NPM PG Guide | Unlock PostgreSQL Power in Node.js
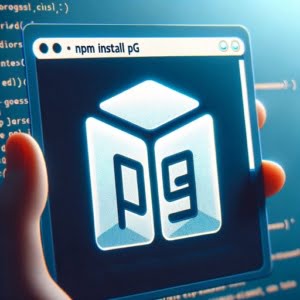
At IOFlood, integrating PostgreSQL databases into our Node.js applications was a common task. Now that we have solved this challenge, we’ve created a guide to help streamline the process. By following these instructions, you’ll confidently navigate PostgreSQL integration, enabling you to build scalable applications with ease.
This guide will walk you through the installation, basic usage, and advanced features of the ‘pg’ package, enabling you to efficiently manage your PostgreSQL database. From setting up your first connection to diving deep into advanced database operations, we’ve got you covered.
Let’s embark on this journey to unlock the full potential of PostgreSQL in your Node.js projects.
TL;DR: How Do I Start Using PostgreSQL in Node.js with ‘npm pg’?
To kickstart your PostgreSQL journey in Node.js, begin by installing the
npm pg
package with the commandnpm install pg
. After installing you mustrequire
thepg
module in your code and then use it to interact with your PostgreSQL database.
Here’s a quick example to get you started:
const { Pool } = require('pg');
const pool = new Pool({
user: 'dbuser',
host: 'database.server.com',
database: 'mydb',
password: 'secretpassword',
port: 5432,
});
# Output:
# Pool object with connection configuration
In this example, we import the Pool
class from the ‘pg’ package and create a new pool instance with our database connection details. This setup is crucial for managing multiple database connections efficiently, ensuring your Node.js app can communicate with your PostgreSQL database seamlessly.
Eager to dive deeper? Continue reading for more detailed instructions and advanced usage tips that will elevate your database management skills.
Table of Contents
Getting Started with npm pg
Installation and Connection Setup
Embarking on your PostgreSQL journey with Node.js begins with the foundational step of installing the ‘npm pg’ package. This package acts as a bridge, facilitating communication between your Node.js application and PostgreSQL database.
To install ‘npm pg’, open your terminal and run:
npm install pg
# Output:
# Adds the pg package to your project dependencies
Once installed, the next step is to establish a connection to your PostgreSQL database. Here’s how you can connect using the Client
class provided by ‘npm pg’:
const { Client } = require('pg');
const client = new Client({
connectionString: 'postgres://dbuser:secretpassword@localhost:5432/mydb'
});
client.connect();
# Output:
# Successfully connected to the database
In this example, we create a new Client
instance and pass our database connection string. The .connect()
method is then called to establish the connection. This step is crucial for executing queries against your database.
Executing Basic Queries
Once connected, you’re ready to execute basic SQL queries. A simple SELECT query can be used to retrieve data from your database:
client.query('SELECT * FROM users;', (err, res) => {
if (err) throw err;
console.log(res.rows);
});
# Output:
# Displays the rows retrieved from the 'users' table
This code snippet demonstrates how to execute a query and handle the response. The query
method takes in your SQL query as a string and a callback function that processes the results. The output shows the rows fetched from the ‘users’ table, illustrating the ease with which you can retrieve data.
Pros and Cons of Using ‘npm pg’
Pros:
– Simplicity: ‘npm pg’ offers a straightforward approach to integrating PostgreSQL with Node.js, making it accessible for beginners.
– Flexibility: It supports a wide range of PostgreSQL features, from basic queries to advanced database operations.
Cons:
– Learning Curve: While simple, mastering ‘npm pg’ and understanding its nuances requires time and practice.
– Connection Management: Without proper handling, managing multiple database connections can become complex, especially in high-load scenarios.
By understanding the basic use of ‘npm pg’, you’re now equipped to start managing your PostgreSQL database within Node.js applications. The journey from here involves exploring more advanced features and best practices, which will further enhance your database management capabilities.
Advanced Database Skills with npm pg
Mastering Connection Pooling
Connection pooling is a crucial technique in managing database connections, especially in applications with high concurrency. It allows multiple clients to share a set of database connections, improving resource utilization and application performance.
