Java String Contains(): A Detailed Usage Guide
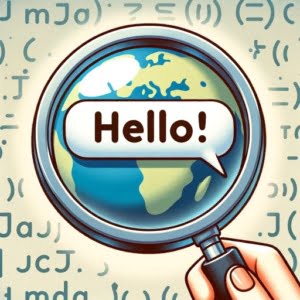
Are you finding it challenging to check if a string contains a certain sequence of characters in Java? You’re not alone. Many developers find themselves puzzled when it comes to using the ‘contains’ method in the Java String class.
Think of the ‘contains’ method as a detective – it helps you find the clues (sequence of characters) within the mystery (the string). It’s a powerful tool that can make your coding tasks a lot easier.
In this guide, we’ll walk you through the process of using the ‘contains’ method in Java String class, from the basics to more advanced techniques. We’ll cover everything from simple checks to more complex uses, as well as alternative approaches and troubleshooting common issues.
Let’s dive in and start mastering the ‘contains’ method in Java String class!
TL;DR: How Do I Use the ‘contains’ Method in Java String Class?
The ‘contains’ method in Java String class is used to check if a string contains a certain sequence of characters. You can use it like this:
boolean result = str.contains('sequence');
where ‘str’ is your string and ‘sequence’ is the sequence of characters you’re looking for.
Here’s a simple example:
String str = 'Hello, world!';
boolean result = str.contains('world');
System.out.println(result);
// Output:
// true
In this example, we have a string ‘Hello, world!’ and we’re using the ‘contains’ method to check if it contains the sequence ‘world’. The method returns ‘true’, which we print out.
This is a basic way to use the ‘contains’ method in Java String class, but there’s much more to learn about it. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding ‘contains’ in Java String
- Advantages and Pitfalls of ‘contains’
- Advanced Usage of ‘contains’ in Java String
- Exploring Alternative Approaches
- Troubleshooting Java’s ‘contains’ Method
- Delving into Java String Class
- The ‘contains’ Method in Larger Projects
- Wrapping Up: Mastering the ‘contains’ Method in Java String Class
Understanding ‘contains’ in Java String
The ‘contains’ method is a part of the Java String class. It’s used to check if a certain sequence of characters is present within a string. This method returns a boolean value – ‘true’ if the sequence is found, and ‘false’ if not.
Let’s look at a basic example of how to use the ‘contains’ method:
String str = 'Java is fun!';
boolean result = str.contains('fun');
System.out.println(result);
// Output:
// true
In this example, we’re checking if the string ‘Java is fun!’ contains the sequence ‘fun’. The ‘contains’ method returns ‘true’, indicating that the sequence is indeed present in the string.
This method is case-sensitive, meaning it distinguishes between uppercase and lowercase letters. So, ‘fun’ and ‘Fun’ would be considered different sequences.
Advantages and Pitfalls of ‘contains’
The ‘contains’ method is straightforward and easy to use, making it a handy tool for beginners. It’s especially useful when you need to quickly check if a certain sequence of characters is present in a string.
However, keep in mind that this method can’t be used directly on null strings. Attempting to do so will result in a NullPointerException. Always ensure that the string you’re checking isn’t null before using the ‘contains’ method.
Advanced Usage of ‘contains’ in Java String
The ‘contains’ method can be used for more complex tasks beyond checking for a single sequence of characters. For instance, you can use it to check if a string contains multiple different sequences. This can be achieved by chaining multiple ‘contains’ calls.
Let’s consider an example:
String str = 'Learning Java is fun and rewarding.';
boolean result1 = str.contains('Java') && str.contains('fun');
boolean result2 = str.contains('Python') && str.contains('challenging');
System.out.println(result1);
System.out.println(result2);
// Output:
// true
// false
In this code, we’re checking if the string contains both ‘Java’ and ‘fun’, and both ‘Python’ and ‘challenging’. The first check returns ‘true’ because both sequences are present in the string. The second check returns ‘false’ because the string does not contain ‘Python’ or ‘challenging’.
This method of chaining ‘contains’ calls is a powerful way to check for the presence of multiple sequences in a string. However, it’s important to remember that the ‘contains’ method is case-sensitive. This means that ‘Java’ and ‘java’ would be considered different sequences.
Exploring Alternative Approaches
While the ‘contains’ method is a powerful tool for checking if a string contains a certain sequence of characters, Java offers other methods that can achieve the same goal. Two such methods are ‘indexOf’ and regular expressions (regex).
Using ‘indexOf’ Method
The ‘indexOf’ method returns the index within the string of the first occurrence of the specified character or substring. If it doesn’t find the character or substring, it returns -1. Here’s how you can use ‘indexOf’ to check if a string contains a certain sequence:
String str = 'Java is fun!';
int index = str.indexOf('fun');
System.out.println(index != -1);
// Output:
// true
In this example, we’re checking if ‘Java is fun!’ contains ‘fun’. The ‘indexOf’ method returns the index of the first occurrence of ‘fun’, which is not -1. Hence, the printed result is ‘true’.
