Print Strings in Bash: Your Scripting Guide to Text Output
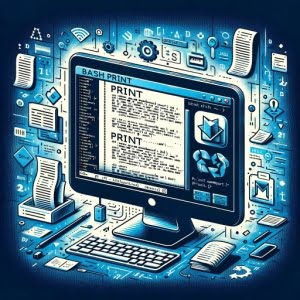
Ever felt stuck trying to print in Bash? You’re not alone. Many developers find themselves puzzled when it comes to handling printing in Bash, but we’re here to help.
Think of Bash’s printing capabilities as a skilled printer – allowing us to output text and variables with ease, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of printing in Bash, from the basics to more advanced techniques. We’ll cover everything from using simple ‘echo’ and ‘printf’ commands to more complex techniques, as well as alternative approaches.
Let’s get started and master printing in Bash!
TL;DR: How Do I Print in Bash?
To print in Bash, you can use the
'echo'
or'printf'
commands. You canecho
with the syntax,echo 'Hello, Bash!'
and printf with the syntax,printf "Hello, World!\n"
These commands allow you to output text or variables to the console.
Here’s a simple example:
echo 'Hello, Bash!'
# Output:
# 'Hello, Bash!'
In this example, we use the ‘echo’ command to print the string ‘Hello, Bash!’ to the console. The ‘echo’ command is one of the most basic ways to print in Bash, and it’s a great starting point for beginners.
But Bash’s printing capabilities go far beyond this. Continue reading for more detailed examples and advanced printing techniques.
Table of Contents
- Basic Printing in Bash: ‘echo’ and ‘printf’
- Advanced Printing in Bash: Formatted Text and Special Characters
- Alternative Printing Methods in Bash: Exploring ‘Here Documents’
- Troubleshooting Bash Print: Common Issues and Solutions
- Bash Scripting and Printing: The Basics
- Beyond Basic Printing: Bash in Larger Scripts and Projects
- Wrapping Up: Mastering Printing in Bash
Basic Printing in Bash: ‘echo’ and ‘printf’
In Bash, the most common commands for printing text and variables are echo
and printf
. Each has its own advantages and potential pitfalls. Let’s explore them in detail.
The ‘echo’ Command
The echo
command is a fundamental tool in Bash scripting. It’s used to output text or variables to the console. Here’s a simple example:
echo 'Bash printing is easy!'
# Output:
# 'Bash printing is easy!'
In this example, we printed a simple string. But echo
can also handle variables. Let’s see how:
name='Alice'
echo "Hello, $name"
# Output:
# 'Hello, Alice'
Here, we first defined a variable name
and then used echo
to print a greeting message that includes the value of the variable.
The ‘printf’ Command
While echo
is simple and easy to use, printf
provides more control over the output format. It’s a bit more complex but also more versatile.
Here’s an example of using printf
:
name='Bob'
printf "Hello, %s\n" $name
# Output:
# 'Hello, Bob'
In this example, %s
is a placeholder for a string, and \n
is used to add a new line at the end of the output. The variable $name
is passed as an argument to printf
, replacing the %s
placeholder in the output.
Both echo
and printf
are powerful tools for printing in Bash. echo
is more straightforward, while printf
offers more formatting options. As a beginner, it’s good to familiarize yourself with both.
Advanced Printing in Bash: Formatted Text and Special Characters
As you become more comfortable with Bash, you’ll find that echo
and printf
can do much more than output simple text and variables. They can handle formatted text and special characters, providing you with powerful tools to improve your scripts’ readability and functionality.
Formatted Text with ‘printf’
The printf
command shines when it comes to printing formatted text. It uses format specifiers, which let you control how the output is displayed. Let’s see an example:
name='Charlie'
age=30
printf "Name: %s, Age: %d\n" $name $age
# Output:
# 'Name: Charlie, Age: 30'
In this example, %s
is a placeholder for a string (the name), and %d
is a placeholder for an integer (the age). We pass the variables $name
and $age
as arguments to printf
, which replace the placeholders in the output. This allows us to create a neatly formatted output.
