Java Method Signature: Understanding and Usage Guide
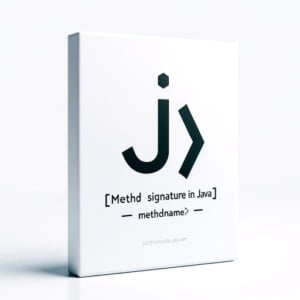
Ever felt like you’re wrestling with understanding method signatures in Java? You’re not alone. Many developers find the concept of method signatures a bit daunting. Think of method signatures as a unique identifier – a tool that helps Java distinguish between methods.
Method signatures are a powerful way to ensure the correct functioning of your Java programs, making them extremely popular for method declaration and overloading.
This guide will walk you through the concept of method signatures in Java, from the basics to more advanced topics. We’ll cover everything from understanding what a method signature is, its role in method declaration, to dealing with common issues and their solutions.
Let’s kick things off and learn about method signatures in Java!
TL;DR: What is a Method Signature in Java?
In Java, a method signature is a part of the method declaration. It’s the combination of the method name and the parameter list, for example
public void sampleMethod(int a, String b)
, with the Method Signature beingsampleMethod(int, String)
. The method signature is used by Java to differentiate methods.
Here’s a simple example:
public void myMethod(int a, String b) {
// method body
}
// Output:
// This declares a method with the signature 'myMethod(int, String)'
In this example, ‘myMethod’ is the method name, and ‘(int a, String b)’ is the parameter list. Together, they form the method signature: ‘myMethod(int, String)’. This signature is unique to this method and allows Java to distinguish it from other methods.
This is just the tip of the iceberg when it comes to understanding method signatures in Java. Continue reading for a more in-depth exploration and advanced usage scenarios.
Table of Contents
- Method Signature in Java: The Basics
- Method Overloading: Advanced Use of Method Signature
- Exploring Method Overriding and Overloading
- Navigating Common Issues with Method Signatures
- Deep Dive into Java Methods and Method Signatures
- The Role of Method Signatures in Object-Oriented Programming
- Wrapping Up: Method Signatures in Java
Method Signature in Java: The Basics
A method signature in Java is a unique identifier that consists of the method name and the parameter list. It’s a critical component in method declaration and plays a key role in how Java differentiates between methods.
Components of a Method Signature
A method signature is made up of two main components:
- Method Name: This is the name you assign to the method. It should be descriptive and follow Java’s naming conventions.
Parameter List: This is the list of parameters that your method accepts. Parameters are declared by specifying their data type followed by their name. Multiple parameters are separated by commas.
Here’s a simple example to illustrate these components:
public void addNumbers(int a, int b) {
int sum = a + b;
System.out.println("The sum is " + sum);
}
// Output:
// This declares a method with the signature 'addNumbers(int, int)'
In this example, ‘addNumbers’ is the method name, and ‘(int a, int b)’ is the parameter list. Together, they form the method signature: ‘addNumbers(int, int)’.
Role in Method Declaration
In Java, method signatures play a crucial role in method declaration. When you declare a method, you’re required to provide a method signature. This helps Java identify and call the correct method when it’s needed.
Advantages and Potential Pitfalls
Method signatures in Java come with several advantages. They make your code easier to read and understand, and they allow Java to differentiate between methods, even if they have the same name (method overloading).
However, there are potential pitfalls to watch out for. One common mistake is creating methods with the same signature within the same class, which Java does not allow. This will result in a compilation error.
Remember, a method’s signature is determined by the method name and the type and order of its parameters, not its return type. So, two methods with the same name and parameter list but different return types will still clash.
In the next section, we’ll dive into more advanced uses of method signatures in Java, including method overloading.
Method Overloading: Advanced Use of Method Signature
Method overloading is an advanced use of method signatures in Java. It allows you to define multiple methods with the same name but different parameter lists. The different parameter lists mean that the methods will have different signatures, even though their names are the same.
Method Overloading in Action
Let’s take a look at an example of method overloading:
public void greet() {
System.out.println("Hello, User!");
}
public void greet(String name) {
System.out.println("Hello, " + name + "!");
}
// Output:
// When calling greet(): 'Hello, User!'
// When calling greet("Alice"): 'Hello, Alice!'
In this example, we have two methods named ‘greet’. The first method doesn’t take any parameters, and the second method takes one parameter of type String. Even though the method names are the same, the method signatures are different due to the different parameter lists. This is method overloading.
