Method Overriding in Java: In-Depth Tutorial
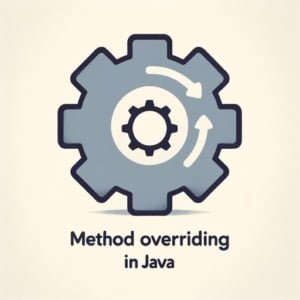
Are you finding it challenging to grasp the concept of method overriding in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling method overriding, but we’re here to help.
Think of method overriding in Java as a skilled actor taking on different roles. A method in Java can take on different forms in subclasses, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of mastering method overriding in Java, from its basic usage to more advanced techniques, as well as alternative approaches. We’ll cover everything from the basics of method overriding to more complex scenarios, along with common issues and their solutions.
Let’s dive in and start mastering method overriding in Java!
TL;DR: What is Method Overriding in Java?
Method overriding in Java is when a subclass provides a specific implementation of a method that is already provided by its parent class. This allows the subclass to inherit the methods of the parent class and modify them as needed.
Here’s a simple example:
class Parent {
void show() {
System.out.println('Parent's show()');
}
}
class Child extends Parent {
void show() {
System.out.println('Child's show()');
}
}
// Create an instance of Child
Child child = new Child();
// Call the show method
child.show();
// Output:
// 'Child's show()'
In this example, we have a Parent
class with a show()
method, and a Child
class that extends the Parent
class and overrides the show()
method. When we create an instance of Child
and call the show()
method, the overridden method in the Child
class is executed, not the one in the Parent
class.
This is a basic example of method overriding in Java, but there’s much more to learn about this powerful feature. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Unraveling Method Overriding in Java
- Diving Deeper into Method Overriding
- Exploring Alternatives: Method Overloading vs Method Overriding
- Navigating Troubles with Method Overriding
- Understanding Inheritance: The Foundation of Method Overriding
- Object-Oriented Principles and Method Overriding
- Method Overriding in the Real World
- Wrapping Up: Method Overriding in Java
Unraveling Method Overriding in Java
Method overriding in Java is a core concept of object-oriented programming that allows a subclass to provide a different implementation of a method that is already provided by its parent class. This is a powerful feature that allows developers to write flexible and maintainable code.
Let’s consider a basic example to understand how method overriding works in Java:
class Animal {
void sound() {
System.out.println('Animals make sounds');
}
}
class Dog extends Animal {
void sound() {
System.out.println('Dogs bark');
}
}
// Create an instance of Dog
Dog dog = new Dog();
// Call the sound method
dog.sound();
// Output:
// 'Dogs bark'
In this example, the Animal
class has a method named sound()
. The Dog
class, which is a subclass of Animal
, overrides the sound()
method. When we create an instance of Dog
and call the sound()
method, the overridden method in the Dog
class is executed, not the one in the Animal
class.
Advantages of Method Overriding
Method overriding provides several benefits:
- Code Reusability: We can use the method from the parent class and modify it in the subclass as needed, which promotes code reusability.
- Flexibility: It allows subclasses to behave differently. In our example, different animal classes can provide different implementations of the
sound()
method. - Abstraction: The user does not need to know which method implementation is being used. They can simply call the
sound()
method on an animal object, and the correct method will be executed.
Potential Pitfalls
While method overriding is a powerful feature, it’s important to be aware of potential pitfalls:
- Method Signature: The method in the subclass must have the exact same name, return type, and parameters as the method in the parent class. If these do not match, the method will not be overridden.
- Access Level: The access level cannot be more restrictive in the subclass. For example, if the method in the parent class is
public
, the overridden method in the subclass cannot beprivate
orprotected
.
Understanding these basics will set the foundation for further exploration into the world of method overriding in Java.
Diving Deeper into Method Overriding
As you become more comfortable with the concept of method overriding in Java, it’s time to delve into its more complex uses. Let’s discuss when to use the @Override annotation, how method overriding works with access modifiers, and how it interacts with exception handling.
The @Override Annotation
The @Override annotation is a handy tool in Java that ensures that a method is actually overriding a method from a superclass. If it’s not, the compiler will throw an error.
Here’s an example:
class Animal {
void sound() {
System.out.println('Animals make sounds');
}
}
class Dog extends Animal {
@Override
void sound() {
System.out.println('Dogs bark');
}
}
// Create an instance of Dog
Dog dog = new Dog();
// Call the sound method
dog.sound();
// Output:
// 'Dogs bark'
In this example, the sound()
method in the Dog
class is annotated with @Override, which tells the compiler that the method should override a method in the superclass. If the sound()
method in the Animal
class didn’t exist or had a different method signature, the compiler would throw an error.
