Java String .trim() Method: Efficient Whitespace Removal
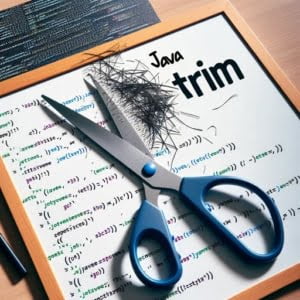
Are you finding it challenging to remove unwanted spaces in your Java strings? You’re not alone. Many developers find themselves grappling with this task, but there’s a tool that can make this process a breeze.
Just like a skilled barber, Java’s trim function can neatly cut off the excess – the unnecessary white spaces in your strings. These trimmed strings can make your code cleaner and more efficient.
This guide will walk you through the ins and outs of the Java String trim function, from basic usage to advanced techniques. We’ll explore the trim function’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Java String trim!
TL;DR: How Do Use the Java String trim() method??
You use the
trim()
method to trim a string in Java. This method removes leading and trailing white spaces from a string, making your code cleaner and more efficient.
Here’s a simple example:
String str = ' Hello World! ';
String trimmedStr = str.trim();
System.out.println(trimmedStr);
// Output:
// 'Hello World!'
In this example, we’ve created a string str
with leading and trailing spaces. We then use the trim()
method to remove these spaces, resulting in the string ‘Hello World!’.
This is just a basic way to use the
trim()
method in Java, but there’s much more to learn about string manipulation. Continue reading for a deeper understanding and more advanced usage scenarios.
Table of Contents
- Getting Started with Java String Trim
- Trimming Beyond Spaces in Java
- Exploring Alternatives to Java String Trim
- Troubleshooting Java String Trimming Issues
- Understanding Java’s String Class and White Spaces
- The Relevance of String Trimming in Data Cleaning and File Handling
- Exploring Related Concepts in Java
- Further Resources for Mastering Java String Manipulation
- Wrapping Up: Mastering Java’s String trim() Function
Getting Started with Java String Trim
The trim()
method in Java is a built-in function of the String class. It is designed to eliminate leading and trailing spaces from a string. The method works by checking and removing any white spaces at the beginning and end of the string.
Here’s a basic example:
String str = ' Welcome to Java! ';
String trimmedStr = str.trim();
System.out.println(trimmedStr);
// Output:
// 'Welcome to Java!'
In this example, the trim()
method is called on the string str
. The string initially has spaces at the beginning and end. After the trim()
method is applied, these spaces are removed, and the string ‘Welcome to Java!’ is printed.
This function is especially useful when dealing with user input, as users often unintentionally include white spaces that can cause errors or inconsistencies in the program.
However, it’s important to note that the trim()
method only removes spaces. It does not remove other white space characters such as tabs or newlines. If your string includes these types of white space characters, you’ll need to use a different approach, which we’ll explore in the next section.
Trimming Beyond Spaces in Java
While the trim()
function is excellent for removing leading and trailing spaces, it falls short when dealing with other types of white spaces like tabs or newlines. This limitation can be a hurdle when dealing with more complex strings.
Let’s explore an example where our string contains a tab and a newline character:
String str = ' Hello, Java!
';
String trimmedStr = str.trim();
System.out.println(trimmedStr);
// Output:
// ' Hello, Java!
'
In this case, the trim()
method fails to remove the tab () and newline (
) characters because it is designed to remove only space characters.
To handle these scenarios, we can use the replaceAll()
method in combination with a regular expression. This method replaces each substring of the string that matches the given regular expression with the given replacement.
Here’s how you can use replaceAll()
to remove all types of white spaces:
String str = ' Hello, Java!
';
String trimmedStr = str.replaceAll("\\s", "");
System.out.println(trimmedStr);
// Output:
// 'Hello,Java!'
In this example, \s
is a regular expression that matches any white space character, including space, tab, and newline. By using replaceAll()
, we are able to remove all these white space characters, not just spaces.
While this approach provides more control over the trimming process, it’s important to use it judiciously as it can potentially remove white spaces that are necessary for the string’s context. Understanding your data and choosing the right method for trimming is key to effective string manipulation in Java.
