How to Find Length of String Java | Effective Methods
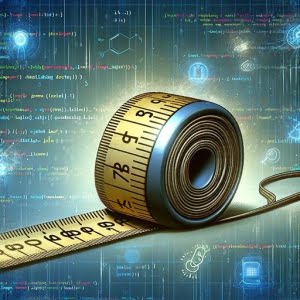
Calculating the length of string in Java is a useful technique to ensure proper text formatting in our web developments projects at IOFLOOD. This operation helps in various aspects of data handling and consistent java string size checks. As we know our customer’s also utilize their Dedicated bare metal servers to develop web applications, we have populated today’s article with helpful step-by-step instructions.
In this guide, we will walk you through the process of finding Java string length, from the basics to more advanced techniques. We’ll cover everything from using the length()
method, dealing with different types of characters, to alternative approaches and common issues you might encounter.
So, let’s dive in to String length Java methods!
TL;DR: How Do I Find the Length of String in Java?
The simplest way to find the length of a string in Java is to use the
length()
method of the String class,int len = str.length();
This method returns the number of characters in the string.
Here’s a quick example:
String str = "Hello World";
int len = str.length();
System.out.println(len);
// Output:
// 11
In this example, we have a string str
with the value “Hello World”. We use the length()
method to find the length of the string and store it in the integer len
. The length of the string is then printed to the console, resulting in the output ’11’.
This is just a basic way to find the length of a string in Java. There’s much more to learn about string manipulation in Java, including dealing with different types of characters and alternative methods for finding string length. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
Basic Java String Size Method
The length()
method is a built-in function in Java that belongs to the String class. Its primary function is to return the number of characters present in a string, thus allowing you to measure its length. This method is particularly useful when you need to perform operations based on the length of the string, such as looping through each character or validating user input.
Let’s look at a simple example:
String message = "Hello, Java!";
int length = message.length();
System.out.println("The length of the string is: " + length);
// Output:
// The length of the string is: 12
In this example, we have a string message
that contains the text “Hello, Java!”. We then use the length()
method to find the length of the string and store it in the variable length
. The system then prints out a message indicating the length of the string.
One of the main advantages of the length()
method is its simplicity and ease of use. It provides a straightforward way to measure the length of a string, making it a fundamental tool for any Java programmer.
It’s important to be aware of potential pitfalls. For instance, the
length()
method counts all characters, including spaces and punctuation. So, if you need to count only specific characters, you’ll need to use a different approach, which we’ll discuss later.
Another thing to note is that the length()
method will throw a NullPointerException
if you try to use it on a null string. Always ensure that your string is not null before trying to find its length.
Unicode Characters in Length Java
When working with strings in Java, you may come across different types of characters, including Unicode characters. Unicode is a universal character encoding standard that includes characters from almost all written languages. It’s important to understand how these characters affect the length of a string in Java.
Let’s explore this with an example:
String unicodeStr = "Hello, 你好!";
int length = unicodeStr.length();
System.out.println("The length of the string is: " + length);
// Output:
// The length of the string is: 9
In this example, the string unicodeStr
contains both English and Chinese characters. When we use the length()
method, it returns ‘9’. This is because the length()
method counts Unicode characters as a single character, regardless of their byte size.
This behavior is beneficial in most cases, as it provides a consistent way to measure the length of a string, irrespective of the languages used. However, it’s crucial to be aware of this when working with Unicode strings, especially when memory usage is a concern.
Best Practices
When using the length()
method with Unicode strings, always remember:
- The
length()
method counts each Unicode character as one, irrespective of its byte size. - Be mindful of memory usage when working with large Unicode strings.
- Always validate that your string is not null before using the
length()
method to avoidNullPointerException
.
Understanding these nuances will help you effectively measure the length of strings in Java, regardless of the types of characters they contain.
Other String Length Java Methods
While the length()
method is the most common way to find the length of a string in Java, there are other methods that can be used, especially when dealing with more complex scenarios. Let’s explore some of these alternative approaches.
The getChars()
Method
The getChars()
method is another way to determine the length of a string. This method copies the characters from a string to an array, allowing you to count the number of characters in the array.
String str = "Hello, Java!";
char[] charArray = new char[str.length()];
str.getChars(0, str.length(), charArray, 0);
int length = charArray.length;
System.out.println("The length of the string is: " + length);
// Output:
// The length of the string is: 12
In this example, we first create a character array charArray
with the same length as the string str
. We then use the getChars()
method to copy the characters from the string to the array. Finally, we find the length of the array using the length
property.
Third-Party Libraries
There are also third-party libraries, such as Apache Commons Lang, which provide utilities to measure the length of a string. These libraries offer more advanced features, but they also add extra dependencies to your project.
// Requires: Apache Commons Lang
import org.apache.commons.lang3.StringUtils;
String str = "Hello, Java!";
int length = StringUtils.length(str);
System.out.println("The length of the string is: " + length);
// Output:
// The length of the string is: 12
In this example, we use the StringUtils.length()
method from the Apache Commons Lang library to find the length of the string.
