Java Format Method Explained: From Basics to Advanced
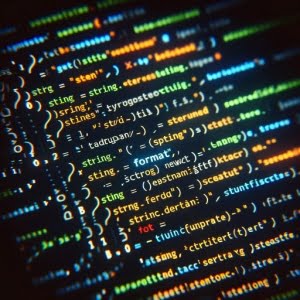
Ever found yourself grappling with string formatting in Java? You’re not alone. Many developers find themselves in a similar predicament. Think of Java’s string formatting as a craftsman’s tool – a powerful utility that allows you to shape your strings precisely the way you want.
This guide will walk you through the key methods for formatting strings in Java, from basic to advanced techniques. We’ll cover everything from the String.format()
method, dealing with different data types, to more complex formatting scenarios and alternative approaches.
So, let’s dive in and start mastering string formatting in Java!
TL;DR: How Do I use the .format() Method in Java?
The
String.format()
method in java is part of the String class, used with the sytnaxString formattedString = String.format()
. This method allows you to create a formatted string using placeholders and arguments.
Here’s a simple example:
String formattedString = String.format("Hello, %s!", "World");
System.out.println(formattedString);
// Output:
// 'Hello, World!'
In this example, we’ve used the String.format()
method to format a string. The %s
inside the string is a placeholder where the variable gets inserted. In this case, ‘World’ is inserted in place of %s
, resulting in the output ‘Hello, World!’.
But Java’s string formatting capabilities go far beyond this. Continue reading for more detailed examples and advanced formatting techniques.
Table of Contents
String Formatting in Java: The Basics
In Java, the String.format()
method is one of the most commonly used tools for string formatting. This method works by taking in a format string and an arbitrary number of arguments. The format string includes placeholders, denoted by %
, followed by a format specifier.
The format specifiers define the data type of the argument. For instance, %s
is used for strings, %d
for decimal integers, and %f
for floating-point numbers.
Let’s take a look at an example:
String name = "John";
int age = 30;
String formattedString = String.format("My name is %s and I am %d years old.", name, age);
System.out.println(formattedString);
// Output:
// 'My name is John and I am 30 years old.'
In the above example, %s
is replaced by the string variable name
and %d
is replaced by the integer variable age
. The String.format()
method replaces the placeholders with the provided arguments in the order they are given.
This method is incredibly versatile and can handle different data types with ease. However, one potential pitfall to be aware of is the IllegalFormatException
. This occurs when the format specifier doesn’t match the argument’s data type. For instance, if you were to use %d
with a string argument, Java would throw an IllegalFormatException
.
Advanced String Formatting: Delving Deeper
As you get more comfortable with Java’s String.format()
method, you’ll find that it can handle more complex formatting scenarios. This includes using different format specifiers and flags to give you more control over your output.
Format Specifiers and Flags
Format specifiers are not limited to just %s
, %d
, and %f
. There are many more, each with a specific purpose. For example, %b
for boolean, %c
for character, and %e
for scientific notation.
Flags are used to control the output format. For instance, you can use the -
flag to left-justify the output, or the 0
flag to pad numbers with leading zeros.
Let’s see an example of these advanced techniques:
int number = 15;
String formattedString = String.format("Binary: %s, Octal: %o, Hexadecimal: %x", Integer.toBinaryString(number), number, number);
System.out.println(formattedString);
// Output:
// 'Binary: 1111, Octal: 17, Hexadecimal: f'
In this example, we’ve used the %s
, %o
, and %x
format specifiers to convert a decimal number into its binary, octal, and hexadecimal representations. The Integer.toBinaryString(number)
method is used to convert the integer into a binary string.
While the String.format()
method provides a great deal of flexibility and control, it can become complex and hard to read with many format specifiers and flags. It’s essential to keep your format strings as simple and clear as possible for the sake of readability and maintainability.
Alternative Formatting Techniques: DecimalFormat
and MessageFormat
While String.format()
is a versatile tool, Java doesn’t limit you to just one way of formatting strings. There are other classes in Java that provide additional methods for string formatting, such as DecimalFormat
and MessageFormat
.
The DecimalFormat
Class
The DecimalFormat
class is a part of java.text
package and is primarily used to format decimal numbers. It allows you to control the precision of your output and can also handle locale-specific formats.
Here’s an example of DecimalFormat
in action:
import java.text.DecimalFormat;
DecimalFormat df = new DecimalFormat("#.##");
String formattedString = df.format(123.456);
System.out.println(formattedString);
// Output:
// '123.46'
In this example, we’ve used DecimalFormat
to control the precision of a floating-point number. The #.##
pattern means we want up to two decimal places. The format()
method then rounds the number to the closest two decimal places.
The MessageFormat
Class
The MessageFormat
class, also a part of java.text
package, provides a means to produce concatenated messages in a language-neutral way. It formats messages by replacing placeholders with the arguments given. It’s similar to String.format()
, but it’s more powerful when dealing with complex, parameterized messages.
Here’s an example of MessageFormat
:
import java.text.MessageFormat;
String name = "John";
int age = 30;
String formattedString = MessageFormat.format("My name is {0} and I am {1} years old.", name, age);
System.out.println(formattedString);
// Output:
// 'My name is John and I am 30 years old.'
In this example, we’ve used MessageFormat
to format a string. The {0}
and {1}
inside the string are placeholders where the variables get inserted. The format()
method replaces the placeholders with the provided arguments in the order they are given.
Both DecimalFormat
and MessageFormat
provide unique advantages over String.format()
. DecimalFormat
is excellent for controlling the precision of decimal numbers, and MessageFormat
is powerful when dealing with complex, parameterized messages. However, they can be overkill for simple formatting tasks where String.format()
would suffice.
