Java Annotations: Mastering the Basics and Beyond
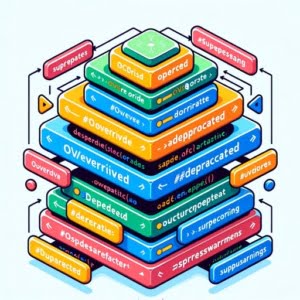
Are you finding it challenging to decipher Java Annotations? You’re not alone. Many developers find themselves puzzled when it comes to understanding and using Java Annotations effectively.
Think of Java Annotations as a secret code – a powerful tool that can add meaningful metadata to your code, enhancing its functionality and readability.
This guide will walk you through the ins and outs of using Java Annotations, from the basics to more advanced techniques. We’ll cover everything from using built-in Java Annotations like @Override, @Deprecated, @SuppressWarnings, to creating and using custom annotations, and even discuss alternative approaches to handle metadata in Java.
So, let’s dive in and start mastering Java Annotations!
TL;DR: What are Java Annotations?
Java Annotations are a form of metadata that provide data about the program that is not part of the program itself. They are instantiated by preceding text with an
@
, for example@Deprecated
. They have no direct effect on the operation of the code they annotate.
Here’s a simple example:
@Override
public String toString() {
return "This is an override method";
}
# Output:
# 'This is an override method'
In this example, we’ve used the @Override
annotation. This tells the Java compiler that the following method overrides a method of its superclass. If the method doesn’t exist in one of the superclasses, the compiler will throw an error.
This is just a basic way to use Java Annotations, but there’s much more to learn about them. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
Understanding Basic Java Annotations
Java provides several built-in annotations which you can use in your programs. In this section, we will discuss three of them: @Override
, @Deprecated
, and @SuppressWarnings
. Each of these annotations serves a unique purpose and can be quite useful in different scenarios.
The @Override Annotation
The @Override
annotation indicates that a method is intended to override a method in a superclass. It helps to catch errors at compile time.
Here is an example of how to use the @Override
annotation:
public class Vehicle {
public void run() {
System.out.println("The vehicle is running");
}
}
public class Car extends Vehicle {
@Override
public void run() {
System.out.println("The car is running");
}
}
# Output:
# 'The car is running'
In the code above, the run
method in the Car
class overrides the run
method in the Vehicle
class. The @Override
annotation ensures that the compiler checks this.
The @Deprecated Annotation
The @Deprecated
annotation indicates that the marked element is deprecated and should no longer be used. The compiler generates a warning whenever a program uses a method, class, or field with the @Deprecated
annotation.
Here is an example:
public class Vehicle {
@Deprecated
public void start() {
System.out.println("The vehicle starts");
}
}
# Output:
# 'The vehicle starts'
In the code above, the start
method is marked as deprecated, which means it should no longer be used.
The @SuppressWarnings Annotation
The @SuppressWarnings
annotation tells the compiler to suppress specific warnings that it would otherwise generate.
Here is an example:
@SuppressWarnings("unchecked")
public void addItems(List list) {
list.add("apple");
list.add("banana");
}
# Output:
# 'apple'
# 'banana'
In the code above, the @SuppressWarnings
annotation suppresses unchecked warnings that could be generated during the compilation.
Understanding these basic Java Annotations and their correct use can help you write more efficient and error-free code.
Creating and Using Custom Annotations
As you become more comfortable with Java Annotations, you may find that the built-in annotations don’t cover all your needs. This is where custom annotations come into play. Custom annotations allow you to create your own annotations for specific use cases.
Creating a Custom Annotation
Creating a custom annotation involves defining an interface with the @interface
keyword. Let’s create a simple custom annotation called MyAnnotation
:
public @interface MyAnnotation {
String value() default "Default String";
int number() default 0;
}
In this code, we have defined a custom annotation MyAnnotation
with two elements, value
and number
. Each element has a default value.
Using a Custom Annotation
Once you have defined a custom annotation, you can use it just like any other annotation. Here’s how you might use our MyAnnotation
:
@MyAnnotation(value = "Custom String", number = 10)
public class MyClass {
// Class contents go here
}
In this code, we have annotated the MyClass
class with our MyAnnotation
. We have specified values for the value
and number
elements.
