Python Sorted Function: A Comprehensive Guide
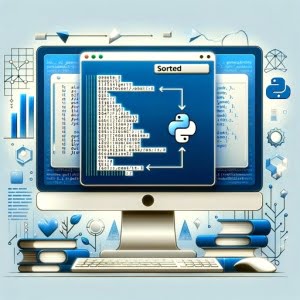
Ever felt overwhelmed trying to sort lists in Python? Just like a librarian organizing books, Python’s ‘sorted’ function can help you bring order to your data.
This guide will walk you through the ins and outs of using the ‘sorted’ function in Python. Whether you’re a beginner just starting out or an experienced coder looking to brush up on your skills, we’ve got you covered.
Let’s dive in and start bringing order to chaos with Python’s ‘sorted’ function!
TL;DR: How Do I Use the ‘sorted’ Function in Python?
The ‘sorted’ function in Python takes an iterable and returns a new sorted list of the elements. Here’s a simple example:
numbers = [5, 1, 9, 3]
sorted_numbers = sorted(numbers)
print(sorted_numbers)
# Output:
# [1, 3, 5, 9]
In the above example, we have a list of numbers which we sort using the ‘sorted’ function. The function returns a new list that contains the same elements but in ascending order.
For a more detailed understanding and advanced usage scenarios, continue reading.
Table of Contents
- Understanding the Basics of Python’s ‘sorted’ Function
- Advanced Sorting with Python’s ‘sorted’ Function
- Exploring Alternative Sorting Techniques in Python
- Troubleshooting Common Issues with Python’s ‘sorted’ Function
- Python’s Sorting Mechanism: Unveiling the ‘sorted’ Function
- The Power of Sorting in Data Analysis and Machine Learning
- Wrapping Up: Python’s ‘sorted’ Function
Understanding the Basics of Python’s ‘sorted’ Function
Python’s ‘sorted’ function is a built-in function that takes an iterable (like a list or a string) and returns a new sorted list of the elements. Here’s a simple example:
words = ['apple', 'banana', 'cherry']
sorted_words = sorted(words)
print(sorted_words)
# Output:
# ['apple', 'banana', 'cherry']
In this example, we have a list of words which we sort in alphabetical order using the ‘sorted’ function. The function returns a new list that contains the same elements but in ascending alphabetical order.
Sorting Different Types of Iterables
The ‘sorted’ function can also work with other types of iterables, such as tuples or strings. For instance:
my_tuple = (5, 1, 9, 3)
sorted_tuple = sorted(my_tuple)
print(sorted_tuple)
# Output:
# [1, 3, 5, 9]
In the above example, we used a tuple instead of a list. The ‘sorted’ function still returns a sorted list, even when provided with a tuple.
Advantages and Potential Pitfalls
The ‘sorted’ function is a powerful tool for sorting data in Python. However, it’s important to note that it always returns a new list, leaving the original iterable untouched. This can be an advantage if you want to preserve the original data, but it might lead to unexpected results if you’re not aware of this behavior. Also, remember that ‘sorted’ function sorts data in ascending order by default. If you want your data sorted in descending order, you’ll need to use additional parameters, which we’ll discuss in the next section.
Advanced Sorting with Python’s ‘sorted’ Function
Now that we’ve covered the basics, let’s delve into some more advanced uses of the ‘sorted’ function. Specifically, we’ll look at how to use the ‘key’ parameters and reverse sorting.
Sorting with Key Parameters
The ‘key’ parameter allows you to specify a function of one argument that is used to extract a comparison key from each element in the list.
For example, if you want to sort a list of strings by their length, you can pass the built-in len
function as the ‘key’ parameter:
words = ['apple', 'banana', 'cherry', 'date']
sorted_words = sorted(words, key=len)
print(sorted_words)
# Output:
# ['apple', 'date', 'banana', 'cherry']
In this example, the ‘sorted’ function sorts the list of words not by alphabetical order, but by their length, thanks to the ‘key=len’ parameter.
Reverse Sorting
By default, the ‘sorted’ function sorts the elements in ascending order. If you want to sort them in descending order, you can use the ‘reverse=True’ parameter:
numbers = [5, 1, 9, 3]
sorted_numbers = sorted(numbers, reverse=True)
print(sorted_numbers)
# Output:
# [9, 5, 3, 1]
In the above example, the ‘sorted’ function sorts the list of numbers in descending order, thanks to the ‘reverse=True’ parameter.
