Using Java Reflection: From Basics to Advanced
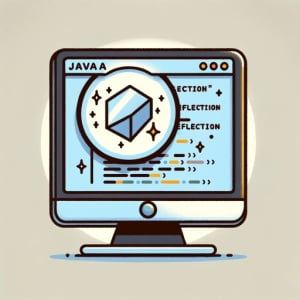
Are you finding it challenging to navigate the world of Java Reflection? You’re not alone. Many developers find themselves puzzled when it comes to understanding and implementing Java Reflection, but we’re here to help.
Think of Java Reflection as a mirror – a mirror that allows your Java code to examine itself, opening up a world of dynamic programming. It’s a powerful tool that can provide a versatile and handy solution for various tasks.
In this guide, we’ll walk you through the process of mastering Java Reflection, from the basics to more advanced techniques. We’ll cover everything from inspecting classes, interfaces, fields, and methods at runtime, to using Java Reflection for dynamic loading, examining class modifiers and types, and manipulating fields and methods.
So, let’s get started and start mastering Java Reflection!
TL;DR: What is Java Reflection?
Java Reflection is a powerful feature in Java that allows a program to inspect and manipulate its own structure and behavior at runtime. Can be utilized with single lines of code such as:
Class c = String.class;
However there are other methods available for more in-depth performance. It’s like giving your code a mirror to look at itself, enabling dynamic programming.
Here’s a basic example:
Class c = String.class;
System.out.println(c.getName());
// Output:
// 'java.lang.String'
In this example, we’re using Java Reflection to get the name of the class String
. We first get a Class
object representing the String
class, and then we call the getName()
method on this object, which returns the name of the class as a string.
This is just a basic introduction to Java Reflection. There’s a lot more to learn, including how to use it for more advanced tasks like inspecting fields and methods, dynamic loading, and manipulating fields and methods. Continue reading for a deeper understanding and more advanced usage scenarios.
Table of Contents
- Getting Started with Java Reflection
- Exploring Advanced Java Reflection Techniques
- Exploring Alternatives to Java Reflection
- Troubleshooting Common Java Reflection Issues
- Unpacking the Concept of Introspection
- Java Reflection in Larger Projects
- Complementary Concepts to Java Reflection
- Wrapping Up: Mastering Java Reflection
Getting Started with Java Reflection
Java Reflection is a powerful tool that allows you to inspect classes, interfaces, fields, and methods at runtime. Let’s dive into how you can use it, even as a beginner.
Inspecting Classes with Java Reflection
To inspect a class, you first need to get a Class
object representing the class you want to inspect. You can do this in three ways:
- Calling
getClass()
on an object. - Using the
.class
syntax on a class name. - Using the
Class.forName()
method with the fully qualified name of the class.
Here’s an example of each method:
// Using getClass()
Object object = new String("Hello World");
Class c1 = object.getClass();
// Using .class syntax
Class c2 = String.class;
// Using Class.forName()
Class c3 = Class.forName("java.lang.String");
System.out.println(c1.getName());
System.out.println(c2.getName());
System.out.println(c3.getName());
// Output:
// java.lang.String
// java.lang.String
// java.lang.String
In this code block, we’re inspecting the String
class using three different methods. Each method returns a Class
object representing the String
class, and we then print the name of the class using the getName()
method.
Inspecting Interfaces with Java Reflection
You can also use Java Reflection to inspect the interfaces implemented by a class. Here’s an example:
Class c = String.class;
Class[] interfaces = c.getInterfaces();
for (Class i : interfaces) {
System.out.println(i.getName());
}
// Output:
// java.io.Serializable
// java.lang.Comparable
// java.lang.CharSequence
In this example, we’re getting an array of Class
objects representing the interfaces implemented by the String
class, and then we’re printing the names of these interfaces.
Inspecting Fields and Methods with Java Reflection
Java Reflection allows you to inspect the fields and methods of a class as well. Here’s an example:
Class c = String.class;
Field[] fields = c.getDeclaredFields();
for (Field field : fields) {
System.out.println(field.getName());
}
Method[] methods = c.getDeclaredMethods();
for (Method method : methods) {
System.out.println(method.getName());
}
// Output:
// (A list of fields and methods of the String class)
In this example, we’re getting arrays of Field
and Method
objects representing the fields and methods of the String
class, and then we’re printing their names.
Using Java Reflection to inspect classes, interfaces, fields, and methods can be very useful, but it also comes with some potential pitfalls. For example, it can lead to security issues if used improperly, and it can make your code more complex and harder to understand. Therefore, it’s important to use it wisely and understand its implications.
Exploring Advanced Java Reflection Techniques
As you become more comfortable with Java Reflection, you can start to explore its more advanced features. These include dynamic loading, examining class modifiers and types, and manipulating fields and methods.
Dynamic Loading with Java Reflection
Dynamic loading allows you to load a class at runtime. This can be useful when you don’t know which class you’ll need to use at compile time. Here’s an example:
Class c = Class.forName("java.util.ArrayList");
Object object = c.newInstance();
System.out.println(object instanceof ArrayList);
// Output:
// true
In this example, we’re dynamically loading the ArrayList
class and creating a new instance of it. We then check whether this object is an instance of ArrayList
, which it is, so the output is true
.