Method Overriding and Access Modifiers
When overriding methods in Java, the access level can’t be more restrictive in the subclass. For example, if the method in the parent class is public
, the overridden method in the subclass can’t be private
or protected
. However, it can be public
.
Method Overriding and Exception Handling
When it comes to exception handling, the overridden method in the subclass can’t throw a broader checked exception than the method in the parent class. However, it can throw narrower checked exceptions, any unchecked exceptions, or no exceptions at all.
By understanding these advanced concepts, you’ll be able to utilize method overriding in Java more effectively and write more flexible and maintainable code.
Exploring Alternatives: Method Overloading vs Method Overriding
While method overriding is a powerful feature in Java, it’s not the only way to modify the behavior of methods. Another related concept is method overloading, which has its own unique uses and benefits. Understanding the differences between these two concepts can help you choose the right tool for the job.
What is Method Overloading?
Method overloading in Java occurs when two or more methods in the same class have the same name but different parameters. This allows multiple methods to perform similar tasks but with different input types or numbers of inputs.
Here’s an example:
class Calculator {
int add(int a, int b) {
return a + b;
}
int add(int a, int b, int c) {
return a + b + c;
}
}
// Create an instance of Calculator
Calculator calculator = new Calculator();
// Call the add method
System.out.println(calculator.add(2, 3)); // Output: 5
System.out.println(calculator.add(2, 3, 4)); // Output: 9
In this example, the Calculator
class has two add()
methods. One takes two parameters and the other takes three. When we call the add()
method with two or three arguments, the appropriate method is executed.
Comparing Method Overloading and Method Overriding
While both method overloading and method overriding allow us to modify the behavior of methods, they serve different purposes and have their own benefits and drawbacks:
- Method Overriding allows a subclass to provide a different implementation of a method that is already provided by its parent class. This is useful when we want subclasses to share a common interface but behave differently.
Method Overloading allows a class to have multiple methods with the same name but different parameters. This is useful when we want a method to perform similar tasks but with different types or numbers of inputs.
Understanding these differences can help you make more informed decisions when designing your classes and methods in Java.
As with any programming concept, method overriding in Java can present its own set of challenges. Let’s go through some common errors you might encounter and how to solve them.
Incorrect Method Signature
One common error is incorrect method signature. Remember, the method in the subclass must have the exact same name, return type, and parameters as the method in the parent class. If these do not match, the method will not be overridden, and the superclass’s method will be called instead.
Consider this example:
class Parent {
void display() {
System.out.println('Parent's display()');
}
}
class Child extends Parent {
void display(int num) {
System.out.println('Child's display() ' + num);
}
}
// Create an instance of Child
Child child = new Child();
// Call the display method
child.display();
// Output:
// 'Parent's display()'
In this example, we intended to override the display()
method in the Child
class, but we added an extra parameter. This changes the method signature, and so the display()
method in the Parent
class is called instead.
More Restrictive Access Level
Another common error is making the access level of the overridden method more restrictive. The access level can’t be more restrictive in the subclass. For example, if the method in the parent class is public
, the overridden method in the subclass can’t be private
or protected
.
Here’s an example that demonstrates this error:
class Parent {
public void show() {
System.out.println('Parent's show()');
}
}
class Child extends Parent {
private void show() {
System.out.println('Child's show()');
}
}
// This code will not compile because
// the show() method in the Child class
// is more restrictive than in the Parent class.
In this example, the show()
method in the Child
class is private
, which is more restrictive than the public
show()
method in the Parent
class. This code will not compile.
Best Practices and Optimization
When using method overriding in Java, here are some best practices to keep in mind:
- Use the @Override annotation: This helps catch errors at compile time if a method intended to override a superclass’s method does not do so correctly.
- Follow the Liskov Substitution Principle (LSP): Subclasses should be substitutable for their superclass without causing issues. This means the behavior of the overridden method should not contradict the behavior of the superclass’s method.
- Don’t override methods marked as final: The
final
keyword in Java is used to restrict users from changing the value (for variables), changing the class (for classes), or overriding the method (for methods).
By understanding these common errors and best practices, you can avoid pitfalls and utilize method overriding in Java more effectively.
Understanding Inheritance: The Foundation of Method Overriding
Before we can fully grasp the concept of method overriding in Java, we need to understand the principle of inheritance, which is fundamental to method overriding.
