Bash While Loop: Shell Scripting Reference Guide
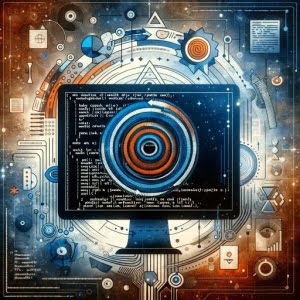
Are you finding it challenging to master ‘while’ loops in Bash scripting? You’re not alone. Many developers find themselves puzzled when it comes to handling ‘while’ loops in Bash, but we’re here to help.
While loops in Bash allow your script to perform tasks multiple times until a certain condition is met. They are a powerful tool for automating repetitive tasks in your scripts, making them extremely popular for a wide range of scripting tasks.
In this guide, we’ll walk you through the process of using while loops in Bash scripting, from the basics to more advanced techniques. We’ll cover everything from writing a simple ‘while’ loop, handling different types of conditions, to dealing with common issues and even troubleshooting.
Let’s dive in and start mastering while loops in Bash!
TL;DR: How Do I Use a While Loop in Bash Scripting?
In Bash scripting, a basic while loop can be written using the following syntax:
while [ condition ]; do commands; done
. This structure allows your script to perform a set of commands repeatedly until a certain condition is met.
Here’s a simple example:
i=0
while [ $i -lt 5 ]; do
echo $i
i=$((i+1))
done
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, we initialize a variable i
to 0. The while loop then continues to echo the value of i
and increment it by 1 until i
is no longer less than 5. As a result, we get a sequence of numbers from 0 to 4 printed out.
This is a basic way to use a while loop in Bash scripting, but there’s much more to learn about handling different conditions and using loops effectively. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Bash While Loop: Basic Use
- Exploring Advanced Bash While Loop Usage
- Alternative Techniques in Bash Scripting
- Troubleshooting Common Issues in Bash While Loops
- Understanding Loops in Programming
- Understanding Bash While Loop Conditions
- The Relevance of Bash While Loops in Real-World Tasks
- Wrapping Up: Mastering Bash While Loops
Bash While Loop: Basic Use
Let’s start with the basics of using a while loop in Bash scripting. The structure of a while loop is simple: It begins with a condition, and as long as that condition is true, the loop will continue to execute the commands inside it.
Here’s a basic example of a while loop that reads a text file line by line:
while IFS= read -r line
do
echo "$line"
done < myfile.txt
# Output:
# [Lines of myfile.txt]
In this example, IFS=
prevents leading/trailing whitespace from being trimmed – read -r line
reads each line, and echo "$line"
prints each line. The loop continues until there are no more lines to read from myfile.txt
.
Advantages of Using While Loops
While loops are incredibly useful for automating repetitive tasks. Instead of writing the same command multiple times, you can write it once inside a loop and let the loop handle the repetition.
Potential Pitfalls
One of the most common pitfalls when using while loops is creating an infinite loop. This happens when the condition for the loop is always true, so the loop never ends. For example:
while true; do
echo 'Infinite loop!'
done
# Output:
# Infinite loop!
# Infinite loop!
# ...
This loop will continue to print ‘Infinite loop!’ indefinitely because the condition true
never changes.
Exploring Advanced Bash While Loop Usage
As your familiarity with Bash scripting grows, you might find yourself needing to use more complex structures, such as nested while loops or loops with multiple conditions. Let’s dive into these advanced uses of while loops in Bash scripting.
Nested While Loops
A nested while loop is a loop within another loop. This can be useful when you want to perform a set of tasks multiple times, and for each time, you need to perform another set of tasks multiple times.
Here’s an example of a nested while loop that prints a multiplication table:
i=1
while [ $i -le 5 ]; do
j=1
while [ $j -le 5 ]; do
echo -n "$((i*j)) "
j=$((j+1))
done
echo
i=$((i+1))
done
# Output:
# 1 2 3 4 5
# 2 4 6 8 10
# 3 6 9 12 15
# 4 8 12 16 20
# 5 10 15 20 25
In this example, the outer loop iterates over the values 1 to 5, and for each iteration, the inner loop also iterates over the values 1 to 5, printing the product of the outer and inner loop variables. This results in a 5×5 multiplication table.
