How to Create and Manipulate Java String Arrays
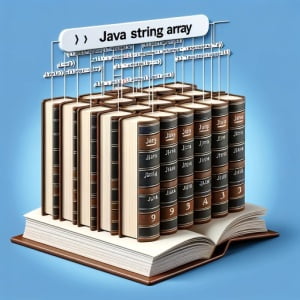
Are you finding it challenging to work with string arrays in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling string arrays in Java, but we’re here to help.
Think of Java’s string arrays as a collection of bookshelves, each holding a different book (string). This analogy will help you visualize and understand the concept of string arrays better.
In this guide, we’ll walk you through the process of working with string arrays in Java, from their creation, manipulation, and usage. We’ll cover everything from the basics to more advanced techniques, as well as alternative approaches.
Let’s get started and master string arrays in Java!
TL;DR: How Do I Create and Use a String Array in Java?
In Java, you can create and use a string array by declaring an array and initializing it with string values,
String[] stringArray = new String[]{"stringA", "stringB"};
. You can then access the elements of the array using their index.
Here’s a simple example:
String[] strArray = new String[]{"Hello", "World"};
System.out.println(strArray[0]);
// Output:
// Hello
In this example, we’ve created a string array named strArray
and initialized it with two elements: ‘Hello’ and ‘World’. We then print the first element of the array (index 0), which outputs ‘Hello’.
This is a basic way to create and use a string array in Java, but there’s much more to learn about manipulating and using string arrays effectively. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Basic Usage of Java String Arrays
- Intermediate-Level Java String Array Operations
- Exploring Alternative Data Structures for Storing Strings
- Troubleshooting Common Issues with Java String Arrays
- Understanding Java Arrays
- The Role of String Arrays
- Applying Java String Arrays in Real-world Projects
- Further Resources for Mastering Java String Arrays
- Wrapping Up: Java String Arrays
Basic Usage of Java String Arrays
Creating a Java String Array
In Java, creating a string array is simple. You declare an array and initialize it with string values. Here’s an example:
String[] strArray = new String[]{"Java", "String", "Array"};
In this code, we’ve created a string array named strArray
and initialized it with three elements: ‘Java’, ‘String’, and ‘Array’.
Accessing Array Elements
You can access the elements of the array using their index. Remember, array indices start at 0. Let’s access the first element (index 0) of our strArray
:
System.out.println(strArray[0]);
// Output:
// Java
This code prints out ‘Java’, the string at index 0 of strArray
.
Modifying Array Elements
You can also modify an element of a string array using its index. Let’s change the second element (index 1) of our strArray
to ‘Array’:
strArray[1] = "Array";
System.out.println(strArray[1]);
// Output:
// Array
We’ve changed the string at index 1 from ‘String’ to ‘Array’.
Iterating Over a String Array
To iterate over a string array, you can use a for-each loop. Here’s how you can print all elements of strArray
:
for (String str : strArray) {
System.out.println(str);
}
// Output:
// Java
// Array
// Array
This for-each loop goes through each element of strArray
and prints it. As you can see, the second element has been changed to ‘Array’.
Intermediate-Level Java String Array Operations
Multidimensional String Arrays
Java allows the creation of multidimensional arrays. In the context of string arrays, these can be thought of as a library with multiple bookshelves, each containing different books. Here’s an example of a two-dimensional string array:
String[][] multiStrArray = new String[][]{{"Hello", "World"}, {"Java", "String", "Array"}};
System.out.println(multiStrArray[0][1]);
System.out.println(multiStrArray[1][2]);
// Output:
// World
// Array
In this code, we’ve created a two-dimensional string array multiStrArray
and accessed elements from both dimensions.
