Python Multiprocessing | Threaded Programming Guide
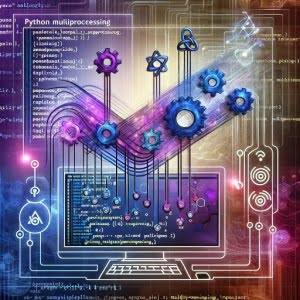
Ever wondered how to make your Python programs run faster and more efficiently? Like a skilled conductor leading an orchestra, Python’s multiprocessing module allows your programs to perform multiple tasks simultaneously.
This power-packed feature leverages the full potential of your computer’s processors, making your Python programs run like a breeze.
In this comprehensive guide, we will walk you through the ins and outs of Python multiprocessing. We will start from understanding the basic usage and gradually move towards mastering advanced techniques.
So, are you ready to dive into the world of Python multiprocessing? Let’s get started!
TL;DR: How Do I Use Multiprocessing in Python?
Python’s multiprocessing module allows you to create separate processes, which can run concurrently. Here’s a simple example:
from multiprocessing import Process
def print_func(continent='Asia'):
print('The name of continent is : ', continent)
if __name__ == "__main__": # confirms that the code is under main function
names = ['America', 'Europe', 'Africa']
procs = []
proc = Process(target=print_func) # instantiating without any argument
procs.append(proc)
proc.start()
# instantiating process with arguments
for name in names:
proc = Process(target=print_func, args=(name,))
procs.append(proc)
proc.start()
# complete the processes
for proc in procs:
proc.join()
# Output:
# The name of continent is : Asia
# The name of continent is : America
# The name of continent is : Europe
# The name of continent is : Africa
This simple Python script leverages the multiprocessing module to create four separate processes. Each process is tasked with printing the name of a continent. The Process
class is used to create processes, and the start()
method to initiate them. The join()
method ensures that the main program waits for all processes to complete before proceeding.
Intrigued? Read on for a more detailed explanation and advanced usage scenarios of Python’s multiprocessing module!
Table of Contents
- Getting Started with Python Multiprocessing
- Leveraging Python Multiprocessing: Beyond the Basics
- Exploring Concurrency Alternatives in Python
- Navigating Common Pitfalls in Python Multiprocessing
- Understanding Multiprocessing and Multithreading
- Python Multiprocessing: A Key for Data-Intensive Applications
- Further Resources for Python Modules
- Python Multiprocessing: A Recap and Review
Getting Started with Python Multiprocessing
Python’s multiprocessing module is a powerful tool that enables you to create and manage multiple processes concurrently. It is particularly useful when you need to perform several tasks simultaneously or when you want to leverage the full power of your multi-core processor.
Here’s a simple example of how to use the multiprocessing module:
from multiprocessing import Process
def worker():
print('Worker process is working.')
if __name__ == '__main__':
processes = [Process(target=worker) for _ in range(5)]
for process in processes:
process.start()
for process in processes:
process.join()
# Output:
# Worker process is working.
# Worker process is working.
# Worker process is working.
# Worker process is working.
# Worker process is working.
In this example, we first import the Process
class from the multiprocessing module. We then define a simple function worker()
that prints a message when called. In the if __name__ == '__main__'
block, we create a list of five Process
objects, each targeting the worker()
function. We then start each process using the start()
method and wait for all processes to complete using the join()
method.
The multiprocessing module provides a simple and intuitive API for managing concurrent processes. It allows you to create processes that run independently of each other, thus making your program faster and more efficient. However, it’s important to be mindful of potential pitfalls such as deadlocks and race conditions which can occur in concurrent programming. We’ll delve into these issues and how to avoid them in later sections.
Leveraging Python Multiprocessing: Beyond the Basics
As you become more comfortable with Python’s multiprocessing module, you’ll discover it offers much more than just running tasks concurrently. It provides advanced features like worker pools, process synchronization, and state sharing, which can significantly enhance your program’s performance and efficiency.
Worker Pools in Python Multiprocessing
Worker pools are a powerful feature that allows you to manage multiple worker processes. Instead of manually creating, starting, and joining processes, you can use a pool to automatically manage these tasks.