Examining Class Modifiers and Types with Java Reflection
Java Reflection allows you to examine the modifiers and types of a class. Here’s an example:
Class c = String.class;
int modifiers = c.getModifiers();
System.out.println(Modifier.isFinal(modifiers));
System.out.println(Modifier.isPublic(modifiers));
// Output:
// true
// true
In this example, we’re checking whether the String
class is final and public, which it is, so the output is true
for both checks.
Manipulating Fields and Methods with Java Reflection
You can also use Java Reflection to manipulate the fields and methods of a class. Here’s an example:
Class c = String.class;
Field field = c.getDeclaredField("value");
field.setAccessible(true);
String str = "Hello World";
char[] value = (char[]) field.get(str);
System.out.println(Arrays.toString(value));
// Output:
// [H, e, l, l, o, , W, o, r, l, d]
In this example, we’re accessing the private value
field of the String
class, which holds the characters of the string. We then get the value of this field for the string “Hello World”, which is an array of characters, and print this array.
While these advanced features of Java Reflection can be very powerful, they also come with some drawbacks. For example, they can lead to security issues if used improperly, and they can make your code more complex and harder to understand. Therefore, it’s important to use them wisely and understand their implications.
Exploring Alternatives to Java Reflection
While Java Reflection is a powerful tool, there are other related features and libraries that can accomplish similar tasks. Two of these are Method Handles and the Java Native Interface (JNI).
Leveraging Method Handles
Method Handles, introduced in Java 7, provide a way to directly execute Java methods, similar to Reflection but with better performance and more flexibility. Here’s an example:
MethodHandles.Lookup lookup = MethodHandles.lookup();
MethodHandle mh = lookup.findVirtual(String.class, "substring", MethodType.methodType(String.class, int.class));
String result = (String) mh.invoke("Hello World", 6);
System.out.println(result);
// Output:
// World
In this example, we’re using a Method Handle to call the substring
method on a String
. The output is ‘World’, the substring of ‘Hello World’ starting from index 6.
Utilizing Java Native Interface (JNI)
The Java Native Interface (JNI) is a framework that allows Java code running in a Java Virtual Machine (JVM) to call and be called by native applications and libraries written in other languages, such as C, C++, and assembly. Although it’s a more advanced and complex tool than Java Reflection, it can be very powerful when used properly.
// Java code
public class HelloWorld {
public native void print();
static {
System.loadLibrary("HelloWorld");
}
public static void main(String[] args) {
new HelloWorld().print();
}
}
// C++ code
#include <jni.h>
#include <iostream>
#include "HelloWorld.h"
JNIEXPORT void JNICALL Java_HelloWorld_print(JNIEnv*, jobject) {
std::cout << "Hello, World!
";
}
// Output:
// Hello, World!
In this example, we’re using JNI to call a C++ function from Java. The C++ function prints ‘Hello, World!’.
While these alternative approaches can be useful, they also come with their own benefits and drawbacks. Method Handles can be faster and more flexible than Java Reflection, but they’re also more complex and harder to use. JNI can be very powerful, but it also introduces the complexity of dealing with native code and the potential for serious errors. Therefore, it’s important to carefully consider which tool is the best fit for your specific needs.
Troubleshooting Common Java Reflection Issues
While Java Reflection is a powerful tool, it’s not without its challenges. Here, we’ll discuss some common errors or obstacles you might encounter when using Java Reflection, along with their solutions. We’ll also provide tips for best practices and optimization.
Dealing with ClassNotFoundException
One common error you might encounter is ClassNotFoundException
. This occurs when the class you’re trying to load with Class.forName()
doesn’t exist.
try {
Class c = Class.forName("NonExistentClass");
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
// Output:
// java.lang.ClassNotFoundException: NonExistentClass
In this example, we’re trying to load a non-existent class, which throws a ClassNotFoundException
. The solution is to ensure that the class you’re trying to load actually exists and that its name is spelled correctly.
Handling IllegalAccessException
Another common error is IllegalAccessException
, which occurs when you’re trying to access a private field or method.
Class c = String.class;
Field field = c.getDeclaredField("value");
char[] value = (char[]) field.get("Hello World");
// Output:
// java.lang.IllegalAccessException: Class Example can not access a member of class java.lang.String with modifiers "private final"
In this example, we’re trying to access the private value
field of the String
class, which throws an IllegalAccessException
. The solution is to call field.setAccessible(true)
before trying to access the field.
Best Practices and Optimization
When using Java Reflection, it’s important to keep in mind that it can be slower than direct code and can lead to security issues if used improperly. Therefore, it’s best to use it sparingly and only when necessary.
Also, when using Java Reflection to call methods, it’s often faster to use Method.invoke()
rather than Class.newInstance()
and MethodHandle.invokeExact()
. This is because Method.invoke()
performs some optimizations that the other two methods don’t.
