Java StringBuilder Class: Methods for String Manipulation
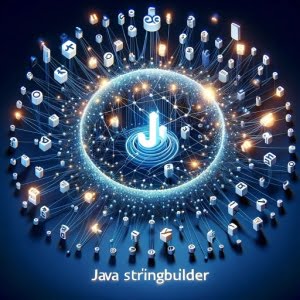
Are you finding it challenging to manipulate strings in Java? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Like a skilled craftsman, Java’s StringBuilder class can help you efficiently construct and modify strings. These strings can be manipulated in a variety of ways, even in complex scenarios.
This guide will walk you through using StringBuilder in Java. We’ll explore StringBuilder’s core functionality, delve into its advanced features, and even discuss when to use it over other options like String and StringBuffer.
So, let’s dive in and start mastering StringBuilder in Java!
TL;DR: How Do I Use StringBuilder in Java?
To use StringBuilder in Java, you create an instance of the StringBuilder class with the syntax,
StringBuilder sb = new StringBuilder()
. You can then use its methods to manipulate strings, such as:append(), insert(), replace(), and delete()
.
Here’s a simple example:
StringBuilder sb = new StringBuilder();
sb.append('Hello');
sb.append(' World');
String result = sb.toString();
// Output:
// 'Hello World'
In this example, we create an instance of the StringBuilder class (sb
). We then use the append()
method to add ‘Hello’ and ‘ World’ to our StringBuilder. Finally, we convert our StringBuilder back to a String using the toString()
method. The result is the string ‘Hello World’.
This is a basic way to use StringBuilder in Java, but there’s much more to learn about string manipulation in Java. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
Getting Started with StringBuilder
The first step in using the StringBuilder class is to create an instance of it. This process is known as instantiation. Here’s how you can do it:
StringBuilder sb = new StringBuilder();
In this example, sb
is an instance of the StringBuilder class. Now that we have our StringBuilder, we can start manipulating strings using its methods.
The append() Method
The append()
method is one of the most commonly used methods in the StringBuilder class. It allows you to add (or append) a string to the end of the StringBuilder. Here’s how you can use it:
StringBuilder sb = new StringBuilder();
sb.append('Hello');
sb.append(' World');
// Output:
// 'Hello World'
In this example, we append ‘Hello’ and ‘ World’ to our StringBuilder. The append()
method is efficient and easy to use, making it a staple in many Java programs.
The insert() Method
The insert()
method allows you to insert a string at a specific position in the StringBuilder. Here’s how you can use it:
StringBuilder sb = new StringBuilder('Hello');
sb.insert(5, ' World');
// Output:
// 'Hello World'
In this example, we insert ‘ World’ at position 5 in our StringBuilder. The insert()
method is powerful, but you must be careful to avoid IndexOutOfBoundsException.
The delete() Method
The delete()
method allows you to remove a substring from the StringBuilder. Here’s how you can use it:
StringBuilder sb = new StringBuilder('Hello World');
sb.delete(5, 6);
// Output:
// 'HelloWorld'
In this example, we remove the space between ‘Hello’ and ‘World’ using the delete()
method. This method is useful for removing unwanted characters or substrings from a StringBuilder.
The toString() Method
Finally, the toString()
method allows you to convert a StringBuilder back to a String. Here’s how you can use it:
StringBuilder sb = new StringBuilder('Hello World');
String result = sb.toString();
// Output:
// 'Hello World'
In this example, we convert our StringBuilder back to a String using the toString()
method. This is a crucial step, as many Java methods require strings, not StringBuilders.
These are the basic methods you need to understand to start using StringBuilder effectively in Java. Each has its own advantages and potential pitfalls, but with practice, you can use them to manipulate strings efficiently and effectively.
Advanced StringBuilder Techniques
As you become more comfortable with StringBuilder, you can start to use it in more complex ways. Let’s take a look at a few advanced techniques.
Manipulating Large Strings
StringBuilder is particularly useful when dealing with large strings in Java. Let’s say we want to create a string that contains the numbers from 1 to 1000, separated by commas.
StringBuilder sb = new StringBuilder();
for (int i = 1; i <= 1000; i++) {
sb.append(i).append(',');
}
String result = sb.toString();
// Output: '1,2,3,...,999,1000,'
In this example, we use a for loop to append the numbers 1 through 1000 to our StringBuilder, each followed by a comma. This is much more efficient than concatenating strings using the ‘+’ operator, especially for large strings.
Using StringBuilder with Loops
StringBuilder can be used effectively with loops to manipulate strings. For example, you can reverse a string using StringBuilder and a loop.
StringBuilder sb = new StringBuilder('Hello World');
StringBuilder reverse = new StringBuilder();
for (int i = sb.length() - 1; i >= 0; i--) {
reverse.append(sb.charAt(i));
}
String result = reverse.toString();
// Output: 'dlroW olleH'
In this example, we create a new StringBuilder reverse
and use a for loop to append the characters from sb
in reverse order. The result is the reverse of the original string.
Handling Synchronization Issues
StringBuilder is not synchronized, meaning it is not thread-safe. If you need to use StringBuilder in a multi-threaded environment, you should consider using StringBuffer instead, which is a synchronized (thread-safe) version of StringBuilder.