Using Regular Expressions
Java also supports regular expressions (regex), which can be used to check if a string contains a certain sequence. Here’s an example:
String str = 'Java is fun!';
boolean result = str.matches('.*fun.*');
System.out.println(result);
// Output:
// true
Here, we’re using the ‘matches’ method with a regular expression to check if ‘Java is fun!’ contains ‘fun’. The regular expression ‘.fun.‘ means any number of any characters, followed by ‘fun’, followed by any number of any characters. The ‘matches’ method returns ‘true’ if the string matches the regular expression.
While ‘contains’, ‘indexOf’, and regex can all be used to check if a string contains a certain sequence, the best method to use depends on your specific needs and the complexity of your task.
Troubleshooting Java’s ‘contains’ Method
While the ‘contains’ method in Java String class is straightforward, there are common issues that you might encounter when using it. Understanding these issues and how to resolve them can save you much time and frustration.
Dealing with Null Pointer Exceptions
One common issue is the NullPointerException. This occurs when you try to use the ‘contains’ method on a null string. Java does not allow methods to be called on null objects, and doing so will result in this exception.
Consider the following example:
String str = null;
boolean result = str.contains('fun');
System.out.println(result);
// Output:
// Exception in thread 'main' java.lang.NullPointerException
In this example, we’re trying to use the ‘contains’ method on a null string. This results in a NullPointerException.
To avoid this, always ensure that the string you’re checking is not null before using the ‘contains’ method. You can do this with a simple if statement:
String str = null;
if (str != null) {
boolean result = str.contains('fun');
System.out.println(result);
} else {
System.out.println('The string is null.');
}
// Output:
// The string is null.
In this revised example, we first check if the string is null. If it is not, we proceed to use the ‘contains’ method. If it is null, we print out a message indicating this. This way, we avoid the NullPointerException.
Understanding these considerations when using the ‘contains’ method in Java String class can help you write more robust and error-free code.
Delving into Java String Class
To fully grasp the ‘contains’ method and its usage, it’s crucial to understand the Java String class and its other methods. The String class in Java is a fundamental class provided by the Java library. It’s used for the creation and manipulation of strings.
A string in Java is essentially an object that represents a sequence of characters. The String class provides many methods for string manipulation, such as ‘substring’, ‘length’, ‘replace’, ‘toLowerCase’, ‘toUpperCase’, and of course, ‘contains’.
Let’s consider an example of string manipulation using some of these methods:
String str = 'Java is Fun!';
str = str.replace('Fun', 'Awesome');
str = str.toLowerCase();
System.out.println(str);
// Output:
// java is awesome
In this example, we first replace ‘Fun’ with ‘Awesome’ using the ‘replace’ method. Then, we convert the string to lowercase using the ‘toLowerCase’ method. The final result is ‘java is awesome’.
Understanding the Java String class and its methods is essential for effective string manipulation in Java. The ‘contains’ method is just one of many tools provided by this class for handling strings.
The ‘contains’ Method in Larger Projects
The ‘contains’ method in the Java String class isn’t just useful for simple tasks or beginner-level programming. It plays a significant role in larger projects, especially those involving text processing or web scraping.
For instance, in text processing or natural language processing (NLP), the ‘contains’ method can be used to identify specific patterns or phrases in large bodies of text. Similarly, in web scraping, it can be used to filter out specific information from the extracted data.
Exploring Related Concepts
If you’re interested in going beyond the ‘contains’ method, there are other related concepts in Java that you might find useful. These include string comparison (using methods like ‘equals’, ‘compareTo’, etc.), and pattern matching (using classes like Pattern and Matcher).
Further Resources for Mastering Java String
If you’re eager to delve deeper into Java String and its methods, here are some useful resources:
- Best Practices for Using Strings in Java – Learn about the Java String class’s methods for checking string length and emptiness.
Java StringUtils.isEmpty – Learn how to use isEmpty() to handle null checks and avoid NullPointerExceptions.
Comparing Strings in Java – Master comparing strings for sorting, searching, and other data processing tasks in Java.
Java String Documentation – The official Java documentation provides detailed explanations of the String class.
Java String contains() method – Programiz provides a beginner-friendly walkthrough of the
contains()
method.Java String Class by GeeksforGeeks provides explanations and examples of the String class and its methods.
Wrapping Up: Mastering the ‘contains’ Method in Java String Class
In this comprehensive guide, we’ve delved into the ‘contains’ method of the Java String class, a fundamental tool for checking if a string contains a certain sequence of characters.
We began with the basics, explaining how to use the ‘contains’ method in simple scenarios. We then progressed to more advanced uses, such as checking for multiple sequences of characters. We also explored alternative approaches, like using the ‘indexOf’ method or regular expressions.
Along the way, we addressed common issues you might encounter when using the ‘contains’ method, such as null pointer exceptions, providing solutions and workarounds for each problem.
Here’s a quick comparison of the methods we’ve discussed:
Method | Case-Sensitive | Can Check Multiple Sequences | Can Cause NullPointerException |
---|---|---|---|
‘contains’ | Yes | Yes | Yes |
‘indexOf’ | Yes | No | Yes |
Regular Expressions | Depends on the regex | Yes | Yes |
Whether you’re just starting out with Java or looking to deepen your understanding of the String class, we hope this guide has given you a comprehensive understanding of the ‘contains’ method and its uses.
The ‘contains’ method is a powerful tool in any Java developer’s toolkit, and now you’re well-equipped to use it effectively in your coding journey. Happy coding!