Python getattr() Function Guide (With Examples)
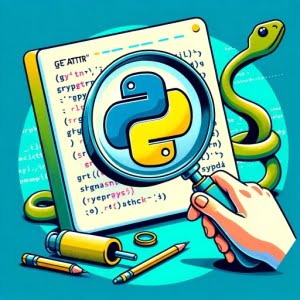
Are you finding Python’s getattr function a bit elusive? You’re not alone. Many developers find themselves puzzled when it comes to using this powerful Python built-in function.
Think of Python’s getattr function as a secret agent – it can retrieve hidden information, in this case, the attributes of an object. It’s a powerful tool that can significantly simplify your code, especially when you’re dealing with dynamic attribute access.
In this guide, we’ll walk you through the process of mastering Python’s getattr function, from basic usage to advanced techniques. We’ll cover everything from the fundamentals of attribute access in Python, practical examples of using getattr, to troubleshooting common issues and exploring alternative approaches.
So, let’s dive in and start mastering Python’s getattr function!
TL;DR: How Do I Use Python’s getattr Function?
The getattr function in Python is used to retrieve the value of a named attribute of an object. If you have an object with an attribute ‘x’, you can access the value of ‘x’ using
getattr(object, 'x')
.
Here’s a simple example:
class Test:
x = 5
t = Test()
print(getattr(t, 'x'))
# Output:
# 5
In this example, we’ve created a class Test
with an attribute x
set to 5. We then create an instance of Test
named t
. Using getattr
, we retrieve the value of ‘x’ from the object t
, which outputs 5.
This is a basic way to use Python’s getattr function, but there’s much more to learn about dynamic attribute access in Python. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Understanding Python’s getattr Function: A Beginner’s Guide
- Advanced getattr Usage: Retrieving Methods and Modules
- Alternative Approaches to Accessing Attributes
- Troubleshooting Common Issues with getattr
- Python Attribute Access: The Fundamentals
- Exploring Further: getattr in Larger Projects
- Wrapping Up: Mastering Python’s getattr Function
Understanding Python’s getattr Function: A Beginner’s Guide
The getattr
function in Python is a built-in function designed to retrieve the value of a named attribute from an object. The syntax is as follows:
getattr(object, attribute_name[, default_value])
Here, object
is the object from which you want to retrieve the attribute, attribute_name
is a string that contains the name of the attribute, and default_value
is an optional value that will be returned if the attribute does not exist.
Let’s take a look at a simple example:
class Test:
x = 10
t = Test()
print(getattr(t, 'x'))
# Output:
# 10
In this example, we’ve created a class Test
with an attribute x
set to 10. We then create an instance of Test
named t
. Using getattr
, we retrieve the value of ‘x’ from the object t
, which outputs 10.
One of the main advantages of getattr
is that it allows for dynamic attribute access. This means you can determine the attribute to be accessed at runtime, which is not possible with traditional dot notation.
However, one potential pitfall of getattr
is that it can raise an AttributeError
if the attribute does not exist and no default value is provided. To avoid this, always provide a default value when you’re not certain the attribute exists.
print(getattr(t, 'y', 'Attribute does not exist'))
# Output:
# Attribute does not exist
In this example, since ‘y’ does not exist in the Test
object, getattr
returns the default value ‘Attribute does not exist’.
Advanced getattr Usage: Retrieving Methods and Modules
As you become more familiar with Python’s getattr
function, you’ll discover that it’s not limited to retrieving simple attribute values. It can also be used to retrieve methods and even access attributes from imported modules.
Retrieving Methods with getattr
If an attribute of an object is a method, getattr
can be used to retrieve that method, and you can subsequently call it. Let’s take a look at an example:
class Test:
def greet(self):
return 'Hello, getattr!'
t = Test()
greeting = getattr(t, 'greet')
print(greeting())
# Output:
# 'Hello, getattr!'
In this example, the Test
class has a method greet
. We use getattr
to retrieve this method from an instance of Test
, and then we call it. The output is the string ‘Hello, getattr!’.
