Python hasattr() Function Guide: Usage and Examples
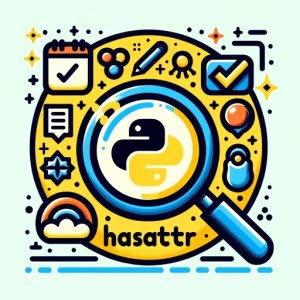
Are you finding it challenging to understand Python’s hasattr function? You’re not alone. Many developers find themselves puzzled when it comes to using hasattr, but we’re here to help.
Think of Python’s hasattr as a detective – it allows you to uncover hidden attributes of an object, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of using hasattr in Python, from its basic usage to more advanced techniques. We’ll cover everything from the basics of attribute checking to alternative approaches and common issues.
So, let’s dive in and start mastering Python’s hasattr!
TL;DR: What is Python’s hasattr()
Function?
Python’s hasattr function is a built-in function used to check if an object has a specific attribute, like
print(hasattr(test, 'x'))
. It returns True if the attribute exists and False otherwise.
Here’s a simple example:
class Test:
x = 10
test = Test()
print(hasattr(test, 'x'))
# Output:
# True
In this example, we’ve created a class Test
with an attribute x
. We then create an instance of Test
and use hasattr
to check if x
is an attribute of our instance. The function returns True, indicating that x
is indeed an attribute of test
.
This is just a basic usage of Python’s hasattr function. Stick around for a more detailed exploration of hasattr’s functionality, use cases, and some advanced techniques.
Table of Contents
Python’s hasattr: Basic Usage
Python’s hasattr
function is an inbuilt function that checks whether an object has a specific attribute or not. It takes two parameters: the object and the attribute (in the form of a string) you want to check for. The function returns True
if the attribute exists, and False
if it doesn’t.
Let’s take a look at a basic example:
class Animal:
species = 'Mammal'
animal = Animal()
print(hasattr(animal, 'species'))
# Output:
# True
In this example, we have a class Animal
with an attribute species
. We create an instance of Animal
and use hasattr
to check if species
is an attribute of our instance. The function returns True
, indicating that species
is indeed an attribute of animal
.
Advantages of Using hasattr
The hasattr
function is a powerful tool that allows you to handle Python objects more flexibly. If you’re not sure whether an object has a particular attribute, hasattr
can help you check before trying to access or manipulate it, thus avoiding potential errors.
Pitfalls of Using hasattr
While hasattr
is incredibly useful, it’s important to note that it only checks for the existence of an attribute, not its value. This means that if an attribute exists but its value is None
, hasattr
will still return True
.
Furthermore, hasattr
only checks for the existence of an attribute, not whether the attribute is callable or not. This means that if you’re checking for a method, hasattr
will return True
even if the method isn’t callable.
In the next section, we’ll cover more complex usages of the hasattr
function.
Attributes vs Variables
What is an attribute? Is it just a variable?
While all attributes could be thought of as variables in the broad sense, an attribute specifically refers to a value that is associated with an object, such as my_object.my_attribute
. This is in contrast to a ‘free’ variable, which might be defined in your script but not as part of a class or object.
In Python, an attribute of an object can be:
- An instance variable that belongs to the object. Instance variables represent the data that makes up the object. For example, in an object of class
Dog
, instance variables might includename
,age
, orbreed
. A class variable that belongs to the class that the object is an instance of. Class variables store values that should be shared across all instances of a class – they are not specific to any one instance.
A method that is defined within the class the object is an instance of. Methods are functions which can be called on objects, and will often manipulate the instance and class variables of those objects.
Note, however, that attributes are not limited only to these. Technically, an attribute in Python is just a value associated with an object; the nature of this value can be very diverse. Additional forms that attributes can take, albeit less commonly, include properties, or even other objects or classes.
Attributes for hasattr()
For hasattr()
to return True
, the attribute
in question must indeed fall explicitly under one of these categories: it should be either a part of the object’s instance variables, or a method within the object’s class, or a class variable of the object’s class. If it’s not, then hasattr()
will return False
, because as far as it is concerned, the object does not have that attribute.
When using the hasattr()
function in Python, we address whether a particular object
possesses a specific attribute
, which could be either a data attribute or a method. However, if the attribute
in question is a variable defined outside of the object
or its class, hasattr
won’t know about it and will return False
.
