Java String.equals(): Mastering String Comparison
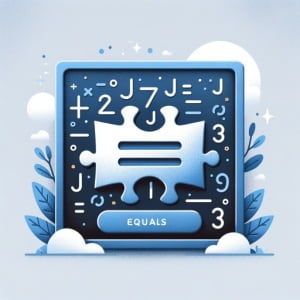
Are you finding it challenging to compare strings in Java? You’re not alone. Many developers grapple with this task, but there’s a method that can make this process a breeze.
Think of Java’s equals() method as a meticulous proofreader – it can help you compare strings accurately, ensuring that your code behaves as expected.
In this guide, we’ll walk you through the ins and outs of the equals() method in Java, from basic usage to advanced scenarios. We’ll cover everything from the fundamentals of string comparison, the equals() method’s advantages and potential pitfalls, to more complex uses and alternative approaches.
So, let’s dive in and start mastering string comparison in Java!
TL;DR: How Do I Use the equals() Method for String Comparison in Java?
In Java, you can use the equals() method to compare two strings for equality:
str1.equals(str2);
The equals() method returns a boolean value, true if the strings are identical and false if not. Here’s a simple example:
String str1 = "Hello";
String str2 = "Hello";
boolean result = str1.equals(str2);
System.out.println(result);
// Output:
// true
In this example, we have two strings, str1
and str2
, both containing the text “Hello”. We use the equals() method to compare these two strings, and the result is true, indicating that the strings are identical.
This is just a basic usage of the equals() method in Java. But there’s much more to learn about string comparison in Java, including more complex uses and alternative approaches. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Understanding the Basics of the equals() Method
- Advanced String Comparison with equals()
- Exploring Alternatives to equals()
- Troubleshooting Common Issues with equals()
- String Comparison in Java: The Fundamentals
- The Bigger Picture: String Comparison in Broader Java Programming
- Wrapping Up: Mastering String Comparison in Java with equals()
Understanding the Basics of the equals() Method
The equals() method in Java is a built-in function used to compare two strings. It checks whether two strings are equal character-by-character and returns a boolean value – true if they are equal and false if not.
Let’s take a look at a simple example:
String str1 = "Hello, World!";
String str2 = "Hello, World!";
boolean result = str1.equals(str2);
System.out.println(result);
// Output:
// true
In this example, we have two strings, str1
and str2
, both containing the text “Hello, World!”. The equals() method is used to compare these two strings, and the result is true, meaning the strings are identical.
Advantages of Using equals()
The equals() method is case-sensitive, which means it considers the case while comparing strings. This can be advantageous when you want to compare two strings and case matters.
Potential Pitfalls
While the equals() method is a powerful tool for string comparison in Java, it’s important to note that it can throw a NullPointerException if you try to use it on a null reference.
String str1 = null;
String str2 = "Hello, World!";
try {
boolean result = str1.equals(str2);
System.out.println(result);
} catch (NullPointerException e) {
System.out.println("Caught a NullPointerException.");
}
// Output:
// Caught a NullPointerException.
In the above example, we tried to use the equals() method on str1
, which is null. This resulted in a NullPointerException. Therefore, it’s always a good practice to check for null before using the equals() method.
Advanced String Comparison with equals()
As we dive deeper into the equals() method, let’s explore some more complex scenarios. One such scenario is comparing strings of different cases.
The equals() method in Java is case-sensitive. This means that it considers the case (lowercase or uppercase) of characters while comparing strings. Let’s take a look at an example:
String str1 = "Hello, World!";
String str2 = "HELLO, WORLD!";
boolean result = str1.equals(str2);
System.out.println(result);
// Output:
// false
In this case, even though str1
and str2
contain the same characters, the equals() method returns false because the case of the characters is different.
The Case-Sensitivity of equals()
The case-sensitivity of the equals() method can be both a pro and a con, depending on your needs.
