Python Type Hints: A Comprehensive Usage Guide
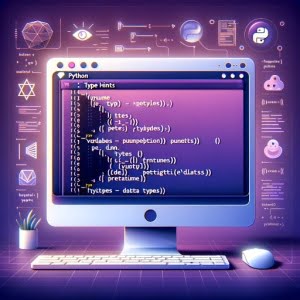
Confused about Python type hints? If you’ve ever found yourself lost in a maze of Python code, you’re not alone. Python type hints are like a roadmap, guiding you and others through the labyrinth of your code, helping to understand what type of data your functions expect and return.
In this article, we’ll provide a step-by-step guide on how to use type hints in Python, taking you from the basics to advanced techniques. Whether you’re a beginner just starting out or an experienced developer looking to refine your skills, this comprehensive guide will serve as your trusty companion on the journey to mastering Python type hints.
TL;DR: How Do I Use Type Hints in Python?
To use type hints in Python, you annotate your function arguments and return values with the expected types. Here’s a simple example:
def greet(name: str) -> str:
return 'Hello, ' + name
# Output:
# Hello, Anton!
In this example, the function greet
expects a string as an argument (name: str
) and returns a string (-> str
). The type hint str
indicates that the function expects a string input and also returns a string.
Dive further into this article for a more detailed exploration of Python type hints, including advanced usage scenarios.
Table of Contents
Basic Use of Python Type Hints
Python type hints are a handy feature that allows you to annotate your function arguments and return values with the expected types. This practice not only makes your code more readable but also reduces the likelihood of runtime errors. Let’s look at how to implement type hints in Python.
Annotating Function Arguments and Return Values
In Python, you can use the colon (:
) to annotate the type of a function’s arguments, and the arrow (->
) to annotate the type of the value it returns. Consider the following example:
def add_numbers(num1: int, num2: int) -> int:
return num1 + num2
print(add_numbers(3, 5))
# Output:
# 8
In this code, the function add_numbers
takes two arguments, num1
and num2
, both of which are expected to be of type int
. The function returns an int
as well, which is the sum of num1
and num2
. When we call the function with the arguments 3 and 5, it returns 8, as expected.
This basic use of Python type hints can greatly improve the readability of your code and make it easier for others (and your future self) to understand.
Advanced Use of Python Type Hints
As you become more proficient with Python type hints, you’ll discover that they offer a wealth of advanced features that can further enhance your code’s readability and robustness. Let’s delve into some of these advanced techniques.
Using Generics in Type Hints
Generics are a powerful feature that allows you to specify type parameters for classes and functions, thereby creating reusable code components. Here’s an example:
from typing import List, Tuple
def process_data(data: List[Tuple[int, str]]) -> None:
for item in data:
print(f'Processing item {item[0]}: {item[1]}')
process_data([(1, 'apple'), (2, 'banana')])
# Output:
# Processing item 1: apple
# Processing item 2: banana
In this example, the function process_data
expects a list of tuples as input, where each tuple contains an integer and a string. The function prints out each item in the list.
Utilizing Optional Types and Type Aliases
Optional types and type aliases can make your type hints more flexible and readable. Here’s how you can use them:
from typing import Optional, List, Tuple, TypeVar
T = TypeVar('T', int, float, complex)
Vector = List[Tuple[T, T]]
def scale(scalar: T, vector: Optional[Vector[T]] = None) -> Vector[T]:
if vector:
return [(scalar * x, scalar * y) for x, y in vector]
else:
return []
print(scale(2, [(1, 2), (3, 4)]))
# Output:
# [(2, 4), (6, 8)]
In this code, T
is a type variable that can be an int
, float
, or complex
number. Vector
is a type alias for a list of tuples, where each tuple contains two elements of type T
. The function scale
multiplies each element of the vector by a scalar, and it can handle vectors of None
type.
By mastering these advanced techniques, you can leverage Python type hints to make your code more robust and maintainable.
Alternative Approaches to Python Type Hints
Python type hints are versatile and can be applied in several ways, depending on your specific needs and the Python version you’re using. Let’s explore some of these alternative approaches.
Variable Annotations in Python
Starting from Python 3.6, you can use variable annotations to provide hints about the expected type of a variable. This is particularly useful when you’re initializing variables that don’t have a value yet. Here’s an example:
from typing import List
names: List[str] = []
In this code, the variable names
is expected to be a list of strings. Note that the variable is initially empty, but the type hint gives a clear indication of what type of data it will contain.
Type Hints in Comments for Older Python Versions
If you’re working with a Python version that doesn’t support variable annotations (i.e., Python 3.5 or older), you can still use type hints by including them in comments. This method, known as type hinting in comments, is a bit more verbose but still effective. Here’s how you can do it:
names = [] # type: List[str]
In this code, the type hint is included in a comment immediately after the variable declaration. This tells us that names
is expected to be a list of strings, just like in the previous example.
