StringUtils.isEmpty() in Java: Your Method Guide
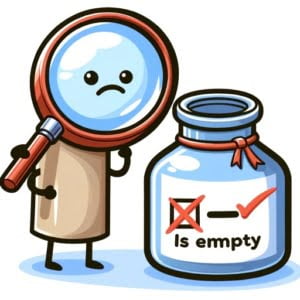
Are you finding it challenging to use StringUtils.isEmpty in Java? You’re not alone. Many developers struggle with this task, but there’s a tool that can make this process a breeze.
Like a diligent proofreader, StringUtils.isEmpty checks if a given string is empty or null. This method is a part of the Apache Commons Lang library, which provides a host of helper utilities for the java.lang API, making our programming lives easier.
This guide will walk you through the usage of StringUtils.isEmpty, from basic to advanced scenarios. We’ll explore StringUtils.isEmpty’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering StringUtils.isEmpty in Java!
TL;DR: How Do I Use StringUtils.isEmpty in Java?
StringUtils.isEmpty is a method in the Apache Commons Lang library that checks if a given string is empty or null. Used with the syntax,
boolean isEmpty = StringUtils.isEmpty(sampleString);
, It’s a handy tool for validating and handling strings in Java.
Here’s a simple example:
String str = "";
boolean isEmpty = StringUtils.isEmpty(str);
System.out.println(isEmpty);
# Output:
# true
In this example, we’ve created a string str
and assigned it an empty value. We then use StringUtils.isEmpty(str)
to check if str
is empty or null. The method returns true
, indicating that the string is indeed empty.
This is just a basic way to use StringUtils.isEmpty in Java, but there’s much more to learn about handling strings efficiently. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Basic Use of StringUtils.isEmpty in Java
- Advanced StringUtils.isEmpty Usage
- Exploring Alternatives to StringUtils.isEmpty
- Troubleshooting StringUtils.isEmpty
- Digging Deeper: StringUtils Class and Null Strings
- The Relevance of StringUtils.isEmpty in Java Applications
- Wrapping Up: Mastering StringUtils.isEmpty in Java
Basic Use of StringUtils.isEmpty in Java
At its core, StringUtils.isEmpty is a simple yet powerful tool in Java for checking if a string is empty or null. It’s a part of the Apache Commons Lang library, a package filled with utility classes that are ‘must-haves’ for any Java programmer’s toolkit.
Let’s look at a basic example of StringUtils.isEmpty in action:
String str = "Hello, world!";
boolean isEmpty = StringUtils.isEmpty(str);
System.out.println(isEmpty);
# Output:
# false
In this example, we have a string str
with the value Hello, world!
. We then use StringUtils.isEmpty(str)
to check if str
is empty or null. The method returns false
, indicating that the string is not empty.
Advantages of StringUtils.isEmpty
One of the primary advantages of using StringUtils.isEmpty is its null-safe operation. Unlike the String.isEmpty()
method, which throws a NullPointerException if the string is null, StringUtils.isEmpty gracefully handles null values and returns true.
Potential Pitfalls
While StringUtils.isEmpty is a handy tool, it’s important to remember that it only checks whether a string is empty or null. It does not check for strings that are filled with whitespace. For that, you will need to use StringUtils.isBlank()
method, which we will cover in the advanced use section.
Advanced StringUtils.isEmpty Usage
As you become more familiar with StringUtils.isEmpty, you’ll find that there are scenarios where a string might not technically be ’empty’, but it might as well be. For example, a string full of whitespace characters. StringUtils.isEmpty would return false
for such a string, but for practical purposes, you might want it to be considered ’empty’.