Here’s an example of how to use a worker pool:
from multiprocessing import Pool
def square(n):
return n * n
if __name__ == '__main__':
with Pool(5) as p:
numbers = [1, 2, 3, 4, 5]
results = p.map(square, numbers)
print(results)
# Output:
# [1, 4, 9, 16, 25]
In this example, we create a pool of five worker processes using the Pool
class. We then use the map
method to apply a function square
to a list of numbers. The map
method distributes the tasks to the worker processes and collects the results.
Synchronizing Processes in Python Multiprocessing
In concurrent programming, it’s often necessary to synchronize processes to ensure they don’t interfere with each other. Python’s multiprocessing module provides several ways to synchronize processes, such as Locks, Semaphores, and Conditions.
Here’s an example of how to use a Lock to synchronize processes:
from multiprocessing import Process, Lock
def printer(lock, text):
lock.acquire()
try:
print(text)
finally:
lock.release()
if __name__ == '__main__':
lock = Lock()
for i in range(10):
Process(target=printer, args=(lock, 'Hello world',)).start()
# Output:
# Hello world
# Hello world
# Hello world
# Hello world
# Hello world
# Hello world
# Hello world
# Hello world
# Hello world
# Hello world
In this example, we use a Lock
to ensure that only one process can access the print
function at a time. This prevents the processes from interfering with each other and ensures the output is as expected.
Sharing State Between Processes in Python Multiprocessing
Python’s multiprocessing module also allows processes to share state using shared memory or server processes. However, sharing state between processes can be tricky and should be done carefully to avoid issues like race conditions.
Here’s an example of how to share state using a Value:
from multiprocessing import Process, Value
def adder(num, val):
num.value += val
if __name__ == '__main__':
num = Value('d', 0.0)
Process(target=adder, args=(num, 1.0)).start()
Process(target=adder, args=(num, 2.0)).start()
Process(target=adder, args=(num, 3.0)).start()
print(num.value)
# Output:
# 6.0
In this example, we use a Value
to share a double (represented by ‘d’) between three processes. Each process adds a different value to the shared Value
. The final value of num
is the sum of the values added by each process.
These advanced features of Python’s multiprocessing module can greatly enhance your program’s performance and efficiency. However, they should be used carefully and correctly to avoid potential issues.
Exploring Concurrency Alternatives in Python
While Python’s multiprocessing module is a powerful tool for achieving concurrency, it’s not the only option. Python offers other methods for concurrent execution, such as threading and asyncio. Additionally, third-party libraries like Celery provide alternative ways to handle concurrent tasks.
Python Threading
Threading is a technique for concurrent execution where a single process contains multiple threads that can run simultaneously. Here’s a simple example of how to use threading in Python:
import threading
def worker(number):
print(f'Worker {number} is working.')
if __name__ == '__main__':
for i in range(5):
threading.Thread(target=worker, args=(i,)).start()
# Output:
# Worker 0 is working.
# Worker 1 is working.
# Worker 2 is working.
# Worker 3 is working.
# Worker 4 is working.
In this example, we create and start five threads, each targeting the worker function and passing a unique number as an argument. However, due to Python’s Global Interpreter Lock (GIL), threading might not provide a significant performance boost for CPU-bound tasks.
Python Asyncio
Asyncio is a library to write single-threaded concurrent code using coroutines, multiplexing I/O access over sockets and other resources, running network clients and servers, and other related primitives. Here’s a simple example:
import asyncio
async def main():
print('Hello')
await asyncio.sleep(1)
print('world')
asyncio.run(main())
# Output:
# Hello
# (after one second) world
This example demonstrates the use of asyncio to handle IO-bound tasks efficiently. However, it might not be suitable for CPU-bound tasks due to the single-threaded nature of the event loop.
Celery
Celery is a powerful third-party library that allows you to distribute tasks across multiple worker nodes. It supports both task queues for distributing work across threads or machines and scheduling for executing tasks at specific times. However, it requires a message broker like RabbitMQ or Redis, which might increase the complexity of your setup.
In conclusion, while Python’s multiprocessing module is a powerful tool for achieving concurrency, other methods like threading, asyncio, and third-party libraries like Celery provide alternative ways to handle concurrent tasks. Depending on your specific needs and the nature of your tasks (CPU-bound or IO-bound), you might find one method more suitable than others. It’s recommended to understand the advantages and disadvantages of each method and choose the one that fits your needs best.