The key takeaway is that hasattr()
is limited to checking whether a specified object has a defined attribute or method. It doesn’t concern itself with checking for variables by the same name elsewhere in your code.
So how do I know if something is an attribute?
The bottom line is, if you can refer to it via dot notation like my_object.my_attribute
, it is considered an attribute and hasattr() will return True if it exists.
The Role of hasattr in Python
In Python, attributes are dynamic. They can be added, modified, or deleted. This is where hasattr
comes in. The hasattr
function allows you to check if an object has a specific attribute before you try to access or manipulate it. This can help you avoid errors and make your code more robust.
In the context of Python’s dynamic attributes, hasattr
is a powerful tool. It allows you to write code that can handle a wide range of objects, regardless of their attributes. This flexibility makes hasattr
a valuable function to understand and master.
Advanced hasattr Usage: Methods and Private Attributes
As we get more comfortable with Python’s hasattr
function, we can start to explore its more advanced uses. This includes checking for methods and private attributes of an object.
Checking for Methods
Remember, hasattr
can also be used to check if an object has a specific method. Let’s look at an example:
class Animal:
def make_sound(self):
return 'Roar'
animal = Animal()
print(hasattr(animal, 'make_sound'))
# Output:
# True
In the above code, we have a class Animal
with a method make_sound
. We create an instance of Animal
and use hasattr
to check if make_sound
is a method of our instance. The function returns True
, indicating that make_sound
is indeed a method of animal
.
Checking for Private Attributes
hasattr
can also check for private attributes. A private attribute in Python is one that starts with a double underscore (__
).
class Animal:
__name = 'Lion'
animal = Animal()
print(hasattr(animal, '__name'))
# Output:
# True
In this code, we have a class Animal
with a private attribute __name
. We create an instance of Animal
and use hasattr
to check if __name
is an attribute of our instance. The function returns True
, indicating that __name
is indeed an attribute of animal
.
Pros and Cons
Utilizing hasattr
for checking methods and private attributes can provide a more dynamic approach to handling objects in Python. It can help you write more robust and error-free code.
However, it’s important to remember that hasattr
only checks for the existence of an attribute or method, not its accessibility or callability. This means that even if a method or private attribute exists, you may not necessarily be able to access or call it.
In the next section, we’ll explore alternative approaches to checking for attributes in Python.
Exploring Alternatives
While hasattr
is a powerful tool, Python offers other ways to check for attributes. Two notable alternatives are the getattr()
function and the dir()
function.
Using getattr to Check for Attributes
The getattr()
function can also be used to check if an object has a specific attribute. Unlike hasattr
, getattr
can return the value of the attribute if it exists. If the attribute doesn’t exist, it returns a default value if provided, otherwise it raises an AttributeError
.
Here’s an example:
class Animal:
species = 'Lion'
animal = Animal()
print(getattr(animal, 'species', 'Not found'))
# Output:
# Lion
In this example, we use getattr
to try to get the value of species
from our animal
instance. Since species
does exist, getattr
returns its value, ‘Lion’.
Using dir to Check for Attributes
The dir()
function returns a list of all the attributes and methods of an object. You can use this list to check if an attribute exists.
class Animal:
name = 'Lion'
animal = Animal()
print('name' in dir(animal))
# Output:
# True
In this code, we use dir
to get a list of all the attributes and methods of our animal
instance. We then check if ‘name’ is in this list. Since it is, the code returns True
.
Weighing Your Options
Both getattr
and dir
offer different advantages over hasattr
. getattr
can return the value of an attribute, and dir
provides a comprehensive list of an object’s attributes and methods. However, getattr
raises an error if the attribute doesn’t exist and no default value is provided, and dir
can be slower if you’re only checking for one attribute.
In the end, the best function to use depends on your specific needs and the situation at hand.
Troubleshooting Common hasattr Issues
While using the hasattr
function in Python, you may encounter various challenges or errors. This section will discuss some common obstacles and their solutions, along with some best practices for optimization.