Checking for Whitespace-Only Strings with StringUtils.isBlank
In such cases, you can use the StringUtils.isBlank()
method. This method not only checks if a string is empty or null, but it also checks if it is whitespace. Let’s illustrate this with a code example:
String str = " ";
boolean isEmpty = StringUtils.isEmpty(str);
boolean isBlank = StringUtils.isBlank(str);
System.out.println("isEmpty: " + isEmpty);
System.out.println("isBlank: " + isBlank);
# Output:
# isEmpty: false
# isBlank: true
In this example, str
is a string that consists of whitespace characters. StringUtils.isEmpty(str)
returns false
because the string is not technically ’empty’. However, StringUtils.isBlank(str)
returns true
, indicating that the string is either empty, null, or whitespace.
Best Practices
When dealing with strings in Java, it’s important to consider the context. If you only want to check if a string is null or truly empty, use StringUtils.isEmpty()
. However, if you also want to treat strings that only contain whitespace as ’empty’, use StringUtils.isBlank()
. Understanding the difference between these two methods and using them appropriately will help you write more robust and bug-free code.
Exploring Alternatives to StringUtils.isEmpty
While StringUtils.isEmpty is a powerful tool for checking if a string is empty or null, Java provides other methods that can be used for the same purpose. These alternatives, such as the equals
method or the length
method of the String class, can be useful in different contexts. Let’s explore these alternatives and their effectiveness.
Using the Equals Method
One way to check if a string is empty is by using the equals
method of the String class. Here’s an example:
String str = "";
boolean isEmpty = str.equals("");
System.out.println(isEmpty);
# Output:
# true
In this example, we’re checking if the string str
is equal to an empty string. The equals
method returns true
, indicating that the string is empty. However, the equals
method is not null-safe and would throw a NullPointerException if str
is null.
Using the Length Method
Another alternative is to use the length
method of the String class. If a string is empty, its length would be 0. Here’s how you can use the length
method to check if a string is empty:
String str = "";
boolean isEmpty = str.length() == 0;
System.out.println(isEmpty);
# Output:
# true
In this example, we’re checking if the length of the string str
is 0. The length
method returns true
, indicating that the string is empty. Similar to the equals
method, the length
method is not null-safe and would throw a NullPointerException if str
is null.
Recommendations
While these alternatives can be useful in some scenarios, they are not null-safe and can lead to unexpected errors if not used carefully. StringUtils.isEmpty, on the other hand, is null-safe and can handle both empty and null strings gracefully. Therefore, it’s generally recommended to use StringUtils.isEmpty when checking if a string is empty or null in Java.
Troubleshooting StringUtils.isEmpty
While StringUtils.isEmpty is a reliable tool for checking if a string is empty or null, you may encounter some issues during its use. Let’s discuss some common problems and their solutions.
Dealing with NullPointerException
One common issue is the NullPointerException. This error occurs when the StringUtils class is not properly imported, and you try to use the StringUtils.isEmpty method. Here’s an example:
import org.apache.commons.lang3.StringUtils;
public class Main {
public static void main(String[] args) {
String str = null;
boolean isEmpty = StringUtils.isEmpty(str);
System.out.println(isEmpty);
}
}
# Output:
# true
In this code, we’re trying to check if a null string is empty using StringUtils.isEmpty. However, if the StringUtils class is not properly imported, this code will throw a NullPointerException.
Solutions and Workarounds
To fix this issue, ensure that the StringUtils class is properly imported at the beginning of your code. If you’re using an IDE like Eclipse or IntelliJ IDEA, it should automatically suggest the correct import statement.
Another common problem is forgetting to include the Apache Commons Lang library in your project. If the library is not included, the StringUtils class and its methods will not be available for use. You can add the library to your project by including it in your project’s build path or by adding it as a dependency in your project’s build file if you’re using a build tool like Maven or Gradle.
Remember, StringUtils.isEmpty is a powerful tool for checking if a string is empty or null, but like any tool, it’s important to use it correctly. By understanding its potential issues and how to solve them, you can use StringUtils.isEmpty effectively and efficiently in your Java projects.
Digging Deeper: StringUtils Class and Null Strings
Before we dive into more complex scenarios, it’s important to understand the basics. Let’s take a closer look at the StringUtils class in the Apache Commons Lang library and the concept of empty and null strings in Java.
