Python map() | Function Guide (With Examples)
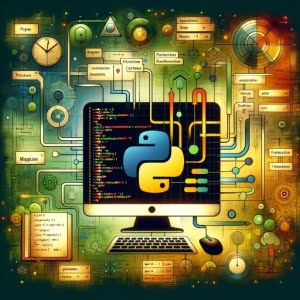
Ever found yourself caught up in the tedious task of transforming a list of values in Python? You might have been writing a loop, line after line, just to apply a simple operation to each item. Well, Python has a magical tool that can simplify this task for you – the map function.
The map function in Python is like a magician with a wand. It can transform your data in a single, elegant line of code. This guide will walk you through the ins and outs of using Python’s map function, from basic usage to advanced techniques.
Whether you’re a beginner just starting out with Python, or an experienced developer looking to refine your skills, this guide will serve as a comprehensive resource for mastering the map function. So, let’s jump right in and start transforming data with Python’s map function!
TL;DR: How do I use the Python map function?
The map function in Python applies a given function to each item of an iterable (such as a list) and returns a list of the results.
Here’s a simple example:
numbers = [1, 2, 3, 4]
squares = list(map(lambda x: x ** 2, numbers))
print(squares)
# Output:
# [1, 4, 9, 16]
In this example, the map function applies the lambda function lambda x: x ** 2
to each item in the list numbers
. The lambda function takes an input x
and returns the square of x
. The result is a new list squares
where each item is the square of the corresponding item in numbers
.
Dive deeper into this guide to learn more about how to use the map function in Python, including advanced techniques and alternative approaches.
Table of Contents
Mastering the Basics: Python Map Function
The map function in Python is a built-in function that allows you to process and transform all the items in an iterable without using an explicit for loop, a technique commonly known as mapping. The map function is one of the foundations of functional programming.
Let’s break it down. The map function takes two arguments: a function and an iterable. The function is applied to all the items in the iterable. Here’s a simple example:
# Define a list of numbers
numbers = [1, 2, 3, 4, 5]
# Define a simple function
def square(number):
return number ** 2
# Use the map function
squares = list(map(square, numbers))
print(squares)
# Output:
# [1, 4, 9, 16, 25]
In this example, the map function applies the square
function to each item in the list numbers
. The result is a new list squares
where each item is the square of the corresponding item in numbers
.
Using Lambda Functions
In Python, a lambda function is an anonymous function, meaning it is a function without a name. Lambda functions are often used with the map function. Here’s how you can use a lambda function with the map function:
# Define a list of numbers
numbers = [1, 2, 3, 4, 5]
# Use the map function with a lambda function
squares = list(map(lambda x: x ** 2, numbers))
print(squares)
# Output:
# [1, 4, 9, 16, 25]
In this example, the lambda function lambda x: x ** 2
does the same thing as the square
function in the previous example. It takes an input x
and returns the square of x
.
Advantages and Pitfalls
The map function is a powerful tool that can make your code more readable and easier to understand. It allows you to process all the items in an iterable in a concise and efficient way.
However, keep in mind that the map function returns a map object, which is an iterable, but not a list or a tuple. If you want to get a list or a tuple, you need to convert the map object using the list or tuple function, as shown in the examples.
Stay tuned for more advanced uses of the map function in Python!
Python Map Function: Leveling Up
As you become more familiar with Python’s map function, you can start to explore its more advanced capabilities. One such feature is the ability to use the map function with multiple iterables. This allows you to apply a function to multiple lists (or other iterables) at once.
Let’s look at an example where we add the corresponding elements of two lists:
# Define two lists of numbers
numbers1 = [1, 2, 3, 4, 5]
numbers2 = [6, 7, 8, 9, 10]
# Use the map function with a lambda function
result = list(map(lambda x, y: x + y, numbers1, numbers2))
print(result)
# Output:
# [7, 9, 11, 13, 15]
In this example, the lambda function lambda x, y: x + y
takes two inputs x
and y
and returns their sum. The map function applies this lambda function to the corresponding items in numbers1
and numbers2
.
Complex Functions with Map
The map function is not limited to simple functions like addition or squaring. You can use complex functions as well, including functions that call other functions. Here’s an example:
# Define a list of strings
words = ['hello', 'world', 'python', 'map']
# Define a complex function
def reverse_upper(word):
return word[::-1].upper()
# Use the map function
result = list(map(reverse_upper, words))
print(result)
# Output:
# ['OLLEH', 'DLROW', 'NOHTYP', 'PAM']
In this example, the reverse_upper
function takes a string word
, reverses it using the slicing syntax word[::-1]
, and converts it to uppercase using the upper
method. The map function applies this function to each item in words
, resulting in a new list where each word is reversed and converted to uppercase.
