Python Queue Class | Usage Guide (With Examples)
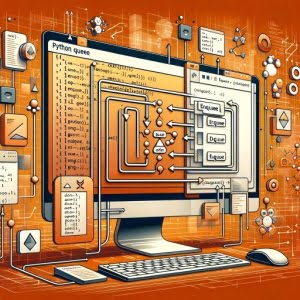
Ever wondered how to manage a sequence of items in Python? Picture a well-organized line at a grocery store, where everyone knows their turn, and the process flows smoothly. This is akin to Python’s queue
module, a powerful tool that allows you to efficiently manage your data.
In this comprehensive guide, we’ll navigate through the ins and outs of using queues in Python. We’ll start with the basics and gradually delve into advanced techniques, ensuring you master Python queues by the end of this read.
Whether you’re a beginner just starting with Python, or an intermediate user looking to expand your knowledge, this guide will serve as a valuable resource. We’ll provide clear code examples, explain each step in detail, and discuss the importance of queues in programming.
So, let’s embark on this journey of mastering Python queues together.
TL;DR: How Do I Use a Queue in Python?
Python’s built-in module,
queue
, provides several classes that help you create and manage queues. Here’s a simple example:
import queue
q = queue.Queue()
q.put('item')
print(q.get()) # Output: 'item'
In the above example, we first import the queue
module. We then initialize a queue instance. Using the put
method, we add an ‘item’ to the queue. The get
method is used to retrieve and remove the first item from the queue, which in this case outputs ‘item’.
This is just a basic example. Stay tuned as we delve into more detailed usage and advanced techniques of Python queues.
Table of Contents
- Python Queues: The Basics
- Exploring Advanced Queues in Python
- Alternative Methods for Queue Management in Python
- Troubleshooting Common Issues in Python Queues
- Understanding the Concept of Queues
- Python Queues in Real-World Applications
- Further Resources for Python Modules
- Python Queues: A Comprehensive Recap
Python Queues: The Basics
Python’s queue
module is a built-in module that provides several classes to create and manage a queue. The most basic class is Queue
, which creates a FIFO (First-In, First-Out) queue. Here’s how you can create a queue, add items to it, and remove items from it:
import queue
# Create a queue
q = queue.Queue()
# Add items to the queue
q.put('Apple')
q.put('Banana')
q.put('Cherry')
# Remove items from the queue
print(q.get()) # Output: 'Apple'
print(q.get()) # Output: 'Banana'
# Check if the queue is empty
print(q.empty()) # Output: False
print(q.get()) # Output: 'Cherry'
print(q.empty()) # Output: True
In the above example, we first import the queue
module and create a queue instance q
. We then use the put
method to add items to the queue. The get
method is used to remove and return the first item from the queue. The empty
method checks if the queue is empty and returns a boolean value.
This basic usage of Python’s queue
module is straightforward and intuitive. However, it’s important to note that the get
method will block if the queue is empty, unless block=False
is set. If block=False
and the queue is empty, the method will raise a queue.Empty
exception. Always make sure to check if the queue is empty before using the get
method to avoid this potential pitfall.
Exploring Advanced Queues in Python
Python’s queue
module offers more than just the basic FIFO queue. It also provides other types of queues such as LifoQueue
and PriorityQueue
.
Using LifoQueue
LifoQueue
, or Last-In-First-Out queue, operates as a stack where the last item added is the first one to be removed. Here’s an example:
import queue
# Create a LifoQueue
lq = queue.LifoQueue()
# Add items to the queue
lq.put('Apple')
lq.put('Banana')
lq.put('Cherry')
# Remove items from the queue
print(lq.get()) # Output: 'Cherry'
print(lq.get()) # Output: 'Banana'
print(lq.get()) # Output: 'Apple'
In the above example, the get
method removes the last item added to the queue, which is different from the FIFO behavior of the basic Queue
.
Using PriorityQueue
PriorityQueue
is another type of queue provided by Python’s queue
module. In a PriorityQueue
, each item is given a priority. Items with higher priority are dequeued before items with lower priority. If two items have the same priority, they are dequeued based on their order in the queue. Here’s how you can use a PriorityQueue
:
import queue
# Create a PriorityQueue
pq = queue.PriorityQueue()
# Add items to the queue
pq.put((2, 'Apple'))
pq.put((3, 'Banana'))
pq.put((1, 'Cherry'))
# Remove items from the queue
print(pq.get()[1]) # Output: 'Cherry'
print(pq.get()[1]) # Output: 'Apple'
print(pq.get()[1]) # Output: 'Banana'
In the above example, each item in the queue is a tuple where the first element is the priority and the second element is the item itself. The get
method removes the item with the highest priority (i.e., the item with the smallest number).
