[FIXED] Help solving error “npm ERR! code ELIFECYCLE”
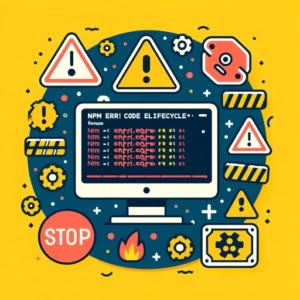
At IOFLOOD, managing Node.js projects often involves troubleshooting various errors, including the pesky ‘npm err! code ELIFECYCLE’. Having encountered this error multiple times, I understand the frustration it can cause during project development. To help other developers facing similar challenges, I’ve created this step-by-step troubleshooting guide.
This guide will navigate you through understanding and resolving this common npm error, ensuring your Node.js projects run smoothly. With a step-by-step approach, we aim to transform this daunting error message into a resolved issue, allowing you to continue your development journey with confidence and ease.
Let’s dive into the error code and learn how to save our project timelines and efficiency.
TL;DR: How Do I Fix ‘npm err! code ELIFECYCLE’?
To swiftly address the ‘npm err! code ELIFECYCLE’ error, begin by scrutinizing the
package.json
scripts for any typos or commands that don’t align with your project’s structure. A common remedy involves ensuring your script accurately reflects the intended action for your application.
Here’s a straightforward example to illustrate:
"scripts": {
"start": "node app.js"
}
In this snippet, we’re ensuring that the start
script points to the correct entry file of our application, app.js
. This adjustment often resolves the error by aligning the script with the actual configuration of the project.
Embarking on this quick fix can pave the way to a smoother development experience. However, the journey to mastering npm errors doesn’t end here. Keep reading for a comprehensive exploration into troubleshooting and preemptively avoiding this common hiccup.
Table of Contents
Understanding npm Lifecycle Scripts
Navigating through ‘npm err! code ELIFECYCLE’ begins with a solid grasp of npm lifecycle scripts. These scripts are pivotal, serving as automated shortcuts for tasks like launching or testing your application. Let’s demystify how to set up these essential scripts within your package.json
file correctly.
Consider a scenario where you need to configure a test script. The aim is to automate running your unit tests every time you execute a certain command. Here’s how you could set it up:
"scripts": {
"test": "mocha **/*.test.js"
}
# Expected output:
# > mocha **/*.test.js
# [Output of your tests here]
In this example, we’ve designated mocha **/*.test.js
as the command to run our tests, where mocha
is the testing framework and **/*.test.js
specifies that all files ending with .test.js
in any subdirectory should be considered. Once this script is added to your package.json
, running npm test
in your terminal will execute this command, thereby running your tests.
The importance of this setup cannot be overstated. By correctly configuring your lifecycle scripts, you not only streamline your development process but also mitigate potential errors like ‘npm err! code ELIFECYCLE’. This proactive approach ensures your project’s scripts align with your development workflow, making your journey smoother and more efficient.
Causes of npm err! code ELIFECYCLE
Encountering ‘npm err! code ELIFECYCLE’ can often feel like navigating a maze without a map. This error frequently arises from a few common issues: missing scripts, environmental discrepancies, or dependency conflicts. Let’s deep dive into how to identify and tackle these challenges.
Missing Scripts Resolution
One of the primary culprits for this error is a missing script in your package.json
that npm expects to find. Imagine you’ve configured a build
script that is nowhere to be found in your package.json
. Here’s how you rectify this:
"scripts": {
"build": "webpack --config webpack.config.js"
}
# Expected output:
# > webpack --config webpack.config.js
# [Webpack compilation output]
In this snippet, we’re adding a build
script pointing to Webpack for bundling our application. This inclusion ensures that when npm tries to run the build
script, it successfully executes Webpack, preventing the ‘npm err! code ELIFECYCLE’ error.
Environmental Issues and Their Fixes
Environmental issues, such as incorrect Node.js or npm versions, can also lead to this error. Ensuring compatibility between your development environment and your project’s requirements is crucial. For instance, if your project requires a specific Node.js version not installed on your machine, you can use nvm
(Node Version Manager) to switch between versions. Here’s how:
nvm install 12.18.3
nvm use 12.18.3
# Expected output:
# Now using node v12.18.3 (npm v6.14.6)
This command sequence installs and switches to Node.js version 12.18.3, aligning your environment with your project’s needs and averting potential ‘npm err! code ELIFECYCLE’ errors.
