Python Priority Queue | Practical Guide with Examples
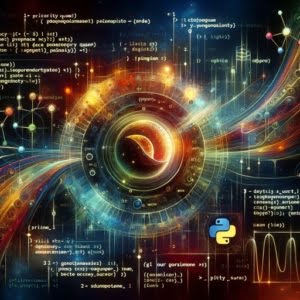
Welcome to the fascinating world of data structures! In this comprehensive guide, we delve into the realm of the Priority Queue, a powerful tool for managing and manipulating data.
We’re also exploring how Python, a popular programming language, enhances the capabilities of the Priority Queue. Think of Priority Queue like a hospital emergency room. Patients are treated based on the severity of their conditions, not on a first-come, first-serve basis.
Similarly, in a Priority Queue, elements are served according to their priority. Whether you’re a coding novice or a seasoned programmer, this guide, complete with practical applications, is sure to enrich your understanding of data management techniques. So, buckle up and let’s embark on this exciting journey!
TL;DR: What is a Priority Queue?
In Python, A priority queue is a unique type of queue where each element is associated with a priority and is served according to that priority. The higher the priority, the sooner the element is served. Imagine you’re at the airport check-in counter. Some passengers, such as those with first-class tickets or special memberships, are given higher priority and get to check-in before others. This scenario perfectly illustrates how a Priority Queue operates.
Priority Queue Example:
from queue import PriorityQueue
# Create a priority queue
q = PriorityQueue()
# Add elements to the queue with their respective priority
q.put((2, 'mid-priority item'))
q.put((1, 'high-priority item'))
q.put((3, 'low-priority item'))
# Pop and print elements in priority order
while not q.empty():
item = q.get()
print(item[1])
When you run this code, the output would be:
high-priority item
mid-priority item
low-priority item
In this example, as well, the item with a high priority is served first, followed by items with mid and low priorities.
Table of Contents
Priority Queue vs Regular Queue
So, how does a Priority Queue differ from a standard Queue? In a typical Queue, the first element to enter is the first to leave (FIFO – First In First Out). However, in a Priority Queue, this order is determined by the priority of the elements. So, an element that enters the queue later could leave earlier if its priority is higher.
Queue Type | Order of Serving Elements |
---|---|
Regular Queue | First In First Out (FIFO) |
Priority Queue | Based on priority of elements |
A Guided Walkthrough to Using PriorityQueue
Python offers a built-in PriorityQueue class in the queue module. This class implements the Priority Queue data structure, enabling us to handle and manipulate data efficiently and intuitively.
In Python’s PriorityQueue, lower numeric values indicate higher priority. Therefore, an element with a priority of 1 will be dequeued before an element with a priority of 2.
Python’s queue
module offers a variety of data structures, one of which is the PriorityQueue
class. This class helps us create a queue where elements are served based on their priority, making it a preferred choice for implementing Priority Queue in Python.
Python’s PriorityQueue Class
Let’s look at a simple example of how to use PriorityQueue
in Python:
from queue import PriorityQueue
# Create an instance of PriorityQueue
pq = PriorityQueue()
# Add elements to the queue
pq.put(3)
pq.put(1)
pq.put(2)
# Remove elements from the queue
while not pq.empty():
print(pq.get())
When you run this code, the output will be:
1
2
3
Interpreting the Output
The elements are removed from the queue in ascending order, not in the sequence they were added. This is a characteristic of Python’s PriorityQueue
, where lower numeric values represent higher priority. Hence, the element with the lowest value (1 in this case) is removed first.
The Role of Min-heap in PriorityQueue
Python’s PriorityQueue
operates using a data structure known as a min-heap. In a min-heap, the parent node is always less than or equal to its child nodes. This structure enables the PriorityQueue
to efficiently serve the element with the highest priority (lowest value).
Efficiency and Time Complexity
In terms of time complexity, inserting an element into a PriorityQueue
takes O(log n) time, while removing an element takes O(1) time.
Operation | Time Complexity |
---|---|
Inserting an element | O(log n) |
Removing an element | O(1) |
This efficiency makes PriorityQueue
a robust choice for handling large data sets. However, if your use case involves frequent priority updates or element searches, other data structures such as a search tree or a balanced binary heap may offer more efficiency.