To implement connection pooling with ‘npm pg’, you utilize the Pool
class. Here’s an example of setting up a pool and executing a query:
const { Pool } = require('pg');
const pool = new Pool({
user: 'dbuser',
host: 'localhost',
database: 'mydb',
password: 'secretpassword',
port: 5432,
});
pool.query('SELECT NOW()', (err, res) => {
if (err) throw err;
console.log('The current time is:', res.rows[0].now);
});
# Output:
# The current time is: 2023-03-15T12:34:56.789Z
This snippet demonstrates how to create a pool of connections and execute a simple query to retrieve the current time from the database. The pool.query
method automatically handles connection borrowing from the pool and returning it after the query completes. This approach significantly simplifies connection management in your application.
Transaction Management Mastery
Transactions are vital for maintaining data integrity, allowing multiple operations to be treated as a single atomic action. ‘npm pg’ supports robust transaction management, enabling you to execute complex workflows with confidence.
Here’s how you can manage transactions using ‘npm pg’:
(async () => {
const client = await pool.connect();
try {
await client.query('BEGIN');
await client.query('INSERT INTO users(name) VALUES($1)', ['Alice']);
await client.query('COMMIT');
} catch (e) {
await client.query('ROLLBACK');
throw e;
} finally {
client.release();
}
})();
# Output:
# Successfully inserted 'Alice' into the users table
This example showcases a simple transaction where a new user is inserted into the users
table. The transaction is explicitly started with BEGIN
and committed with COMMIT
. In case of an error, the transaction is rolled back to maintain data consistency. The client.release()
ensures the connection is returned to the pool, highlighting the importance of resource management.
Listening to PostgreSQL Notifications
‘npm pg’ also allows you to listen for PostgreSQL notifications, enabling real-time data updates and event-driven programming patterns. Implementing this feature can significantly enhance the interactivity of your Node.js applications.
const client = new Client();
client.connect();
client.on('notification', (msg) => {
console.log('New notification:', msg);
});
client.query('LISTEN my_notification');
# Output:
# New notification: { channel: 'my_notification', payload: 'User Alice updated' }
In this example, the client
subscribes to a PostgreSQL notification channel named ‘my_notification’. When a new notification is published to this channel, the event handler logs the message to the console. This feature is particularly useful for applications requiring real-time data synchronization or notifications.
By exploring these advanced features of ‘npm pg’, you’re not just managing your PostgreSQL database more efficiently; you’re also unlocking new potentials in your Node.js applications. Embrace these techniques to take your database management skills to the next level.
Exploring Alternatives to npm pg
Sequelize: ORM Integration
While ‘npm pg’ offers a direct route to PostgreSQL for Node.js applications, exploring ORM (Object-Relational Mapping) alternatives like Sequelize can provide additional layers of abstraction and convenience. Sequelize simplifies database operations by allowing you to work with objects and promises instead of SQL strings, making it an attractive option for complex projects.
Here’s a basic Sequelize setup for comparison:
const { Sequelize } = require('sequelize');
const sequelize = new Sequelize('postgres://dbuser:secretpassword@localhost:5432/mydb');
(async () => {
try {
await sequelize.authenticate();
console.log('Connection has been established successfully.');
} catch (error) {
console.error('Unable to connect to the database:', error);
}
})();
# Output:
# Connection has been established successfully.
This code demonstrates how to establish a connection to a PostgreSQL database using Sequelize. The sequelize.authenticate()
method is called to test the connection, and a success message is logged upon successful connection. This approach abstracts the direct database connection details, focusing instead on model interactions.
Pros and Cons of Sequelize vs. npm pg
Pros of Sequelize:
– Abstraction: Offers a higher level of abstraction, making database interactions more intuitive.
– ORM Features: Supports model definition, associations, transactions, and more, out of the box.
Cons of Sequelize:
– Performance: The abstraction layer can introduce overhead, potentially affecting performance.
– Complexity: May be overkill for simple projects or those with specific performance requirements.
Making the Right Choice
Deciding between ‘npm pg’ and ORM alternatives like Sequelize depends on your project’s complexity, performance needs, and your comfort level with SQL. ‘npm pg’ provides a lean and direct approach, ideal for those who prefer working closely with SQL and need maximum performance. On the other hand, Sequelize and similar ORMs offer convenience and simplicity at the cost of some performance overhead.
By understanding the strengths and limitations of each approach, you can make an informed decision that best suits your Node.js application’s needs. Whether you choose ‘npm pg’ for its directness and efficiency or Sequelize for its ORM benefits, both paths lead to successful PostgreSQL integration in Node.js.