Analyzing the Output
Annotations do not directly affect the execution of your code. They are used by other parts of your code or tools that process your code. For example, you might write a tool that processes your code and uses the MyAnnotation
annotation to generate a report.
Best Practices
When creating and using custom annotations, keep the following best practices in mind:
- Use clear, descriptive names for your annotations and elements.
- Document your annotations thoroughly.
- Be mindful of the retention policy for your annotations. The retention policy determines at what point the annotation is discarded. Java’s built-in retention policies are
SOURCE
(discarded by the compiler),CLASS
(included in the bytecode, but not loaded by the JVM), andRUNTIME
(loaded by the JVM).
Mastering custom annotations can give you a lot more flexibility in how you use metadata in your Java programs.
Exploring Alternative Approaches to Metadata
While Java Annotations are a powerful tool for adding metadata to your code, they are not the only method. Let’s look at two alternative approaches: using comments and adopting naming conventions.
Using Comments for Metadata
One of the simplest ways to add metadata to your code is by using comments. This approach doesn’t require any special syntax or tools. Here’s an example:
// This method calculates the sum of two numbers
public int add(int a, int b) {
return a + b;
}
# Output:
# (No output, this is a comment)
In this code, the comment provides metadata about the add
method. However, comments are not machine-readable, so they cannot be used by tools that process your code.
Adopting Naming Conventions
Another approach is to adopt naming conventions that convey metadata. For example, you might adopt a convention where class names always start with a capital letter, and variable names always start with a lowercase letter.
public class MyClass {
private int myVariable;
}
# Output:
# (No output, this is a naming convention)
In this code, the naming convention provides metadata about MyClass
and myVariable
. Like comments, naming conventions are not machine-readable.
Comparing the Approaches
Approach | Advantages | Disadvantages |
---|---|---|
Java Annotations | Machine-readable, powerful | Requires understanding of annotations |
Comments | Simple, no special syntax | Not machine-readable |
Naming Conventions | Can convey a lot of information, no special syntax | Not machine-readable, requires consistent application |
While each of these methods has its advantages and disadvantages, Java Annotations offer the unique advantage of being machine-readable. This makes them a powerful tool for any Java developer.
Overcoming Challenges with Java Annotations
While Java Annotations can be a powerful tool in your programming arsenal, they can also introduce certain challenges. Let’s discuss some of the common issues you might encounter, along with their solutions and workarounds.
Dealing with Incorrect Usage
One common issue is incorrect usage of annotations. This could take the form of using an annotation in the wrong context, or misusing an annotation’s elements.
For example, using the @Override
annotation on a method that doesn’t actually override a method in a superclass will result in a compiler error.
public class MyClass {
@Override
public void myMethod() {
// Method contents go here
}
}
# Output:
# Compiler error: method does not override or implement a method from a supertype
In this code, the myMethod
method is marked with the @Override
annotation, but it doesn’t actually override a method in a superclass. The compiler will generate an error message.
To avoid this issue, make sure you understand the correct usage of each annotation before you use it.
Understanding Retention Policies
Another potential pitfall is misunderstanding an annotation’s retention policy. The retention policy determines at what point an annotation is discarded.
Java’s built-in retention policies are SOURCE
(discarded by the compiler), CLASS
(included in the bytecode, but not loaded by the JVM), and RUNTIME
(loaded by the JVM).
If you create a custom annotation and specify a retention policy of SOURCE
, the annotation will be discarded by the compiler and won’t be available at runtime.
@Retention(RetentionPolicy.SOURCE)
public @interface MyAnnotation {
// Annotation elements go here
}
# Output:
# (No output, this is a retention policy)
In this code, the MyAnnotation
annotation has a retention policy of SOURCE
, which means it will be discarded by the compiler.
To avoid issues with retention policies, make sure you understand the implications of each policy and choose the appropriate one for your annotations.
Java Annotations are a powerful tool, but like any tool, they require a certain level of understanding to use effectively. By being aware of these common issues and their solutions, you can use annotations more confidently and effectively in your Java programs.
