Using NPM Moment for Date Handling in JS
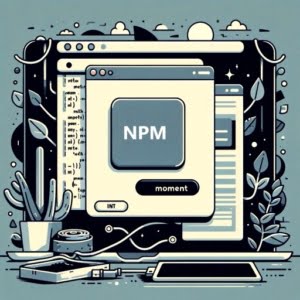
Here at IOFLOOD, we’ve encountered the need to handle dates and times in our projects efficiently, and Moment.js has proven to be a reliable solution. To help others facing similar challenges, we’ve put together a guide on how to use Moment.js with npm. By following these simple instructions, you’ll be able to integrate Moment.js into your projects and manage dates and times effortlessly.
This guide will show you how to leverage npm to add Moment.js to your project, making date manipulation a breeze. With just a few lines of code, you can dramatically simplify the process of working with dates and times in JavaScript.
Let’s dive into the world of Moment.js and discover how it can make your life as a developer much easier.
TL;DR: How Do I Use Moment.js with npm?
To use Moment.js in your project, simply run
npm install moment
. This command integrates Moment.js into your project, allowing you to manipulate dates and times with ease.
Here’s a quick example to get you started:
const moment = require('moment');
console.log(moment().format());
# Output:
# Current date and time in ISO 8601 format.
In this example, we first install Moment.js using npm with the command npm install moment
. Then, by requiring Moment.js in our file, we can use it to display the current date and time in ISO 8601 format. This is just the tip of the iceberg when it comes to what Moment.js can do for you in handling dates and times in JavaScript.
Dive deeper into this guide to explore more advanced features and functionalities of Moment.js that can make your development work a breeze.
Table of Contents
Getting Started with Moment.js
Installing Moment.js with npm
Embarking on your journey with Moment.js begins with a simple installation process. Utilizing npm, the Node Package Manager, you can seamlessly add Moment.js to your project. Here’s how:
npm install moment
This command fetches the Moment.js package and integrates it into your project, making it ready for use. It’s a straightforward step but a crucial one, laying the groundwork for efficient date and time manipulation.
First Steps with Moment.js
Once Moment.js is part of your project, using it to work with dates becomes incredibly intuitive. Let’s start with displaying today’s date in a standard format:
const moment = require('moment');
console.log(moment().format('YYYY-MM-DD'));
# Output:
# 2023-04-05
In this example, after requiring Moment.js, we use the format
method to display the current date in the format ‘YYYY-MM-DD’. This is a basic yet powerful demonstration of Moment.js’s capability to format dates easily. The output shows the current date, which, in this context, is ‘2023-04-05’. This simple operation opens the door to more complex date manipulations and formatting.
Pros and Cons of Moment.js
While Moment.js is renowned for its ease of use and comprehensive documentation, it’s also worth noting its size. Compared to some of the newer libraries available, Moment.js is on the larger side. This might be a consideration for projects where minimizing bundle size is a priority. However, for many developers, the trade-off is worth it given Moment.js’s robust features and straightforward approach to date and time manipulation.
Advanced Moment.js Techniques
Custom Date Formats
One of the most powerful features of Moment.js is its ability to handle custom date formats with ease. Whether you need a specific date format for a user interface or to comply with an API, Moment.js makes this task straightforward. Here’s how you can format a date in a custom way:
const moment = require('moment');
console.log(moment('2023-04-05').format('MMMM Do YYYY, h:mm:ss a'));
# Output:
# April 5th 2023, 12:00:00 am
In this example, we take a specific date ('2023-04-05'
) and format it to a more verbose, user-friendly format. The output is ‘April 5th 2023, 12:00:00 am’, showcasing Moment.js’s flexibility in presenting dates in a manner that suits your application’s needs.
Working with Locales
Moment.js also excels in internationalization, allowing you to work with different locales effortlessly. This feature is invaluable when developing applications that need to support multiple languages. Here’s an example of how to use Moment.js with a different locale:
const moment = require('moment');
moment.locale('fr');
console.log(moment('2023-04-05').format('LLLL'));
# Output:
# mercredi 5 avril 2023 00:00
By setting the locale to French ('fr'
), Moment.js automatically adjusts the date format to match the language preferences, as seen in the output ‘mercredi 5 avril 2023 00:00’. This capability ensures that your application can cater to a global audience with minimal additional effort.
Date Arithmetic
Moment.js isn’t just about formatting; it’s also incredibly useful for performing date arithmetic, such as adding or subtracting time. This functionality can be crucial for features like reminders or expiration dates. Here’s how you can easily add days to a date:
const moment = require('moment');
let futureDate = moment().add(10, 'days').format('YYYY-MM-DD');
console.log(futureDate);
# Output:
# 2023-04-15
In this snippet, we add 10 days to the current date, showcasing Moment.js’s ability to handle date arithmetic seamlessly. The output is ‘2023-04-15’, illustrating how straightforward it is to calculate future or past dates with Moment.js.