Best Practices
When using the map function, it’s important to keep a few best practices in mind. First, try to keep your functions simple. If a function becomes too complex, it can be difficult to understand what the map function is doing. In such cases, it might be better to use a for loop or a list comprehension.
Second, remember that the map function returns a map object. If you need a list or a tuple, you need to convert the map object using the list or tuple function. If you don’t need to use the result as a list or a tuple, you can iterate over the map object directly.
Stay tuned for more ways to handle data transformation tasks in Python, including alternative approaches to the map function!
Exploring Alternatives to Python’s Map Function
While Python’s map function is a powerful tool, there are other techniques for data transformation that you might find more suitable depending on the situation. In this section, we will explore two popular alternatives: list comprehensions and for loops.
Python List Comprehensions
List comprehensions provide a concise way to create lists based on existing lists. Here’s how you can use a list comprehension to square a list of numbers:
numbers = [1, 2, 3, 4, 5]
squares = [x ** 2 for x in numbers]
print(squares)
# Output:
# [1, 4, 9, 16, 25]
In this example, the list comprehension [x ** 2 for x in numbers]
does the same thing as list(map(lambda x: x ** 2, numbers))
. It creates a new list where each item is the square of the corresponding item in numbers
.
Python For Loops
For loops are the most straightforward way to process all the items in a list. Here’s how you can use a for loop to square a list of numbers:
numbers = [1, 2, 3, 4, 5]
squares = []
for x in numbers:
squares.append(x ** 2)
print(squares)
# Output:
# [1, 4, 9, 16, 25]
In this example, the for loop for x in numbers: squares.append(x ** 2)
does the same thing as list(map(lambda x: x ** 2, numbers))
. It creates a new list squares
where each item is the square of the corresponding item in numbers
.
Comparison of Methods
Method | Advantages | Disadvantages |
---|---|---|
Map function | Concise and readable for simple functions | Not Pythonic, requires conversion to list or tuple |
List comprehension | Pythonic, concise and readable, automatically returns a list | Can be slow for large lists |
For loop | Most flexible, can handle complex logic | Verbose, can be slow for large lists |
While the map function, list comprehensions, and for loops can all be used to transform data in Python, the best method to use depends on your specific needs. If you have a simple function and you’re comfortable with functional programming concepts, the map function can be a great choice. If you prefer a more Pythonic approach and your function is still relatively simple, a list comprehension can be the way to go. If you have more complex logic, a for loop might be the best option. As always, it’s important to consider the readability and performance of your code when choosing a method.
Troubleshooting Python’s Map Function
While Python’s map function is a powerful tool, it’s not without its quirks. In this section, we’ll cover some common issues you might encounter and provide solutions and workarounds.
Handling Type Errors
One common issue is a TypeError, which occurs when you try to apply a function to an item of an incompatible type. For example, if you try to apply a function that operates on strings to a list of numbers, you’ll get a TypeError.
Here’s an example:
# Define a list of numbers
numbers = [1, 2, 3, 4, 5]
# Try to use the map function with a string function
result = list(map(str.upper, numbers))
# Output:
# TypeError: descriptor 'upper' requires a 'str' object but received a 'int'
In this example, the str.upper
function expects a string, but it receives an integer from the list numbers
. To fix this issue, you need to ensure that the function and the items in the iterable are of compatible types.
Handling Empty Iterables
Another common issue is handling empty iterables. If you apply the map function to an empty iterable, it will return an empty map object. If you convert this map object to a list or a tuple, you’ll get an empty list or tuple.
Here’s an example:
# Define an empty list
numbers = []
# Use the map function
result = list(map(lambda x: x ** 2, numbers))
print(result)
# Output:
# []
In this example, the map function returns an empty list because the input list numbers
is empty. This is the expected behavior and not an issue in most cases. However, if your code doesn’t handle empty lists correctly, it might cause issues down the line.
Tips and Best Practices
When using the map function, it’s important to keep a few best practices in mind. First, always ensure that the function and the items in the iterable are of compatible types. Second, be prepared to handle empty iterables. Finally, remember that the map function returns a map object, not a list or a tuple. If you need a list or a tuple, you need to convert the map object using the list or tuple function.
Stay tuned for more insights into Python’s functional programming features!
Python’s Functional Programming and Mapping Concept
Python, although not purely functional, incorporates several elements from functional programming. This paradigm emphasizes the use of pure functions, which return outputs solely based on their inputs without affecting or depending on the state of the program. This approach results in code that’s easier to test and debug.