Tackling Dependency Problems
Lastly, dependency issues can trigger this error. Inconsistent or corrupted node modules might cause npm to exit with an ELIFECYCLE error. A clean reinstallation of your node modules can often resolve this. Execute the following to refresh your project’s dependencies:
rm -rf node_modules
npm install
# Expected output:
# [A list of installed packages]
This command removes the existing node modules directory and reinstalls all dependencies, ensuring that any corrupt or inconsistent packages are replaced with fresh, compatible versions. By addressing these common triggers with the outlined fixes, you can navigate away from ‘npm err! code ELIFECYCLE’ errors and towards a more seamless development experience.
Advanced Solutions for npm Errors
When standard troubleshooting doesn’t resolve the ‘npm err! code ELIFECYCLE’, it’s time to explore more advanced techniques. These approaches are particularly useful in complex scenarios where the usual fixes fall short.
Clean Installation with npm ci
A powerful alternative for fixing dependency-related issues is using npm ci
. This command offers a clean slate by removing the existing node_modules
folder and installing all the dependencies afresh, according to the package-lock.json
. This ensures a consistent installation environment.
npm ci
# Expected output:
# added 1250 packages in 15s
The output indicates a successful reinstallation of all project dependencies without the inconsistencies that might have been causing the ‘npm err! code ELIFECYCLE’ error. It’s a more drastic but effective approach, especially in continuous integration environments or when you need to ensure that your dependencies are in exact alignment with your package-lock.json
.
Leveraging Environment Variables
Another advanced technique involves configuring environment variables to solve ‘npm err! code ELIFECYCLE’. Environment variables can influence npm’s behavior, and adjusting them might resolve the issue. For example, increasing the verbosity of npm logs can provide deeper insights into the error’s root cause.
export NPM_CONFIG_LOGLEVEL=verbose
npm run your_script
# Expected output:
# [Detailed npm logs providing insights into the script execution]
By setting the NPM_CONFIG_LOGLEVEL
to verbose
, you increase the detail level of the logs, which can help identify where the process is failing. This detailed logging is invaluable for diagnosing complex issues that aren’t immediately apparent.
These expert-level strategies, from clean installations with npm ci
to leveraging environment variables, provide powerful tools in your arsenal for tackling the ‘npm err! code ELIFECYCLE’. By understanding and applying these techniques, you can navigate even the most challenging npm errors with confidence.
Troubleshooting for npm Errors
When faced with the daunting ‘npm err! code ELIFECYCLE’, a systematic approach to troubleshooting can be your roadmap to resolution. This process involves verifying dependencies, checking for global package conflicts, and delving into npm’s log files for clues. Let’s break down these steps with practical examples and insights.
Verify Your Dependencies
The first step in troubleshooting is to ensure all your project’s dependencies are correctly installed and up-to-date. An outdated or missing dependency can easily trigger the ‘npm err! code ELIFECYCLE’. Here’s how you can check and update your dependencies:
npm outdated
npm update
# Expected output:
# [List of outdated packages, followed by update confirmation]
This command lists any dependencies that are outdated and updates them to the latest version. Keeping your dependencies current is crucial for preventing errors and ensuring compatibility across your project.
Check for Global Package Conflicts
Global package conflicts are another common source of the ‘npm err! code ELIFECYCLE’. Sometimes, a globally installed package might conflict with a project’s local dependency. To identify and resolve these conflicts, you can list your globally installed packages:
npm list -g --depth=0
# Expected output:
# [List of globally installed npm packages]
Reviewing this list can help you spot potential conflicts. If you find a conflicting package, consider either updating it globally or removing it if it’s not needed.
Understanding npm’s Log Files
Npm’s log files are a treasure trove of information when it comes to diagnosing ‘npm err! code ELIFECYCLE’. After encountering the error, inspect the npm log for detailed error messages and clues:
cat npm-debug.log
# Expected output:
# [Detailed error log providing insights into what went wrong]
This command displays the contents of the npm-debug.log file, which contains detailed information about the error. Analyzing this log can pinpoint the exact issue, whether it’s a script failure, a permissions problem, or something else entirely.
By following these systematic troubleshooting steps—verifying dependencies, checking for global package conflicts, and understanding npm’s log files—you can navigate through and resolve ‘npm err! code ELIFECYCLE’ errors more effectively. Each step provides a deeper understanding of the underlying issues, guiding you towards a solution.
npm and Its Ecosystem Explained
To effectively navigate and resolve the ‘npm err! code ELIFECYCLE’, a deep dive into npm and its ecosystem is indispensable. npm, or Node Package Manager, is the cornerstone of managing packages in Node.js environments, facilitating the installation, updating, and management of packages (libraries or tools). One of its core features is the use of lifecycle scripts, which automate tasks such as starting, testing, and building applications.