These advanced features of Moment.js, from custom formatting and internationalization to date arithmetic, demonstrate the library’s versatility and power in managing dates and times in JavaScript. By mastering these techniques, you can enhance the functionality and user experience of your applications.
Exploring Alternatives to Moment.js
Why Consider Alternatives?
While Moment.js is a powerful tool for date and time manipulation in JavaScript, the modern web development landscape offers alternative libraries that might better suit your project’s needs. Two notable alternatives are date-fns
and Luxon
. These libraries provide similar functionalities but with distinct advantages, particularly in terms of bundle size and modular imports.
Date-fns: Modular Date Utility
date-fns
stands out for its modularity and small bundle size. Unlike Moment.js, date-fns
allows you to import only the functions you need, potentially reducing your application’s bundle size significantly. Here’s how you can use date-fns
to format a date:
const { format } = require('date-fns');
console.log(format(new Date(2023, 3, 5), 'yyyy-MM-dd'));
# Output:
# 2023-04-05
In this example, we import the format
function from date-fns
to format a specific date. The output is ‘2023-04-05’, demonstrating date-fns
‘s ability to handle date formatting with precision. The modular nature of date-fns
means you’re only adding what you need to your project, optimizing performance.
Luxon: A Modern Date Library
Developed by one of the original authors of Moment.js, Luxon
is designed to address some of the limitations of Moment.js, including immutability and better support for internationalization. Here’s an example of using Luxon to handle time zones:
const { DateTime } = require('luxon');
let dt = DateTime.now().setZone('America/New_York').toISO();
console.log(dt);
# Output:
# 2023-04-05T12:00:00.000-04:00
This snippet demonstrates Luxon’s capability to easily manage time zones, a common challenge in date and time manipulation. The DateTime.now().setZone('America/New_York').toISO()
chain sets the current date and time to the New York time zone and formats it in ISO 8601, showcasing Luxon’s intuitive API and powerful features.
Comparing the Libraries
Both date-fns
and Luxon
offer compelling advantages over Moment.js, particularly for projects concerned with bundle size and modern JavaScript features like tree shaking and module imports. However, it’s important to evaluate each library’s features and documentation to determine which best fits your project’s needs. While date-fns
and Luxon
may offer benefits in terms of performance and modern standards, Moment.js still holds value for its ease of use, comprehensive documentation, and wide adoption.
In conclusion, exploring alternatives to Moment.js, such as date-fns
and Luxon
, can provide developers with more tools to efficiently manage dates and times in their projects. Depending on your specific requirements, these libraries might offer the right balance of features, size, and performance.
Optimizing Moment.js Performance
Tackling Timezone Troubles
Timezone management is a common hurdle when working with dates and times in JavaScript, and Moment.js offers robust solutions. However, incorrect usage can lead to unexpected results. For instance, displaying a date in a specific timezone requires careful handling:
const moment = require('moment-timezone');
let nyTime = moment.tz('2023-04-05 12:00', 'America/New_York').format();
console.log(nyTime);
# Output:
# 2023-04-05T12:00:00-04:00
In this code block, we use moment-timezone
to set a specific date and time to New York’s timezone. The output reflects the correct date and time, including the timezone offset (-04:00
). This example underscores the importance of using the appropriate Moment.js extensions, like moment-timezone
, for accurate timezone management.
Performance Considerations
While Moment.js simplifies date and time manipulation, its relatively large size can impact your project’s performance, especially if you’re working on a web application that values fast loading times. To mitigate this, consider lazy loading Moment.js or using it only on pages where date manipulation is critical. Additionally, exploring lighter alternatives or native JavaScript Date objects for simple tasks can optimize performance.
Common Pitfalls and Solutions
- Overuse of Moment.js for Simple Tasks: For basic date formatting or calculations, native JavaScript Date methods may suffice, reducing the need for additional libraries.
Ignoring Timezone Management: Always consider the user’s timezone. Utilize
moment-timezone
for applications with users across different time zones to ensure accurate date and time representation.Bundle Size Concerns: Evaluate whether the full functionality of Moment.js is necessary for your project. In cases where only specific features are used, alternative libraries like
date-fns
orLuxon
might offer more efficient solutions.
By addressing these common issues and considering alternative approaches when appropriate, developers can leverage Moment.js effectively while optimizing their applications’ performance and user experience.
The Challenge of Dates and Times
Why Dates Are Hard
Managing dates and times in programming presents unique challenges. At first glance, it might seem straightforward, but once you delve into the complexities of time zones, daylight saving time adjustments, and different locales, it becomes a daunting task. This complexity is where libraries like Moment.js shine, offering simplified methods to handle these issues.