The StringUtils Class
The StringUtils class is a part of the Apache Commons Lang library, a package that provides helper utilities for the java.lang API. StringUtils contains static methods for handling strings and is considered a staple in a Java developer’s toolkit.
Here’s an example of importing the StringUtils class in your Java code:
import org.apache.commons.lang3.StringUtils;
With this import statement, you can now use any of the methods provided by StringUtils, including isEmpty
, isBlank
, and many others.
Empty and Null Strings in Java
In Java, a string is considered empty if it is not null and its length is zero. In other words, an empty string is a string instance of zero length.
Here’s an example of an empty string in Java:
String str = "";
On the other hand, a null string in Java is one that holds no value. It’s not the same as an empty string, which is a string with no characters. A null string simply means the absence of a value or that no value has been assigned yet.
Here’s an example of a null string in Java:
String str = null;
Understanding the difference between null and empty strings is crucial when working with StringUtils.isEmpty, as this method checks for both conditions. It returns true
if the string is either null or empty, and false
otherwise.
The Relevance of StringUtils.isEmpty in Java Applications
StringUtils.isEmpty plays a crucial role in string manipulation tasks in Java applications. It’s an invaluable tool for validating and handling strings, ensuring that your code doesn’t break when encountering null or empty strings.
Exploring Related Concepts
Once you have a solid understanding of StringUtils.isEmpty, it’s beneficial to explore related concepts like string comparison and string manipulation. These topics delve deeper into handling strings in Java and can help you write more efficient and robust code.
For instance, string comparison in Java involves comparing two strings lexicographically, and it’s a common operation in sorting, searching, and many other algorithms. On the other hand, string manipulation encompasses a variety of operations you can perform on strings, such as concatenation, substring, replacement, and many others.
Further Resources for Mastering StringUtils.isEmpty
To deepen your understanding of StringUtils.isEmpty and related concepts, here are some recommended resources:
- IOFlood’s Java String Guide explores the Java String class’s methods and usage cases.
Formatting Strings in Java – Master formatting data for display, logging, and data interchange in Java applications.
String Contains in Java – Learn how to check if a string contains a specific substring in Java using the contains() method.
Apache Commons Lang Documentation – The official Apache Commons Lang library resource, which includes StringUtils.
Guide to isEmpty Method provides a detailed overview of the isEmpty method and more.
Check Empty String Tutorial offers an in-depth look at checking empty strings.
By exploring these resources and practicing regularly, you can master StringUtils.isEmpty and enhance your Java coding skills.
Wrapping Up: Mastering StringUtils.isEmpty in Java
In this comprehensive guide, we’ve explored the ins and outs of StringUtils.isEmpty in Java, a method that checks if a string is empty or null.
We began with the basics, learning how to use StringUtils.isEmpty in simple scenarios. We then delved into more advanced usage, exploring how to handle strings that are filled with whitespace characters using StringUtils.isBlank. We also discussed alternative approaches to checking if a string is empty, such as using the equals
method or the length
method of the String class.
Along the way, we tackled common challenges you might encounter when using StringUtils.isEmpty, such as NullPointerExceptions, and provided solutions to help you overcome these hurdles.
Here’s a quick comparison of the methods we’ve discussed:
Method | Null-Safe | Checks for Whitespace | Complexity |
---|---|---|---|
StringUtils.isEmpty | Yes | No | Low |
StringUtils.isBlank | Yes | Yes | Low |
equals method | No | No | Low |
length method | No | No | Low |
Whether you’re just starting out with StringUtils.isEmpty or you’re looking to level up your Java skills, we hope this guide has given you a deeper understanding of StringUtils.isEmpty and its capabilities.
With its null-safe operation and ease of use, StringUtils.isEmpty is a powerful tool for handling strings in Java. Now, you’re well equipped to handle null and empty strings in your Java projects. Happy coding!