Python Lists Unleashed: A Comprehensive Guide
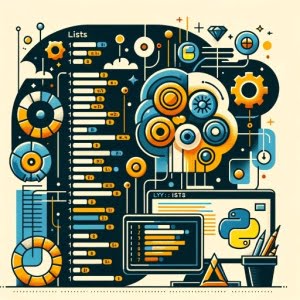
When managing data on Python scripts at IOFLOOD, we frequently use lists to store and manipulate collections. Lists offer significant advantages, such as dynamic resizing and easy indexing, which make them ideal for various programming scenarios. Today’s article tackles what a list in Python is and how to use it effectively, providing our customers with practical coding practices to use on their dedicated cloud servers.
This guide will walk you through everything you need to know about Python lists, from creation to advanced manipulations, providing you with the knowledge you need to effectively manage and manipulate your data.
So without further ado, let’s dive in to the list data structure in Python!
TL;DR: What is a list in Python and how do I use it?
A
list
in Python is a mutable, ordered collection of items and uses the[]
syntax,new_list = ['sample', 'values']
. It’s a versatile tool that allows you to store multiple items in a single variable. Lists are one of four built-in data types in Python used to store collections of data. The other three areTuple
,Set
, andDictionary
, all with different qualities and usage.
Here’s a simple example of creating and using a list:
my_list = [1, 2, 3, 4]
print(my_list[0]) # prints 1
In this example, we created a list named ‘my_list’ and filled it with the numbers 1, 2, 3, and 4. We then printed the first item in the list (Python lists are zero-indexed, so the first item is accessed with 0).
Keep reading to learn more about Python lists and their various operations. We’ll dive into how to create, modify, and manipulate lists in Python, as well as some common pitfalls to avoid.
Table of Contents
The Basics: Delving into Python Lists
Let’s start with the most fundamental aspect of Python lists: how to create them. Creating a list in Python is straightforward. All you need is to enclose your elements in square brackets []
.
Here’s a simple example:
fruits = ['apple', 'banana', 'cherry']
print(fruits)
# Output:
# ['apple', 'banana', 'cherry']
In this example, we created a list named ‘fruits’ and filled it with the strings ‘apple’, ‘banana’, and ‘cherry’.
Adding Elements to a List
To add an element to a list, you can use the append()
method. This method adds an item to the end of the list.
fruits.append('dragonfruit')
print(fruits)
# Output:
# ['apple', 'banana', 'cherry', 'dragonfruit']
As you can see, ‘dragonfruit’ was added to the end of our ‘fruits’ list.
Removing Elements from a List
Python provides several methods to remove elements from a list. The remove()
method removes the specified item.
fruits.remove('banana')
print(fruits)
# Output:
# ['apple', 'cherry', 'dragonfruit']
In the example above, ‘banana’ was removed from the ‘fruits’ list.
Accessing Elements in a List
Accessing elements in a Python list is done by referring to the index number. Remember, Python uses zero-based indexing, so the first element is at index 0.
print(fruits[0])
# Output:
# 'apple'
In the example above, we accessed the first element of our ‘fruits’ list, which is ‘apple’.
Understanding these basic operations is the first step to mastering Python lists. However, it’s important to note that Python lists are mutable, meaning they can be changed. This is a powerful feature, but it also means you need to be careful when modifying lists to avoid unexpected behavior.
Advanced Operations with Python Lists
Now that we’ve covered the basics, let’s move onto more complex Python list operations. These operations can help you manipulate your lists in more sophisticated ways, allowing you to handle more complex data structures and solve more complicated problems.
List Slicing
List slicing is a powerful feature in Python that allows you to access subsets of your list. It’s done by using a colon :
to separate the start, end, and step of the slice.
numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
slice = numbers[2:6]
print(slice)
# Output:
# [2, 3, 4, 5]
In this example, we created a slice of the ‘numbers’ list from index 2 to 5 (end index is exclusive). The result is a new list containing the numbers 2, 3, 4, and 5.