By understanding these alternative approaches, you can apply Python type hints effectively, regardless of the Python version you’re using or the specific requirements of your code.
Troubleshooting Common Issues with Python Type Hints
While Python type hints are a powerful tool, like any other feature, they can sometimes lead to unexpected issues. Let’s discuss some common challenges you might encounter when using type hints and how to resolve them.
Dealing with Missing Type Hints
One common issue is forgetting to include a type hint. This can lead to confusion and potential bugs. Python won’t raise an error if a type hint is missing, but your code might not work as expected. Always double-check your functions to make sure all arguments and return values have appropriate type hints.
Handling Incompatible Types
Another common issue arises when the actual type of a variable doesn’t match its type hint. For instance, consider the following code:
def greet(name: str) -> str:
return 'Hello, ' + name
greet(123)
# Output:
# TypeError: can only concatenate str (not "int") to str
In this example, we’re trying to use an integer as the name
argument, but the function expects a string. This results in a TypeError
. To avoid this issue, always ensure that the data you’re passing to a function matches the types specified in the function’s type hints.
Type Hints and Performance Considerations
Keep in mind that while type hints can improve code readability and catch some errors early, they don’t improve the performance of your Python code. Python is a dynamically typed language, and the type hints are just that – hints. They don’t change the way your code is executed.
By being aware of these common issues and their solutions, you can use Python type hints more effectively and avoid potential pitfalls.
Understanding Python Type Hints
Before we delve deeper into Python type hints, it’s important to understand the concept and the benefits it brings to your code.
The Concept of Type Hints
In Python, type hints are a form of documentation that tell you what type of data a function expects to receive and return. They are optional and do not affect how the code is run. However, they can significantly improve code readability and help catch certain types of errors before the code is even run.
Consider the following example:
def greet(name: str) -> str:
return 'Hello, ' + name
greet('World')
# Output:
# Hello, World!
In this code, the function greet
expects a string as an argument (name: str
) and returns a string (-> str
). The type hint str
indicates that the function expects a string input and also returns a string.
Static Typing vs Dynamic Typing
Python is a dynamically typed language, which means that the type of a variable is checked during runtime. This offers a lot of flexibility, but it can also lead to errors that are hard to debug.
On the other hand, statically typed languages like Java or C++ require you to declare the type of a variable when you create it, and the type is checked at compile time. This can catch certain types of errors early but can also make the code more verbose.
Python type hints bring a bit of static typing into the dynamic world of Python. They don’t change how the code is run, but they can make it easier to understand and debug.
Exploring Beyond: Python Type Hints in Larger Projects
As your Python projects grow in size and complexity, the importance of Python type hints becomes more pronounced. They not only improve readability but also make it easier to debug and maintain your code.
Type Hints and Programming Paradigms
In certain programming paradigms, such as Object-Oriented Programming (OOP), type hints can be especially useful. They can help you understand the structure of your code and the relationships between different objects.
Consider the following example:
from typing import List
class Student:
def __init__(self, name: str, grades: List[int]) -> None:
self.name = name
self.grades = grades
def average_grade(self) -> float:
return sum(self.grades) / len(self.grades)
student = Student('John', [85, 90, 88])
print(student.average_grade())
# Output:
# 87.66666666666667
In this code, we define a Student
class with a name
and a list of grades
. The average_grade
method calculates the average grade of the student. The type hints make it clear what type of data each method expects and returns, making the code easier to understand and debug.
Diving Deeper: Static Type Checking and Gradual Typing
If you find Python type hints helpful, you might want to explore related concepts like static type checking and gradual typing. Static type checking tools like Mypy can check your type hints and catch type errors before your code is run. Gradual typing, on the other hand, is a programming language feature that allows you to gradually add type information to your code, making it more robust and maintainable over time.
Recap: Python Type Hints Unveiled
In this comprehensive guide, we’ve unveiled the power of Python type hints, a feature that can significantly enhance the readability and maintainability of your Python code.
We’ve journeyed from the basics of annotating function arguments and return values, through advanced techniques like using generics, optional types, and type aliases, to alternative approaches for different Python versions and specific requirements.
We’ve also discussed common issues you might encounter when using type hints, such as missing type hints and incompatible types, and shared solutions to these problems. Remember, while type hints can improve code readability and help catch certain types of errors early, they don’t improve the performance of your Python code.
Whether you’re a beginner just starting out or an experienced developer looking to refine your skills, Python type hints can be a powerful tool in your coding arsenal. By understanding and effectively using type hints, you can write cleaner, more robust code that’s easier to understand and debug.
As you continue your Python journey, keep this guide handy for reference and dive deeper into related concepts like static type checking and gradual typing.