Java.lang.NoClassDefFoundError: Causes and Solutions
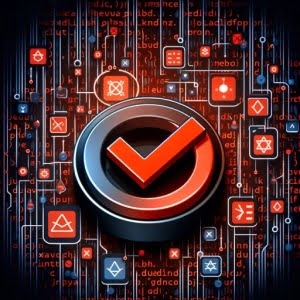
Ever stumbled upon a java.lang.NoClassDefFoundError in your Java program and felt lost? You’re not alone. Many developers find themselves in a similar situation, but consider this error as a missing piece in a puzzle – your program can’t find a class it needs.
Think of java.lang.NoClassDefFoundError as a sign that your Java program is trying to utilize a class that it can’t find. This could be due to a variety of reasons, such as issues with your classpath or problems with static initializers.
In this guide, we’ll help you understand why this error occurs and how to resolve it. We’ll delve into the causes of java.lang.NoClassDefFoundError, discuss solutions, and provide practical examples to help you navigate this error. So, let’s dive in and start mastering java.lang.NoClassDefFoundError!
TL;DR: What is java.lang.NoClassDefFoundError and How Do I Fix It?
Java.lang.NoClassDefFoundError is an error in Java that occurs when the Java Virtual Machine (JVM) or ClassLoader instance tries to load a class definition and the class could not be found. The simple solution is to make sure your classpath includes the class or its containing JAR:
java -cp .;path/to/missing/class HelloWorld
Here’s a simple example of how you might encounter this error:
public class HelloWorld {
public static void main(String[] args) {
MissingClass obj = new MissingClass();
obj.printMessage();
}
}
# Output:
# Error: Could not find or load main class HelloWorld
# Caused by: java.lang.NoClassDefFoundError: MissingClass
In this example, we’re trying to create an instance of MissingClass
and call the printMessage
method on it. However, MissingClass
doesn’t exist in our classpath, leading to a java.lang.NoClassDefFoundError
.
This is just a basic scenario where java.lang.NoClassDefFoundError can occur. There’s much more to learn about this error, its causes, and solutions. Continue reading for more detailed information and advanced scenarios.
Table of Contents
- Understanding java.lang.NoClassDefFoundError: A Beginner’s Guide
- Diving Deeper: Complex Scenarios with java.lang.NoClassDefFoundError
- Proactive Measures: Preventing java.lang.NoClassDefFoundError
- Navigating Common Issues with java.lang.NoClassDefFoundError
- The Backbone: Java Class Loading and Classpath
- The Bigger Picture: java.lang.NoClassDefFoundError in Large Projects
- Wrapping Up: Mastering java.lang.NoClassDefFoundError
Understanding java.lang.NoClassDefFoundError: A Beginner’s Guide
java.lang.NoClassDefFoundError is a runtime error in Java. It occurs when the Java Virtual Machine (JVM) or a ClassLoader instance tries to load the definition of a class and doesn’t find the class. Let’s illustrate this with a simple code example.
public class HelloWorld {
public static void main(String[] args) {
MissingClass obj = new MissingClass();
obj.printMessage();
}
}
# Output:
# Error: Could not find or load main class HelloWorld
# Caused by: java.lang.NoClassDefFoundError: MissingClass
In the above code, we’re trying to use a class named MissingClass
. But MissingClass
is not available in our classpath. As a result, when we try to run the HelloWorld
program, JVM throws a java.lang.NoClassDefFoundError
indicating that it could not find the MissingClass
.
How to Resolve java.lang.NoClassDefFoundError
The most straightforward way to resolve this error is to make sure that the class or JAR that contains the class is included in your classpath. Here’s how you can do it:
- Identify the missing class: In our example, the missing class is
MissingClass
. Find the location of the missing class: You need to find where the missing class is located in your file system. It could be in a directory or inside a JAR file.
Add the missing class to your classpath: Once you’ve located the missing class, you can add it to your classpath using the
-cp
or-classpath
option when running your program from the command line. For example:
java -cp .;path/to/missing/class HelloWorld
In this command, .;path/to/missing/class
is the classpath. It tells JVM where to look for classes. The .
indicates that JVM should look in the current directory, and path/to/missing/class
is the path to the MissingClass
.
After adding the missing class to the classpath, you should be able to run your program without encountering the java.lang.NoClassDefFoundError
.
Diving Deeper: Complex Scenarios with java.lang.NoClassDefFoundError
As you gain more experience with Java, you’ll find that java.lang.NoClassDefFoundError can occur in more complex scenarios. Let’s explore a couple of these situations.