Unpacking the Concept of Introspection
Introspection, in the context of Java, refers to the ability of a program to examine the type or properties of an object at runtime. This is a fundamental concept that underlies Java Reflection.
Introspection and Java Reflection
Java Reflection is a form of introspection. It allows a program to inspect its own structure, including classes, interfaces, fields, and methods. This capability opens up a range of possibilities for dynamic programming, as it allows a program to adapt its behavior based on its structure.
Class c = String.class;
System.out.println("Class Name: " + c.getName());
System.out.println("Package Name: " + c.getPackage().getName());
// Output:
// Class Name: java.lang.String
// Package Name: java.lang
In this code block, we’re using Java Reflection to introspect the String
class. We retrieve and print the class name and package name, demonstrating how a program can examine its own structure.
Beyond Java Reflection
While Java Reflection is a powerful tool for introspection, it’s not the only option. Other features, such as the JavaBeans API, also provide introspection capabilities. These features can be used to inspect and manipulate JavaBeans, which are reusable software components for Java.
BeanInfo info = Introspector.getBeanInfo(MyJavaBean.class);
PropertyDescriptor[] props = info.getPropertyDescriptors();
for (PropertyDescriptor pd : props) {
System.out.println(pd.getName());
}
// Output:
// (List of property names of MyJavaBean)
In this code block, we’re using the JavaBeans API to introspect a JavaBean. We retrieve and print the names of the bean’s properties, demonstrating another form of introspection in Java.
Understanding introspection and its relation to Java Reflection is key to fully leveraging the power of dynamic programming in Java.
Java Reflection in Larger Projects
Java Reflection is not just a tool for small-scale tasks. It also plays a crucial role in larger projects and frameworks. For instance, many Java frameworks like Spring and Hibernate use Reflection extensively for tasks like dependency injection and object-relational mapping.
ApplicationContext context = new ClassPathXmlApplicationContext("beans.xml");
MyBean bean = (MyBean) context.getBean("myBean");
bean.doSomething();
// Output:
// (Depends on the implementation of MyBean's doSomething method)
In this code block, we’re using the Spring framework to load a bean from an XML configuration file and call a method on it. This is made possible by Java Reflection, which Spring uses to instantiate the bean and call its methods.
Complementary Concepts to Java Reflection
When working with Java Reflection, you might also find yourself dealing with related features or concepts. For example, annotations, which provide metadata about your code, are often used in conjunction with Reflection. The Java Reflection API includes methods for reading annotations, which can be useful for tasks like configuration or data validation.
Class c = MyAnnotatedClass.class;
Annotation[] annotations = c.getAnnotations();
for (Annotation annotation : annotations) {
System.out.println(annotation);
}
// Output:
// (List of annotations of MyAnnotatedClass)
In this code block, we’re using Java Reflection to get and print the annotations of a class. This demonstrates how Reflection can be used to read metadata about your code.
Further Resources for Mastering Java Reflection
To deepen your understanding of Java Reflection, consider exploring these additional resources:
- Best Practices for Testing Java Code – Learn about integration testing and how to test Java components.
JMeter Performance Testing – Discover JMeter’s features for scripting test scenarios and generating test reports.
JUnit Testing in Java – Explore the benefits of JUnit for ensuring the correctness of Java code through testing.
Oracle’s Java Tutorials: The Reflection API details the use and benefits of the Reflection API.
Baeldung’s Guide to Java Reflection sheds light on the various aspects of Java reflection.
JournalDev’s Java Reflection Tutorial is a practical tutorial on Java reflection, with coded examples.
These resources provide more in-depth information about Java Reflection, including detailed explanations, examples, and tutorials.
Wrapping Up: Mastering Java Reflection
In this comprehensive guide, we’ve explored the depths of Java Reflection, a powerful feature in Java that allows a program to inspect and manipulate its own structure and behavior at runtime.
We began with the basics, learning how to use Java Reflection to inspect classes, interfaces, fields, and methods at runtime. We then dug deeper, exploring advanced techniques such as dynamic loading, examining class modifiers and types, and manipulating fields and methods. Along the way, we tackled common issues that you might encounter when using Java Reflection, offering solutions to help you overcome these challenges.
We also took a look at alternative approaches to Java Reflection, like Method Handles and the Java Native Interface (JNI), providing you with a broader perspective of the tools available for introspection in Java. Here’s a quick comparison of these methods:
Method | Flexibility | Complexity | Use Case |
---|---|---|---|
Java Reflection | High | Moderate | Inspecting and manipulating structure at runtime |
Method Handles | High | High | Direct method execution |
Java Native Interface (JNI) | Very High | Very High | Interacting with native applications and libraries |
Whether you’re just starting out with Java Reflection or you’re looking to level up your introspection skills, we hope this guide has given you a deeper understanding of Java Reflection and its capabilities.
Java Reflection, with its ability to inspect and manipulate a program’s structure at runtime, is a powerful tool for dynamic programming in Java. Now, you’re well equipped to harness its power. Happy coding!