Importance of Method Signatures in Method Overloading
Method signatures play a crucial role in method overloading. When a method is invoked, Java uses the method signature to determine which version of the overloaded method to call. If the method signatures weren’t unique, Java wouldn’t be able to differentiate between the methods.
Best Practices for Method Overloading
When using method overloading, it’s important to ensure that each overloaded method performs a similar function, with the differences being due to the parameters. This makes your code easier to read and understand. Also, remember that method overloading is determined by the method name and the type and order of parameters, not the return type.
Exploring Method Overriding and Overloading
As you advance in Java, you’ll encounter concepts that extend the use of method signatures beyond basic applications. Two such concepts are method overloading and method overriding.
Method Overloading Revisited
As we discussed, method overloading allows you to define multiple methods with the same name but different parameter lists or types. This is possible because of the uniqueness of method signatures. Let’s look at an additional example:
public void display(int a) {
System.out.println("The integer is " + a);
}
public void display(String b) {
System.out.println("The string is " + b);
}
// Output:
// When calling display(5): 'The integer is 5'
// When calling display("Alice"): 'The string is Alice'
In this example, we have two methods named ‘display’. One takes an integer parameter and the other takes a string. The method signatures are ‘display(int)’ and ‘display(String)’, respectively.
Method Overriding: A New Concept
Method overriding is another concept where method signatures play a critical role. In method overriding, a subclass provides a specific implementation of a method that is already provided by its parent class. Java uses method signatures to determine which method to invoke at runtime.
Here’s an example:
class Animal {
void sound() {
System.out.println("The animal makes a sound");
}
}
class Cat extends Animal {
void sound() {
System.out.println("The cat meows");
}
}
// Output:
// When calling sound() on an Animal object: 'The animal makes a sound'
// When calling sound() on a Cat object: 'The cat meows'
In this example, the ‘sound’ method in the ‘Cat’ class overrides the ‘sound’ method in the ‘Animal’ class. Both methods have the same signature, but the ‘Cat’ class provides its own implementation.
Advantages and Disadvantages
Both method overloading and method overriding have their advantages. They increase the readability and reusability of code and allow you to take advantage of polymorphism in Java. However, they can also increase the complexity of code, and incorrect use can lead to errors that are hard to detect.
Recommendations
When using method overloading and overriding, make sure the methods perform similar functions. This keeps your code consistent and easy to understand. Also, be aware of the potential for errors and test your code thoroughly.
While method signatures are a powerful tool in Java, they can also lead to some common issues. Let’s discuss these problems and provide some practical solutions and tips.
Method Signature Clashes
One common issue is method signature clashes. This occurs when two methods in the same class have the same signature (i.e., the same method name and parameter list). Remember, Java doesn’t allow two methods with the same signature in the same class, even if they have different return types.
Here’s an example of a method signature clash:
public void add(int a, int b) {
// method body
}
public int add(int a, int b) {
// method body
}
// Output:
// Compilation error: method add(int,int) is already defined in class MyClass
In this example, the two ‘add’ methods have the same signature, ‘add(int, int)’, leading to a compilation error.
Solution: To resolve this issue, you can change the name or the parameters of one of the methods to make the signatures unique.
Problems with Method Overloading
Another common issue is related to method overloading. If the overloaded methods are not defined correctly, it can lead to unexpected behavior.
For instance, consider the following example:
public void display(int a, int b) {
System.out.println(a + b);
}
public void display(int a) {
System.out.println(a);
}
// Output:
// When calling display(5): '5'
// When calling display(5, 10): '15'
In this example, the ‘display’ method is overloaded. If you call ‘display(5)’, Java will invoke the ‘display(int a)’ method, not the ‘display(int a, int b)’ method. This might not be the intended behavior.
Solution: To avoid this, ensure that your overloaded methods are defined correctly. Test your code thoroughly to ensure that the correct method is called in each scenario.
Remember, understanding method signatures and their potential issues is key to writing effective Java code. In the next section, we’ll delve into the fundamentals of method signatures in Java.
Deep Dive into Java Methods and Method Signatures
Before we delve further into method signatures, let’s revisit the fundamentals of methods in Java and their declaration.
Understanding Java Methods
In Java, a method is a collection of statements that perform a specific task. It’s a way to bundle code into reusable blocks. Here’s a simple example of a method declaration:
public void greet() {
System.out.println("Hello, World!");
}
// Output:
// 'Hello, World!'
In this example, ‘greet’ is the method name, and it takes no parameters. The method prints out ‘Hello, World!’ when called.