These are just a couple of the advanced features of the ‘sorted’ function in Python. By understanding and using these features, you can sort data in more complex and flexible ways.
Exploring Alternative Sorting Techniques in Python
Python offers a variety of ways to sort data beyond the ‘sorted’ function. Let’s explore some of these alternatives, including the ‘sort’ method, lambda functions, and third-party libraries.
Using the ‘sort’ Method
Python lists have a built-in method called ‘sort’ that sorts the list in-place. Unlike ‘sorted’, which returns a new list, ‘sort’ changes the original list:
numbers = [5, 1, 9, 3]
numbers.sort()
print(numbers)
# Output:
# [1, 3, 5, 9]
In the above example, the ‘sort’ method rearranges the elements of the original list in ascending order.
Leveraging Lambda Functions
If you want to sort a list of objects or a list of lists based on a specific attribute, you can use a lambda function as the key parameter:
fruits = [('apple', 3), ('banana', 2), ('cherry', 1)]
sorted_fruits = sorted(fruits, key=lambda fruit: fruit[1])
print(sorted_fruits)
# Output:
# [('cherry', 1), ('banana', 2), ('apple', 3)]
In this example, the ‘sorted’ function sorts a list of tuples based on the second element of each tuple, thanks to the ‘key=lambda’ parameter.
Utilizing Third-Party Libraries
For more complex sorting needs, you might want to use third-party libraries like NumPy or pandas. These libraries provide powerful data structures and functions for sorting data:
import numpy as np
numbers = np.array([5, 1, 9, 3])
sorted_numbers = np.sort(numbers)
print(sorted_numbers)
# Output:
# array([1, 3, 5, 9])
In the above example, we use the ‘np.sort’ function from the NumPy library to sort an array of numbers.
Each of these methods has its advantages and disadvantages. The ‘sorted’ function and ‘sort’ method are built-in and easy to use, but they might not be sufficient for more complex sorting needs. Lambda functions offer more flexibility, but they can be harder to read and understand. Third-party libraries are powerful and versatile, but they require additional installation and learning. Choose the method that best fits your specific needs.
Troubleshooting Common Issues with Python’s ‘sorted’ Function
While Python’s ‘sorted’ function is a powerful tool, it’s not without its quirks. Let’s discuss some common issues you might encounter when sorting data in Python, and how to resolve them.
Encountering TypeError During Sorting
One common issue is the TypeError that occurs when you try to sort a list containing different types of data. For instance, if you have a list containing both strings and integers, Python doesn’t know how to compare these different types and will raise a TypeError:
mixed_list = ['apple', 1, 'banana', 2]
try:
sorted_list = sorted(mixed_list)
except TypeError as e:
print(e)
# Output:
# '<' not supported between instances of 'int' and 'str'
In the above example, Python raises a TypeError because it doesn’t know how to compare a string (‘apple’) and an integer (1).
Solutions and Workarounds
To avoid this issue, you can either make sure that the list only contains comparable types, or provide a key function that can handle different types. For instance, you can convert all elements to strings before sorting:
mixed_list = ['apple', 1, 'banana', 2]
sorted_list = sorted(mixed_list, key=str)
print(sorted_list)
# Output:
# [1, 2, 'apple', 'banana']
In this example, we pass ‘str’ as the key function, which converts all elements to strings before comparing them. This way, Python can compare the elements and sort the list without raising a TypeError.
Remember, Python’s ‘sorted’ function is a powerful tool, but like all tools, it requires a bit of knowledge and finesse to use effectively. Keep these tips in mind, and you’ll be able to sort your data in Python with ease and confidence.
Python’s Sorting Mechanism: Unveiling the ‘sorted’ Function
Python’s ‘sorted’ function is built on top of a powerful sorting algorithm known as Timsort. Timsort is a hybrid sorting algorithm, derived from merge sort and insertion sort, designed to perform well on many kinds of real-world data. It was implemented by Tim Peters in 2002 for use in the Python programming language.
# Python's sorted function in action
numbers = [5, 1, 9, 3]
sorted_numbers = sorted(numbers)
print(sorted_numbers)
# Output:
# [1, 3, 5, 9]
In the example above, the ‘sorted’ function uses Timsort to sort a list of numbers in ascending order.