Using Different Conditions
While loops can also use more complex conditions. For example, you can use logical operators like &&
(AND) and ||
(OR) to create more complex conditions.
Here’s an example of a while loop that reads a file line by line and prints only the lines that contain a certain string:
while IFS= read -r line; do
if [[ $line == *'bash'* ]]; then
echo "$line"
fi
done < myfile.txt
# Output:
# [Lines of myfile.txt containing 'bash']
In this example, the loop reads from myfile.txt
, and for each line, it checks if the line contains the string ‘bash’. If it does, it prints the line. This is a simple way to filter the contents of a file based on specific criteria.
Alternative Techniques in Bash Scripting
While loops are incredibly versatile, there are other methods in Bash scripting that can also be used to accomplish repetitive tasks. Two such alternatives are ‘for’ loops and ‘until’ loops. Let’s take a closer look at these alternatives and how they can be used effectively.
For Loops in Bash Scripting
A ‘for’ loop is a control flow statement that allows code to be executed repeatedly. Here’s an example of a ‘for’ loop that prints the numbers from 1 to 5:
for i in {1..5}; do
echo $i
done
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, the ‘for’ loop iterates over the sequence of numbers from 1 to 5, and for each iteration, it prints the current number. This is a simple way to perform a task a certain number of times.
Until Loops in Bash Scripting
An ‘until’ loop is similar to a while loop, but the logic is inverted. An ‘until’ loop will continue to execute as long as the condition is false. Here’s an example of an ‘until’ loop that prints the numbers from 1 to 5:
i=1
until [ $i -gt 5 ]; do
echo $i
i=$((i+1))
done
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, the ‘until’ loop continues to print the value of i
and increment it by 1 until i
is greater than 5. As a result, we get the same sequence of numbers from 1 to 5 as in the previous examples.
While ‘for’ and ‘until’ loops can be used as alternatives to ‘while’ loops, the best choice depends on the specific task and the conditions that need to be met. By understanding these different types of loops, you can choose the most effective method for your scripting needs.
Troubleshooting Common Issues in Bash While Loops
While loops are a powerful tool in Bash scripting, they can sometimes lead to unexpected issues. Let’s discuss some of the common problems you might encounter and how to solve them.
Dealing with Infinite Loops
One of the most common issues when working with while loops is the creation of an infinite loop. This happens when the loop’s condition is always true, causing the loop to run indefinitely. For example:
while true; do
echo 'Infinite loop!'
done
# Output:
# Infinite loop!
# Infinite loop!
# ...
In this case, the loop will keep printing ‘Infinite loop!’ without end, because the condition true
is always true. To prevent this, always ensure that your loop has a condition that will eventually be false.
Handling Unexpected Input
Another common issue arises from unexpected input. For instance, if your loop relies on user input or data from a file, and that input is not in the expected format, it can cause problems.
Here’s an example where a loop expects numeric input but receives a string instead:
i='hello'
while [ $i -le 5 ]; do
echo $i
i=$((i+1))
done
# Output:
# bash: [: hello: integer expression expected
In this case, the loop fails because it’s trying to compare a string ('hello'
) to a number (5
). To prevent this, you can add error checking to your loops to handle unexpected input gracefully.
Using Break and Continue
Sometimes, you might want to stop a loop before its condition becomes false or skip an iteration under certain circumstances. For this, Bash provides the break
and continue
statements.
The break
statement exits the loop prematurely. For example:
i=1
while [ $i -le 5 ]; do
if [ $i -eq 3 ]; then
break
fi
echo $i
i=$((i+1))
done
# Output:
# 1
# 2
In this case, the loop breaks when i
equals 3
, so it only prints 1
and 2
.
The continue
statement skips the rest of the current iteration and proceeds to the next one. For example:
i=0
while [ $i -lt 5 ]; do
i=$((i+1))
if [ $i -eq 3 ]; then
continue
fi
echo $i
done
# Output:
# 1
# 2
# 4
# 5
In this case, when i
equals 3
, the loop skips the echo
command and proceeds to the next iteration, so 3
is not printed.
By understanding these common issues and how to handle them, you can write more robust and reliable Bash scripts.