The Fundamentals of Java Annotations
Java Annotations are a form of metadata – data about data. They provide a powerful way to add supplementary information to your code, which can be processed by the compiler, runtime environment, or other tools to influence the behavior of your code.
Purpose of Java Annotations
Java Annotations serve several purposes:
- Code Analysis: Tools can process annotation information to generate code, XML files, and so forth.
- Compiler Instructions: Annotations can provide the compiler with additional information about how to treat the annotated code.
- Runtime Processing: Some annotations are available at runtime and can be processed by your application to change its behavior.
Understanding Built-In, Custom, and Meta-Annotations
Java provides a set of built-in annotations, such as @Override
, @Deprecated
, and @SuppressWarnings
, which are designed for common tasks.
In addition to the built-in annotations, you can define your own custom annotations. A custom annotation is defined using the @interface
keyword, and can include elements that store values.
public @interface MyAnnotation {
String value() default "";
}
# Output:
# (No output, this is an annotation definition)
In this code, we define a custom annotation MyAnnotation
with one element, value
.
Finally, there are meta-annotations – annotations that apply to other annotations. These include @Target
, @Retention
, @Inherited
, and @Documented
. Meta-annotations provide information about the annotation they annotate, such as where it can be used, how long it is kept, and whether it is inherited by subclasses.
Understanding the fundamentals of Java Annotations, their purpose, and how they work is the first step to mastering their use in your Java programs.
Java Annotations in Larger Projects and Frameworks
Java Annotations are not just useful in small-scale projects; they play a crucial role in larger projects and popular frameworks like Spring and Hibernate. These frameworks heavily rely on annotations for tasks like dependency injection, transaction management, and ORM mapping, among others.
For example, in Spring, the @Autowired
annotation is used for automatic dependency injection. And in Hibernate, the @Entity
and @Table
annotations are used for ORM mapping.
Diving Deeper: Reflection and JavaBeans
If you’re intrigued by Java Annotations, you might find related concepts like Reflection and JavaBeans interesting. Reflection is a powerful feature in Java that allows an executing Java program to examine or modify its own behavior. JavaBeans, on the other hand, are classes that encapsulate many objects into a single object (the bean).
Further Resources for Mastering Java Annotations
To deepen your understanding of Java Annotations and related concepts, here are some resources you might find helpful:
- IOFlood’s Javadoc Article – Learn how to customize Javadoc output to suit your project’s documentation requirements.
Exploring Javadoc Comments in Java – Master Javadoc commenting for creating comprehensive documentation in Java projects.
Understanding Log4j2 in Java – Learn migration and advanced features of Log4j 2.
Java Annotations Tutorial by Baeldung covers both basic and advanced topics.
Java Reflection Tutorial by Digital Ocean walks you through Java Reflection, a related concept that you might find interesting.
Spring Framework Documentation – The official documentation for the Spring Framework, which makes heavy use of annotations.
By exploring these resources and practicing on your own, you can master the use of Java Annotations and enhance your skills as a Java developer.
Wrapping Up: Mastering Java Annotations
In this comprehensive guide, we’ve delved into the world of Java Annotations, a powerful tool for adding metadata to your Java code.
We began with the basics, learning how to use built-in Java Annotations like @Override
, @Deprecated
, and @SuppressWarnings
. We then ventured into more advanced territory, exploring the creation and usage of custom annotations. Along the way, we tackled common challenges you might face when using Java Annotations, such as incorrect usage and understanding retention policies, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to handle metadata in Java, comparing Java Annotations with other methods like using comments and adopting naming conventions. Here’s a quick comparison of these methods:
Method | Machine-Readable | Flexibility | Complexity |
---|---|---|---|
Java Annotations | Yes | High | Moderate |
Comments | No | Low | Low |
Naming Conventions | No | Moderate | Low |
Whether you’re just starting out with Java Annotations or you’re looking to level up your Java skills, we hope this guide has given you a deeper understanding of Java Annotations and their capabilities.
With its balance of machine-readability, flexibility, and complexity, Java Annotations is a powerful tool for any Java developer. Happy coding!