However, if you must use StringBuilder in a multi-threaded environment, you can synchronize it manually using the synchronized
keyword.
StringBuilder sb = new StringBuilder();
synchronized(sb) {
sb.append('Hello');
sb.append(' World');
}
String result = sb.toString();
// Output: 'Hello World'
In this example, we use the synchronized
keyword to ensure that only one thread can access the StringBuilder at a time. This can help prevent race conditions and other concurrency issues.
These are just a few examples of how you can use StringBuilder in more advanced ways. With practice, you can use StringBuilder to efficiently manipulate strings in a variety of complex scenarios.
Comparing StringBuilder, String, and StringBuffer
While StringBuilder is a powerful tool for string manipulation in Java, it’s not the only option. Depending on your needs, you might find that String or StringBuffer is a better fit. Let’s compare these three classes and discuss when you might want to use each one.
Performance Considerations
When it comes to performance, StringBuilder is generally the fastest option for string manipulation, especially for large strings. This is because StringBuilder is mutable, meaning it can be changed after it’s created. This allows StringBuilder to append, insert, or delete characters without creating a new string each time.
On the other hand, String is immutable, meaning it cannot be changed after it’s created. This means that every time you append, insert, or delete characters from a String, a new String is created. This can be inefficient, especially for large strings or in loops.
Here’s a simple example that demonstrates the performance difference between String and StringBuilder:
long startTime = System.currentTimeMillis();
// Using String
String str = '';
for (int i = 0; i < 10000; i++) {
str += 'Hello';
}
long endTime = System.currentTimeMillis();
System.out.println('Time using String: ' + (endTime - startTime) + 'ms');
startTime = System.currentTimeMillis();
// Using StringBuilder
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 10000; i++) {
sb.append('Hello');
}
endTime = System.currentTimeMillis();
System.out.println('Time using StringBuilder: ' + (endTime - startTime) + 'ms');
In this example, we use a loop to append ‘Hello’ to a String and a StringBuilder 10,000 times, and measure the time taken for each operation. You’ll find that using StringBuilder is significantly faster.
Thread-Safety Considerations
While StringBuilder is faster, it’s not synchronized, meaning it’s not thread-safe. If you’re working in a multi-threaded environment and need to manipulate strings, you might want to use StringBuffer instead.
StringBuffer is a synchronized (thread-safe) version of StringBuilder. It has the same methods as StringBuilder, but they’re synchronized to ensure that only one thread can access the StringBuffer at a time.
Here’s an example of how you might use StringBuffer in a multi-threaded environment:
StringBuffer sb = new StringBuffer();
Thread t1 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
sb.append('Hello');
}
});
Thread t2 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
sb.append('World');
}
});
t1.start();
t2.start();
t1.join();
t2.join();
System.out.println(sb.length());
In this example, we create two threads that append ‘Hello’ and ‘World’ to a StringBuffer 1,000 times, respectively. Because StringBuffer is thread-safe, we can be sure that our final string will be correct, even with multiple threads.
In conclusion, while StringBuilder is generally the fastest option for string manipulation in Java, you might want to consider using String or StringBuffer depending on your needs. Understanding the differences between these classes can help you make informed decisions about which one to use in different scenarios.
Troubleshooting StringBuilder Issues
While StringBuilder is a powerful class for string manipulation in Java, there are potential pitfalls and issues that might arise. Let’s discuss some of the common ones and how to address them.
Handling Large Strings
StringBuilder is an excellent tool for handling large strings, but there are still potential issues to be aware of. For instance, if you’re appending a large number of strings to a StringBuilder, you might run into memory issues.
One solution is to clear the StringBuilder periodically if you don’t need to keep all the data in memory. You can do this using the setLength()
method:
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000000; i++) {
sb.append('Hello');
if (i % 1000 == 0) {
process(sb.toString());
sb.setLength(0);
}
}
// process() is a placeholder for your actual processing code
In this example, we append ‘Hello’ to the StringBuilder in a loop. Every 1000 iterations, we process the current string and then clear the StringBuilder using sb.setLength(0)
.
Multi-Threading Issues
StringBuilder is not thread-safe. That means if you’re using StringBuilder in a multi-threaded environment, you might run into issues if multiple threads try to access the same StringBuilder at the same time.
One solution is to use StringBuffer instead, which is a thread-safe version of StringBuilder. However, if you need the performance of StringBuilder, you can also synchronize it manually:
StringBuilder sb = new StringBuilder();
synchronized(sb) {
sb.append('Hello');
sb.append(' World');
}
String result = sb.toString();
// Output: 'Hello World'
In this example, we use the synchronized
keyword to ensure that only one thread can access the StringBuilder at a time. This can help prevent race conditions and other concurrency issues.
These are just a couple of examples of issues you might run into when using StringBuilder, along with some potential solutions. With a good understanding of these issues and how to address them, you can use StringBuilder effectively even in complex scenarios.