AttributeError
One common error when working with hasattr
is the AttributeError
. This occurs when you try to access an attribute that does not exist. However, hasattr
is designed to handle this error gracefully, returning False
instead of raising an AttributeError
.
class Animal:
species = 'Lion'
animal = Animal()
print(hasattr(animal, 'name'))
# Output:
# False
In this example, we’re checking if the animal
object has an attribute name
. Since it doesn’t, hasattr
returns False
.
Checking for Callable Methods
As mentioned earlier, hasattr
only checks if an attribute or method exists, not if it’s callable. To check if a method is callable, you can use the callable
function.
class Animal:
def make_sound(self):
return 'Roar'
animal = Animal()
print(callable(getattr(animal, 'make_sound', None)))
# Output:
# True
In this code, we first use getattr
to get the method make_sound
, or None
if it doesn’t exist. We then use callable
to check if the returned method is callable. Since make_sound
is a method and is callable, the code returns True
.
Best Practices and Optimization
When using hasattr
, it’s important to remember its purpose: to check if an object has a specific attribute. Avoid using hasattr
as a means to get the value of an attribute, as getattr
is more suited for this purpose.
Moreover, hasattr
should be used judiciously. Overusing hasattr
can lead to code that is hard to read and debug. Instead, strive to design your classes and objects in a way that you know what attributes they have.
Expanding the Use of hasattr in Larger Projects
Python’s hasattr
function isn’t just for small scripts or isolated use. It can be a valuable tool in larger projects, where objects can have a wide range of attributes, and you need to handle them dynamically.
For instance, in a large codebase, you might have a variety of classes, each with different attributes. When you’re writing a function that needs to handle objects from these classes, you can use hasattr
to check for specific attributes and handle each object appropriately.
Complementary Python Functions
In addition to hasattr
, Python provides other useful functions that often accompany hasattr
in typical use cases. These include getattr
, which gets the value of an attribute if it exists, and setattr
, which sets the value of an attribute.
Here’s a quick example:
class Animal:
species = 'Lion'
animal = Animal()
if hasattr(animal, 'species'):
print(getattr(animal, 'species'))
setattr(animal, 'species', 'Tiger')
print(getattr(animal, 'species'))
# Output:
# Lion
# Tiger
In this code, we first check if the animal
object has a species
attribute. If it does, we get its value using getattr
, set a new value using setattr
, and then get the new value using getattr
again.
Further Resources for Mastering Python’s hasattr
To further your understanding of Python’s hasattr function and related topics, here are some resources:
- Python Built-In Functions: Your Coding Toolkit – Dive deep into the world of string manipulation with Python’s functions.
Python Bytes Data Type: Binary Data Handling – Dive into byte manipulation, encoding, and decoding in Python.
Python getattr() Function: Dynamic Attribute Access – Discover Python’s “getattr” function for retrieving object attributes.
Python’s Official hasattr Documentation – The official Python documentation on the usage of Python’s hasattr function.
Python’s Built-in Functions: An Interactive Guide that offers an interactive look at Python’s myriad of built-in functions.
Tutorial on Python Functions – A beginner-friendly tutorial by DataCamp.
Each of these resources provides in-depth information about Python’s hasattr and related functions, helping you to deepen your understanding and mastery of these important tools.
Wrapping Up: Mastering Python’s hasattr Function
In this comprehensive guide, we’ve navigated the ins and outs of Python’s hasattr
function, a powerful tool for handling attributes in Python objects.
We began with the basics, explaining what hasattr
is and how to use it at a beginner level. We then delved into more complex usages, including checking for methods and private attributes, and even explored alternative approaches like getattr
and dir
.
Along the way, we tackled common issues that you might encounter when using hasattr
, such as AttributeError
and the consideration of callable methods, providing solutions and workarounds for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
hasattr | Checks for attribute existence, handles AttributeError | Only checks existence, not value or callability |
getattr | Can return attribute value, can provide default value | Raises AttributeError if attribute doesn’t exist and no default value is provided |
dir | Returns all attributes and methods | Can be slower if only checking for one attribute |
Whether you’re just starting out with Python’s hasattr
function or looking to deepen your understanding, we hope this guide has been a valuable resource.
Mastering hasattr
allows you to handle Python objects more flexibly and robustly, making your code more dynamic and robust. Now, you’re well equipped to harness the power of hasattr
. Happy coding!