While Python multiprocessing offers powerful capabilities, it’s not without its challenges. Issues such as deadlocks, race conditions, and shared state problems can arise. However, with the right knowledge, these can be effectively managed.
Dealing with Deadlocks
A deadlock is a situation where a process is unable to proceed because it’s waiting for resources held by another process, which in turn is waiting for resources held by the first process. Deadlocks can cause your program to hang indefinitely. Here’s an example of a potential deadlock situation:
from multiprocessing import Process, Lock
def worker(lock1, lock2):
with lock1:
with lock2:
print('Hello, world!')
if __name__ == '__main__':
lock1, lock2 = Lock(), Lock()
Process(target=worker, args=(lock1, lock2)).start()
Process(target=worker, args=(lock2, lock1)).start()
In this example, the two worker processes may deadlock if they acquire their locks in different orders. To avoid deadlocks, always ensure that locks are acquired and released in the same order.
Managing Race Conditions
A race condition occurs when two or more processes access and manipulate shared data concurrently, and the outcome of the execution depends on the particular order in which the access takes place. Here’s an example of a race condition:
from multiprocessing import Process, Value
def adder(num, val):
num.value += val
if __name__ == '__main__':
num = Value('d', 0.0)
Process(target=adder, args=(num, 1.0)).start()
Process(target=adder, args=(num, 2.0)).start()
print(num.value)
# Output:
# 1.0 or 2.0 or 3.0
In this example, the final value of num
depends on the order in which the processes execute. To avoid race conditions, use locks or other synchronization mechanisms to ensure that only one process can access the shared data at a time.
Handling Shared State Issues
Sharing state between processes can be tricky due to the isolated nature of processes. If not managed properly, it can lead to inconsistencies and unexpected behavior. The multiprocessing module provides several ways to share state, such as Value and Array, but they should be used carefully to avoid potential issues.
In conclusion, while Python multiprocessing is a powerful tool, it’s not without its challenges. However, with the right knowledge and careful coding, these challenges can be effectively managed.
Understanding Multiprocessing and Multithreading
Before we delve deeper into Python multiprocessing, it’s crucial to understand the fundamental concepts of multiprocessing and multithreading and how they differ.
Multiprocessing vs. Multithreading
In a nutshell, multiprocessing involves running tasks on different processors simultaneously. Each process runs independently and has its own Python interpreter and memory space. This independence makes multiprocessing ideal for CPU-bound tasks, as it can effectively leverage multiple CPU cores.
On the other hand, multithreading involves running different threads within the same process. Threads share the same memory space, making communication between them faster and more efficient. However, due to this shared memory space, threads need to be coordinated to prevent conflicts, especially when they’re modifying shared data.
Here’s a simple comparison of multiprocessing and multithreading:
— | Multiprocessing | Multithreading |
---|---|---|
Suitability | CPU-bound tasks | I/O-bound tasks |
Memory Space | Separate for each process | Shared among all threads |
Communication | Slower due to interprocess communication | Faster due to shared memory |
Coordination | Less necessary due to process isolation | Necessary to prevent conflicts |
The Global Interpreter Lock (GIL) in Python
In Python, the Global Interpreter Lock (GIL) is a mechanism that prevents multiple native threads from executing Python bytecodes simultaneously. This lock is necessary because Python’s memory management is not thread-safe.
The GIL can be a bottleneck in multithreaded programs, as it prevents threads from running in true parallel on multiple cores. However, each Python process has its own Python interpreter and its own GIL, so the GIL’s impact is mitigated in multiprocessing.
Here’s an example to illustrate the GIL’s impact:
import time
import threading
def count(n):
while n > 0:
n -= 1
# Single thread
start = time.time()
count(100000000)
end = time.time()
print('Single thread:', end - start)
# Two threads
start = time.time()
thread1 = threading.Thread(target=count,args=(50000000,))
thread2 = threading.Thread(target=count,args=(50000000,))
thread1.start()
thread2.start()
thread1.join()
thread2.join()
end = time.time()
print('Two threads:', end - start)
# Output:
# Single thread: X seconds
# Two threads: Y seconds
This example demonstrates that the two-thread version doesn’t run twice as fast as the single-thread version due to the GIL, even though we’re running on a multi-core processor.