Understanding Strings in Java
Before we delve deeper into StringBuilder and its counterparts, it’s crucial to understand how strings work in Java. A string, in essence, is a sequence of characters. In Java, strings are objects of the String class. Here’s a simple example of a string in Java:
String str = 'Hello World';
// Output: 'Hello World'
In this example, str
is a string that contains ‘Hello World’.
The Immutability of Strings
One of the key characteristics of strings in Java is that they are immutable. This means that once a String object is created, it cannot be changed. Any operation that seems to modify a String actually creates a new String.
Here’s an example that demonstrates this:
String str = 'Hello';
str += ' World';
// Output: 'Hello World'
In this example, it might seem like we’re modifying str
, but we’re actually creating a new String that contains ‘Hello World’. The original ‘Hello’ String is left unchanged.
The Need for StringBuilder and StringBuffer
Because String objects are immutable, they can be inefficient for operations that modify strings, especially for large strings or in loops. This is where StringBuilder and StringBuffer come in.
StringBuilder and StringBuffer are mutable classes in Java. This means that they can be changed after they’re created. This allows them to append, insert, or delete characters without creating a new string each time, making them more efficient for string manipulation.
The Concept of String Pooling
Another important concept in Java is string pooling. String pooling is a mechanism by which Java saves memory by reusing common strings.
When you create a string using a string literal (like ‘Hello’), Java first checks the string pool to see if the same string already exists. If it does, Java reuses that string instead of creating a new one. If not, Java creates a new string and adds it to the pool.
Here’s an example that demonstrates this:
String str1 = 'Hello';
String str2 = 'Hello';
System.out.println(str1 == str2);
// Output: true
In this example, str1
and str2
both refer to the same ‘Hello’ string in the string pool. That’s why str1 == str2
returns true.
Understanding these fundamental concepts can help you understand why StringBuilder is useful and when to use it over String and StringBuffer.
Applying StringBuilder in Real-World Scenarios
Now that we have a good understanding of StringBuilder and its functionality, let’s explore how it can be applied in real-world scenarios. StringBuilder holds significant value in various areas, including file I/O, networking, and large-scale data processing.
StringBuilder in File I/O
File I/O (Input/Output) operations often involve reading from or writing to a file. In such scenarios, the data is usually handled as a string. StringBuilder can be used to construct or modify these strings efficiently.
Here’s a simple example where we read a file line by line and append each line to a StringBuilder:
StringBuilder sb = new StringBuilder();
try (BufferedReader br = new BufferedReader(new FileReader('file.txt'))) {
String line;
while ((line = br.readLine()) != null) {
sb.append(line).append('
');
}
} catch (IOException e) {
e.printStackTrace();
}
// Output: Contents of the file, with each line separated by a newline
In this example, we use a BufferedReader to read the file line by line. Each line is then appended to the StringBuilder, followed by a newline character.
StringBuilder in Networking
In networking, data is often sent or received as strings. StringBuilder can be used to construct or modify these strings. For example, when receiving data over a network, you might need to construct a string from the incoming data packets. StringBuilder can help you do this efficiently.
StringBuilder in Large-Scale Data Processing
In large-scale data processing, you often need to manipulate large amounts of string data. StringBuilder can be used to handle this data efficiently, making your programs faster and more memory-efficient.
Further Resources for Mastering StringBuilder
For a complete reference guide on the String Class in Java, you can Click Here. And for further information on other Java String topics, here are some additional resources:
- Java Empty String Explained – Master techniques for handling empty strings gracefully in Java applications.
Java String Trimming Techniques – Master string trimming techniques for data cleaning and validation in Java.
Oracle’s Official Java Documentation provides detailed information about the class and its methods.
GeeksforGeeks’ StringBuilder in Java provides a detailed overview of StringBuilder.
Baeldung’s Guide to Java’s StringBuilder discusses the differences between StringBuilder and StringBuffer.
Wrapping Up: Java’s StringBuilder Class
In this comprehensive guide, we’ve traversed the landscape of Java’s StringBuilder class, an efficient tool for string manipulation in Java. We’ve explored its methods, delved into its use cases, and compared it with its counterparts, String and StringBuffer.
We started with the basics, learning how to instantiate a StringBuilder object and use its core methods like append(), insert(), delete(), and toString(). We then ventured into more complex scenarios, discussing how to handle large strings, use StringBuilder with loops, and address synchronization issues. We also covered some common issues you might encounter when using StringBuilder and provided solutions to these problems.
Along the way, we compared StringBuilder with String and StringBuffer, highlighting the performance and thread-safety considerations that might influence your choice of which to use. Here’s a quick comparison of these classes:
Class | Mutability | Thread-Safety | Performance |
---|---|---|---|
StringBuilder | Mutable | Not Thread-Safe | High |
String | Immutable | Thread-Safe | Moderate |
StringBuffer | Mutable | Thread-Safe | Moderate |
Whether you’re just starting out with StringBuilder or you’re looking to deepen your understanding, we hope this guide has provided you with a solid foundation and a deeper understanding of StringBuilder and its capabilities.
Understanding how to efficiently manipulate strings is a crucial skill in Java programming. With the power of StringBuilder, you’re well-equipped to handle even the most complex string manipulation tasks. Happy coding!