Understanding and utilizing these different types of queues can greatly enhance your data handling capabilities in Python. Remember to choose the type of queue based on your specific needs – FIFO for maintaining order, LIFO for stack-like behavior, and Priority for handling data with varying importance.
Alternative Methods for Queue Management in Python
While Python’s queue
module provides robust queue management, there are alternative approaches available. These include using lists, collections.deque
, and third-party libraries. Each approach has its own advantages and disadvantages.
Using Lists
Python lists can be used as queues by using the append
method to add items and the pop(0)
method to remove items. However, lists are not efficient for this purpose because the pop(0)
operation is not fast—it requires shifting the positions of all other elements.
# Create a queue using a list
list_queue = []
# Add items to the queue
list_queue.append('Apple')
list_queue.append('Banana')
list_queue.append('Cherry')
# Remove items from the queue
print(list_queue.pop(0)) # Output: 'Apple'
print(list_queue.pop(0)) # Output: 'Banana'
Using collections.deque
Python’s collections.deque
is a double-ended queue. It supports adding and removing elements from either end with fast O(1) operations. This makes it an excellent choice for implementing a queue.
import collections
# Create a queue using collections.deque
deque_queue = collections.deque()
# Add items to the queue
deque_queue.append('Apple')
deque_queue.append('Banana')
deque_queue.append('Cherry')
# Remove items from the queue
print(deque_queue.popleft()) # Output: 'Apple'
print(deque_queue.popleft()) # Output: 'Banana'
Using Third-Party Libraries
There are also third-party libraries, such as queue-stacks-python
, that offer additional features like thread-safety and blocking operations.
While these alternative approaches can be useful in certain scenarios, it’s important to consider their trade-offs. Lists are simple and built-in but not efficient for large queues. collections.deque
is fast and efficient but lacks some features of the queue
module. Third-party libraries can provide additional features but come with the cost of external dependencies.
Method | Advantages | Disadvantages |
---|---|---|
List | Simple, built-in | Not efficient for large queues |
collections.deque | Fast, efficient, built-in | Lacks some features of the queue module |
Third-party libs | Can offer additional features | External dependencies |
Choosing the right tool for your specific needs is crucial in programming. Hopefully, this exploration of alternative queue management methods in Python has broadened your toolbox.
Troubleshooting Common Issues in Python Queues
While Python’s queue
module is an efficient tool for managing data sequences, it’s not without its potential pitfalls. Here, we’ll explore common issues you may encounter and offer solutions and workarounds.
Threading Issues
Python queues are thread-safe, meaning they can be accessed by multiple threads simultaneously without the risk of data corruption. However, you might encounter issues when multiple threads try to read from an empty queue at the same time.
To handle this, you can use the queue
module’s empty
method to check if the queue is empty before trying to get
an item. However, this isn’t a foolproof solution due to the inherent race condition between the empty
and get
calls.
A safer approach is to catch the queue.Empty
exception, which is raised when get
is called on an empty queue with block=False
.
import queue
q = queue.Queue()
try:
item = q.get(block=False)
except queue.Empty:
print('The queue is empty.') # Output: 'The queue is empty.'
Queue Blocking
The get
method will block if the queue is empty and block=True
is set (which is the default). This means that the program will halt until an item is available in the queue. To avoid this, you can set block=False
, but be aware that this will raise a queue.Empty
exception if the queue is empty.
import queue
q = queue.Queue()
try:
item = q.get(block=False)
except queue.Empty:
print('The queue is empty.') # Output: 'The queue is empty.'
If you want to avoid blocking without raising an exception, you can use get_nowait
, which is equivalent to get(block=False)
.
import queue
q = queue.Queue()
try:
item = q.get_nowait()
except queue.Empty:
print('The queue is empty.') # Output: 'The queue is empty.'
Understanding these common issues and their solutions can help you use Python queues more effectively and troubleshoot any problems that arise.