Troubleshooting Java String Formatting
As with any programming task, you might encounter some issues while formatting strings in Java. Two of the most common issues are IllegalFormatException
and problems with locale settings.
Handling IllegalFormatException
The IllegalFormatException
is often thrown when the format specifier doesn’t match the argument’s data type. For example, using %d
with a string argument would result in this exception.
Here’s an example that triggers IllegalFormatException
:
try {
String formattedString = String.format("%d", "Hello");
} catch (IllegalFormatException e) {
e.printStackTrace();
}
// Output:
// java.util.IllegalFormatException: d != java.lang.String
In this example, we attempted to format a string as a decimal integer, causing IllegalFormatException
. To avoid this, always ensure your format specifiers match your argument data types.
Dealing with Locale Settings
Another common issue is dealing with locale settings. The way numbers and dates are formatted can vary significantly from one locale to another. If your application needs to support multiple locales, you should use the Locale
class in conjunction with the String.format()
method.
Here’s an example of formatting a number in US and French locales:
import java.util.Locale;
double number = 1234567.89;
String us = String.format(Locale.US, "%,.2f", number);
String france = String.format(Locale.FRANCE, "%,.2f", number);
System.out.println("US: " + us);
System.out.println("France: " + france);
// Output:
// US: 1,234,567.89
// France: 1 234 567,89
In this example, we formatted the same number in two different locales. Notice how the US locale uses commas as thousand separators and a dot as a decimal separator, while the French locale uses spaces as thousand separators and a comma as a decimal separator.
Understanding these common issues and how to handle them will help you format strings effectively in Java.
Understanding Java’s String Class
The String
class in Java is one of the most fundamental classes and is used extensively in almost any Java application. This class provides a wide array of methods to manipulate and work with strings.
One of the powerful features of the String
class is its ability to format strings. This is achieved through the String.format()
method. This method uses format specifiers, flags, and other special characters to format strings in a variety of ways.
Format Specifiers and Flags Explained
Format specifiers are special characters that specify the type of data the string should be formatted to. Here are some common format specifiers:
%s
for strings%d
for integers%f
for floating-point numbers
Flags are special characters that control the output’s formatting. Some common flags are:
-
for left justification0
for padding with zeroes
Here’s an example that uses format specifiers and flags:
int number = 123;
String formattedString = String.format("%05d", number);
System.out.println(formattedString);
// Output:
// 00123
In this example, the %05d
format specifier is used to format the integer. The 0
is a flag that pads the number with leading zeroes, and the 5
specifies the minimum width of the output. So, the number 123
is formatted as 00123
.
Understanding the concept of string formatting, format specifiers, and flags is crucial to mastering Java’s String.format()
method. These concepts allow you to control how your strings are formatted and displayed, making your Java applications more versatile and user-friendly.
The Power of Java String Formatting
Understanding and mastering string formatting in Java is not just about making your code work. It’s about making your applications more user-friendly, your logs more readable, and your data more digestible.
String Formatting in Action
Imagine you’re creating an application that displays user data. Without proper string formatting, dates might be displayed in a hard-to-read format, numbers might not have the right number of decimal places, and text might be jumbled together without proper spacing. By using string formatting, you can control exactly how your data is displayed, making it easier for users to understand and interact with your application.
Similarly, when logging, string formatting can help you create more readable and informative log messages. This can be crucial when debugging your application or analyzing its performance.
Beyond String Formatting: String Manipulation and Regular Expressions
Once you’ve mastered string formatting, there are other related concepts to explore. String manipulation involves changing, splitting, concatenating, and otherwise altering strings. Regular expressions, on the other hand, allow you to match patterns in strings, which can be incredibly powerful for tasks like data validation or searching.
Further Resources for Mastering Java String Formatting
To deepen your understanding of Java string formatting and related topics you can Click Here for our complete guide on navigating the Strings class.
Furthermore, here are some additional resources you might find useful:
- StringUtils.isEmpty in Java – Explore the StringUtils.isEmpty() method in Java for checking if a string is null or empty.
Java Escape Sequences – Learn about common escape sequences like
\n
,\t
,"
, and\
and their meanings.Java Documentation: Formatter – The official Java documentation provides a detailed explanation of the Formatter class.
Guide to Java String Formatting – This guide covers string formatting in more detail, with plenty of examples.
Oracle’s Java Tutorials: Regular Expressions – This tutorial by Oracle is a great resource for learning about regular expressions.
Remember, becoming proficient in Java string formatting is a process. Don’t rush it. Take your time to understand the concepts, experiment with different formatting options, and most importantly, have fun coding!
Wrapping Up: Mastering Java String Formatting
In this comprehensive guide, we’ve journeyed through the world of string formatting in Java, from understanding the basics to exploring advanced techniques. We’ve delved deep into the String.format()
method, discussed its usage with different data types, and highlighted its potential pitfalls.
We began with the basics, learning how to use String.format()
and its format specifiers for different data types. We then ventured into more advanced territory, exploring complex formatting scenarios involving different format specifiers and flags. Along the way, we tackled common issues you might face when using String.format()
, such as IllegalFormatException
and issues with locale settings, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to string formatting in Java, exploring the DecimalFormat
and MessageFormat
classes. Each of these methods provides unique advantages and can be more suitable for certain scenarios.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
String.format() | Versatile, easy to use | Can become complex with many format specifiers |
DecimalFormat | Great for controlling decimal precision | Overkill for simple formatting tasks |
MessageFormat | Powerful for complex, parameterized messages | More complex than String.format() |
Whether you’re just starting out with Java string formatting or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of string formatting in Java and its capabilities. With its balance of versatility, ease of use, and power, string formatting is a crucial tool in any Java developer’s toolkit. Happy coding!