you might need to simulate user behavior. Users don’t perform actions instantaneously; they take time between actions. sleep()
can help simulate this delay:
import time
# Assume we have a function click_button() that simulates a button click
click_button('Start')
time.sleep(2) # Wait for 2 seconds
click_button('Next')
time.sleep(2) # Wait for 2 seconds
click_button('Submit')
In this code, sleep()
is used to introduce a realistic delay between simulated user actions.
The sleep()
function in Python is a versatile tool with a wide range of applications. If you’re interested in diving deeper, consider exploring how sleep()
can be used in other contexts, such as network programming, game development, or data processing.
Further Resources for Python Functions
Venturing further into the realm of Python functions? The following additional resources have been carefully chosen to assist you in your learning journey:
- Python Built-In Functions: Your Essential Toolkit – Learn how to use built-in functions for working with environments and variables.
Python Union: Combining Sets and Data Structures – Explore Python’s “union” operation for combining sets and collections.
Exploring Sorting Algorithms in Python – Dive into sorting techniques such as quicksort, mergesort, and heapsort.
Essential Python Built-In Functions for Data Science – A comprehensive guide highlighting key built-in Python functions for data science.
Python’s Official Documentation on Math Module delves into the functionalities of Python’s math module.
Python’s Official Documentation on Built-In Functions – Go through the official documentation to understand Python’s built-in functions and their usages.
These materials are designed to provide comprehensive insights into Python functions, thereby sharpening your programming skills in Python.
Wrapping Up: A Recap of Python’s sleep()
In our exploration of Python’s sleep()
function, we’ve covered its basic usage, delved into more advanced applications, and even explored alternative methods for pausing a program.
We started with the basics, demonstrating how sleep()
can introduce a simple delay in a Python program:
import time
time.sleep(3)
print('Hello, Python!')
# Output:
# (after 3 seconds) 'Hello, Python!'
We then ventured into more complex scenarios, showing how sleep()
can be used within loops and threads to control the pacing of program execution.
We also discussed potential pitfalls when using sleep()
, such as unresponsive programs and timing discrepancies, and offered solutions to these issues.
Finally, we explored alternative methods for pausing a program, including the timeit
module for timing code execution and asyncio.sleep()
for non-blocking delays in asyncio applications.
The sleep()
function is a versatile tool in Python, useful in a variety of real-world scenarios, from web scraping to simulating user interaction. Whether you’re a beginner just starting out with Python or an experienced developer, understanding sleep()
and its alternatives can be a valuable addition to your programming toolkit.