Understanding the Concept of Queues
In programming, a queue is a collection of elements that maintains the order in which elements are added. It follows the FIFO (First-In, First-Out) principle, which means the first element added is the first one to be removed. This is similar to a real-life queue, such as a line of customers waiting to check out at a grocery store.
import queue
# Create a queue
q = queue.Queue()
# Add items to the queue
q.put('Customer 1')
q.put('Customer 2')
q.put('Customer 3')
# Remove items from the queue
print(q.get()) # Output: 'Customer 1'
print(q.get()) # Output: 'Customer 2'
In the above example, ‘Customer 1’ is the first to be added to the queue and the first to be removed, followed by ‘Customer 2’. This demonstrates the FIFO principle of a queue.
Queues are important in programming because they help manage data in a controlled and orderly manner. They are particularly useful in scenarios where order matters, such as processing tasks in the order they were received.
Python offers different types of queues, each with its own use cases. The basic Queue
follows the FIFO principle and is useful for maintaining order. The LifoQueue
operates as a stack, following the LIFO (Last-In, First-Out) principle, and is useful when you want to access the most recently added element first. The PriorityQueue
allows for complexity by assigning a priority to each element, and is useful when elements need to be processed based on importance or priority.
Understanding the concept and properties of queues is fundamental to using them effectively in your Python programming journey.
Python Queues in Real-World Applications
Python queues are not just abstract data structures. They have practical applications in a variety of real-world scenarios.
Web Server Requests
When a web server receives multiple simultaneous requests, it uses a queue to process them in an orderly manner. This ensures that every request is handled, even during peak traffic times.
import queue
import time
# Simulate web server requests
requests = queue.Queue()
# Add requests to the queue
for i in range(5):
requests.put(f'Request {i + 1}')
# Process requests
while not requests.empty():
request = requests.get()
print(f'Processing {request}...') # Output: Processing Request 1... etc.
time.sleep(1) # Simulate time it takes to process a request
In this example, we simulate a web server processing multiple requests. The server adds each incoming request to a queue and processes them one by one.
Print Spooling
Print spooling is another common use of queues. When multiple print jobs are sent to a printer at the same time, they are added to a queue. The printer then processes each job in the order they were received.
Exploring Related Concepts
Queues are also closely related to other concepts in computer science, such as multithreading and multiprocessing. These topics go hand in hand with queues, as they often involve managing multiple tasks simultaneously.
Further Resources for Python Modules
To deepen your understanding of Python queues and their applications, consider exploring these related topics. You can find many resources online, such as tutorials, documentation, and forums, to help guide your learning journey.
- Python Modules Use Cases Explored – Discover modules for handling file operations, dates, and times.
Simplifying Priority Queue Operations in Python – Learn how to prioritize and manage tasks with Python’s priority queue.
Simplifying Random Integer Generation in Python – Discover how to control and manipulate random integers in Python.
Python Advanced Interview Questions – Dive into queue implementation in Python with WanderInDev’s detailed guide.
A Complete Guide to Queues in Python by Towards Data Science, covers the concept and implementation of queues in Python.
Python Thread Queue – Learn about Python’s Thread Queue for managing threads in high-speed processing scenarios.
Python Queues: A Comprehensive Recap
We’ve taken a deep dive into Python queues, exploring their creation, manipulation, and various types available in Python’s queue
module. We’ve seen how the Queue
class provides a basic FIFO queue, while LifoQueue
and PriorityQueue
offer more specialized queue structures. We also discussed how to use these different queues effectively.
# Basic Queue
q = queue.Queue()
q.put('item')
print(q.get()) # Output: 'item'
# LifoQueue
lq = queue.LifoQueue()
lq.put('item')
print(lq.get()) # Output: 'item'
# PriorityQueue
pq = queue.PriorityQueue()
pq.put((1, 'item'))
print(pq.get()[1]) # Output: 'item'
We’ve looked at common issues, such as threading problems and queue blocking, offering solutions and workarounds for these potential pitfalls. We’ve also explored alternative approaches to handling queues in Python, including using lists, collections.deque
, and third-party libraries, each with its own pros and cons.
Method | Advantages | Disadvantages |
---|---|---|
List | Simple, built-in | Not efficient for large queues |
collections.deque | Fast, efficient, built-in | Lacks some features of the queue module |
Third-party libs | Can offer additional features | External dependencies |
Finally, we’ve discussed the practical applications of Python queues, from web server requests to print spooling, and encouraged exploration of related concepts like multithreading and multiprocessing.
Whether you’re a beginner just starting with Python, or an intermediate user looking to expand your knowledge, we hope this guide has provided a valuable and comprehensive insight into mastering Python queues.