List Comprehensions
List comprehensions are a unique feature of Python that allow you to create a new list from an existing one in a single, readable line of code. They can include conditionals and even nested loops.
squares = [number**2 for number in numbers if number % 2 == 0]
print(squares)
# Output:
# [0, 4, 16, 36, 64]
In the example above, we used a list comprehension to create a new list ‘squares’, which contains the squares of the even numbers in the ‘numbers’ list.
Lambda Functions
Lambda functions, or anonymous functions, are small, single-expression functions that can be used inline in list comprehensions or map()
and filter()
functions.
double = list(map(lambda x: x * 2, numbers))
print(double)
# Output:
# [0, 2, 4, 6, 8, 10, 12, 14, 16, 18]
In the example above, we used a lambda function to double each number in the ‘numbers’ list. The map()
function applies the lambda function to each element in the ‘numbers’ list.
These advanced operations can significantly boost your Python list manipulations. However, they also introduce new potential pitfalls, such as off-by-one errors in list slicing or unexpected behavior from lambda functions. Always test your code thoroughly and understand the tools you’re using.
Alternate Data Collections in Python
While Python lists are incredibly versatile and useful, they’re not always the best tool for the job. Other data structures, like tuples and sets, can sometimes be more appropriate depending on your specific needs.
The Power of Tuples
A tuple is a collection of Python objects much like a list. The sequence of values stored in a tuple can be of any type, and they are indexed by integers. The important difference is that tuples are immutable. Tuples are also faster than lists as they consume less memory.
Here’s how you can create a tuple:
colors = ('red', 'green', 'blue')
print(colors)
# Output:
# ('red', 'green', 'blue')
In this example, we created a tuple named ‘colors’. Since tuples are immutable, they can’t be changed after they’re created. This makes them ideal for storing data that shouldn’t be changed.
Sets for Uniqueness
A set, in Python, is an unordered collection of items. Every element is unique (no duplicates) and must be immutable (cannot be changed). However, a set itself is mutable. We can add or remove items from it.
Here’s how you can create a set:
unique_numbers = set([1, 2, 2, 3, 4, 4, 5])
print(unique_numbers)
# Output:
# {1, 2, 3, 4, 5}
In this example, we created a set named ‘unique_numbers’. Sets are particularly useful when you want to eliminate duplicate values, as they automatically only store unique elements.
Choosing the right data structure can greatly affect the readability, performance, and ease of writing your code. While lists are a great default choice, don’t forget about the other tools in your Python toolbox.
Solutions for Issues with Python Lists
While Python lists are powerful and flexible, they can sometimes lead to unexpected errors, especially for beginners. Let’s walk through some of the most common issues you might encounter when working with Python lists, and how to avoid them.
Index Errors
One of the most common errors you might encounter when working with Python lists is an IndexError
. This error is raised when you try to access an index that doesn’t exist in your list.
fruits = ['apple', 'banana', 'cherry']
print(fruits[3])
# Output:
# IndexError: list index out of range
In the example above, we tried to access the fourth element (index 3) of our ‘fruits’ list, which only has three elements. To avoid IndexError
s, always ensure that the index you’re trying to access exists in your list. One way to ensure this is to first find the length of a list with len() before accessing the given element.
Best Practices
Here are some best practices to keep in mind when working with Python lists:
- Avoid modifying a list while iterating over it. This can lead to unexpected behavior and bugs that are hard to track down.
Use list comprehensions for readability. List comprehensions can make your code more compact and easier to read, especially when you’re performing the same operation on every element in a list.
Choose the right data structure. While lists are versatile, other data structures like tuples or sets might be more appropriate depending on your needs.
By understanding these common issues and best practices, you can avoid many of the pitfalls associated with Python lists and write more robust and efficient code.
The Fundamentals of Python Lists
Before we delve deeper into Python lists, let’s understand some fundamental concepts related to them. Understanding these concepts will make it easier to work with Python lists and help you write more efficient code.
