Comparing Strings in Java: Methods and Tips
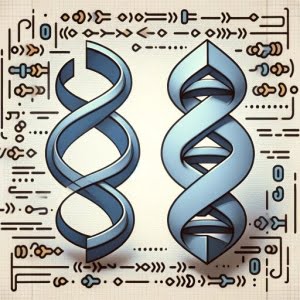
Have you ever found yourself puzzled over how to compare strings in Java? You’re not alone. Many developers find themselves in a similar situation, but Java provides a set of tools that can make this task a breeze.
Think of Java’s string comparison methods as a meticulous librarian. They help you compare and sort strings in various ways, providing a versatile and handy tool for various tasks.
This guide will walk you through the basics to advanced techniques of string comparison in Java. We’ll cover everything from the simple equals()
and compareTo()
methods to more advanced techniques and alternative approaches.
So, let’s dive in and start mastering string comparison in Java!
TL;DR: How Do I Compare Strings in Java?
In Java, you can compare strings using the
equals()
orcompareTo()
methods. These methods allow you to check if two strings are identical or determine their lexicographical order.
Here’s a simple example:
String str1 = "Hello";
String str2 = "Hello";
boolean result = str1.equals(str2);
System.out.println(result);
// Output:
// true
In this example, we have two strings str1
and str2
both containing the text “Hello”. The equals()
method is used to compare these strings, and it returns true
because both strings are identical.
This is just a basic introduction to comparing strings in Java. There’s much more to learn about string comparison, including more advanced techniques and alternative approaches. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Understanding Basic String Comparison in Java
- Dealing with Case Sensitivity in Java String Comparison
- Understanding Lexicographical Order in Java String Comparison
- Exploring Alternative Methods for String Comparison in Java
- Troubleshooting Common Issues in Java String Comparison
- Understanding Java’s String Class and Comparison Concepts
- The Bigger Picture: String Comparison and Its Relevance
- Wrapping Up: Mastering Java String Comparison
Understanding Basic String Comparison in Java
When it comes to comparing strings in Java, the two most commonly used methods are equals()
and compareTo()
. Let’s explore these methods in detail.
The equals()
Method
The equals()
method in Java is used to compare the contents of two strings. It checks whether two string objects contain the same sequence of characters, and it’s case-sensitive.
Here’s a simple example:
String str1 = "Hello";
String str2 = "hello";
boolean result = str1.equals(str2);
System.out.println(result);
// Output:
// false
In this example, str1
and str2
don’t match because the equals()
method is case-sensitive. So, even though both strings contain ‘hello’, the difference in case results in the method returning false
.
The compareTo()
Method
The compareTo()
method compares two strings lexicographically. It returns an integer that indicates whether the first string comes before (negative
), equals (zero
), or comes after (positive
) the second string.
Here’s an example of how compareTo()
works:
String str1 = "Apple";
String str2 = "Banana";
int result = str1.compareTo(str2);
System.out.println(result);
// Output:
// -1
In this example, ‘Apple’ comes before ‘Banana’ in the dictionary order, so compareTo()
returns a negative number.
One thing to keep in mind when using compareTo()
is that it’s also case-sensitive. An uppercase letter is considered to be less than a lowercase letter. If you want to compare strings without considering the case, you can use compareToIgnoreCase()
.
These methods form the foundation of string comparison in Java. But as with any tool, they have their advantages and potential pitfalls. For instance, they work perfectly for simple string comparisons, but things can get tricky with null strings or when case-insensitivity is required. In the next sections, we’ll explore some advanced techniques to handle these scenarios.
Dealing with Case Sensitivity in Java String Comparison
As you delve deeper into Java string comparison, you’ll encounter cases where you need to ignore the case while comparing strings. This is where equalsIgnoreCase()
comes in handy.
The equalsIgnoreCase()
Method
The equalsIgnoreCase()
method works similarly to the equals()
method, but it ignores the case. It checks whether two string objects contain the same sequence of characters, disregarding their case.
Here’s an example of how equalsIgnoreCase()
works:
String str1 = "Hello";
String str2 = "hello";
boolean result = str1.equalsIgnoreCase(str2);
System.out.println(result);
// Output:
// true
In this example, str1
and str2
match because equalsIgnoreCase()
ignores the case difference. Thus, the method returns true
.
Understanding Lexicographical Order in Java String Comparison
When comparing strings based on their lexicographical order, Java provides a couple of methods: compareTo()
and compareToIgnoreCase()
. We’ve already discussed compareTo()
, so let’s focus on compareToIgnoreCase()
.