Pro: When case matters in your comparison, the equals() method is a perfect choice. It ensures that two strings are identical, not just in characters, but also in their case.
Con: On the other hand, if you want to compare two strings without considering their case, the equals() method might not be the best choice. In such a scenario, you might want to use the equalsIgnoreCase() method instead, which ignores the case while comparing strings.
String str1 = "Hello, World!";
String str2 = "HELLO, WORLD!";
boolean result = str1.equalsIgnoreCase(str2);
System.out.println(result);
// Output:
// true
In the code above, using equalsIgnoreCase() returns true, indicating that str1
and str2
are equal when case is not considered.
Exploring Alternatives to equals()
While the equals() method is a robust tool for string comparison in Java, it’s not the only one. Depending on your needs, other methods like the ==
operator, the compareTo() method, and the equalsIgnoreCase() method might be more suitable.
The ==
Operator
The ==
operator checks if two string references point to the same object, rather than comparing the actual characters in the strings.
String str1 = "Hello, World!";
String str2 = str1;
boolean result = (str1 == str2);
System.out.println(result);
// Output:
// true
In this example, str1
and str2
point to the same object, so the ==
operator returns true. However, if str2
were assigned the value “Hello, World!” directly, the ==
operator would return false, even though the characters in the strings are identical.
The compareTo() Method
The compareTo() method not only tells you whether two strings are equal, but also how they compare lexicographically. If the strings are equal, it returns 0. If the first string comes before the second lexicographically, it returns a negative value, and if it comes after, a positive value.
String str1 = "Hello";
String str2 = "World";
int result = str1.compareTo(str2);
System.out.println(result);
// Output:
// -15
In this case, str1
comes before str2
lexicographically, so the compareTo() method returns a negative value.
The equalsIgnoreCase() Method
As we’ve already seen, the equalsIgnoreCase() method compares two strings, ignoring their case. This can be useful when you want to check if two strings are equal regardless of their case.
String str1 = "Hello, World!";
String str2 = "HELLO, WORLD!";
boolean result = str1.equalsIgnoreCase(str2);
System.out.println(result);
// Output:
// true
In this example, equalsIgnoreCase() returns true, indicating that str1
and str2
are equal when case is not considered.
Each of these methods has its own benefits and drawbacks. The key is to understand your needs and choose the most appropriate tool for your specific scenario.
Troubleshooting Common Issues with equals()
While the equals() method is generally straightforward, it can sometimes lead to unexpected results. Let’s troubleshoot some common issues and discuss their solutions.
Comparing with Null Values
One common pitfall is trying to use equals() on a null reference, which results in a NullPointerException. For example:
String str1 = null;
String str2 = "Hello";
try {
boolean result = str1.equals(str2);
System.out.println(result);
} catch (NullPointerException e) {
System.out.println("Caught a NullPointerException.");
}
// Output:
// Caught a NullPointerException.
In this example, str1
is null. When we try to use equals() on str1
to compare it with str2
, it throws a NullPointerException.
To avoid this, always check if the string is null before using equals().
String str1 = null;
String str2 = "Hello";
if (str1 != null && str1.equals(str2)) {
System.out.println("Strings are equal.");
} else {
System.out.println("Strings are not equal.");
}
// Output:
// Strings are not equal.
In this revised code, we first check if str1
is not null before calling equals(). This prevents the NullPointerException.
Best Practices and Optimization
When using equals(), remember that it’s case-sensitive. If you want to compare strings without considering case, use equalsIgnoreCase() instead.
Also, be aware of the difference between equals() and the ==
operator. While equals() checks if two strings are identical character-by-character, ==
checks if two string references point to the same object. Choose the one that suits your needs.
By understanding these common issues and best practices, you can use the equals() method more effectively and avoid common pitfalls.
String Comparison in Java: The Fundamentals
To fully understand the equals() method, it’s important to delve into the fundamentals of how string comparison works in Java.