Encountering Equal Priorities: A Common Challenge
In an ideal scenario, each item in our Priority Queue would possess a unique priority. However, we frequently encounter situations where multiple items share the same priority. This presents a dilemma: when two items have identical priorities, which one takes precedence?
Here, the concept of order stability comes into play. In a stable Priority Queue, if two items share the same priority, the item that was inserted first is served first.
Unfortunately, Python’s
PriorityQueue
does not guarantee order stability for items with equal priorities. Attempting to insert two items with the same priority might result in an error or unexpected behavior.
Overloading Comparison Operators: A Potential Solution
One approach to this problem involves using a custom class with overloaded comparison operators. By establishing our own comparison rules for objects, we can maintain order stability, even when priorities are equal. Here’s an example:
class Item:
def __init__(self, name):
self.name = name
def __lt__(self, other):
return self.name < other.name
pq = PriorityQueue()
pq.put((2, Item('item1')))
pq.put((1, Item('item2')))
pq.put((2, Item('item3')))
while not pq.empty():
print(pq.get()[1].name)
In this code, we’re defining an Item
class with a __lt__
method, which stands for ‘less than’. This method is utilized by Python’s PriorityQueue
to compare items and ascertain their order.
Handling Complex Scenarios
A Priority Queue stands out due to its ability to handle varied objects, not just numbers. This unique feature distinguishes it from many other data structures in Python. With the appropriate setup, you can prioritize strings, tuples, or even your custom objects. This versatility makes the Priority Queue a potent tool in Python’s data structure toolkit.
Let’s delve deeper with a more intricate example. Suppose we’re managing tasks for various customers, each assigned a priority. However, tasks from the same customer also have their individual priorities. To manage this, we can use the Task
and Customer
classes like so:
class Customer:
def __init__(self, name):
self.name = name
class Task:
def __init__(self, customer, priority):
self.customer = customer
self.priority = priority
def __lt__(self, other):
if self.priority == other.priority:
return self.customer.name < other.customer.name
return self.priority < other.priority
In this code, the Task
class has a __lt__
method that initially compares the tasks’ priorities. If the priorities are equal, it then compares the customers’ names.
The Role of Entry Counter
Another strategy to guarantee order stability involves using an entry counter. Each time an item is added to the queue, the entry counter increments. This counter then serves as a secondary priority to decide the order of items with equal priorities.
Here is an example of this by using the PriorityQueue class with an entry counter:
from queue import PriorityQueue
class PriorityQueueWithCounter:
def __init__(self):
self.pq = PriorityQueue()
self.entry_counter = 0
def put(self, item, priority):
# entry_counter serves to order items with the same priority
# (also ensures that two items with the same priority won't attempt to sort by comparing the values)
entry = [priority, self.entry_counter, item]
self.pq.put(entry)
self.entry_counter += 1
def get(self):
return self.pq.get()[-1]
def empty(self):
return self.pq.empty()
# Create a priority queue
q = PriorityQueueWithCounter()
# Add elements to the queue with their respective priority
q.put('Mid-priority item 1', 2)
q.put('High-priority item', 1)
q.put('Low-priority item', 3)
q.put('Mid-priority item 2', 2)
# Pop and print elements in priority order
while not q.empty():
print(q.get())
In this code block, the PriorityQueueWithCounter
class encapsulates a PriorityQueue
and an entry counter. When an item is pushed to the priority queue, its priority and the entry counter is stored with it. The get()
, put()
, and empty()
methods are used to interact with the underlying priority queue.
When you run the above code, you will get:
High-priority item
Mid-priority item 1
Mid-priority item 2
Low-priority item
Even though ‘Mid-priority item 1’ and ‘Mid-priority item 2’ have the same priority, they maintain the order in which they were inserted, thanks to the entry counter.
Performance with Large Data Sets
While Python’s PriorityQueue
class is efficient and user-friendly, remember that its performance gets worse with large data sets, particularly when many items share the same priority.