Special Characters with ‘echo’ and ‘printf’
Both echo
and printf
can handle special characters, which can be useful in a variety of scenarios. For instance, you might want to include a tab character (\t
) to align your output or a newline character (\n
) to move to the next line.
Here’s an example using echo
:
echo -e "Hello,\n Bash!"
# Output:
# 'Hello,
# Bash!'
And here’s a similar example with printf
:
printf "Hello,\nBash!"
# Output:
# 'Hello,
# Bash!'
In these examples, the -e
option with echo
and the double quotes with printf
allow us to interpret backslashes and special characters. The \n
character adds a newline, splitting our message into two lines.
Understanding these advanced uses of echo
and printf
will give you more control over your Bash print operations and open up new possibilities for your scripts.
Alternative Printing Methods in Bash: Exploring ‘Here Documents’
While echo
and printf
are the most commonly used commands for printing in Bash, they are not the only tools at your disposal. As you dive deeper into Bash scripting, you’ll encounter alternative methods such as ‘here documents’ that can provide more flexibility or better suit certain use cases.
Understanding ‘Here Documents’
A ‘here document’ (often abbreviated as ‘heredoc’) is a type of redirection that allows you to pass multiline strings to a command. This can be particularly useful when you need to print large blocks of text.
Here’s an example of a heredoc in action:
cat << EOF
Hello,
This is a multiline string.
EOF
# Output:
# Hello,
# This is a multiline string.
In this example, we use the cat
command along with a heredoc to print a multiline string. The << EOF
syntax starts the heredoc, and the EOF
on a line by itself ends it. Everything in between is treated as the input to the cat
command, which simply prints it out.
Weighing the Pros and Cons
Like any tool, heredocs have their advantages and drawbacks. They’re great for printing large blocks of text, and they can even handle variables and command substitution. However, they can also make your scripts more complex and harder to read, especially for beginners. Therefore, it’s important to use them judiciously.
In the end, the method you choose for printing in Bash will depend on your specific needs and the complexity of your script. Whether you choose echo
, printf
, or a heredoc, each has its place in the Bash scripting toolkit.
Troubleshooting Bash Print: Common Issues and Solutions
As you experiment with printing in Bash, you’ll likely encounter a few common issues. Let’s discuss these problems and how to resolve them.
Unintended Newlines with ‘echo’
One common issue with echo
is that it automatically adds a newline at the end of the output. This can be problematic when you want to print multiple items on the same line. Here’s an example:
echo 'Hello,'
echo 'Bash!'
# Output:
# 'Hello,'
# 'Bash!'
As you can see, echo
prints each statement on a new line. To prevent this, you can use the -n
option with echo
:
echo -n 'Hello,'
echo ' Bash!'
# Output:
# 'Hello, Bash!'
Now, echo
doesn’t add a newline after ‘Hello,’ allowing ‘Bash!’ to be printed on the same line.
Special Characters Not Interpreted
Another common issue is that special characters like \n
(newline) or \t
(tab) are not interpreted as expected. This is because echo
and printf
don’t interpret these characters by default.
For echo
, you can use the -e
option to enable the interpretation of these backslash escapes:
echo -e "Hello,\nBash!"
# Output:
# 'Hello,
# Bash!'
For printf
, you need to include the special characters within the format string:
printf "Hello,\nBash!"
# Output:
# 'Hello,
# Bash!'
By understanding these common issues and their solutions, you can avoid potential pitfalls and make your Bash printing tasks much smoother.
Bash Scripting and Printing: The Basics
Before we delve deeper into the world of printing in Bash, it’s crucial to understand some fundamental concepts about Bash and its scripting capabilities.