Using getattr with Modules
You can also use getattr
to access attributes from imported modules. This can be particularly useful when you want to dynamically import and use different modules based on certain conditions.
Here’s an example using the built-in math
module:
import math
# Get the sqrt function from the math module
sqrt_func = getattr(math, 'sqrt')
print(sqrt_func(16))
# Output:
# 4.0
In this example, we import the math
module and use getattr
to retrieve the sqrt
function. We then call this function to calculate the square root of 16, which outputs 4.0.
As you can see, Python’s getattr
function is a versatile tool that can greatly simplify your code when you need to dynamically access attributes, methods, or module functions.
Alternative Approaches to Accessing Attributes
While getattr
is a powerful function, Python offers other ways to access attributes. Two common alternatives are dot notation and the hasattr
function.
Dot Notation
Dot notation is the most straightforward way to access an attribute. You simply use a dot followed by the attribute name. Here’s an example:
class Test:
x = 10
t = Test()
print(t.x)
# Output:
# 10
In this example, we access the ‘x’ attribute of the Test
object using dot notation. This is a simple and readable way to access attributes, but it doesn’t allow for dynamic attribute access like getattr
.
Using hasattr Function
The hasattr
function is another alternative. It checks if an object has a particular attribute and returns True
or False
. This can be useful to avoid AttributeError
when trying to access an attribute that may not exist.
print(hasattr(t, 'x'))
print(hasattr(t, 'y'))
# Output:
# True
# False
In this example, hasattr
checks if the Test
object has attributes ‘x’ and ‘y’. Since ‘x’ exists, it returns True
, and since ‘y’ does not exist, it returns False
.
While hasattr
can help avoid errors, it’s generally more pythonic to handle exceptions using try-except blocks. Also, hasattr
can’t retrieve the attribute value like getattr
, it can only check its existence.
In conclusion, while getattr
is a powerful tool for dynamic attribute access, dot notation and hasattr
also have their uses depending on your specific needs.
Troubleshooting Common Issues with getattr
While Python’s getattr
function is a powerful tool, it’s not without its quirks. Let’s discuss some common issues you might encounter and how to handle them.
Handling AttributeError
One common issue when using getattr
is encountering an AttributeError
. This error is raised when you try to access an attribute that doesn’t exist on an object, and you haven’t provided a default value. Here’s an example:
class Test:
x = 10
t = Test()
print(getattr(t, 'y'))
# Output:
# AttributeError: 'Test' object has no attribute 'y'
In this example, we’re trying to access the ‘y’ attribute of the Test
object, but ‘y’ does not exist. Since we didn’t provide a default value, Python raises an AttributeError
.
To handle this, you can use a try-except block to catch the AttributeError
and take appropriate action:
try:
print(getattr(t, 'y'))
except AttributeError:
print('Attribute does not exist')
# Output:
# Attribute does not exist
Alternatively, you can provide a default value to getattr
, which will be returned if the attribute does not exist:
print(getattr(t, 'y', 'Default value'))
# Output:
# Default value
These are some ways to handle common issues when using Python’s getattr
function. With careful error handling and the use of default values, you can use getattr
effectively and avoid common pitfalls.
Python Attribute Access: The Fundamentals
Before we delve deeper into the advanced uses of Python’s getattr
function, it’s crucial to understand the fundamentals of how attribute access works in Python.
In Python, everything is an object – from numbers and strings to functions and classes. These objects can have attributes, which are essentially variables associated with the object. For instance, a class can have data attributes (variables) and method attributes (functions).
class Test:
x = 10 # data attribute
def greet(self): # method attribute
return 'Hello, Python!'
t = Test()
print(t.x)
print(t.greet())
# Output:
# 10
# 'Hello, Python!'
In this example, Test
is a class with a data attribute x
and a method attribute greet
. We create an instance t
of Test
and access these attributes using dot notation.
