Java Array Length: How To Get the Array Size in Java
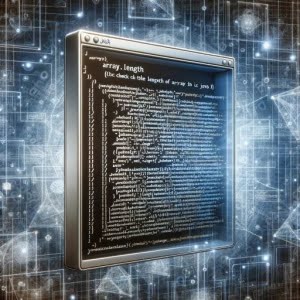
Are you finding it challenging to determine the length of an array in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling this task, but there’s a solution at hand.
Think of Java’s array length property as a measuring tape – allowing us to quickly and accurately determine the size of our arrays. It’s a simple yet powerful tool that can greatly assist in various programming scenarios.
This guide will walk you through the process of finding the length of an array in Java, from basic usage to advanced techniques. We’ll cover everything from the basics of the ‘length’ property, its usage in different contexts, to more advanced scenarios and even alternative approaches.
So, let’s dive in and start mastering Java array length!
TL;DR: How Do I Find the Length of an Array in Java?
In Java, you can use the
.length
property of an array to find its length by using the syntax,int length = array.length;
. It’s a simple and straightforward way to determine the size of your array.
Here’s a simple example:
int[] array = {1, 2, 3, 4, 5};
int length = array.length;
System.out.println(length);
# Output:
# 5
In this example, we’ve created an array of integers with five elements. By using the ‘length’ property (array.length
), we’re able to quickly determine the size of the array, which in this case, outputs ‘5’.
This is the basic way to find the length of an array in Java, but there’s much more to learn about handling arrays in Java. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
Understanding Java Array Length: The Basics
In Java, arrays are objects that store multiple variables of the same type. However, once an array is created, its size is fixed. This is where the ‘length’ property comes into play, providing you the ability to determine the size of the array quickly and easily. It’s a built-in property of an array and is always available for use.
Consider the following example:
int[] myArray = {10, 20, 30, 40, 50};
int arrayLength = myArray.length;
System.out.println("The length of the array is: " + arrayLength);
# Output:
# The length of the array is: 5
In this example, myArray
is an array of integers containing five elements. The ‘length’ property (myArray.length
) returns the size of the array, which is then stored in the arrayLength
variable. When printed, it outputs ‘The length of the array is: 5’.
Advantages of Using ‘length’ Property
The ‘length’ property offers a simple and effective way to determine the size of an array in Java. It’s readily available and doesn’t require any additional method calls. This makes it a preferred choice for developers when dealing with array length.
Potential Pitfalls
While the ‘length’ property is straightforward, it’s important to remember that it returns the total size of the array, not the number of occupied elements. If an array has a length of 5 but only 3 elements are filled, the ‘length’ property will still return 5. This can lead to ‘ArrayIndexOutOfBoundsException’ if not handled properly.
In the next section, we’ll explore how to use the ‘length’ property in more complex scenarios.
Using Java Array Length in Complex Scenarios
The ‘length’ property can be incredibly useful in more complex scenarios, such as when used within loops or conditional statements. This allows for dynamic and efficient handling of arrays in Java.
Looping Through an Array
One common use of the ‘length’ property is in controlling the iteration of a loop. For example, you can use the ‘length’ property to loop through all the elements in an array:
int[] myArray = {10, 20, 30, 40, 50};
for (int i = 0; i < myArray.length; i++) {
System.out.println(myArray[i]);
}
# Output:
# 10
# 20
# 30
# 40
# 50
In this example, we use the ‘length’ property (myArray.length
) as the condition in the for loop. This ensures that the loop iterates exactly as many times as there are elements in the array, preventing an ‘ArrayIndexOutOfBoundsException’.
Array Length in Conditional Statements
The ‘length’ property can also be used in conditional statements, such as to check if an array is empty:
int[] myArray = {};
if (myArray.length == 0) {
System.out.println("The array is empty");
} else {
System.out.println("The array is not empty");
}
# Output:
# The array is empty
In this example, we use the ‘length’ property (myArray.length
) to check if the array is empty. If the length of the array is 0, it means the array is empty, and we print ‘The array is empty’. Otherwise, we print ‘The array is not empty’.
These examples demonstrate how the ‘length’ property can be utilized in more complex scenarios, providing greater flexibility and efficiency when working with arrays in Java.
Exploring Alternative Approaches: Array.getLength()
While the ‘length’ property is the most common way to determine the size of an array in Java, there are alternative approaches available for more complex scenarios. One such alternative is the Array.getLength()
method from the java.lang.reflect
package.
Using Array.getLength()
The Array.getLength()
method provides a way to find the length of an array, similar to the ‘length’ property. However, it’s more flexible as it can handle arrays of any type, including arrays of objects.
Here’s how you can use it:
import java.lang.reflect.Array;
int[] myArray = {10, 20, 30, 40, 50};
int length = Array.getLength(myArray);
System.out.println(length);
# Output:
# 5
In this example, we import the Array
class from the java.lang.reflect
package and use the getLength()
method to find the length of myArray
. The result is the same as using the ‘length’ property.
Advantages and Disadvantages of Array.getLength()
The Array.getLength()
method is a powerful tool that offers greater flexibility than the ‘length’ property. It can handle arrays of any type, including arrays of objects, which can be beneficial in certain scenarios.
However, it also has its downsides. Firstly, it’s slower than using the ‘length’ property because it involves a method call. Secondly, it can throw an IllegalArgumentException
if the object passed to it is not an array. Therefore, it’s important to use this method judiciously and only when necessary.
In the next section, we’ll discuss some common issues you might encounter when finding the length of an array and how to troubleshoot them.
Troubleshooting Java Array Length Issues
While working with Java arrays and determining their length, you may encounter a few common issues. The most prevalent of these is the ‘ArrayIndexOutOfBoundsException’. Let’s delve into this error and how to troubleshoot it.
