Mastering Java: How to Initialize and Declare an Array
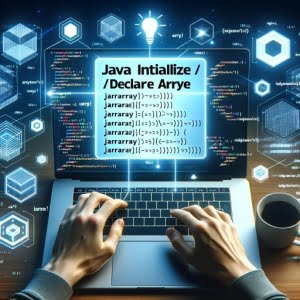
Are you finding it challenging to manage data in Java? You’re not alone. Many developers find it difficult to handle arrays in Java, but with the right guidance, it can become second nature.
Think of Java arrays as a well-organized bookshelf – they help you store and manage your data efficiently, providing a versatile tool for various tasks.
This guide will walk you through the process of declaring and initializing arrays in Java, from the basics to more advanced techniques. We’ll cover everything from the simple declaration of arrays to initializing multi-dimensional arrays and even discuss alternative approaches.
So, let’s dive in and start mastering arrays in Java!
TL;DR: How Do I Declare and Initialize an Array in Java?
To declare and initialize an array in Java, you can use the following syntax:
int[] myArray = new int[]{1, 2, 3, 4, 5};
. This creates an array of integers with the values 1, 2, 3, 4, and 5.
Here’s a simple example:
int[] myArray = new int[]{1, 2, 3, 4, 5};
System.out.println(Arrays.toString(myArray));
# Output:
# [1, 2, 3, 4, 5]
In this example, we declare and initialize an array named myArray
with the integer values 1, 2, 3, 4, and 5. We then print the array using Arrays.toString(myArray)
, which converts the array into a string format that can be printed.
This is just a basic way to declare and initialize an array in Java, but there’s much more to learn about handling arrays efficiently. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Declaring and Initializing Arrays: The Basics
When working with Java, declaring and initializing arrays is a fundamental skill. Let’s start with the basics.
Declaring an Array
In Java, you declare an array with the following syntax:
DataType[] arrayName;
For instance, if we want to declare an array of integers, we would do:
int[] myArray;
Here, int
is the data type of the array, and myArray
is the name of the array. At this point, the array is declared, but it doesn’t contain any values.
Initializing an Array
After declaring an array, we need to initialize it by assigning it a set of values. Here’s the syntax to initialize an array in Java:
arrayName = new DataType[]{value1, value2, ..., valueN};
Let’s initialize the myArray
we declared earlier:
myArray = new int[]{1, 2, 3, 4, 5};
Now, myArray
is an array of integers containing the values 1, 2, 3, 4, and 5.
Declaring and Initializing in One Line
In Java, you can also declare and initialize an array in the same line for convenience:
DataType[] arrayName = new DataType[]{value1, value2, ..., valueN};
Here’s how we can declare and initialize myArray
in one line:
int[] myArray = new int[]{1, 2, 3, 4, 5};
This is a compact and efficient way to handle arrays in Java. However, it’s important to remember that once an array’s size is set during initialization, it can’t be changed. This is one of the potential pitfalls you should be aware of when working with arrays.
Multidimensional Arrays and Arrays of Objects in Java
As you continue your journey with Java, you’ll encounter scenarios where basic arrays just won’t cut it. That’s where multidimensional arrays and arrays of objects come into play.
Multidimensional Arrays in Java
A multidimensional array is essentially an array of arrays. It’s useful when you need to store data in a structured format like a table or a grid. Here’s how you can declare and initialize a two-dimensional array:
int[][] twoDArray = new int[][]{{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
In this example, twoDArray
is a 2D array with three rows and three columns. You can access elements in twoDArray
using two indices. For instance, twoDArray[0][1]
would give you the value 2.
Arrays of Objects in Java
Arrays in Java can also hold objects. This is beneficial when you need to manage a collection of complex data types. Here’s an example of declaring and initializing an array of String
objects:
String[] stringArray = new String[]{"Java", "Python", "JavaScript"};
In this example, stringArray
is an array of String
objects with the values “Java”, “Python”, and “JavaScript”.
Best Practices
When working with multidimensional arrays or arrays of objects, it’s crucial to remember the following best practices:
- Always specify the size of the array during initialization. Java doesn’t allow dynamic resizing of arrays.
Be cautious when working with arrays of objects. If not handled correctly, they can lead to memory leaks.
Use appropriate data structures for your needs. If you’re dealing with complex data, consider using collections like ArrayList or LinkedList.
Exploring Alternative Methods for Array Initialization
As you dive deeper into Java, you’ll encounter alternative ways to declare and initialize arrays. Let’s explore two such methods: using the Array class and the Collections framework.
Using the Array Class
Java provides the Arrays
class in java.util
package that offers static methods to easily manipulate arrays. Here’s an example of initializing an array using the Arrays.fill()
method:
int[] array = new int[5];
Arrays.fill(array, 10);
System.out.println(Arrays.toString(array));
# Output:
# [10, 10, 10, 10, 10]
In this example, we declare an array of size 5 and use Arrays.fill()
to fill all elements with the value 10. The Arrays
class provides various other utility methods for sorting, searching, and comparing arrays.
Using the Collections Framework
Java’s Collections framework provides the ArrayList
class, a resizable array implementation. Here’s how to declare and initialize an ArrayList:
ArrayList<Integer> arrayList = new ArrayList<>(Arrays.asList(1, 2, 3, 4, 5));
System.out.println(arrayList);
# Output:
# [1, 2, 3, 4, 5]
In this example, we declare and initialize an ArrayList
with the values 1, 2, 3, 4, and 5. The primary advantage of using ArrayList
is its dynamic size. You can add or remove elements from an ArrayList
at any time.