The Role of Method Signatures
A method signature is a part of the method declaration in Java. It’s the combination of the method name and the parameter list, and it plays a key role in differentiating methods in Java. For example, in the method declaration ‘public void greet()’, the method signature is ‘greet()’.
Method Overloading and Overriding
Method overloading and overriding are two concepts in Java where method signatures play a crucial role.
- Method Overloading: This is when multiple methods in the same class share the same name but have different parameter lists. Java uses the method signature to determine which method to invoke. Here’s an example:
public void display(int a) {
System.out.println("Number: " + a);
}
public void display(String b) {
System.out.println("String: " + b);
}
// Output:
// When calling display(5): 'Number: 5'
// When calling display("Hello"): 'String: Hello'
- Method Overriding: This is when a subclass provides a specific implementation of a method that is already provided by its parent class. Again, Java uses the method signature to determine which method to invoke at runtime. Here’s an example:
class Animal {
void sound() {
System.out.println("The animal makes a sound");
}
}
class Cat extends Animal {
void sound() {
System.out.println("The cat meows");
}
}
// Output:
// When calling sound() on an Animal object: 'The animal makes a sound'
// When calling sound() on a Cat object: 'The cat meows'
Understanding the fundamentals of methods, method signatures, and their role in method overloading and overriding is crucial to mastering Java. In the next section, we’ll explore the relevance of method signatures beyond Java.
The Role of Method Signatures in Object-Oriented Programming
Method signatures are not exclusive to Java; they are a fundamental part of object-oriented programming (OOP). They play a significant role in key OOP concepts like encapsulation and polymorphism.
Method Signatures and Encapsulation
Encapsulation is the OOP principle that binds together the data and functions that manipulate the data. It keeps both safe from outside interference and misuse. Method signatures contribute to encapsulation by defining the interface through which interaction with the data occurs.
Method Signatures and Polymorphism
Polymorphism is the OOP principle that allows one interface to be used for a general class of actions. The specific action is determined by the exact nature of the situation. Method signatures play a crucial role in polymorphism. By overloading or overriding methods, you can define multiple behaviors under the same method name. The correct behavior is determined at runtime based on the method signature.
Exploring Related Concepts
Method signatures in Java open the door to understanding more advanced concepts like constructors and interfaces. Constructors in Java have the same name as the class and do not have a return type. Despite these differences, they still have method signatures, which are used when creating instances of the class.
Interfaces in Java are a collection of abstract methods (methods without a body). Each of these abstract methods has a method signature, and when a class implements an interface, it must provide an implementation for each of these methods, matching the method signature.
Further Resources for Mastering Method Signatures in Java
To explore a Complete Guide on Java Methods Click Here! And we’ve also gathered other resources below:
- Understanding Method Override in Java – Learn how to override methods in Java.
Exploring Method Overloading Techniques in Java – Dive into the concept of method overloading in Java.
Oracle Java Documentation provides a comprehensive overview of methods in Java, including method signatures.
Geeks for Geeks Java Course covers method signatures, method overloading, and method overriding in Java.
JavaTpoint’s Java Tutorial covers method signatures and constructors and interfaces in Java.
Exploring these resources will help you gain a deeper understanding of method signatures and their role in Java and object-oriented programming.
Wrapping Up: Method Signatures in Java
In this comprehensive guide, we’ve journeyed through the world of method signatures in Java, exploring their significance, usage, and the common issues you might encounter.
We began with the basics, understanding what method signatures are and their role in method declaration. We then delved into more advanced topics, discussing method overloading and how method signatures play a crucial role in it. Along the way, we tackled common challenges that might arise, such as method signature clashes and problems with method overloading, providing solutions and workarounds for each issue.
We also explored related concepts like method overloading, method overriding, and how method signatures play a role in these concepts. We discussed their advantages and disadvantages, and provided recommendations for their usage.
Here’s a quick comparison of these concepts:
Concept | Advantages | Disadvantages |
---|---|---|
Method Overloading | Increases readability, allows methods to perform similar functions with different parameters | Can increase code complexity if not used correctly |
Method Overriding | Allows a subclass to provide a specific implementation of a method from its parent class | Can lead to errors if not used correctly |
Whether you’re just starting out with Java or you’re looking to level up your programming skills, we hope this guide has given you a deeper understanding of method signatures and their role in Java programming.
With this knowledge, you’re now better equipped to write effective and efficient Java code. Happy coding!