Understanding ‘ArrayIndexOutOfBoundsException’
The ‘ArrayIndexOutOfBoundsException’ is thrown to indicate that you’re trying to access an array element using an illegal index, either negative or greater than or equal to the size of the array.
Consider this example:
int[] myArray = {10, 20, 30, 40, 50};
System.out.println(myArray[5]);
# Output:
# Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 5 out of bounds for length 5
In this example, we’re trying to access the element at index 5 (myArray[5]
). However, since the array’s length is 5 and the index is zero-based, the maximum index we can access is 4. Thus, an ‘ArrayIndexOutOfBoundsException’ is thrown.
Handling ‘ArrayIndexOutOfBoundsException’
To avoid this exception, always ensure that the index you’re trying to access is within the range of 0 to (array length – 1). You can use the ‘length’ property to dynamically control this, as shown in the looping example in the advanced section.
int[] myArray = {10, 20, 30, 40, 50};
for (int i = 0; i < myArray.length; i++) {
System.out.println(myArray[i]);
}
# Output:
# 10
# 20
# 30
# 40
# 50
By using the ‘length’ property as the condition in the loop (i < myArray.length
), we ensure that we only access valid indices, thus avoiding the ‘ArrayIndexOutOfBoundsException’.
Understanding these considerations can help you handle arrays more efficiently in Java, and avoid common pitfalls that could hamper your programming tasks.
Fundamentals of Java Arrays
Before diving deeper into the intricacies of Java array length, it’s essential to understand the basics of arrays in Java and why knowing their length is crucial.
What is an Array in Java?
An array in Java is a data structure that can store multiple values of the same type. It’s essentially a container that holds a fixed number of values of a single type, such as integers, strings, or even objects.
Here’s an example of declaring and initializing an array in Java:
int[] myArray = {10, 20, 30, 40, 50};
In this example, myArray
is an array of integers that stores five elements.
Why is Array Length Important?
Knowing the length of an array is crucial for several reasons. It allows you to iterate over the array, access its elements, and perform various operations on it, such as sorting or searching. Without knowing the length of an array, you risk accessing an index that doesn’t exist and causing an ‘ArrayIndexOutOfBoundsException’.
How are Arrays Structured in Java?
In Java, arrays are objects. When you create an array, Java reserves a block of memory in the system capable of storing the specified number of elements. Each element in the array is assigned a unique index, starting from 0 and going up to one less than the size of the array.
Understanding the ‘length’ Property
The ‘length’ property is a built-in property of arrays in Java. It returns the size of the array, which is determined at the time of creation and remains constant. The ‘length’ property is always available and doesn’t require any method calls, making it a quick and efficient way to determine the size of an array.
Here’s how you can use it:
int[] myArray = {10, 20, 30, 40, 50};
int length = myArray.length;
System.out.println(length);
# Output:
# 5
In this example, we use the ‘length’ property (myArray.length
) to find the length of myArray
, which outputs ‘5’.
The Bigger Picture: Java Array Length and Larger Projects
Understanding how to determine the length of an array in Java is a fundamental skill that has a wide range of applications, especially in larger projects.
Array Length in Sorting Algorithms
In sorting algorithms, such as bubble sort or quick sort, knowing the length of the array is crucial. It’s used to control the number of iterations and to access the elements of the array during the sorting process. Without the ‘length’ property, implementing these algorithms would be significantly more complex.
Array Length in Searching Algorithms
Similarly, in searching algorithms like binary search or linear search, the ‘length’ property plays a pivotal role. It’s used to determine the boundaries of the search and to access the elements of the array during the search process.
Further Resources for Mastering Java Arrays
To further your understanding of Java arrays and related concepts, here are a few resources that you might find useful:
- Best Practices for Using Arrays in Java – Explore advanced array operations such as copying, merging, and splitting in Java.
Working with 2D Arrays in Java – Learn about initializing, accessing, and manipulating elements in 2D arrays.
Java Array Initialization Guide – Master declaring and initializing arrays to store and manage data effectively in Java.
Oracle’s Java Arrays Documentation provides an overview of arrays in Java, including how to declare and manipulate them.
GeekforGeek’s Java Array Length Guide provides a detailed explanation of the ‘length’ property in Java.
Tutorialspoint’s Java Array Length Tutorial covers various aspects of Java arrays, including how to determine their length.
By leveraging these resources, you can deepen your knowledge of Java arrays and enhance your programming skills.
Wrapping Up: Java Array Length
In this comprehensive guide, we’ve delved into the process of determining the length of an array in Java. From the basic usage of the ‘length’ property to its application in more complex scenarios, we’ve explored the various aspects of handling array length in Java.
We began with the basics, understanding how the ‘length’ property works and its application in a simple context. We then ventured into more advanced territory, using the ‘length’ property in loops and conditional statements. We also explored an alternative approach, the Array.getLength()
method from the java.lang.reflect
package, providing you with a broader range of tools to handle array length.
Along the way, we tackled common challenges, such as the ‘ArrayIndexOutOfBoundsException’, providing you with solutions and workarounds for each issue. We also delved into the fundamentals of arrays in Java, giving you a deeper understanding of why the ‘length’ property is essential and how it works.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Speed | Ease of Use |
---|---|---|---|
‘length’ Property | Moderate | High | High |
Array.getLength() | High | Moderate | Moderate |
Whether you’re just starting out with Java arrays or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of how to determine the length of an array in Java.
The ability to handle array length effectively is a fundamental skill in Java programming. With the knowledge you’ve gained from this guide, you’re well equipped to handle arrays more efficiently in your future projects. Happy coding!