Sorting String Arrays
Java provides built-in methods to sort string arrays. Here’s how you can sort a string array in ascending order:
import java.util.Arrays;
String[] strArray = new String[]{"Java", "String", "Array"};
Arrays.sort(strArray);
for (String str : strArray) {
System.out.println(str);
}
// Output:
// Array
// Java
// String
In this example, we’ve used the Arrays.sort()
method from the java.util.Arrays
class to sort strArray
. The sorted array is then printed, showing the strings in alphabetical order.
Converting a String Array to ArrayList
Sometimes, it’s more convenient to work with an ArrayList than a traditional array. Here’s how you can convert a string array to an ArrayList:
import java.util.ArrayList;
import java.util.Arrays;
String[] strArray = new String[]{"Java", "String", "Array"};
ArrayList<String> strArrayList = new ArrayList<>(Arrays.asList(strArray));
System.out.println(strArrayList);
// Output:
// [Java, String, Array]
In this code, we’ve used the Arrays.asList()
method to convert strArray
to a List, which is then passed to the ArrayList constructor. The resulting ArrayList is then printed.
Exploring Alternative Data Structures for Storing Strings
Using ArrayList for Storing Strings
Java’s ArrayList is a resizable array that provides more flexibility compared to a traditional array. It’s an excellent choice when you’re dealing with a dynamic number of elements. Here’s how you can create an ArrayList and add strings to it:
import java.util.ArrayList;
ArrayList<String> strArrayList = new ArrayList<>();
strArrayList.add("Java");
strArrayList.add("String");
strArrayList.add("Array");
System.out.println(strArrayList);
// Output:
// [Java, String, Array]
In this example, we’ve created an ArrayList strArrayList
and added three strings to it. The ArrayList automatically resizes to accommodate the added elements.
Leveraging LinkedList for Storing Strings
Java’s LinkedList is another alternative for storing strings. It’s especially beneficial when you need to perform frequent insertions and deletions as it provides efficient performance for these operations. Here’s how you can create a LinkedList and add strings to it:
import java.util.LinkedList;
LinkedList<String> strLinkedList = new LinkedList<>();
strLinkedList.add("Java");
strLinkedList.add("String");
strLinkedList.add("Array");
System.out.println(strLinkedList);
// Output:
// [Java, String, Array]
In this code, we’ve created a LinkedList strLinkedList
and added three strings to it. Just like ArrayList, LinkedList also provides dynamic resizing.
Making the Right Choice
Choosing between a string array, ArrayList, and LinkedList depends on your specific needs. If you know the number of elements in advance, a string array is a good choice. If you need a resizable array and efficient random access, go for ArrayList. If you’re performing many insertions and deletions, LinkedList might be the best option.
Troubleshooting Common Issues with Java String Arrays
Handling ArrayIndexOutOfBoundsException
One common issue when working with Java string arrays is the ArrayIndexOutOfBoundsException
. This exception is thrown when you try to access an array element using an index that is outside the valid range. The valid range of indices for an array is from 0 to the array’s length minus one.
String[] strArray = new String[]{"Java", "String", "Array"};
System.out.println(strArray[3]);
// Output:
// Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 3 out of bounds for length 3
In this example, we’re trying to access the element at index 3 of strArray
, but the array only has elements at indices 0, 1, and 2. This causes an ArrayIndexOutOfBoundsException
.
To avoid this exception, always ensure that the index you’re using to access an array element is within the valid range.
Dealing with NullPointerException
Another common issue is the NullPointerException
, which is thrown when you try to access an array that has not been initialized.
String[] strArray = null;
System.out.println(strArray[0]);
// Output:
// Exception in thread "main" java.lang.NullPointerException
In this code, strArray
is declared but not initialized, so it’s null
. Trying to access strArray[0]
results in a NullPointerException
.
To avoid this exception, always ensure that your array is initialized before trying to access its elements.
Understanding Java Arrays
Arrays in Java form the bedrock of many data structures and are an essential concept in programming. They are fixed-size, sequential collections of elements of the same type. This means that once an array is created, its size cannot be changed, and it can only store elements of the declared type.
int[] intArray = new int[5]; // an array of integers
String[] strArray = new String[5]; // an array of strings
In these examples, intArray
can only hold integers, and strArray
can only hold strings. Both arrays have a fixed size of 5.