Lifecycle Scripts in Depth
Lifecycle scripts are predefined commands in your package.json
that npm recognizes and can automatically execute at specific phases of your project lifecycle. For instance, prestart
, start
, and poststart
are scripts that can be executed before, during, and after the start
command, respectively. Here’s an example of setting a custom script:
"scripts": {
"greet": "echo 'Hello, World!'"
}
# Expected output:
# 'Hello, World!'
In this code block, we’ve added a custom script named greet
to our package.json
. When we run npm run greet
in the terminal, npm executes the command specified in the script, which in this case, prints ‘Hello, World!’ to the console. This demonstrates how npm lifecycle scripts can be used to automate tasks, enhancing productivity and streamlining project workflows.
Understanding npm lifecycle scripts and their execution order is crucial for debugging issues like ‘npm err! code ELIFECYCLE’. It allows developers to pinpoint where in the lifecycle a problem may occur and provides a framework for systematically resolving it. By grasping these fundamentals, you’re better equipped to troubleshoot errors and optimize your Node.js project’s development process.
Best Development Practices for npm
Mastering npm errors like ‘npm err! code ELIFECYCLE’ not only smoothens your development workflow but also unveils new avenues for automation and project efficiency. Understanding and resolving such errors is a stepping stone towards a more robust development practice. As you grow more comfortable with npm and its ecosystem, you’ll begin to see these errors less as roadblocks and more as opportunities to fine-tune your projects.
Automating with npm Scripts
One of the ways to leverage npm for better efficiency is through the use of npm scripts for automation. Beyond the basic lifecycle scripts, you can create custom scripts for a wide range of tasks, from linting your code to deploying your application. Here’s an example of a custom script for linting:
"scripts": {
"lint": "eslint . --fix"
}
# Expected output:
# [List of linting errors fixed or reported]
In this script, eslint . --fix
is used to automatically find and fix linting errors across your project. This not only ensures code quality but also saves time by automating a task that would otherwise be manual.
Enhancing Project Setup with npm
Another aspect of mastering npm involves optimizing your project setup. Utilizing npm init
and npm install
commands effectively can streamline the creation and configuration of new projects. Here’s an example demonstrating the use of npm init
to create a new project:
npm init -y
# Expected output:
# Wrote to /path/to/your/new/project/package.json:
# {
# "name": "your-new-project",
# "version": "1.0.0",
# ...
# }
This command quickly scaffolds a new project with a default package.json
, speeding up the project setup process.
Further Resources for Navigating npm Errors
To deepen your understanding and enhance your skills in managing npm and its related errors, consider exploring the following resources:
- npm Documentation: The official npm documentation provides comprehensive guides and references for npm commands, packages, and errors.
Node.js Guides: These guides offer insights into Node.js, covering topics from getting started to advanced concepts.
The npm Blog: Stay updated with the latest npm features, tips, and best practices by following the npm blog.
These resources serve as valuable tools in your journey towards mastering npm and enhancing your development workflow. By embracing the challenges and learning from errors like ‘npm err! code ELIFECYCLE’, you pave the way for a more efficient and error-resilient development process.
Recap: npm err! code Elifecycle Fix
In this thorough exploration, we’ve dissected the ‘npm err! code ELIFECYCLE’ error, a common stumbling block for developers working with npm and Node.js projects. From the initial confusion to a structured approach towards resolution, this guide aimed to equip you with the knowledge and tools necessary for troubleshooting this error.
We began with a foundational understanding of npm lifecycle scripts, illustrating how they form the backbone of task automation in your projects. By correctly setting up these scripts in your package.json
, you’re less likely to encounter lifecycle errors.
Next, we delved into common triggers of the ‘npm err! code ELIFECYCLE’ error, such as missing scripts, environmental mismatches, and dependency issues. Through practical examples, we demonstrated how to identify and rectify these problems, enhancing your project’s stability.
Our journey took us further into advanced troubleshooting techniques, including clean installations with npm ci
and the strategic use of environment variables. These expert-level strategies offer robust solutions in complex scenarios, ensuring your development process remains uninterrupted.
Strategy | Application | Effectiveness |
---|---|---|
Correct script setup | Prevents missing script errors | High |
Environmental adjustments | Solves version and compatibility issues | Medium to High |
Dependency management | Addresses corrupt or inconsistent packages | High |
As we conclude, remember that mastering npm and its intricacies, including the ‘npm err! code ELIFECYCLE’, not only smooths out your development process but also empowers you to leverage npm’s full potential for project efficiency and automation. This guide has laid out a comprehensive approach to understanding and resolving this error, paving the way for a more seamless and productive development experience.
With the insights and strategies shared, you’re now better equipped to tackle ‘npm err! code ELIFECYCLE’ and other npm-related challenges. Embrace these learnings to enhance your Node.js projects, ensuring a smoother, more efficient development journey. Happy coding!