Choosing the Right Approach
While these alternative methods offer flexibility, they come with their own trade-offs. The Arrays
class is easy to use but lacks the flexibility of dynamic resizing. On the other hand, ArrayList
offers dynamic resizing but may have performance implications for large datasets.
As a rule of thumb, stick with basic arrays for simple, fixed-size data structures. For more complex or dynamic data structures, consider using the Arrays
class or ArrayList
.
Troubleshooting Common Array Issues in Java
While working with arrays in Java, you might encounter a few common issues. Let’s discuss them along with their solutions and workarounds.
ArrayIndexOutOfBoundsException
The ArrayIndexOutOfBoundsException
occurs when you try to access an array element using an invalid index, i.e., an index that is either negative or greater than or equal to the size of the array.
Here’s an example that throws ArrayIndexOutOfBoundsException
:
int[] array = new int[]{1, 2, 3, 4, 5};
System.out.println(array[5]);
# Output:
# Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 5 out of bounds for length 5
In this example, we try to access array[5]
, but the valid indices of array
are from 0 to 4. To avoid this exception, always ensure that your index is within the valid range.
NullPointerException
A NullPointerException
occurs when you try to access an array that hasn’t been initialized. Here’s an example:
int[] array;
System.out.println(array.length);
# Output:
# Exception in thread "main" java.lang.NullPointerException
In this example, we declare an array but don’t initialize it, and then we try to access its length. To avoid this exception, always initialize your arrays before using them.
Best Practices
While working with arrays in Java, keep these tips in mind:
- Always initialize your arrays before using them.
Be cautious with array indices to avoid
ArrayIndexOutOfBoundsException
.Consider using
ArrayList
or other collection classes for dynamic and complex data structures.
Understanding Java Arrays: The Fundamentals
Before we dive deeper, let’s take a moment to understand what arrays in Java are and why they are so important.
What are Arrays in Java?
In Java, an array is a container object that holds a fixed number of values of a single type. The length of an array is established when the array is created, and array elements are accessed using their numerical, zero-based index.
Here’s a simple example:
int[] array = new int[]{1, 2, 3, 4, 5};
System.out.println(array[0]);
# Output:
# 1
In this example, we declare and initialize an array with five elements. We then print the first element of the array using array[0]
.
The Role of Arrays in Data Management
Arrays play a critical role in data management in Java. They are used to store and manipulate data efficiently, particularly when dealing with a large number of similar data types. Arrays are also used as the underlying data structure for many algorithms and data structures.
The Importance of Arrays for Efficient Java Programming
Understanding arrays is fundamental to efficient programming in Java. With a solid grasp of arrays, you can manage data more efficiently, write cleaner code, and implement complex algorithms and data structures. Whether you’re working on a small project or a large-scale application, arrays are an essential tool in your Java toolkit.
The Power of Arrays in Larger Java Projects
Arrays in Java are not just for managing simple data. They are an integral part of larger Java projects, playing a crucial role in data manipulation, sorting algorithms, and more.
Arrays in Data Manipulation
In larger projects, you will often find yourself dealing with large datasets. Arrays provide a fast and efficient way to store and manipulate this data. For instance, you can use arrays to implement various sorting algorithms, perform statistical calculations, or even manage data in databases.
Arrays in Sorting Algorithms
Sorting is a fundamental concept in computer science, and arrays play a key role in implementing sorting algorithms. Whether it’s a simple algorithm like Bubble Sort or a more complex one like Quick Sort, arrays are at the heart of these algorithms.
Exploring Related Concepts
Once you’re comfortable with arrays, you can explore related concepts like ArrayLists and LinkedLists. These Java collections offer more flexibility than arrays and are better suited for certain scenarios.
For instance, ArrayLists, like arrays, are ordered and allow duplicates. However, unlike arrays, they are dynamic and can grow or shrink at runtime. LinkedLists, on the other hand, are ideal for scenarios where frequent insertions and deletions are required.
Further Resources for Mastering Java Arrays
For those who want to delve deeper into the world of Java arrays, here are some valuable resources:
- Beginner’s Guide to Arrays in Java – Discover how arrays can store multiple values of the same type in Java.
Java String Array – Explore the usage of string arrays in Java for storing and manipulating text data.
Finding Array Length in Java – Explore techniques for accessing the number of elements in an array.
Java Arrays – Oracle Documentation provides an in-depth look at arrays in Java.
Java Programming – Arrays by W3Schools offers plenty of examples to help you understand arrays better.
Java Arrays by GeeksforGeeks covers topics from basic array operations to multidimensional arrays.
Wrapping Up:
In this comprehensive guide, we’ve explored the ins and outs of declaring and initializing arrays in Java. We’ve covered everything from the basic syntax to more advanced techniques, providing you with a solid foundation in Java arrays.
We started with the basics, learning how to declare and initialize arrays in Java. We then dove into more advanced topics, such as multidimensional arrays and arrays of objects. Along the way, we tackled common issues like ArrayIndexOutOfBoundsException
and NullPointerException
, providing solutions and workarounds to help you avoid these pitfalls.
We also explored alternative methods for declaring and initializing arrays, such as using the Arrays
class and the ArrayList
in the Collections framework. Here’s a quick comparison of these methods:
Method | Flexibility | Performance | Use Case |
---|---|---|---|
Basic Arrays | Low | High | Simple, fixed-size data structures |
Arrays Class | Moderate | High | More complex data structures |
ArrayList | High | Moderate | Dynamic and complex data structures |
Whether you’re just starting out with Java or looking to deepen your understanding of arrays, we hope this guide has been a valuable resource. With this knowledge, you’re well-equipped to handle arrays in Java with confidence and efficiency. Happy coding!