Understanding Loops in Programming
Before we delve deeper into the specifics of while loops in Bash scripting, let’s take a step back and understand the fundamental concept of loops in programming.
A loop is a control flow structure that allows a piece of code to be executed repeatedly. The code, which we’ll call the loop body, is executed as long as a certain condition is met.
Here’s a simple loop in pseudo-code:
while condition is true
execute loop body
end loop
In this example, as long as the condition remains true, the loop body will be executed. Once the condition becomes false, the loop ends, and the program continues with whatever code comes after the loop.
Understanding Bash While Loop Conditions
In Bash scripting, conditions are expressed using test constructs like [ expression ]
or [[ expression ]]
.
Here’s a simple Bash while loop that prints the numbers from 1 to 5:
i=1
while [ $i -le 5 ]; do
echo $i
i=$((i+1))
done
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, the condition is $i -le 5
, which checks if the variable i
is less than or equal to 5
. The loop body, which is the code between do
and done
, is executed as long as this condition is true. Inside the loop body, we print the current value of i
and then increment i
by 1.
By understanding the basics of loops and conditions in Bash scripting, you can create powerful scripts that automate repetitive tasks and make your life as a developer easier.
The Relevance of Bash While Loops in Real-World Tasks
In the world of scripting and automation, Bash while loops are an indispensable tool. They are widely used in system administration tasks, data processing, web scraping, and even in generating reports. The ability to repeat a task until a certain condition is met is undeniably powerful and can greatly enhance the efficiency of your scripts.
Exploring Related Concepts
While mastering while loops is a significant step, it’s also beneficial to explore related concepts in Bash scripting. Conditional statements, for instance, are often used in conjunction with loops to control the flow of the script based on certain conditions. Functions, on the other hand, allow you to encapsulate a piece of code that performs a specific task and reuse it throughout your script.
Here’s an example of a script that uses a while loop, a conditional statement, and a function to print the first ten Fibonacci numbers:
fibonacci() {
a=0
b=1
while [ $a -le $1 ]; do
echo $a
sum=$((a+b))
a=$b
b=$sum
done
}
fibonacci 100
# Output:
# 0
# 1
# 1
# 2
# 3
# 5
# 8
# 13
# 21
# 34
# 55
# 89
In this script, the fibonacci
function calculates the Fibonacci sequence up to a given number. The while loop continues as long as a
is less than or equal to the input number, and the variables a
and b
are updated in each iteration to hold the current and next numbers in the sequence, respectively.
Further Resources for Mastering Bash While Loops
For a deeper understanding of while loops and Bash scripting in general, check out the following resources:
- GNU Bash Manual: The official manual for Bash, providing comprehensive documentation on all aspects of the shell, including while loops.
Bash Academy: An online platform that offers interactive lessons on Bash scripting, including the use of loops.
Linux Command: A website dedicated to teaching Linux commands and shell scripting, with a section on control structures like while loops.
Wrapping Up: Mastering Bash While Loops
In this in-depth guide, we’ve journeyed through the world of Bash scripting, with a particular focus on while loops, a powerful tool for automating repetitive tasks.
We started with the basics of while loops, learning how to write a simple loop and understanding its structure and flow. We then ventured into more complex territory, exploring nested loops and different conditions, providing you with the knowledge to handle more advanced scripting tasks.
Along the way, we tackled common challenges you might face when using while loops, such as infinite loops and unexpected input, providing you with solutions and workarounds for each issue. We also discussed alternative approaches to repetitive tasks, comparing while loops with ‘for’ and ‘until’ loops. Here’s a quick comparison of these methods:
Method | Flexibility | Ease of Use | Best Used For |
---|---|---|---|
While Loops | High | Moderate | Complex conditions and tasks |
For Loops | Moderate | High | Fixed number of iterations |
Until Loops | High | Moderate | Conditions that become false |
Whether you’re just starting out with Bash scripting or looking to enhance your skills, we hope this guide has given you a deeper understanding of while loops and their capabilities in Bash scripting.
The ability to automate repetitive tasks using while loops is a powerful tool in any developer’s arsenal. Now, you’re well equipped to harness that power in your Bash scripts. Happy scripting!