The compareToIgnoreCase()
Method
The compareToIgnoreCase()
method compares two strings lexicographically, ignoring case differences. It returns an integer that indicates whether the first string comes before (negative
), equals (zero
), or comes after (positive
) the second string, disregarding the case.
Here’s an example of how compareToIgnoreCase()
works:
String str1 = "Apple";
String str2 = "banana";
int result = str1.compareToIgnoreCase(str2);
System.out.println(result);
// Output:
// -1
In this example, ‘Apple’ and ‘banana’ are compared without considering the case. ‘Apple’ comes before ‘banana’ in the dictionary order, so compareToIgnoreCase()
returns a negative number.
These methods provide more flexibility when comparing strings in Java. However, they should be used judiciously based on the requirements of your program. It’s always best to consider the implications of case sensitivity in your string comparisons and choose the appropriate method accordingly.
Exploring Alternative Methods for String Comparison in Java
While equals()
, compareTo()
, and their case-insensitive counterparts are the most common methods for string comparison in Java, there are other alternatives. These include the ==
operator, contentEquals()
, regionMatches()
, and certain third-party libraries.
Using the ==
Operator
The ==
operator compares the references of two strings, not their contents. It checks whether two string references point to the same String
object in memory.
Here’s an example:
String str1 = new String("Hello");
String str2 = new String("Hello");
boolean result = (str1 == str2);
System.out.println(result);
// Output:
// false
In this example, str1
and str2
are two different objects, so the ==
operator returns false
. Note that using ==
can lead to unexpected results if you’re intending to compare the contents of the strings.
The contentEquals()
Method
The contentEquals()
method compares the content of the String
object with the content of any CharSequence
or StringBuffer
object.
String str1 = "Hello";
StringBuffer str2 = new StringBuffer("Hello");
boolean result = str1.contentEquals(str2);
System.out.println(result);
// Output:
// true
In this example, str1
and str2
have the same content, so contentEquals()
returns true
.
The regionMatches()
Method
The regionMatches()
method compares a specific region inside a string with another specific region in another string.
String str1 = "Hello World";
String str2 = "Hello Java";
boolean result = str1.regionMatches(0, str2, 0, 5);
System.out.println(result);
// Output:
// true
In this example, the method compares the first five characters of str1
and str2
and returns true
because they match.
Third-Party Libraries
There are also third-party libraries like Apache Commons Lang and Guava that provide powerful utilities for string comparison. These libraries offer more sophisticated and flexible methods for string comparison, but they add external dependencies to your project.
Each of these methods has its own advantages and disadvantages, and their usage depends on the specific requirements of your project. Always consider the implications of each method before deciding which one to use.
Troubleshooting Common Issues in Java String Comparison
While comparing strings in Java might seem straightforward, there are several common issues that you might encounter. This section discusses some of these problems, including null pointer exceptions and case sensitivity issues, and provides solutions and workarounds.
Dealing with Null Strings
One of the most common issues when comparing strings in Java is dealing with null strings. If you try to call a method on a null string, Java will throw a NullPointerException
.
Here’s an example:
String str1 = null;
String str2 = "Hello";
boolean result = str1.equals(str2);
// Output:
// Exception in thread "main" java.lang.NullPointerException
To avoid this, you can add a null check before calling the equals()
method:
String str1 = null;
String str2 = "Hello";
boolean result = (str1 != null && str1.equals(str2));
System.out.println(result);
// Output:
// false
Addressing Case Sensitivity Issues
Another common issue is dealing with case sensitivity. As mentioned earlier, methods like equals()
and compareTo()
are case-sensitive. If you need to perform case-insensitive comparisons, you can use equalsIgnoreCase()
and compareToIgnoreCase()
.
String str1 = "Hello";
String str2 = "hello";
boolean result = str1.equalsIgnoreCase(str2);
System.out.println(result);
// Output:
// true
In this example, str1
and str2
match when using equalsIgnoreCase()
, even though they have different cases.
These are just a couple of the common issues you might encounter while comparing strings in Java. Always keep these considerations in mind to avoid unexpected results and errors in your code.
Understanding Java’s String Class and Comparison Concepts
Before diving deeper into string comparison, it’s crucial to understand the fundamentals of the Java String class and the key concepts that underlie string comparison.
The Java String Class
In Java, strings are objects that are backed internally by a final char[]
array. Since arrays are mutable in Java, you might wonder why Strings are considered immutable. The answer lies in the final
keyword, which means that once a String object is created, it cannot be changed.