Troubleshooting Tips with npm pg
Handling Connection Timeouts
One of the frequent challenges developers face when using ‘npm pg’ involves connection timeouts. These occur when a connection to the PostgreSQL database takes too long to establish, often due to network issues or server overload.
To mitigate this, you can configure connection timeout settings in your pool or client setup. Here’s how to set a connection timeout of 3000 milliseconds (3 seconds):
const { Pool } = require('pg');
const pool = new Pool({
connectionString: 'postgres://dbuser:secretpassword@localhost:5432/mydb',
connectionTimeoutMillis: 3000
});
# Output:
# If a connection cannot be established within 3 seconds, it times out
This code snippet demonstrates adding a connectionTimeoutMillis
option to your pool configuration. Adjusting this setting helps prevent your application from hanging indefinitely while trying to connect, enhancing its responsiveness and reliability.
Managing Disconnections Gracefully
Disconnections can disrupt your application’s flow, especially in long-running applications that maintain persistent connections to the database. Implementing reconnection logic is crucial for maintaining uninterrupted database interactions.
Here’s an example of handling disconnections by listening to the ‘error’ event on the client:
const { Client } = require('pg');
const client = new Client();
client.on('error', (err) => {
console.error('Unexpected disconnection:', err);
client.connect();
});
# Output:
# Logs any disconnection errors and attempts to reconnect
In this scenario, the client.on('error', ...)
listener detects disconnections. Upon an error, it logs the issue and attempts to reconnect. This proactive approach ensures your application remains robust against unexpected database disconnections.
Optimizing for Large Datasets
Working with large datasets presents its own set of challenges, such as increased memory usage and slower query responses. To optimize performance, consider using cursors for fetching large amounts of data incrementally.
const { Pool, Cursor } = require('pg');
const pool = new Pool();
const cursor = new Cursor('SELECT * FROM large_table');
const client = await pool.connect();
client.query(cursor);
cursor.read(100, (err, rows) => {
console.log('Fetched rows:', rows);
});
# Output:
# Fetched rows: [Array of rows, up to 100 at a time]
This example introduces the use of a Cursor
to fetch data from a large table in chunks. By specifying how many rows to read at a time, you can manage memory usage more effectively and keep your application responsive.
By addressing these common issues with strategic solutions and workarounds, you can enhance the stability and performance of your Node.js applications using ‘npm pg’. Embracing these practices will not only solve immediate problems but also contribute to a more resilient and efficient application architecture.
PostgreSQL: The Robust Database
Why Choose PostgreSQL?
PostgreSQL stands out in the world of database systems for its reliability, robust feature set, and its open-source nature. As a powerful object-relational database system, it provides extensive data types, including JSON support, and sophisticated features like table inheritance and function overloading. These capabilities make PostgreSQL a versatile choice for a wide range of applications, from small startups to large enterprises.
npm pg: Bridging Node.js and PostgreSQL
Integrating Node.js applications with PostgreSQL is made seamless with the ‘npm pg’ package. This Node.js module serves as a client for interfacing with your PostgreSQL database, offering both a low-level client for executing queries and a high-level pool for managing connections efficiently.
To demonstrate the synergy between Node.js and PostgreSQL using ‘npm pg’, consider this example where we query the version of the PostgreSQL server:
const { Client } = require('pg');
const client = new Client({
connectionString: 'postgres://dbuser:secretpassword@localhost:5432/mydb'
});
client.connect();
client.query('SELECT version();', (err, res) => {
if (err) throw err;
console.log(res.rows[0].version);
});
# Output:
# PostgreSQL 13.3 on x86_64-pc-linux-gnu, compiled by gcc (GCC) 9.3.0, 64-bit
This code snippet illustrates how to establish a connection to a PostgreSQL database and execute a query to retrieve the server version. The output displays the PostgreSQL version, showcasing the ability to interact directly with the database and retrieve valuable information. This example highlights the importance of ‘npm pg’ in facilitating direct communication between Node.js applications and PostgreSQL databases, enabling developers to leverage the full power of PostgreSQL’s features within their applications.
Efficient Database Management with npm pg
Efficient database management is crucial for the performance and scalability of applications. ‘npm pg’ plays a pivotal role in this, offering features like connection pooling and transaction management that help in optimizing database interactions. By harnessing these features, developers can ensure their applications run smoothly, even under high loads, making ‘npm pg’ an invaluable tool for modern web development.