Exploring Alternatives to Java String Trim
While the trim()
method is a valuable tool for handling spaces in strings, Java offers other methods for string trimming, especially from Java 11 onwards. Let’s explore some of these alternatives and their effectiveness.
Using the strip()
Function in Java 11 and Onwards
Java 11 introduced the strip()
, stripLeading()
, and stripTrailing()
methods as part of the String class. These methods are Unicode-aware, meaning they consider all white space characters defined in the latest Unicode standard.
Here’s an example of how to use the strip()
method:
String str = ' Hello, Java!
';
String strippedStr = str.strip();
System.out.println(strippedStr);
// Output:
// 'Hello, Java!'
In this example, the strip()
method successfully removes the leading tab and trailing newline characters, demonstrating its effectiveness compared to the trim()
method.
Using Regular Expressions
As we saw earlier, we can use the replaceAll()
method with a regular expression to remove all types of white spaces. This method provides the most control over the trimming process but requires a good understanding of regular expressions.
String str = ' Hello, Java!
';
String trimmedStr = str.replaceAll("\\s", "");
System.out.println(trimmedStr);
// Output:
// 'Hello,Java!'
This method is powerful, but it removes all white spaces, not just leading and trailing ones. So, it’s important to use it judiciously.
Comparing the Methods
Method | Removes Spaces | Removes Other White Spaces | Available From |
---|---|---|---|
trim() | Yes | No | Java 1.0 |
strip() | Yes | Yes | Java 11 |
replaceAll() | Yes | Yes | Java 1.4 |
Each of these methods has its own advantages and disadvantages. The trim()
method is simple to use but only removes spaces. The strip()
method is Unicode-aware but is only available from Java 11 onwards. The replaceAll()
method provides the most control but can potentially remove necessary white spaces.
Troubleshooting Java String Trimming Issues
While Java’s string trimming methods are powerful tools, they come with their own set of issues. Let’s discuss some of these common problems and their solutions.
Not Removing Non-Space White Characters
As we’ve seen, the trim()
method only removes spaces. It does not remove other white space characters like tabs or newlines. This can lead to unexpected results if your string includes these types of white space characters.
Here’s an example:
String str = ' Hello, Java!
';
String trimmedStr = str.trim();
System.out.println(trimmedStr);
// Output:
// ' Hello, Java!
'
In this case, the trim()
method fails to remove the tab () and newline (
) characters. To handle this issue, you can use the
replaceAll()
method with a regular expression, as we discussed earlier.
Removing Necessary White Spaces
While the replaceAll()
method can remove all types of white spaces, it can sometimes remove white spaces that are necessary for the string’s context. This is especially true when using the replaceAll()
method with the \s
regular expression, which matches any white space character.
To avoid this issue, you can use the \s
regular expression in combination with ^
(start of line) and $
(end of line) to only remove leading and trailing white spaces, like so:
String str = ' Hello, Java!
';
String trimmedStr = str.replaceAll("^\\s+|\\s+$", "");
System.out.println(trimmedStr);
// Output:
// 'Hello, Java!
'
In this example, the replaceAll()
method only removes the leading and trailing spaces, preserving the tab and newline characters within the string.
Understanding these issues and their solutions can help you use Java’s string trimming methods more effectively and avoid common pitfalls.
Understanding Java’s String Class and White Spaces
To fully grasp the concept of string trimming in Java, it’s crucial to understand the fundamentals of Java’s String class and the concept of white spaces in programming.
Java’s String Class
In Java, strings are objects that are backed internally by a char array. The String class provides many methods for safely creating, manipulating, and comparing strings.
String greeting = "Hello, Java!";
System.out.println(greeting);
// Output:
// 'Hello, Java!'
In this example, we’ve created a string object using the String class. We can then use the various methods provided by the class to manipulate and compare this string.
White Spaces in Programming
In programming, white spaces refer to any character or series of characters that represent horizontal or vertical space. These include spaces, tabs, and newline characters. While they are often necessary for formatting and readability, they can cause issues when processing strings.
