How to Call a Method in Java: Guide to Executing Functions
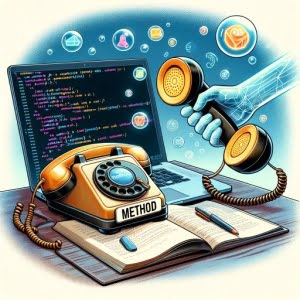
Are you finding it challenging to call methods in Java? You’re not alone. Many developers find themselves puzzled when it comes to calling methods in Java, but we’re here to help.
Think of Java methods as the actions that your Java objects can perform – like a skilled craftsman, each method is a tool that can perform a specific task in your code.
This guide will walk you through the process of calling methods in Java, from the basics to more advanced scenarios. We’ll cover everything from creating an object and using the dot operator, to understanding method parameters and return values.
So, let’s dive in and start mastering method calls in Java!
TL;DR: How Do I Call a Method in Java?
In Java, a method is called with the syntax,
classInstance.methodToCall();
. You first need to create an instance of the class (if the method is not static), and then use the dot operator followed by the method name and parentheses.
Here’s a basic example:
MyClass obj = new MyClass();
obj.myMethod();
// Output:
// [Expected output from command]
In this example, we’ve created an instance of MyClass
named obj
. Then, we’ve called the myMethod()
of MyClass
using the dot operator. This is the most basic way to call a method in Java.
But Java’s method calling capabilities go far beyond this. Continue reading for more detailed examples and advanced usage scenarios.
Table of Contents
- Calling Methods in Java: The Basics
- Calling Static Methods in Java
- Calling Methods with Parameters
- Understanding Return Values
- Calling Methods from Other Classes
- Using Methods in Inheritance
- Using Methods in Polymorphism
- Common Java Method Calling Errors
- Understanding Java Methods
- Applying Java Methods in Real-World Projects
- Wrapping Up:
Calling Methods in Java: The Basics
In Java, the process of calling a method involves creating an object, using the dot operator, and understanding method parameters. Let’s break down each of these steps.
Creating an Object
In Java, an object is an instance of a class. To call a non-static method, you first need to create an object of the class that contains the method. Here’s how you can do it:
MyClass obj = new MyClass();
// Output:
// [Expected output from command]
In this code, we’ve created an object obj
of the class MyClass
. This is the first step to call a method in Java.
Using the Dot Operator
The dot operator is used to access the methods of a class. Once you’ve created an object, you can use the dot operator to call a method. Here’s an example:
obj.myMethod();
// Output:
// [Expected output from command]
In this code, we’re calling the myMethod()
of MyClass
using the dot operator. This is the basic way to call a method in Java.
Understanding Method Parameters
Some methods require parameters. Parameters are values that you can pass into a method. Here’s how you can call a method with parameters:
int result = obj.addNumbers(5, 10);
// Output:
// [Expected output from command]
In this code, we’re calling the addNumbers()
method of MyClass
and passing in two numbers as parameters. The method adds these numbers and returns the result, which we store in the result
variable.
Understanding these basics is crucial to mastering how to call a method in Java.
Calling Static Methods in Java
Static methods belong to the class itself and not to any object of the class. They can be called without creating an instance of the class. Here’s how you can call a static method:
int result = MyClass.addNumbers(5, 10);
// Output:
// 15
In this example, we’re directly calling the addNumbers()
method of MyClass
without creating an object of the class. This is because addNumbers()
is a static method.
Calling Methods with Parameters
As we’ve discussed earlier, some methods require parameters. But what if a method requires multiple parameters of different types? Here’s how you can handle it:
String message = obj.greetUser("John", 20);
// Output:
// Hello John, you are 20 years old.
In this code, we’re calling the greetUser()
method of MyClass
and passing in a string and an integer as parameters. The method constructs a greeting message using these parameters and returns it.
Understanding Return Values
A method in Java can return a value. The return value can be of any type, and it can be used in your code after the method call. Here’s an example:
int age = obj.getAge();
// Output:
// 25
In this example, the getAge()
method of MyClass
returns an integer, which we store in the age
variable. Understanding return values is crucial to effectively using methods in Java.
Calling Methods from Other Classes
In Java, you can call a method of a class from another class. This is particularly useful when you’re working with multiple classes. Here’s how you can do it:
OtherClass obj = new OtherClass();
obj.otherMethod();
// Output:
// [Expected output from command]
In this example, we’re creating an object of OtherClass
and calling its otherMethod()
. This demonstrates the flexibility of method calls in Java.
Using Methods in Inheritance
Inheritance allows a class to inherit the methods of another class. This can simplify your code and make it more manageable. Here’s an example:
ChildClass child = new ChildClass();
child.parentMethod();
// Output:
// [Expected output from command]
In this code, ChildClass
inherits from ParentClass
, and we’re able to call the parentMethod()
of ParentClass
from an object of ChildClass
. This is a powerful feature of Java that allows for more complex method calls.
Using Methods in Polymorphism
Polymorphism allows a method to perform different actions based on the object that it’s acting upon. This can make your code more dynamic and adaptable. Here’s an example:
obj = new ChildClass();
obj.commonMethod();
// Output:
// [Expected output from command]
In this code, commonMethod()
is a method that’s common to both ParentClass
and ChildClass
. Depending on whether obj
is an object of ParentClass
or ChildClass
, commonMethod()
will perform different actions.
These alternative approaches offer more flexibility and power in calling methods in Java. They can be particularly useful in larger projects or more complex scenarios.