Here’s a simple example:
String str = "Hello";
str = str + " World";
System.out.println(str);
// Output:
// Hello World
In this example, when we append ” World” to str
, Java doesn’t modify the original string. Instead, it creates a new String object with the new value and assigns the new object to str
.
String Literals vs. String Objects
Another important concept in Java is the difference between string literals and string objects.
String literals are defined in double quotes and are stored in a special area of memory called the string constant pool. When you create a string using double quotes, Java first checks the string constant pool. If the string already exists there, it returns the reference to the same instance. Otherwise, it creates a new string in the pool and returns its reference.
On the other hand, when you create a string using the new
keyword, Java creates a new String object in the heap memory.
Here’s an example to illustrate this:
String str1 = "Hello"; // String literal
String str2 = "Hello"; // String literal
String str3 = new String("Hello"); // String object
System.out.println(str1 == str2); // true
System.out.println(str1 == str3); // false
In this example, str1
and str2
point to the same object in the string constant pool, so str1 == str2
returns true
. However, str3
points to a different object in the heap memory, so str1 == str3
returns false
.
Understanding these concepts is crucial for effective string comparison in Java. It helps you avoid common pitfalls and write more efficient and reliable code.
The Bigger Picture: String Comparison and Its Relevance
Understanding string comparison in Java isn’t just about being able to compare two strings. It’s a fundamental concept that has wide-ranging implications in many areas of programming, including data sorting and searching algorithms.
String Comparison in Data Sorting
In data sorting, string comparison methods play a vital role. For instance, when sorting a list of names alphabetically, string comparison methods help determine the order of the names. The compareTo()
method can be particularly useful in this context, as it allows for lexicographical comparison.
String Comparison in Searching Algorithms
String comparison is also crucial in searching algorithms. When searching for a particular string in a data structure (like an array or a database), string comparison methods are used to match the search query with the data.
Exploring Related Concepts
Once you’ve mastered string comparison, there are other related concepts in Java that you might find interesting. String manipulation, for instance, involves modifying strings using various methods provided by the Java String class. Regular expressions, on the other hand, provide a powerful tool for pattern matching in strings.
Further Resources for Mastering Java String Comparison
To deepen your understanding of string comparison and related concepts in Java, here are some resources that you might find useful:
- Use Cases Explored: Strings in Java – Discover how to convert between strings and primitive data types in Java.
Java String Containment – Understand the usage of contains() for efficient substring search and validation in Java.
String Equals in Java – Learn how to compare strings for equality using the equals() method in Java.
The Official Java String Documentation provides a comprehensive overview of the String class and its methods.
Java Tutorials by Oracle cover a wide range of topics in Java, including strings and related concepts.
Java String comparisons by w3schools explains string comparisons in Java, specifically detailing the use of
equals()
andcompareTo()
.
By exploring these resources and practicing string comparison in different contexts, you’ll become a more proficient and versatile Java programmer.
Wrapping Up: Mastering Java String Comparison
In this comprehensive guide, we’ve delved into the intricacies of comparing strings in Java. We’ve explored the various methods Java provides for this essential task, from the basic equals()
and compareTo()
methods to more advanced techniques and alternative approaches.
We kicked off with the basics, learning how to use the equals()
and compareTo()
methods for simple string comparisons. We then moved onto more advanced techniques, such as dealing with case sensitivity using equalsIgnoreCase()
and compareToIgnoreCase()
, and comparing strings based on their lexicographical order.
Our journey didn’t stop there. We ventured into expert territory, exploring alternative methods for string comparison, such as the ==
operator, contentEquals()
, regionMatches()
, and even third-party libraries. Along the way, we addressed common issues you might encounter, such as null pointer exceptions and case sensitivity issues, offering solutions and workarounds for each challenge.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
equals() | Simple, reliable | Case-sensitive, can’t handle nulls |
compareTo() | Allows lexicographical comparison | Case-sensitive |
equalsIgnoreCase() | Ignores case | Can’t handle nulls |
compareToIgnoreCase() | Allows case-insensitive lexicographical comparison | – |
== operator | Compares references, not contents | Can lead to unexpected results |
contentEquals() | Compares content with any CharSequence or StringBuffer | – |
regionMatches() | Compares specific regions in strings | – |
Third-party libraries | Offer more sophisticated methods | Add external dependencies |
Whether you’re just starting out with Java string comparison or you’re looking to deepen your understanding, we hope this guide has been a valuable resource. With a solid grasp of these methods and concepts, you’re well-equipped to handle any string comparison task that comes your way. Happy coding!