Scenario 1: Issues with Static Initializers
One common scenario involves issues with static initializers. A static initializer is a block of code in a class that is executed when the class is loaded into memory. If a static initializer throws an exception the first time JVM tries to load the class, JVM will record the error and throw a java.lang.NoClassDefFoundError the next time it tries to load the class.
Here’s an example of how this can happen:
public class HelloWorld {
static {
int x = 1 / 0;
}
public static void main(String[] args) {
HelloWorld obj = new HelloWorld();
System.out.println("Hello, World!");
}
}
# Output:
# Exception in thread "main" java.lang.ExceptionInInitializerError
# Caused by: java.lang.ArithmeticException: / by zero
The HelloWorld
class has a static initializer that throws an ArithmeticException
. When we try to create an instance of HelloWorld
, JVM tries to load the HelloWorld
class and execute the static initializer, which throws the ArithmeticException
. As a result, JVM throws an ExceptionInInitializerError
and records that the HelloWorld
class could not be initialized. If we try to create another instance of HelloWorld
in the same run of the program, JVM will throw a java.lang.NoClassDefFoundError
.
Scenario 2: Classpath Problems in a Web Application Environment
Another common scenario involves classpath problems in a web application environment. In a web application, classes can be loaded by different class loaders. If a class loaded by one class loader tries to access a class loaded by a different class loader, and the second class loader is no longer available, a java.lang.NoClassDefFoundError can occur.
This scenario is more complex and beyond the scope of this guide, but it’s important to be aware of it. If you’re developing web applications in Java, make sure to understand how class loading works in your web application server and how to configure the classpath for your web application.
Proactive Measures: Preventing java.lang.NoClassDefFoundError
As the old saying goes, ‘prevention is better than cure’. This holds true for java.lang.NoClassDefFoundError as well. By adopting certain best practices, you can prevent this error from happening in the first place.
Classpath Management
Classpath issues are the most common cause of java.lang.NoClassDefFoundError. Therefore, managing your classpath effectively is crucial. Here are a few tips:
- Keep your classpath clean: Avoid including unnecessary directories or JAR files in your classpath. This not only helps prevent classpath issues, but also improves the performance of class loading.
Use relative paths: Whenever possible, use relative paths instead of absolute paths in your classpath. This makes your setup more portable and less prone to issues caused by changes in the file system.
Check your classpath before running your program: Before you run your program, check your classpath to make sure it includes all necessary directories and JAR files. A simple way to do this is to print the classpath in your program or script.
Using Build Tools
Build tools like Maven or Gradle can help manage your classpath and prevent java.lang.NoClassDefFoundError. These tools automatically handle dependencies and classpaths, reducing the chances of classpath issues.
Here’s an example of how to specify dependencies in a Maven pom.xml
file:
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
</dependencies>
In this example, we’re specifying a dependency on JUnit 4.12. Maven will automatically download the JUnit JAR file and include it in the classpath when running tests.
By following these best practices, you can greatly reduce the likelihood of encountering java.lang.NoClassDefFoundError in your Java programs.
While resolving java.lang.NoClassDefFoundError, you may encounter a few common issues. In this section, we’ll discuss these potential challenges and provide solutions to help you navigate them smoothly.
Case Sensitivity
Java is case sensitive, which means MyClass
and myclass
are considered different classes. If the case of the class name in your code doesn’t match the case of the class file, you’ll encounter a java.lang.NoClassDefFoundError
.
public class MyClass {
// ...
}
public class Test {
public static void main(String[] args) {
myclass obj = new myclass();
}
}
# Output:
# Error: Could not find or load main class Test
# Caused by: java.lang.NoClassDefFoundError: myclass
In this example, we’ve defined a class MyClass
but tried to use it as myclass
in the Test
class. As a result, we get a java.lang.NoClassDefFoundError
. The solution is to ensure the case of the class name matches exactly in your code and the class file.
Incorrect Classpath
As we’ve discussed, an incorrect classpath is a common cause of java.lang.NoClassDefFoundError
. If you’ve checked the classpath and the problem persists, try the following:
- Check for typos: Make sure the classpath doesn’t contain any typos or incorrect paths.
Use the -verbose:class JVM option: This option prints a message each time a class is loaded. This can help you identify what’s being loaded and what’s not.
java -verbose:class -cp . HelloWorld
- Check for classpath issues in third-party libraries: If you’re using third-party libraries, make sure they’re included in the classpath and they don’t have any classpath issues of their own.
By being aware of these common issues and knowing how to troubleshoot them, you can resolve java.lang.NoClassDefFoundError
more effectively.