Understanding the fundamentals of PostgreSQL and the capabilities of ‘npm pg’ provides a solid foundation for developers looking to integrate these powerful technologies into their Node.js applications. With ‘npm pg’, harnessing the full potential of PostgreSQL becomes an achievable goal, paving the way for building robust and efficient applications.
Best Practices: Scaling with npm pg
Integrating npm pg with Express.js
When building web applications with Node.js, integrating PostgreSQL using ‘npm pg’ with frameworks like Express.js can significantly streamline development. This combination allows for the efficient handling of database operations alongside server-side logic. Here’s an example of how you can integrate ‘npm pg’ within an Express.js route to fetch data:
const express = require('express');
const { Pool } = require('pg');
const app = express();
const pool = new Pool();
app.get('/users', async (req, res) => {
const { rows } = await pool.query('SELECT * FROM users');
res.json(rows);
});
app.listen(3000, () => console.log('App running on port 3000'));
# Output:
# App running on port 3000
In this snippet, an Express.js application is set up with a route to fetch all users from a PostgreSQL database and return them as JSON. This example illustrates the seamless integration of ‘npm pg’ with Express.js, enabling the creation of dynamic web applications that interact with PostgreSQL databases efficiently.
Mastering Complex Queries and Migrations
As applications grow, handling complex queries and managing database schema migrations become critical. ‘npm pg’ supports these advanced requirements, allowing developers to execute complex SQL queries and manage database changes programmatically. For migrations, tools like node-pg-migrate
can be integrated with ‘npm pg’ to automate and manage schema changes smoothly.
// Example of a complex query
const result = await pool.query('SELECT * FROM users WHERE age > $1', [25]);
console.log('Users older than 25:', result.rows);
# Output:
# Users older than 25: [Array of user objects]
This code demonstrates executing a parameterized query, fetching users older than 25. It showcases ‘npm pg’s ability to handle complex SQL queries efficiently, providing developers with the flexibility to interact with the database according to their application’s needs.
Further Resources for Mastering npm pg
To deepen your understanding of ‘npm pg’ and PostgreSQL, consider exploring the following resources:
- PG NPM Package – A non-blocking PostgreSQL client for Node.js.
Official PostgreSQL Documentation – A comprehensive guide detailing all aspects of PostgreSQL.
Integrating NodeJS with Relational Databases – A step by step guide for integrating Node.js.
By leveraging these resources, you can elevate your skills in database management, optimization, and security. Whether you’re integrating ‘npm pg’ with Express.js, handling complex queries, or managing database migrations, these resources provide a wealth of knowledge to support your journey in building scalable and efficient Node.js applications with PostgreSQL.
Recap: npm pg Usage Guide
In this comprehensive guide, we’ve navigated the essentials of utilizing ‘npm pg’ for PostgreSQL database management within Node.js applications. From the initial setup to leveraging advanced features, ‘npm pg’ serves as a powerful tool for developers seeking efficient database interaction.
We began with the basics, detailing how to install ‘npm pg’ and establish a connection to your PostgreSQL database. We then delved into executing simple queries, highlighting the ease with which developers can interact with their databases.
Moving to more complex scenarios, we explored connection pooling and transaction management, demonstrating ‘npm pg’s capabilities to handle high-demand environments effectively. We also touched on the utility of listening for PostgreSQL notifications, a feature that enriches real-time application responsiveness.
Alternative approaches such as Sequelize were discussed, providing insights into the broader ecosystem of Node.js database management solutions. This comparison allowed us to appreciate ‘npm pg’s direct approach versus the abstraction offered by ORM solutions.
Approach | Directness | Performance | Complexity |
---|---|---|---|
npm pg | High | Excellent | Moderate |
Sequelize (ORM) | Lower | Good | Higher |
Whether you’re just starting out with ‘npm pg’ or seeking to deepen your database management skills, this guide has aimed to equip you with the knowledge to confidently manage PostgreSQL databases in your Node.js applications. The journey doesn’t end here; continuing to explore and experiment with ‘npm pg’ and its advanced features will further enhance your capabilities.
With its robustness and flexibility, ‘npm pg’ stands as a testament to the powerful synergy between Node.js and PostgreSQL. Embrace these tools to build scalable, efficient, and dynamic applications. Happy coding!