In such instances, alternative data structures or techniques may offer improved efficiency. However, for most applications, PriorityQueue
provides a satisfactory balance between simplicity and performance.
Thread Safety in Python’s PriorityQueue
Thread safety is a crucial concept in concurrent programming. A data structure is deemed thread-safe if it functions correctly when accessed from multiple threads, regardless of the scheduling or interleaving of the executions of these threads.
Python’s
PriorityQueue
is engineered to be thread-safe, enabling multiple threads to safely add or remove items without the need for additional synchronization mechanisms or locks. This characteristic makesPriorityQueue
an apt choice for multi-threaded applications.
Distinguishing PriorityQueue and Heap
PriorityQueue
and Heap
are both utilized to manage data in a specific order, but they are not identical. A Heap is a specialized tree-based data structure that satisfies the heap property.
In a min-heap, parent nodes are either less than or equal to their child nodes, while in a max-heap, parent nodes are greater than or equal to their child nodes.
Python’s heapq
module provides a min-heap, which is also used by the PriorityQueue
class. However, with some modifications, it can also function as a max-heap.
Conversely, PriorityQueue
is a higher-level abstraction that can employ a Heap as its underlying data structure but incorporates additional features, such as thread safety.
The heapq Module in Python
Python also offers a heapq
module, implementing the heap queue algorithm, also known as the priority queue algorithm.
While the heapq
module does not provide the higher-level features of the PriorityQueue
class, like thread safety, it delivers more control over the underlying data structure and can be more efficient for certain use cases.
Pros and Cons of heapq
While employing the heapq
module to implement a priority queue in Python offers more control and can be more efficient, it also presents its own set of challenges.
It lacks the thread safety of PriorityQueue
, and it necessitates a solid understanding of the heap queue algorithm for effective use. However, for specific use cases, particularly those that require custom comparison functions or frequent priority updates, heapq
can be a highly effective tool.
Priority Queue in Dijkstra’s Algorithm
Dijkstra’s algorithm, one of the most renowned algorithms that employ Priority Queue, is used to identify the shortest path in a graph from a source node to all other nodes. The Priority Queue is used to select the node with the smallest tentative distance to visit next. By leveraging a Priority Queue, Dijkstra’s algorithm can efficiently pinpoint the shortest path, even in expansive graphs.
Further Resources for Python Modules
To support your ongoing learning of Python Modules, we’ve compiled a selection of comprehensive resources:
- Python Modules Basics: Quick Insights – Learn about the “os” and “sys” modules for system-level tasks.
Parallel Processing with Python Multiprocessing – Dive into parallelism and distributed computing in Python.
Python Queue: A Quick Overview – Discover Python’s “queue” module for implementing data structures.
Queuelib on PyPI – Explore Queuelib, a Python library that provides a collection of persistent and in-memory queue implementations.
Stack & Queue in Python Using Module Queue – Navigate the concept and practical usage of the queue module in Python.
Python Queue Tutorial by Intellipaat explores the functionality and usage of Python’s queue.
By delving into these resources and applying the knowledge you gain, you’re investing in professional development and personal growth as a Python developer.
Wrapping Up
In this comprehensive guide, we journeyed through the fascinating world of data structures, focusing on the versatile Priority Queue. We learned that the Priority Queue serves elements based on their priority, not just the order they were added.
We delved into the practical implementation of a Priority Queue in Python, exploring the built-in PriorityQueue class. We understood its workings and the use of the min-heap data structure to efficiently serve the highest-priority element. We also discussed the time complexities of inserting and removing elements from a PriorityQueue.
We tackled the challenge of equal priorities and order stability, understanding how to resolve this issue using a custom class with overloaded comparison operators or an entry counter. We also mentioned the heapq module in Python, comparing its advantages and disadvantages to the PriorityQueue class.
From basics to advanced topics, find it all in our Python syntax guidebook.
The Priority Queue is not just a theoretical concept; it’s a powerful tool with applications in a wide range of fields. Whether you’re a beginner just starting out or a seasoned programmer looking for more efficient data management techniques, understanding and using Priority Queue can significantly enhance your capabilities. So, go ahead, give it a try, and unlock the power of Priority Queue in Python!