Inheritance in Java is a mechanism where one class acquires the properties (fields and methods) of another. With the use of inheritance, information is managed in a hierarchical order. The class which inherits the properties of another is known as the subclass (or derived class), and the class whose properties are inherited is known as the superclass (or base class).
Here’s a simple example:
class Animal {
void eat() {
System.out.println('Eating...');
}
}
class Dog extends Animal {
}
// Create an instance of Dog
Dog dog = new Dog();
// Call the eat method
dog.eat();
// Output:
// 'Eating...'
In this example, the Dog
class inherits from the Animal
class. This means that a Dog
object can use the eat()
method defined in the Animal
class. Inheritance is a way to promote code reuse and is a key part of object-oriented programming.
Object-Oriented Principles and Method Overriding
Method overriding is a perfect example of the ‘Polymorphism’ principle in object-oriented programming. Polymorphism allows objects of different classes to be treated as objects of a common superclass. With method overriding, a subclass can provide a different implementation of a method that is already defined in its superclass, which allows the same method call to behave differently depending on whether it’s called on an object of the superclass or an object of the subclass.
By understanding inheritance and the principles of object-oriented programming, you can see how method overriding fits into the bigger picture of Java programming.
Method Overriding in the Real World
Method overriding in Java is not just a theoretical concept, it’s a practical tool that developers use in real-world applications. Let’s explore how method overriding can be used to create customizable software components and implement polymorphism in Java.
Customizable Software Components
In software development, it’s common to have components that share a lot of common features but also have some specific behaviors. By using method overriding, you can create a base class with all the common features and then create subclasses that override specific methods to customize their behavior.
For example, consider a software for a library. You might have a Book
class with methods like borrow()
, return()
, and renew()
. If you want to introduce different types of books, like RegularBook
and ReferenceBook
, you can create these as subclasses of Book
and override the methods as needed. For instance, ReferenceBook
might override the borrow()
method because reference books can’t be borrowed.
Implementing Polymorphism
Polymorphism is a core concept in object-oriented programming that allows objects to take on many forms. Method overriding is a key way to implement polymorphism in Java. By overriding methods, subclasses can provide their own unique behaviors while still being treated as instances of the superclass.
For example, consider a Shape
class with a draw()
method. You can create subclasses like Circle
, Square
, and Triangle
, each overriding the draw()
method to draw the appropriate shape. When you call the draw()
method on a Shape
reference, the correct method will be called based on the actual object type, even though the reference type is Shape
. This is polymorphism in action.
Further Resources for Method Overriding
If you’re interested in learning more about method overriding in Java, here are some resources that you might find helpful:
- Importance of Java Methods in Programming – Gain practical insights into Java methods for effective coding.
Understanding Method Declaration in Java – Explore Java method signatures for specifying method names, return types, and parameters.
Exploring Java Main Method Signature – Tthe anatomy of the public static void main(String[] args) method in Java.
Oracle Java Documentation provides a thorough explanation of method overriding.
Geeks for Geeks Java Method Overriding offers a detailed guide on method overriding in Java.
JavaTpoint Method Overriding in Java goes over method overriding complete with diagrams and examples.
Wrapping Up: Method Overriding in Java
In this comprehensive guide, we’ve journeyed through the concept of method overriding in Java, a fundamental feature in object-oriented programming that allows a subclass to provide a different implementation of a method that is already provided by its parent class.
We began with the basics, clarifying what method overriding is and providing simple examples for beginners. We then ventured into more advanced territory, discussing the use of the @Override annotation, how method overriding works with access modifiers, and its interaction with exception handling. Along the way, we tackled common challenges you might face when using method overriding, such as incorrect method signature and more restrictive access level, providing you with solutions for each issue.
We also looked at alternative approaches to method modification, comparing method overriding with method overloading. Here’s a quick comparison of these concepts:
Concept | Description | Use Case |
---|---|---|
Method Overriding | Allows a subclass to provide a different implementation of a method from its parent class | When you want subclasses to share a common interface but behave differently |
Method Overloading | Allows a class to have multiple methods with the same name but different parameters | When you want a method to perform similar tasks but with different types or numbers of inputs |
Whether you’re just starting out with method overriding or you’re looking to level up your Java programming skills, we hope this guide has given you a deeper understanding of method overriding and its capabilities.
With its ability to allow subclasses to behave differently and promote code reusability, method overriding is a powerful tool in Java programming. Happy coding!