The Role of String Arrays
String arrays in Java hold a sequence of strings. They are especially useful when you want to store multiple strings in an ordered manner. For instance, you might use a string array to store a list of words, sentences, or names.
String[] names = new String[]{"Alice", "Bob", "Charlie"};
// Output:
// Alice
// Bob
// Charlie
In this example, the string array names
holds three names. You can access each name using its index in the array.
Understanding the fundamentals of arrays is crucial to mastering string arrays in Java. With this knowledge, you can create, manipulate, and traverse string arrays effectively.
Applying Java String Arrays in Real-world Projects
Word Frequency Counter Using Java String Arrays
One practical application of Java string arrays is creating a word frequency counter. This tool can analyze a piece of text and count how many times each word appears. Here’s a simplified example of how you might do this:
import java.util.HashMap;
import java.util.Map;
String[] words = new String[]{"Hello", "Hello", "World"};
Map<String, Integer> wordCount = new HashMap<>();
for (String word : words) {
wordCount.put(word, wordCount.getOrDefault(word, 0) + 1);
}
System.out.println(wordCount);
// Output:
// {Hello=2, World=1}
In this code, we’ve created a string array words
and a HashMap wordCount
. For each word in words
, we add the word to wordCount
with a count of 1, or increment the count if the word is already in wordCount
. The output shows the frequency of each word in words
.
Digging Deeper: Multidimensional Arrays and ArrayLists
If you’re interested in going beyond one-dimensional string arrays, you might explore multidimensional arrays and ArrayLists. Multidimensional arrays can store data in a grid-like structure, while ArrayLists provide a dynamic-size array with more flexibility than a traditional array.
Further Resources for Mastering Java String Arrays
To continue your journey in mastering Java string arrays, here are some useful resources:
- Understanding Array Manipulation in Java – Understand the concept of arrays as ordered collections of elements in Java.
Array to String in Java – Learn how to convert arrays to string representations in Java.
Initialize/Declare Array in Java – Understand different ways to declare and initialize arrays in Java.
Oracle’s Java Tutorials cover many aspects of Java programming, including arrays.
GeeksforGeeks Java Array Library offers a collection of articles and tutorials on Java arrays, including string arrays.
Baeldung’s Guide to Java Arrays provides a deep dive into Java string arrays, and array-related classes in the Java API.
Wrapping Up: Java String Arrays
In this comprehensive guide, we’ve delved into the world of Java string arrays, exploring their creation, manipulation, and effective usage. We’ve also touched on the fundamental concept of arrays in Java, setting a solid foundation for understanding string arrays and their role in Java programming.
We began with the basics, learning how to create, access, modify, and iterate over a Java string array. We then progressed into more advanced territory, discussing multidimensional string arrays, sorting string arrays, and converting a string array to an ArrayList. We also explored alternative data structures for storing strings, such as ArrayLists and LinkedLists, and provided a comparison to help you make the right choice for your specific needs.
Along the way, we tackled common issues you might encounter when working with Java string arrays, such as ArrayIndexOutOfBoundsException and NullPointerException, providing you with solutions and preventative measures for these common pitfalls.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity | Use Case |
---|---|---|---|
String Array | Fixed size, single type | Low | Storing a known number of strings |
ArrayList | Dynamic size, single type | Moderate | Storing a dynamic number of strings |
LinkedList | Dynamic size, single type | High | Frequent insertions and deletions |
Whether you’re a beginner just starting out with Java string arrays or an experienced developer looking for a refresher, we hope this guide has provided you with valuable insights and practical knowledge.
Java string arrays are a fundamental tool in any Java programmer’s toolkit. With the knowledge gained from this guide, you’re now well-equipped to use Java string arrays effectively in your programming projects. Happy coding!