Consider the challenge of displaying the current time across various time zones. Without a library like Moment.js, this requires intricate calculations and understanding of the nuances of each time zone. Here’s an example using Moment.js to display the current time in two different time zones:
const moment = require('moment-timezone');
let nyTime = moment().tz('America/New_York').format();
let laTime = moment().tz('America/Los_Angeles').format();
console.log('New York:', nyTime);
console.log('Los Angeles:', laTime);
# Output:
# New York: 2023-04-05T15:00:00-04:00
# Los Angeles: 2023-04-05T12:00:00-07:00
In this code block, we use moment-timezone
to easily obtain the current time in both New York and Los Angeles, accounting for their respective time zones. The output clearly shows the three-hour difference between the two cities, illustrating how Moment.js simplifies what would otherwise be a complex calculation.
The Role of Locales
Another layer of complexity in date and time manipulation is handling different locales. Dates are represented differently around the world, not just in format but also in language. Moment.js provides comprehensive support for locales, allowing dates to be formatted according to the local conventions and languages.
Here’s how you can use Moment.js to display a date in a specific locale:
const moment = require('moment');
moment.locale('de');
let germanDate = moment().format('LLLL');
console.log(germanDate);
# Output:
# Mittwoch, 5. April 2023 15:00
By setting the locale to German ('de'
), Moment.js automatically formats the current date and time according to German conventions. The output ‘Mittwoch, 5. April 2023 15:00’ demonstrates Moment.js’s ability to adapt to different cultural contexts, making it an invaluable tool for international applications.
These examples underscore the importance of libraries like Moment.js in addressing the inherent challenges of date and time manipulation in programming. By abstracting the complexities of time zones and locales, Moment.js enables developers to focus on building features that matter, without getting bogged down by the intricacies of date and time calculations.
Practical Usage Cases of Moment.js
Moment.js in Data Processing
Moment.js isn’t just for displaying dates and times; it’s a powerful ally in data processing. When dealing with timestamps, scheduling, or analytics, Moment.js can parse, validate, manipulate, and display dates in any format required. For example, converting a Unix timestamp into a human-readable date is straightforward with Moment.js:
const moment = require('moment');
let readableDate = moment.unix(1617981373).format('YYYY-MM-DD HH:mm:ss');
console.log(readableDate);
# Output:
# 2021-04-09 12:22:53
This code snippet demonstrates how to take a Unix timestamp (1617981373
) and convert it into a more understandable format (2021-04-09 12:22:53
). This capability is crucial for applications that need to present timestamp data in a format that’s accessible to users.
Enhancing User Interfaces with Moment.js
Moment.js also plays a pivotal role in user interface development. Whether you’re building a calendar, scheduling events, or displaying time-sensitive notifications, Moment.js ensures that dates and times are shown in a user-friendly manner. Integrating Moment.js with UI libraries can enhance your application’s interactivity and user experience.
Combining Moment.js with Other Tools
For full-stack development, Moment.js complements a wide array of tools and libraries. Whether you’re using React for your frontend or Node.js for your backend, Moment.js integrates seamlessly, providing consistent date and time manipulation across your stack. This interoperability is key to developing robust, scalable applications.
Further Resources for Mastering Moment.js
To dive deeper into Moment.js and enhance your skills, here are three invaluable resources:
- Moment.js Official Documentation – Comprehensive guides, API references, and examples on Moment.js.
Practical Applications of Moment.js – Insightful exploration of real-world use cases of Moment.js in application development.
Advanced Date Manipulation with Moment.js – Learn about handling timezones and custom formats with Moment.js.
By exploring these resources and incorporating Moment.js into your projects, you can streamline date and time manipulation, enhance your applications, and tackle the challenges of working with dates in modern web development.
Recap: Date and Time Usage in npm
In this comprehensive guide, we’ve explored the ins and outs of using Moment.js, a powerful library for date and time manipulation in JavaScript, through npm. From simplifying the complexities of date and time handling to enhancing your projects with advanced formatting and manipulation capabilities, Moment.js proves to be an invaluable tool for developers.
We began with the basics, introducing how to install Moment.js using npm and showcasing simple yet effective examples of date formatting. This set the foundation for further exploration into the library’s capabilities, including custom date formats, locale support, and date arithmetic, which enable developers to handle a wide range of date and time manipulation tasks with ease.
Venturing into more advanced territory, we discussed the use of Moment.js for handling time zones and performing complex date arithmetic, illustrating the library’s versatility. We also explored alternative libraries like date-fns and Luxon, offering developers options to optimize performance and bundle size according to their project’s specific needs.
Library | Bundle Size | Modularity | Locale Support |
---|---|---|---|
Moment.js | Larger | No | Excellent |
date-fns | Smaller | Yes | Good |
Luxon | Moderate | Yes | Excellent |
Whether you’re just starting out with Moment.js or looking to deepen your understanding of date and time manipulation in JavaScript, this guide has provided you with the knowledge and tools needed to leverage Moment.js effectively in your projects. The comparison above highlights the key differences between Moment.js and its alternatives, helping you make an informed decision based on your project’s requirements.
With its comprehensive features and ease of use, Moment.js remains a go-to choice for many developers. However, understanding the library’s performance implications and considering lighter alternatives can ensure that your applications remain efficient and responsive. Happy coding!