Comparison of Methods
Method | Advantages | Disadvantages |
---|---|---|
length() | Simple, straightforward, no extra dependencies | Counts all characters, can throw NullPointerException |
getChars() | Can exclude certain characters from count | More complex, requires an array |
Third-party libraries | Advanced features, can handle more complex scenarios | Adds extra dependencies |
While the length()
method is suitable for most scenarios, the getChars()
method and third-party libraries offer more flexibility and advanced features. The choice of method depends on your specific needs and the complexity of the string you’re working with.
Troubleshooting: Length Java
While finding the length of a string in Java is generally straightforward, there are common issues that developers may encounter. It’s essential to know how to navigate these issues to effectively work with strings in Java.
Null Strings
One common issue is dealing with null strings. If you attempt to use the length()
method on a null string, Java will throw a NullPointerException
. To avoid this, it’s good practice to always check if a string is null before trying to find its length.
String str = null;
if (str != null) {
int length = str.length();
System.out.println("The length of the string is: " + length);
} else {
System.out.println("The string is null.");
}
// Output:
// The string is null.
In this example, we first check if the string str
is not null before calling the length()
method. If the string is null, we print a message indicating that the string is null.
White Space
Another important consideration is that the length()
method counts all characters in the string, including white spaces. This could lead to unexpected results if you’re not aware of it.
String str = " Hello, Java! ";
int length = str.length();
System.out.println("The length of the string is: " + length);
// Output:
// The length of the string is: 18
In this example, the string str
contains leading and trailing white spaces. The length()
method counts these white spaces, resulting in a length of ’18’ instead of ’12’. If you want to exclude white spaces when finding the length of a string, you can use the trim
() method to remove them before calling length()
.
Understanding Java String Class
In Java, a string is an object that represents a sequence of characters. The String class is a built-in class in Java, and it includes various methods for manipulating and working with strings. The length()
method, which we’ve been discussing, is one of these methods.
String str = "Hello, Java!";
System.out.println(str.length());
// Output:
// 12
In this example, we create a string str
and use the length()
method to print its length. The length()
method returns the number of characters in the string, including spaces and punctuation.
Practical Uses: Length of String Java
Let’s explore a few practical applications for finding the length of a string in Java .
Data Validation
One common use of string length is in data validation. For example, you may want to ensure that a user’s password meets a certain length requirement for security purposes.
String password = "password123";
if (password.length() < 8) {
System.out.println("Password is too short.");
} else {
System.out.println("Password length is acceptable.");
}
// Output:
// Password length is acceptable.
In this example, we check the length of a password string to ensure it meets a minimum length requirement.
Java String Manipulation
Another area where length of string Java is useful is in string manipulation. For example, you may need to extract a substring from a string based on its length.
String str = "Hello, Java!";
String substring = str.substring(0, 5);
System.out.println(substring);
// Output:
// Hello
In this example, we use the substring()
method along with the string length to extract the first five characters from a string.
Concepts Related to String Length Java
Once you’ve mastered finding the length of a string in Java, there are other related concepts to explore, such as string comparison and string concatenation. These concepts further expand your ability to manipulate and work with strings in Java.
More Info on Java String Length
To deepen your understanding of working with strings in Java, here are some additional resources:
- Simplifying Best Practices for Strings in Java – Discover how to handle special characters and escape sequences in Java strings.
String Methods in Java – Explore essential methods available for string manipulation and analysis in Java.
Java String Size Equality – Understand the differences between reference equality and value equality when comparing strings.
Oracle’s Java Documentation on Strings provides a comprehensive overview of the String class and its methods.
Length of String Java Method by GeeksforGeeks provides a dedicated tutorial on the string length method in Java.
Java String length() Method by TutorialsPoint explains the use of the length method in Java strings.
Recap: Finding Length of String Java
In this comprehensive guide, we’ve delved into the process of finding the length of a string in Java, exploring the various methods and nuances that come into play.
We began with the basics, discussing how to use the length()
method, a simple yet powerful tool in any Java developer’s toolkit. We then expanded our knowledge to more advanced topics, discussing how to handle Unicode characters and the impact they have on string length.
We didn’t stop there. We also explored alternative approaches to finding string length, such as using the getChars()
method and third-party libraries like Apache Commons Lang. We discussed the advantages and disadvantages of each method, giving you a broader understanding of the options available.
Method | Advantages | Disadvantages |
---|---|---|
length() | Simple and straightforward | Can throw a NullPointerException on null strings |
getChars() | Can exclude certain characters | Requires an array, more complex |
Third-party libraries | Advanced features | Adds extra dependencies |
We also addressed common issues that developers may encounter when working with string lengths, such as dealing with null strings and the impact of white spaces. This knowledge will help you navigate these issues and build more robust Java applications.
Whether you’re a beginner just starting out with Java or an experienced developer looking to brush up your skills, we hope this guide has provided a comprehensive understanding of how to find the length of a string in Java. With this knowledge, you’re well-equipped to handle any string length scenario that comes your way. Happy coding!