In Java, strings are objects, not primitive types. This means that when you create a string, you’re actually creating an object instance of the String class.
String Equality vs. Identity
In Java, string equality and identity are two different concepts.
String Equality: Two strings are considered equal if they contain the same characters in the same order. This is what the equals() method checks for.
String str1 = new String("Hello");
String str2 = new String("Hello");
boolean result = str1.equals(str2);
System.out.println(result);
// Output:
// true
In this example, str1
and str2
are different objects, but they contain the same characters, so equals() returns true.
String Identity: Two strings are identical if they refer to the same object. This is what the ==
operator checks for.
String str1 = new String("Hello");
String str2 = str1;
boolean result = (str1 == str2);
System.out.println(result);
// Output:
// true
In this case, str1
and str2
refer to the same object, so the ==
operator returns true.
Understanding these fundamental concepts can help you use the equals() method more effectively and avoid common pitfalls.
The Bigger Picture: String Comparison in Broader Java Programming
The importance of string comparison extends far beyond the equals() method. It plays a crucial role in several areas of Java programming, such as data structures and algorithms.
String Comparison in Data Structures
In data structures like arrays, linked lists, and trees, string comparison is often used to sort, search, or categorize data. Understanding how to effectively compare strings can help you manipulate these data structures more efficiently.
String Comparison in Algorithms
Many algorithms, especially those dealing with text processing, rely heavily on string comparison. For instance, algorithms for text search, pattern matching, and text compression all use string comparison in one form or another.
Exploring Related Concepts
If you’re interested in delving deeper into string manipulation in Java, you might want to explore concepts like string concatenation, substring extraction, and regular expressions. These tools can provide more flexibility and power in handling strings.
Further Resources for Mastering String Comparison in Java
To further your understanding of string comparison in Java, here are some resources you might find helpful:
- Tips and Techniques for Using Strings in Java – Explore the Java String class’s methods for searching and matching patterns.
String Compare in Java – Explore various methods for comparing strings in Java to determine their relative order.
Java String Length – Learn about the length() method and its usage for obtaining the number of characters in a string.
Java String Documentation provides a comprehensive overview of the String class, including the equals() method.
Java Tutorials by Oracle provide a deep dive into strings in Java, including string comparison.
GeeksforGeeks’ Java String equals() article provides a detailed explanation of the various methods to compare strings.
Mastering string comparison in Java can open up new possibilities in your coding projects. So keep exploring, keep learning, and keep coding!
Wrapping Up: Mastering String Comparison in Java with equals()
In this comprehensive guide, we’ve journeyed through the intricacies of the equals() method in Java, a crucial tool for comparing strings. We’ve seen how it can be used in a variety of scenarios, from basic comparisons to more complex cases, and we’ve discussed how to handle common issues such as null values.
We began with an introduction to the equals() method, explaining its function and how to use it in basic scenarios. We then delved into more advanced uses, highlighting how the equals() method handles case sensitivity. We also discussed alternative approaches to string comparison, such as the ==
operator, the compareTo() method, and the equalsIgnoreCase() method.
Along the way, we provided solutions to common issues you might encounter when using equals(), such as NullPointerExceptions when comparing with null values. We also offered tips for best practices and optimization to help you use the equals() method more effectively.
Here’s a quick comparison of the methods we’ve discussed:
Method | Case-Sensitivity | Null-Safety | Comparing References |
---|---|---|---|
equals() | Yes | No | No |
== operator | N/A | Yes | Yes |
compareTo() | Yes | No | No |
equalsIgnoreCase() | No | No | No |
Whether you’re just starting out with Java or you’re looking to level up your string comparison skills, we hope this guide has given you a deeper understanding of the equals() method and its alternatives.
Mastering string comparison is a vital part of Java programming. With the tools and techniques we’ve discussed, you’re well-equipped to handle any string comparison scenario that comes your way. Happy coding!