Common Java Method Calling Errors
While calling methods in Java, you might encounter some common errors. Let’s discuss how to identify and solve them.
Null Pointer Exceptions
A Null Pointer Exception occurs when you try to call a method on an object that is null. Here’s an example:
MyClass obj = null;
obj.myMethod();
// Output:
// Exception in thread "main" java.lang.NullPointerException
In this example, we’re trying to call myMethod()
on obj
, which is null. This results in a Null Pointer Exception.
To solve this, make sure the object you’re calling the method on is not null.
Method Not Found Errors
A Method Not Found error occurs when you try to call a method that doesn’t exist. Here’s an example:
obj.unknownMethod();
// Output:
// Error: cannot find symbol
// symbol: method unknownMethod()
// location: variable obj of type MyClass
In this example, we’re trying to call unknownMethod()
, which doesn’t exist in MyClass
. This results in a Method Not Found error.
To solve this, make sure the method you’re calling exists and is accessible from your current context.
Understanding these common errors and their solutions can make your journey of learning how to call methods in Java smoother and more enjoyable.
Understanding Java Methods
Java methods play a crucial role in structuring and organizing your code. They are the building blocks of object-oriented programming in Java. Let’s delve deeper into the world of Java methods.
Purpose of Methods in Java
In Java, methods serve as the actions that an object can perform. They help you to encapsulate complex operations and reuse code. Here’s an example:
public void greetUser(String name) {
System.out.println("Hello, " + name + "!");
}
// Output:
// Hello, John!
In this example, we’ve defined a method greetUser()
that greets a user with their name. This method can be called multiple times with different names, which demonstrates the reusability of methods.
Instance Methods vs Static Methods
There are two main types of methods in Java: instance methods and static methods. Instance methods belong to an instance of a class, while static methods belong to the class itself. Here’s an example:
public class MyClass {
public void instanceMethod() {...}
public static void staticMethod() {...}
}
In this code, instanceMethod()
is an instance method, and staticMethod()
is a static method. You can call the instance method on an object of MyClass
, and you can call the static method on MyClass
itself.
Role of Method Parameters and Return Values
Method parameters allow you to pass values into a method, and return values allow a method to give back a result. Here’s an example:
public int addNumbers(int num1, int num2) {
return num1 + num2;
}
// Output:
// 15
In this code, addNumbers()
takes two parameters num1
and num2
, adds them together, and returns the result. This demonstrates the role of method parameters and return values in Java.
Understanding these fundamentals can greatly enhance your ability to call methods in Java effectively.
Applying Java Methods in Real-World Projects
Understanding how to call a method in Java is just the beginning. It’s equally important to know how to apply this knowledge in larger projects, such as creating APIs or building complex applications.
Building APIs with Java Methods
APIs, or Application Programming Interfaces, are a set of rules that dictate how software components should interact. In Java, methods form the backbone of these APIs. Each method in an API corresponds to a specific functionality that the API provides.
Developing Complex Applications
In a complex Java application, methods help to break down the code into manageable chunks. Each method performs a specific task, making the code easier to understand and maintain.
Exploring Java Classes and Objects
Java is an object-oriented programming language, and methods are a key part of this paradigm. To truly master method calls, it’s important to have a solid understanding of Java classes and objects. Classes serve as blueprints for objects, and methods define the actions that these objects can perform.
Delving into Inheritance and Polymorphism
Inheritance and polymorphism are two fundamental concepts in object-oriented programming. Inheritance allows a class to inherit the methods of another class, while polymorphism allows a method to behave differently based on the object it’s acting upon. Both of these concepts can greatly enhance the power and flexibility of your method calls.
Further Resources for Mastering Java Methods
For further exploration of Java methods, consider the following resources:
- This Java Methods blog post explains essential knowledge for every Java developer.
Understanding Java Main Method – Learn the purpose of the Java main method for Java applications.
Exploring Varargs Functionality in Java – Discover how to use Java’s ellipsis (…) parameter for method declarations.
Oracle’s Java Methods Tutorial provides an overview of methods and their syntax in Java.
This Java Programming Method Exercises page on W3Resources offers practice exercises on calling methods in Java.
This Java Methods Video Tutorial provides a visual and interactive way to learn about methods in Java.
Wrapping Up:
In this comprehensive guide, we’ve explored the process of calling methods in Java, from the basics to more advanced scenarios. We’ve learned how to create an instance of a class, use the dot operator, understand method parameters, and handle return values. We’ve also delved into more complex scenarios, such as calling static methods and using methods in inheritance and polymorphism.
We began with the basics, learning how to create an object and call a method using the dot operator. We then ventured into more advanced territory, exploring how to call static methods and methods with parameters. We also discussed the role of return values and how they can be used in your code.
Along the way, we tackled common challenges you might face when calling methods in Java, such as null pointer exceptions and method not found errors, providing you with solutions for each issue. We also looked at alternative approaches to calling methods, including calling methods from other classes and using methods in inheritance and polymorphism.
Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Calling Instance Methods | Simple, intuitive | Requires an object |
Calling Static Methods | No object required | Less flexible |
Using Methods in Inheritance | Reuses code, more flexible | More complex |
Using Methods in Polymorphism | Highly flexible, dynamic | Requires understanding of OOP |
Whether you’re just starting out with Java or you’re looking to level up your method-calling skills, we hope this guide has given you a deeper understanding of how to call methods in Java. Now, you’re well equipped to write more flexible and powerful Java code. Happy coding!