In conclusion, Python’s multiprocessing module provides a solution to the GIL limitation by allowing us to create separate processes that can run concurrently on different processors. This makes it a powerful tool for optimizing the performance of CPU-bound tasks in Python.
Python Multiprocessing: A Key for Data-Intensive Applications
Python’s multiprocessing module isn’t just a tool for optimizing performance; it’s a key that unlocks new possibilities for data-intensive applications. Whether you’re web scraping, analyzing large data sets, or building complex simulations, multiprocessing can help you get the job done faster and more efficiently.
Multiprocessing in Data-Intensive Applications
Consider a data analysis task where you need to apply a complex computation to a large dataset. Without multiprocessing, you’d have to apply the computation to each data point sequentially, which could take a significant amount of time. With multiprocessing, you could split the dataset into chunks and process them concurrently, potentially reducing the computation time drastically.
Here’s an example of how you might use multiprocessing in a data analysis task:
from multiprocessing import Pool
import numpy as np
def compute(data):
return np.sum(data ** 2)
if __name__ == '__main__':
data = np.random.rand(1000000)
with Pool(4) as p:
results = p.map(compute, np.array_split(data, 4))
total = np.sum(results)
print(total)
# Output:
# [A random number]
In this example, we use a Pool
of worker processes to compute the sum of squares of a large array of random numbers. We split the array into four chunks and process them concurrently. The final result is the sum of the results from each chunk.
Python Multiprocessing in Web Scraping
In web scraping, you often need to send multiple requests to different URLs. Without multiprocessing, you’d have to send these requests one by one, waiting for each to complete before sending the next. With multiprocessing, you can send multiple requests concurrently, significantly speeding up the scraping process.
Exploring Related Concepts
While multiprocessing is a powerful tool, it’s just one piece of the concurrency puzzle in Python. Other concepts like asynchronous programming with asyncio
, distributed computing with dask
or ray
, and parallel programming with joblib
or concurrent.futures
can further enhance your ability to write efficient, high-performance Python code.
Further Resources for Python Modules
If you’re interested in diving deeper into these topics, we recommend checking out the following resources:
- Python Modules Mastery: Step-by-Step – Discover modules for networking, socket programming, and web requests.
Concurrent Programming with Python Threading – Learn to create multithreaded applications using Python’s threading.
Priority Queue Implementation in Python – Dive deep into priority queue concepts and real-world examples.
Multiprocessing in Python explains the concept and implementation of multiprocessing in Python in this Medium article.
Multiprocessing in Python Article on Linux Journal breaks down the complexities of Python multiprocessing.
Python Multiprocessing Basics – Learn about the functions and usage of Python’s multiprocessing module with the Python Module of the Week series.
Remember, the key to mastering concurrency in Python is understanding the underlying concepts and knowing when and how to apply them in your code.
Python Multiprocessing: A Recap and Review
In this comprehensive guide, we’ve explored Python’s multiprocessing module, a powerful tool for optimizing the performance of CPU-bound tasks. We’ve seen how to create, start, and manage processes, and how to share state and synchronize processes to prevent issues like race conditions and deadlocks.
We’ve also looked at advanced features like worker pools, which can simplify the management of multiple worker processes, and considered the potential pitfalls and how to avoid them.
In addition to Python’s built-in multiprocessing module, we’ve also touched upon alternative approaches to handle concurrent tasks, such as threading, asyncio, and third-party libraries like Celery. These alternatives each have their strengths and weaknesses, and the best choice depends on your specific needs and the nature of your tasks.
Here’s a quick comparison of the methods we’ve discussed:
— | Multiprocessing | Threading | Asyncio | Celery |
---|---|---|---|---|
Suitability | CPU-bound tasks | I/O-bound tasks | I/O-bound tasks | Distributed tasks |
Memory Space | Separate for each process | Shared among all threads | Shared among all tasks | Depends on the setup |
Communication | Interprocess communication | Shared memory | Event loop | Message broker |
Coordination | Less necessary | Necessary | Necessary | Necessary |
Remember, the key to mastering concurrency in Python is understanding the underlying concepts and knowing when and how to apply them in your code. Python’s multiprocessing module is a powerful tool, but it’s just one piece of the puzzle. Don’t be afraid to explore other options and choose the one that fits your needs best.