Mutability
In Python, lists are mutable. This means that we can change their content without changing their identity. You can modify a list by adding, removing, or changing elements.
fruits = ['apple', 'banana', 'cherry']
fruits[1] = 'mango'
print(fruits)
# Output:
# ['apple', 'mango', 'cherry']
In the above example, we replaced the second element of the ‘fruits’ list. The list ‘fruits’ still refers to the same list, but the list’s content has changed.
Ordering
Lists in Python are ordered. This means that the elements in a list have a specific order that is maintained. The order of elements in a list is the order in which they were added, starting from index 0.
Indexing
Python lists are indexed, meaning you can access any item in the list by its position in the list. Python uses zero-based indexing, so the first item is at index 0, the second item is at index 1, and so on.
print(fruits[0])
# Output:
# 'apple'
In the example above, we accessed the first element of our ‘fruits’ list, which is ‘apple’.
Underlying Data Structure
Python lists are implemented as dynamic arrays in the background. This means that they automatically resize themselves as elements are added or removed. This makes lists efficient for most operations, but operations that change the size of the list (like append()
or pop()
) can be slower because they may require reallocating memory and copying the entire list.
Understanding these fundamental concepts will help you work more effectively with Python lists and understand why they behave the way they do.
Python Lists in the Real World
Python lists are not just theoretical constructs, but practical tools that are widely used in real-world applications. They are often used in larger scripts or projects where managing and manipulating data is crucial.
For instance, in data analysis, Python lists are used to store and manipulate data, while in web development, they can be used to manage user inputs, store data from databases, and much more.
Exploring Further: List Comprehension, Lambda Functions, and More
While we’ve covered a lot of ground in this guide, there’s still plenty more to learn about Python lists and related concepts. For example, list comprehension is a powerful tool that allows you to create new lists based on existing ones in a single, succinct line of code.
Lambda functions, on the other hand, are small, anonymous functions that can be incredibly useful when working with lists, especially in conjunction with functions like filter()
and map()
.
Other data structures, such as sets, tuples, and dictionaries, also play a crucial role in Python programming. Each of these structures has its own strengths and weaknesses, and understanding when to use each one can make your code more efficient and readable.
Resources for Deeper Understanding
If you want to explore these topics further, there are many excellent resources available. Here are some to get you started:
- Click here for a comprehensive guide on data types in Python, including integers, floats, strings, lists, tuples, sets, and dictionaries.
How to Flatten a Python Nested List by IOFlood: Explores different methods to flatten a nested list in Python, including ‘For Loops, ‘list comprehension’, and ‘recursion’.
Python List Methods Command Guide: This article walks you through the various methods available for Python lists. It also talks about list concepts such as manipulation, addition, removal, and search operations.
W3Schools Python Lists Tutorial: This tutorial on W3Schools provides a beginner-friendly introduction to lists in Python.
Python Documentation on Data Structures: The official Python documentation offers a comprehensive guide on data structures.
Programiz Python List Tutorial: Programiz offers a tutorial on Python lists.
Remember, the key to mastering Python lists, like any programming concept, is practice. Don’t be afraid to experiment with different operations and techniques, and always be curious about how things work under the hood.
Wrapping Up: Python Lists Decoded
Python lists, an integral part of the Python programming language, are versatile and powerful tools for handling collections of items.
We’ve delved into the nitty-gritty of Python lists, from the basics of creating and manipulating lists to more advanced operations like list slicing, list comprehensions, and lambda functions. We’ve also covered common issues, such as IndexError
s, and provided best practices to avoid these pitfalls.
We’ve explored alternative data structures like tuples and sets, which can be more suitable for certain situations. Tuples, being immutable, are ideal for storing data that shouldn’t change, whereas sets, with their unique elements, are perfect for eliminating duplicates.
Data Structure | Mutable | Ordered | Use Case |
---|---|---|---|
List | Yes | Yes | When you need a general-purpose, flexible, and mutable collection of items. |
Tuple | No | Yes | When you need an immutable collection of items. |
Set | Yes | No | When you need a collection of unique items. |
Remember, the key to mastering Python lists, like any programming concept, is practice. So, keep experimenting, keep learning, and most importantly, keep coding!