However, what if you want to access an attribute dynamically, i.e., you don’t know the attribute’s name until runtime? That’s where getattr
comes in. It allows you to retrieve the value of a named attribute of an object, even if that name is stored in a variable.
attr_name = 'x'
print(getattr(t, attr_name))
# Output:
# 10
In this example, we use getattr
to access the ‘x’ attribute of the Test
object, even though the attribute name is stored in a variable. This is the essence of dynamic attribute access in Python, and it’s one of the key reasons why getattr
is such a powerful tool.
Exploring Further: getattr in Larger Projects
Python’s getattr
function can be particularly useful in larger scripts or projects. Its ability to dynamically access attributes can greatly simplify code and make it more flexible. This can be especially beneficial in situations where you need to interact with objects whose structure you may not know in advance, or when you’re working with complex data structures.
But getattr
is only one part of the picture. Python also provides setattr
and delattr
functions, which allow you to set and delete attributes dynamically, respectively.
For example, setattr
can be used to set the value of an attribute, even if it doesn’t exist yet:
class Test:
pass
t = Test()
setattr(t, 'x', 10)
print(t.x)
# Output:
# 10
In this example, we create an instance t
of the Test
class, which initially has no attributes. We then use setattr
to assign the value 10 to ‘x’. When we print t.x
, it outputs 10.
Similarly, delattr
can be used to delete an attribute:
class Test:
x = 10
t = Test()
delattr(t, 'x')
print(hasattr(t, 'x'))
# Output:
# False
In this example, we delete the ‘x’ attribute from the Test
object using delattr
. When we check if ‘x’ exists using hasattr
, it returns False
.
Utilizing getattr
, setattr
, and delattr
together can give you a high degree of control over object attributes, making your code more flexible and dynamic.
Further Resources for Mastering Python’s getattr
To deepen your understanding of Python’s getattr
function and related concepts, here are some resources you might find helpful:
- IOFlood’s Python Built-In Functions Guide – Dive into the world of date and time handling using Python’s functions.
Checking for Attributes in Python with hasattr() – Learn how to use “hasattr” to enhance error handling in Python code.
Python input() Function: User Input Handling – Explore Python’s “input” function for user interaction in console applications.
Python’s official documentation on built-in functions offers a detailed explanation of
getattr
and other built-in functions.Real Python’s guide on Python classes and object-oriented programming provides an intro to Python classes and attributes.
Python’s official tutorial on classes provides an in-depth look at classes, including a section on attribute references.
Wrapping Up: Mastering Python’s getattr Function
In this comprehensive guide, we’ve delved into the world of Python’s getattr
function, a powerful tool for dynamic attribute access. We’ve explored its usage, from basic attribute retrieval to advanced scenarios involving methods and modules.
We began with the basics, explaining how getattr
works and how to use it to retrieve the value of a named attribute from an object. We then delved into more advanced usage, demonstrating how getattr
can be used to retrieve methods and even access attributes from imported modules.
We also discussed common issues you might encounter when using getattr
such as handling AttributeError
, and provided solutions to help you effectively use getattr
in your Python code.
In addition to getattr
, we explored alternative approaches to accessing attributes in Python, such as using dot notation and the hasattr
function. We compared these methods and discussed their benefits and drawbacks to give you a broader understanding of attribute access in Python.
Here’s a quick comparison of these methods:
Method | Dynamic Access | Error Handling | Use Cases |
---|---|---|---|
getattr | Yes | Requires try-except or default value | When attribute name is not known until runtime |
Dot Notation | No | Raises AttributeError if attribute does not exist | When attribute name is known and exists |
hasattr | No | Returns False if attribute does not exist | When you need to check if an attribute exists, but not retrieve its value |
Whether you’re just starting out with Python or you’re looking to level up your Python skills, we hope this guide has given you a deeper understanding of Python’s getattr
function and its capabilities.
With its ability to dynamically access attributes, getattr
is a powerful tool that can make your Python code more flexible and efficient. Happy coding!