The Key Parameter: A Sorting Game-Changer
One of the most powerful features of the ‘sorted’ function is the ‘key’ parameter. This parameter lets you specify a function of one argument that is used to extract a comparison key from each input element. The key function transforms each element before comparison, it’s a way to tell Python how to sort the elements.
For example, if you want to sort a list of strings by their length, you can pass the built-in len
function as the ‘key’ parameter:
words = ['apple', 'banana', 'cherry']
sorted_words = sorted(words, key=len)
print(sorted_words)
# Output:
# ['apple', 'banana', 'cherry']
In this example, the ‘sorted’ function uses the length of each word (obtained by calling the len
function) as the comparison key. This way, it sorts the words by their length, not by their alphabetical order.
By understanding Python’s sorting algorithm and how the ‘key’ parameter works, you can harness the full power of the ‘sorted’ function and sort data in more complex and flexible ways.
The Power of Sorting in Data Analysis and Machine Learning
Sorting isn’t just for organizing lists or strings. It plays a vital role in data analysis, machine learning, and other fields where data manipulation and analysis are key. For instance, sorting can help identify patterns, make data easier to visualize, or speed up certain algorithms.
import numpy as np
import matplotlib.pyplot as plt
# Generate some random data
np.random.seed(0)
data = np.random.randn(1000)
# Sort the data
sorted_data = np.sort(data)
# Plot the sorted data
plt.plot(sorted_data)
plt.title('Plot of Sorted Random Data')
plt.show()
# Output: A plot of sorted random data
In the above example, we generate a thousand random numbers using NumPy, sort them, and then plot them. The sorted data reveals a clear pattern that would be hard to see in the unsorted data.
Exploring Related Concepts
If you’re interested in Python’s ‘sorted’ function, you might also want to explore related concepts like list comprehensions and lambda functions. These features can make your code more concise and expressive.
# List comprehension with 'sorted'
squared_sorted_numbers = [n**2 for n in sorted([5, 1, 9, 3])]
print(squared_sorted_numbers)
# Output:
# [1, 9, 25, 81]
In this example, we use a list comprehension to square each number in a sorted list. The result is a new list of squared numbers in ascending order.
Finding More Resources
If you’re eager to dive deeper into Python functions and their potential applications, the following resources could prove invaluable:
- Python Built-In Functions for Efficient Coding – Discover the utility of Python’s built-in functions for error handling.
Iterable Transformation with map() in Python – Dive into functional programming and element-wise transformations with “map.”
Python min() Function: Finding the Minimum Value – Python’s “min” function for finding the smallest element in a sequence.
Educba’s Guide to Python’s Built-in Functions – Delve into Python’s wide array of built-in functions with Educba’s extensive guide.
JavaTpoint’s Comprehensive Guide on Python Built-in Functions explains Python’s built-in functions.
Python’s Official Data Model Documentation – Offers info on Python’s data model and related concepts.
These selected materials will help enhance your understanding of Python functions, ultimately fostering your growth as a skilled Python programmer.
Wrapping Up: Python’s ‘sorted’ Function
In this guide, we delved into the depths of Python’s ‘sorted’ function, exploring its capabilities, usage, and how it fits into Python’s data manipulation toolkit. We saw how the ‘sorted’ function can handle different types of iterables, from lists to tuples, and even strings.
numbers = [5, 1, 9, 3]
sorted_numbers = sorted(numbers)
print(sorted_numbers)
# Output:
# [1, 3, 5, 9]
In the example above, we sorted a list of numbers in ascending order using the ‘sorted’ function. This is the basic usage of the function, which can be extended with the ‘key’ and ‘reverse’ parameters for more advanced sorting needs.
We also discussed common issues you might encounter when using the ‘sorted’ function, such as TypeError when sorting different types of data together. We provided solutions and workarounds for these issues, helping you to avoid common pitfalls.
Beyond the ‘sorted’ function, we explored alternative sorting techniques, such as the ‘sort’ method, lambda functions, and third-party libraries like NumPy. Each of these methods has its advantages and disadvantages, offering different ways to sort data in Python.
In conclusion, Python’s ‘sorted’ function is a powerful tool for sorting data. Whether you’re a beginner just starting out or an experienced coder looking to brush up on your skills, understanding this function can greatly enhance your data manipulation capabilities in Python.