Understanding Bash
Bash, or the Bourne Again SHell, is a command interpreter and a well-established Unix shell. It’s widely used for its scripting capabilities, which allow us to automate tasks in Unix-based systems.
In Bash scripting, printing is a common operation. Whether you’re outputting the status of a task, displaying the value of a variable, or creating a report, you’ll often find yourself needing to print text or data.
Here’s a simple example of a Bash script that uses the echo
command to print a status message:
#!/bin/bash
echo 'Starting the task...'
# Output:
# 'Starting the task...'
In this script, #!/bin/bash
is a shebang that tells the system to execute the script using Bash. The echo
command then prints the message ‘Starting the task…’.
Bash Print Commands: ‘echo’ and ‘printf’
As we’ve discussed, echo
and printf
are the primary commands for printing in Bash. They are built-in commands, meaning they are part of the Bash shell itself and don’t require any external programs.
Understanding these fundamentals of Bash and its scripting capabilities will provide a solid foundation as we explore more complex topics related to printing in Bash.
Beyond Basic Printing: Bash in Larger Scripts and Projects
While we’ve focused on printing in Bash, it’s important to understand that Bash scripting is a vast field with numerous applications. Printing is just one aspect of it. In larger scripts or projects, you’ll often find yourself using printing alongside other Bash features like variable assignment, loops, and conditional statements.
Variable Assignment and Printing
Variable assignment is a common operation in Bash. You assign a value to a variable and then use that variable in your script. When combined with printing, this allows you to output dynamic data.
name='Alice'
echo "Hello, $name"
# Output:
# 'Hello, Alice'
In this example, we assign the string ‘Alice’ to the variable name
and then print a greeting message that includes the value of the variable.
Loops, Conditional Statements, and Printing
Loops and conditional statements add logic to your Bash scripts. With printing, you can output different messages based on the script’s logic.
for i in {1..5}
do
if (( i % 2 == 0 ))
then
echo "$i is even."
else
echo "$i is odd."
fi
done
# Output:
# '1 is odd.'
# '2 is even.'
# '3 is odd.'
# '4 is even.'
# '5 is odd.'
In this script, we use a for loop to iterate over the numbers 1 through 5. We then use an if-else statement to check if the current number is even or odd, printing a different message for each case.
Further Resources for Bash Scripting Mastery
To deepen your understanding of Bash scripting and its various aspects, consider exploring the following resources:
- GNU Bash Manual: The official manual for Bash, providing comprehensive information about its features and capabilities.
- Bash Academy: An interactive platform for learning Bash scripting, with numerous examples and exercises.
- Bash Scripting Guide on LinuxCommand: A detailed guide on writing Bash scripts, covering topics like variable assignment, loops, and conditional statements.
Remember, mastering Bash scripting takes practice. Don’t be afraid to experiment with different commands and techniques, and keep learning!
Wrapping Up: Mastering Printing in Bash
In this comprehensive guide, we’ve explored the various methods of printing in Bash, from basic to advanced techniques.
We started with the basics, learning how to use the echo
and printf
commands for simple printing tasks. We then delved into more advanced techniques, exploring formatted text, special characters, and alternative methods like ‘here documents’.
Throughout our journey, we’ve tackled common issues you might encounter when printing in Bash, such as unintended newlines and uninterpreted special characters, providing solutions for each problem.
We also compared the different methods of printing in Bash, helping you understand when to use each one. Here’s a quick comparison:
Method | Simplicity | Versatility | Use Case |
---|---|---|---|
echo | High | Moderate | Simple text and variable printing |
printf | Moderate | High | Formatted text and special characters |
Here Documents | Low | High | Multiline strings and large blocks of text |
Whether you’re just starting out with Bash or looking to level up your scripting skills, we hope this guide has given you a deeper understanding of Bash’s printing capabilities.
With its versatility and power, Bash is an indispensable tool for scripting in Unix-based systems. Now, you’re well equipped to master the art of printing in Bash. Happy scripting!