Mapping is a core concept in functional programming. It involves applying a function to each item in a collection, such as a list or a tuple, and creating a new collection with the results.
# Define a list of numbers
numbers = [1, 2, 3, 4, 5]
# Use the map function to square each number
squares = list(map(lambda x: x ** 2, numbers))
print(squares)
# Output:
# [1, 4, 9, 16, 25]
In this example, the map function applies the lambda function lambda x: x ** 2
to each item in numbers
, resulting in a new list squares
where each item is the square of the corresponding item in numbers
.
Demystifying Lambda Functions and Iterables
Lambda functions, also known as anonymous functions, are small, nameless functions defined with the lambda
keyword. They are useful when you need a short, one-time function that you won’t reuse elsewhere in your code.
Iterables, on the other hand, are objects that you can iterate over. In Python, lists, tuples, strings, dictionaries, sets, and more are all iterable. You can process each item in an iterable individually, either by using a loop or by using functions like map
.
In the context of the map
function, the iterable is the collection of items that you want to process, and the lambda function (or any other function) is the operation that you want to apply to each item.
By understanding these fundamental concepts, you can better appreciate the power and utility of Python’s map
function. Stay tuned for more insights into Python’s functional programming features!
The Map Function: Beyond Basic Python
The map function is not just a tool for basic Python programming. It’s a powerful function that plays a crucial role in data processing and machine learning, where handling large datasets efficiently is key.
In data processing, the map function allows you to transform data quickly and efficiently. For example, you might need to normalize a list of numerical values or clean a list of text strings. The map function allows you to apply these transformations in a single line of code.
In machine learning, the map function can be used to preprocess data, such as scaling numerical features or encoding categorical features. The map function can also be used to apply transformations to the output of a machine learning model, such as converting class probabilities to class labels.
# Example of using the map function in data preprocessing
# Define a list of numbers
numbers = [1, 2, 3, 4, 5]
# Define a normalization function
def normalize(number):
return (number - min(numbers)) / (max(numbers) - min(numbers))
# Use the map function to normalize the numbers
normalized_numbers = list(map(normalize, numbers))
print(normalized_numbers)
# Output:
# [0.0, 0.25, 0.5, 0.75, 1.0]
In this example, the map function applies the normalize
function to each item in numbers
, resulting in a new list normalized_numbers
where each item is the normalized value of the corresponding item in numbers
.
Exploring Related Concepts: Filter and Reduce Functions
If you find the map function useful, you might want to explore related concepts in Python’s functional programming toolbox. The filter function is similar to map, but it filters items out of an iterable based on a condition. The reduce function, meanwhile, applies a function to an iterable in a cumulative way.
Further Resources for Python Functions
For a deeper understanding of Python related topics, here are a few available online resources:
- Python Built-In Functions: A Quick Overview – Learn how to use built-in functions for sorting and searching algorithms.
Function Definitions with Python Lambda – Learn how to use “lambda” for on-the-fly function definitions in Python.
Python sorted() Function: Sorting Data with Ease – Explore Python’s “sorted” function for sorting sequences and collections.
Guide on Python’s Reduce Method – Learn how to use the reduce method in Python with this guide from Educative.
Python’s Official Documentation on functools Module – Dive into Python’s functools library for working with functions and callable objects.
W3Schools’ Reference on Python’s filter Function offers examples and detailed explanations on the filter function.
Remember, the best way to learn is by doing, so don’t hesitate to experiment with these functions in your own code!
Python Map Function: A Recap
Throughout this comprehensive guide, we’ve explored the power and versatility of Python’s map function. From its basic usage to more advanced techniques, we’ve seen how this function can transform data in Python with elegance and efficiency.
We’ve learned how to use map with both predefined and lambda functions, and how it can handle multiple iterables and complex functions. We’ve also discussed common issues such as TypeErrors and handling of empty iterables, providing solutions and workarounds for each.
In addition to the map function, we’ve explored alternative approaches like list comprehensions and for loops. Each method has its own advantages and is best suited to different situations.
Method | Advantages | Disadvantages |
---|---|---|
Map function | Concise and readable for simple functions | Requires conversion to list or tuple |
List comprehension | Pythonic, concise and readable, automatically returns a list | Can be slow for large lists |
For loop | Most flexible, can handle complex logic | Verbose, can be slow for large lists |
In the end, the best method depends on your specific needs and the nature of your data. Whether you choose to use the map function, a list comprehension, or a for loop, Python offers a variety of powerful tools for data transformation.
Finally, we’ve seen how the map function extends beyond basic Python programming, playing a crucial role in data processing and machine learning. With Python’s map function, you’re well-equipped to handle any data transformation task that comes your way.