String str = ' Hello, Java!
';
System.out.println(str);
// Output:
// ' Hello, Java!
'
In this example, the string includes a space, a tab (), and a newline (
) character. While these white spaces are necessary for the string’s formatting, they can cause issues when processing the string, such as when comparing strings or parsing them into other data types.
Unicode and ASCII
Unicode and ASCII are character encoding standards that are used to represent text in computers and other devices that use text. ASCII only includes unaccented characters, numbers, and common symbols, while Unicode includes almost all characters from all writing systems, plus many symbols.
Understanding these concepts is key to understanding the underlying mechanisms of string trimming in Java. In the next section, we’ll discuss how these concepts are relevant to data cleaning and file handling.
The Relevance of String Trimming in Data Cleaning and File Handling
String trimming plays a crucial role in various areas of programming, particularly in data cleaning and file handling. Unwanted white spaces can lead to inconsistencies and errors in your data, affecting the accuracy of your analyses and the functionality of your applications.
Let’s consider a CSV file with strings that have leading and trailing spaces. When you read this file into a Java program, these spaces can affect the equality checks and other string manipulations, leading to inaccurate results. In these scenarios, using the trim()
method or its alternatives can help ensure the accuracy and reliability of your data.
String csvData = ' Hello, Java! , 100 , true ';
String[] data = csvData.split(",");
for (int i = 0; i < data.length; i++) {
data[i] = data[i].trim();
}
// Output:
// ['Hello, Java!', '100', 'true']
In this example, we’re reading a line from a CSV file and splitting it into an array of strings. We then use the trim()
method to remove the leading and trailing spaces from each string, ensuring that our data is clean and ready for further processing.
Exploring Related Concepts in Java
Mastering string trimming in Java opens the door to understanding more complex string manipulation techniques. For instance, you can delve into regular expressions, which provide powerful tools for string matching, replacing, and formatting. You might also explore other methods of the String class, such as substring()
, replace()
, and charAt()
, among others.
Further Resources for Mastering Java String Manipulation
To deepen your understanding of Java string manipulation and related concepts, consider exploring these resources:
- IOFlood’s Java String Class Guide – Explore Java String indexing and slicing.
Concatenating Strings in Java – Learn about the concatenation operator (+) and the concat method.
Java StringBuilder: Usage Guide – Explore the StringBuilder class in Java for efficient string manipulation.
Java’s Official String Documentation provides a comprehensive overview of the String class and its methods.
Java Regular Expressions Tutorial by Vogella provides a detailed guide to using regular expressions in Java.
Java String Handling Tutorial – Series of tutorials on various aspects of string handling in Java, including manipulation methods.
Wrapping Up: Mastering Java’s String trim() Function
In this comprehensive guide, we’ve delved into the world of Java’s String trim function, a powerful tool for removing unwanted spaces from your strings.
We began with the basics, learning how to use the trim()
function to remove leading and trailing spaces from a string. We then explored more advanced usage scenarios, such as handling different types of white spaces and using regular expressions for more control over the trimming process.
Along the way, we tackled common issues you might encounter when using the trim()
function, such as not removing non-space white characters and unintentionally removing necessary white spaces. We provided solutions and workarounds for each issue, equipping you with the knowledge to handle these challenges effectively.
We also explored alternative approaches to string trimming, such as using the strip()
function in Java 11 and onwards, and using the replaceAll()
method with a regular expression. Here’s a quick comparison of these methods:
Method | Removes Spaces | Removes Other White Spaces | Available From |
---|---|---|---|
trim() | Yes | No | Java 1.0 |
strip() | Yes | Yes | Java 11 |
replaceAll() | Yes | Yes | Java 1.4 |
Whether you’re just starting out with Java’s String trim function or you’re looking to deepen your understanding, we hope this guide has given you a comprehensive overview of its usage, common issues, and their solutions.
With its balance of simplicity and control, the trim()
function is a powerful tool for string manipulation in Java. Happy coding!