The Backbone: Java Class Loading and Classpath
To fully understand the java.lang.NoClassDefFoundError, it’s essential to grasp two fundamental concepts in Java: the class loading mechanism and the classpath.
Java Class Loading Mechanism
In Java, classes are dynamically loaded into memory as required by the program. This is handled by a component of the JVM called the ClassLoader. The ClassLoader reads the binary data of a class file and creates a java.lang.Class
object from that data.
Here’s a simple example:
public class Test {
public static void main(String[] args) throws ClassNotFoundException {
Class<?> cls = Class.forName("java.util.ArrayList");
System.out.println("Class loaded: " + cls.getName());
}
}
# Output:
# Class loaded: java.util.ArrayList
In this example, we’re using the Class.forName
method to load the java.util.ArrayList
class. The Class.forName
method uses the ClassLoader to load the class.
The Role of Classpath
The classpath is a parameter that tells the JVM where to look for user-defined classes and packages. It can be set either when launching the JVM using the -cp
or -classpath
option, or by setting the CLASSPATH
environment variable.
When the JVM starts, it initializes a ClassLoader instance that uses the classpath to find and load classes. If a class is not found in the classpath, a java.lang.NoClassDefFoundError
is thrown. This is why an incorrect classpath is one of the most common causes of java.lang.NoClassDefFoundError
.
In conclusion, the Java class loading mechanism and the classpath are closely related to java.lang.NoClassDefFoundError
. Understanding these concepts is key to diagnosing and resolving this error.
The Bigger Picture: java.lang.NoClassDefFoundError in Large Projects
Understanding the java.lang.NoClassDefFoundError is not just about fixing an error. It’s about mastering the fundamentals of Java, which are crucial in larger projects. The more you understand about the Java Virtual Machine (JVM), class loaders, and classpath, the better you’ll be at diagnosing and resolving issues that arise in complex applications.
Exploring Java Class Loaders
Java class loaders play a vital role in the JVM. They’re responsible for finding and loading class files at runtime. Understanding how class loaders work can help you navigate issues related to class loading, such as java.lang.NoClassDefFoundError. You can delve deeper into Java class loaders in the Java documentation.
Unraveling JVM Internals
The JVM is the engine that drives Java applications. It’s responsible for executing Java bytecode and provides features like garbage collection and multithreading. To truly master java.lang.NoClassDefFoundError and related issues, it’s beneficial to understand the internals of the JVM. The JVM specification is a great resource for this.
Further Resources for Mastering java.lang.NoClassDefFoundError
To continue your journey in mastering java.lang.NoClassDefFoundError and related concepts, here are a few resources that you might find helpful:
- Strengthening Your Error Handling Skills in Java – Master Java error resolution techniques.
Java Assert Statement: Basics – Understand the assert statement in Java for testing assumptions.
Fixing “No Main Manifest Attribute” Error – Understand common causes and solutions.
Oracle’s Java Tutorials cover a wide range of topics in Java, including exceptions and the classpath.
Jenkov’s Java Classloader Tutorial provides a deep dive into the Java class loader.
Baeldung’s Java NoClassDefFoundError Explanation and Solutions gives a detailed explanations and solutions to the java.lang.NoClassDefFoundError in Java.
Remember, mastering a programming language and its intricacies is a journey. Keep exploring, learning, and coding!
Wrapping Up: Mastering java.lang.NoClassDefFoundError
In this comprehensive guide, we’ve delved into the world of java.lang.NoClassDefFoundError, a common but often misunderstood error in Java.
We began with the basics, explaining what java.lang.NoClassDefFoundError is and why it occurs. We then dove into practical examples, demonstrating how to resolve this error in both simple and more complex scenarios. We provided code examples and step-by-step solutions to help you understand and fix this error.
From there, we ventured into the realm of prevention. We discussed best practices for managing your classpath and using build tools like Maven or Gradle to prevent java.lang.NoClassDefFoundError from happening in the first place. We also tackled common issues you might encounter while resolving this error and provided tips for troubleshooting.
Finally, we took a step back to look at the bigger picture. We explored the fundamentals of the Java class loading mechanism and the classpath, and discussed the importance of understanding these concepts in larger projects. We also pointed you to further resources for mastering java.lang.NoClassDefFoundError and related concepts.
In conclusion, java.lang.NoClassDefFoundError is more than just an error. It’s a window into the workings of the Java Virtual Machine and the Java class loading mechanism. Understanding this error and knowing how to resolve it equips you with a deeper understanding of Java, preparing you to tackle more complex challenges in your Java journey. Happy coding!